Understanding the Role of Middleware in Applications
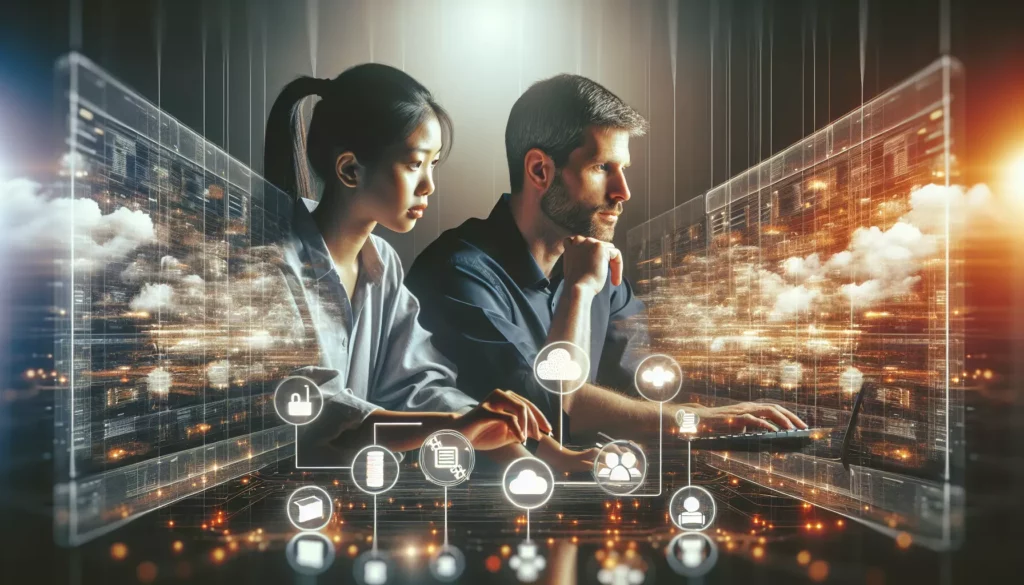
In the world of software development, middleware plays a crucial role in connecting various components of an application, facilitating communication between different systems, and enhancing overall functionality. As we delve into the intricacies of middleware, we’ll explore its importance, types, and practical applications in modern software architecture. This comprehensive guide will help both beginners and experienced developers gain a deeper understanding of middleware and its significance in building robust, scalable applications.
What is Middleware?
Middleware is a software layer that sits between the operating system and the applications running on it. It acts as a bridge, facilitating communication and data management between disparate systems, applications, and software components. Essentially, middleware provides a set of common services and capabilities to applications, beyond what’s offered by the operating system, making it easier for developers to focus on the specific functionality of their applications.
Key Characteristics of Middleware:
- Interoperability: Enables different systems and applications to work together seamlessly.
- Scalability: Supports the growth of applications and systems without compromising performance.
- Abstraction: Hides the complexities of underlying systems from developers and end-users.
- Reusability: Provides common services that can be used across multiple applications.
- Flexibility: Allows for easy integration of new technologies and services.
Types of Middleware
Middleware comes in various forms, each designed to address specific needs in application development and system integration. Let’s explore some of the most common types:
1. Message-Oriented Middleware (MOM)
Message-Oriented Middleware facilitates communication between distributed systems through message passing. It allows applications to send and receive messages asynchronously, enabling loose coupling between components.
Key features:
- Asynchronous communication
- Message queuing
- Guaranteed message delivery
- Load balancing
Examples of MOM include Apache Kafka, RabbitMQ, and IBM MQ.
2. Object Request Broker (ORB)
Object Request Brokers manage communication between objects in a distributed computing environment. They allow objects to make requests and receive responses transparently, regardless of their location or programming language.
Key features:
- Language and platform independence
- Location transparency
- Object lifecycle management
- Remote method invocation
Common ORB implementations include CORBA (Common Object Request Broker Architecture) and Java RMI (Remote Method Invocation).
3. Remote Procedure Call (RPC)
RPC middleware enables a program to execute a procedure or function on a remote computer as if it were a local call. This type of middleware simplifies the development of distributed applications by abstracting the complexity of network communication.
Key features:
- Transparent remote execution
- Synchronous communication
- Protocol independence
- Error handling and recovery
Examples of RPC middleware include gRPC, XML-RPC, and JSON-RPC.
4. Database Middleware
Database middleware provides a layer of abstraction between applications and databases, allowing for easier management of database connections, query optimization, and data access.
Key features:
- Connection pooling
- Query caching
- Load balancing
- Data transformation
Examples include ODBC (Open Database Connectivity) and JDBC (Java Database Connectivity).
5. Application Server Middleware
Application server middleware provides a runtime environment for applications, managing resources, security, and communication between different tiers of an application.
Key features:
- Resource management
- Transaction management
- Security services
- Load balancing and clustering
Popular application servers include Apache Tomcat, JBoss, and WebSphere.
6. Web Server Middleware
Web server middleware handles HTTP requests and responses, serving static content, and forwarding dynamic requests to application servers.
Key features:
- HTTP request handling
- Static content caching
- SSL/TLS encryption
- URL rewriting and redirection
Common web servers include Apache HTTP Server, Nginx, and Microsoft IIS.
The Importance of Middleware in Modern Applications
Middleware plays a critical role in modern application development and deployment for several reasons:
1. Simplifying Complex Systems
As applications grow in complexity, middleware helps manage the interactions between various components, reducing the burden on developers to handle low-level system integration tasks.
2. Enabling Microservices Architecture
Middleware is essential in microservices-based architectures, facilitating communication between services, managing service discovery, and handling load balancing.
3. Improving Scalability and Performance
By providing services like caching, connection pooling, and load balancing, middleware helps applications scale more efficiently and perform better under high loads.
4. Enhancing Security
Many middleware solutions offer built-in security features, such as authentication, authorization, and encryption, helping to protect applications and data from various threats.
5. Supporting Cloud-Native Development
Middleware plays a crucial role in cloud-native applications, providing the necessary abstraction and services to build and deploy applications in cloud environments.
Implementing Middleware in Your Applications
Now that we understand the importance and types of middleware, let’s explore how to implement middleware in your applications. We’ll use Node.js with Express as an example, as it’s a popular choice for building web applications and APIs.
Basic Middleware in Express
In Express, middleware functions are functions that have access to the request object (req), the response object (res), and the next middleware function in the application’s request-response cycle, commonly denoted by a variable named next.
Here’s a simple example of a middleware function:
const express = require('express');
const app = express();
const myMiddleware = (req, res, next) => {
console.log('This is a middleware function');
next(); // Call the next middleware function
};
app.use(myMiddleware);
app.get('/', (req, res) => {
res.send('Hello, World!');
});
app.listen(3000, () => {
console.log('Server is running on port 3000');
});
In this example, myMiddleware is a simple middleware function that logs a message to the console and then calls the next() function to pass control to the next middleware.
Error-Handling Middleware
Error-handling middleware functions are defined with four arguments instead of three, specifically with the signature (err, req, res, next):
app.use((err, req, res, next) => {
console.error(err.stack);
res.status(500).send('Something broke!');
});
Third-Party Middleware
Express has a robust ecosystem of third-party middleware that you can use to add functionality to your application. Here are a few examples:
1. Body Parser
Body-parser is middleware to parse incoming request bodies:
const bodyParser = require('body-parser');
app.use(bodyParser.json()); // for parsing application/json
app.use(bodyParser.urlencoded({ extended: true })); // for parsing application/x-www-form-urlencoded
2. CORS
CORS middleware can be used to enable Cross-Origin Resource Sharing:
const cors = require('cors');
app.use(cors());
3. Morgan
Morgan is a popular logging middleware:
const morgan = require('morgan');
app.use(morgan('combined'));
Best Practices for Using Middleware
When working with middleware, keep these best practices in mind:
1. Order Matters
The order in which you define middleware is important. Middleware functions are executed sequentially, so place them in the order you want them to run.
2. Use Next() Properly
Always call next() when you’re done with your middleware function, unless you’re ending the request-response cycle by sending a response to the client.
3. Error Handling
Implement proper error handling middleware to catch and process errors effectively.
4. Keep It Modular
Create separate files for complex middleware functions to keep your main application file clean and organized.
5. Be Mindful of Performance
While middleware can add powerful functionality to your application, be aware of the performance impact of each middleware you add.
Conclusion
Middleware is a fundamental concept in modern application development, providing a powerful way to extend and enhance the functionality of your applications. By understanding the different types of middleware and how to implement them effectively, you can build more robust, scalable, and maintainable applications.
As you continue your journey in software development, remember that middleware is not just a tool but a design philosophy. It encourages modular, loosely coupled architectures that can adapt to changing requirements and scale with your application’s needs.
Whether you’re building a simple web application or a complex distributed system, mastering the use of middleware will be invaluable in your career as a developer. Keep exploring different middleware solutions, experiment with implementing your own custom middleware, and always strive to understand how middleware can solve the unique challenges of your projects.
By leveraging the power of middleware, you’ll be well-equipped to tackle the complexities of modern software development and create applications that are not only functional but also efficient, secure, and scalable.