Understanding the Role of a Tech Lead: A Comprehensive Guide
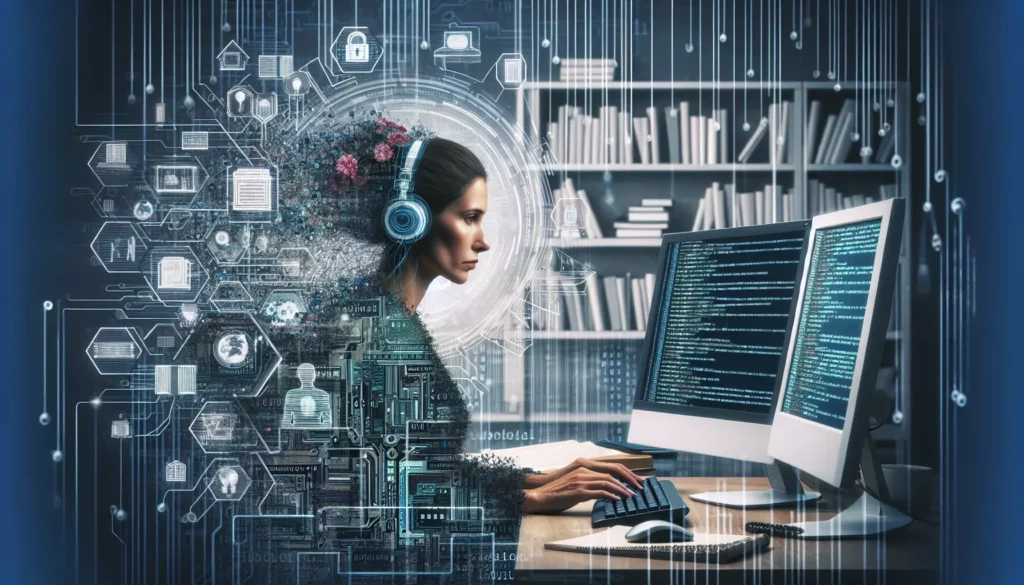
In the fast-paced world of software development, the role of a Tech Lead has become increasingly crucial. As organizations strive to innovate and stay competitive, they rely on skilled professionals who can bridge the gap between technical expertise and leadership. This article will delve deep into the multifaceted role of a Tech Lead, exploring their responsibilities, skills required, and the impact they have on development teams and projects.
What is a Tech Lead?
A Tech Lead, short for Technical Lead, is a senior-level position in software development that combines technical expertise with leadership and management skills. They are responsible for guiding the technical direction of a project or team while also contributing to the actual development work. Tech Leads serve as a bridge between management and developers, ensuring that technical decisions align with business goals and that the team operates efficiently.
Key Responsibilities of a Tech Lead
- Technical Direction: Setting the technical vision and making key architectural decisions
- Team Leadership: Mentoring and guiding team members, fostering a positive work environment
- Project Management: Overseeing project timelines, resource allocation, and risk management
- Code Quality: Ensuring high standards of code quality and implementing best practices
- Communication: Acting as a liaison between technical teams and non-technical stakeholders
- Problem Solving: Addressing complex technical challenges and finding innovative solutions
The Technical Expertise of a Tech Lead
At the core of a Tech Lead’s role is their deep technical knowledge. They are expected to have a comprehensive understanding of various programming languages, frameworks, and development methodologies. This expertise allows them to make informed decisions about technology choices, architecture design, and coding standards.
Programming Languages and Frameworks
A Tech Lead should be proficient in multiple programming languages and frameworks relevant to their field. For example, in web development, this might include:
- Frontend: JavaScript, React, Angular, Vue.js
- Backend: Python, Java, Node.js, Ruby on Rails
- Database: SQL, NoSQL (MongoDB, Cassandra)
- Cloud Technologies: AWS, Azure, Google Cloud Platform
Here’s an example of how a Tech Lead might review and suggest improvements to a piece of code:
// Original code
function calculateTotal(items) {
let total = 0;
for (let i = 0; i < items.length; i++) {
total += items[i].price;
}
return total;
}
// Tech Lead's improved version
const calculateTotal = (items) => items.reduce((total, item) => total + item.price, 0);
In this example, the Tech Lead has refactored the code to use modern JavaScript features, making it more concise and efficient.
System Architecture and Design
Tech Leads are often responsible for making high-level architectural decisions. This includes:
- Designing scalable and maintainable systems
- Choosing appropriate design patterns
- Ensuring system security and performance
- Planning for future growth and adaptability
For instance, a Tech Lead might decide to implement a microservices architecture to improve scalability and maintainability of a large application:
<!-- Simplified microservices architecture diagram -->
<mxGraphModel>
<root>
<mxCell id="0"/>
<mxCell id="1" parent="0"/>
<mxCell id="2" value="API Gateway" style="rounded=1;" vertex="1" parent="1">
<mxGeometry x="300" y="100" width="120" height="60" as="geometry"/>
</mxCell>
<mxCell id="3" value="User Service" style="rounded=1;" vertex="1" parent="1">
<mxGeometry x="100" y="200" width="120" height="60" as="geometry"/>
</mxCell>
<mxCell id="4" value="Product Service" style="rounded=1;" vertex="1" parent="1">
<mxGeometry x="300" y="200" width="120" height="60" as="geometry"/>
</mxCell>
<mxCell id="5" value="Order Service" style="rounded=1;" vertex="1" parent="1">
<mxGeometry x="500" y="200" width="120" height="60" as="geometry"/>
</mxCell>
</root>
</mxGraphModel>
Leadership and Management Skills
While technical skills are crucial, a Tech Lead’s role extends far beyond coding. They must possess strong leadership and management skills to effectively guide their team and ensure project success.
Team Management
Tech Leads are responsible for managing and mentoring their team members. This includes:
- Assigning tasks and responsibilities
- Providing guidance and support
- Conducting code reviews and giving feedback
- Identifying and nurturing talent within the team
- Resolving conflicts and maintaining a positive team dynamic
Effective team management often involves creating and maintaining project management tools. For example, a Tech Lead might set up a Kanban board using tools like Jira or Trello:
<!-- Simplified Kanban board structure -->
<table>
<tr>
<th>To Do</th>
<th>In Progress</th>
<th>Review</th>
<th>Done</th>
</tr>
<tr>
<td>
<ul>
<li>Task 1</li>
<li>Task 2</li>
</ul>
</td>
<td>
<ul>
<li>Task 3</li>
</ul>
</td>
<td>
<ul>
<li>Task 4</li>
</ul>
</td>
<td>
<ul>
<li>Task 5</li>
<li>Task 6</li>
</ul>
</td>
</tr>
</table>
Project Management
Tech Leads play a crucial role in project management, ensuring that technical projects are delivered on time and within scope. This involves:
- Breaking down projects into manageable tasks
- Estimating time and resources required for each task
- Tracking progress and identifying potential bottlenecks
- Managing project risks and dependencies
- Adapting to changes in project requirements or timelines
A Tech Lead might use project management methodologies like Agile or Scrum to effectively manage projects. Here’s an example of a sprint planning document:
<!-- Sprint Planning Document -->
Sprint Goal: Implement user authentication system
User Stories:
1. As a user, I want to be able to register for an account
2. As a user, I want to be able to log in to my account
3. As a user, I want to be able to reset my password
Tasks:
1. Design database schema for user accounts
2. Implement registration API endpoint
3. Create registration form frontend
4. Implement login API endpoint
5. Create login form frontend
6. Implement password reset functionality
7. Write unit tests for authentication system
8. Conduct security review of authentication system
Estimated Story Points: 21
Sprint Duration: 2 weeks
Team Capacity: 3 developers
Communication Skills
Effective communication is a cornerstone of the Tech Lead role. They must be able to:
- Clearly articulate technical concepts to non-technical stakeholders
- Facilitate discussions and decision-making within the team
- Present project updates and technical proposals to management
- Write clear and concise documentation
- Provide constructive feedback to team members
For example, a Tech Lead might create a technical specification document to communicate a new feature to both the development team and stakeholders:
<!-- Technical Specification Document -->
Feature: User Authentication System
1. Overview
The user authentication system will allow users to register, log in, and manage their accounts securely.
2. Technical Stack
- Backend: Node.js with Express.js
- Database: MongoDB
- Frontend: React.js
- Authentication: JWT (JSON Web Tokens)
3. API Endpoints
- POST /api/auth/register
- POST /api/auth/login
- POST /api/auth/reset-password
- GET /api/auth/user
4. Security Measures
- Password hashing using bcrypt
- HTTPS for all API calls
- Rate limiting to prevent brute force attacks
- Input validation and sanitization
5. Performance Considerations
- Implement caching for frequently accessed user data
- Use database indexing for efficient queries
6. Testing Strategy
- Unit tests for all authentication functions
- Integration tests for API endpoints
- End-to-end tests for user flows
7. Deployment Plan
- Staged rollout starting with beta users
- Monitoring and logging for early detection of issues
8. Future Enhancements
- Two-factor authentication
- OAuth integration for social login
Problem Solving and Decision Making
Tech Leads are often called upon to solve complex technical problems and make critical decisions that impact the entire project or product. This requires:
- Analytical thinking and logical reasoning
- The ability to consider multiple solutions and their trade-offs
- Quick thinking and decisiveness in urgent situations
- Balancing technical ideals with practical constraints
- Staying up-to-date with industry trends and emerging technologies
For instance, a Tech Lead might need to decide between two competing technologies for a new project. They might create a decision matrix to evaluate the options:
<!-- Technology Decision Matrix -->
<table>
<tr>
<th>Criteria</th>
<th>Weight</th>
<th>Technology A</th>
<th>Technology B</th>
</tr>
<tr>
<td>Performance</td>
<td>0.3</td>
<td>8</td>
<td>7</td>
</tr>
<tr>
<td>Scalability</td>
<td>0.25</td>
<td>9</td>
<td>8</td>
</tr>
<tr>
<td>Learning Curve</td>
<td>0.2</td>
<td>6</td>
<td>8</td>
</tr>
<tr>
<td>Community Support</td>
<td>0.15</td>
<td>7</td>
<td>9</td>
</tr>
<tr>
<td>Cost</td>
<td>0.1</td>
<td>8</td>
<td>6</td>
</tr>
<tr>
<td><strong>Weighted Score</strong></td>
<td></td>
<td><strong>7.75</strong></td>
<td><strong>7.55</strong></td>
</tr>
</table>
Continuous Learning and Adaptation
The tech industry is known for its rapid pace of change, and Tech Leads must stay ahead of the curve. This involves:
- Regularly updating their technical skills
- Exploring new technologies and methodologies
- Attending conferences and workshops
- Participating in online communities and forums
- Encouraging a culture of learning within their team
A Tech Lead might create a learning roadmap for their team to ensure everyone is continually improving their skills:
<!-- Team Learning Roadmap -->
Q1 Focus: Microservices Architecture
- Week 1-2: Introduction to microservices concepts
- Week 3-4: Designing microservices
- Week 5-6: Implementing microservices with Docker and Kubernetes
- Week 7-8: Microservices testing and deployment strategies
Q2 Focus: Advanced JavaScript and React
- Week 1-2: ES6+ features and best practices
- Week 3-4: React hooks and context API
- Week 5-6: State management with Redux
- Week 7-8: Performance optimization in React applications
Q3 Focus: Cloud Technologies
- Week 1-2: Introduction to cloud computing concepts
- Week 3-4: AWS fundamentals
- Week 5-6: Serverless architecture with AWS Lambda
- Week 7-8: CI/CD pipelines in the cloud
Q4 Focus: Machine Learning and AI
- Week 1-2: Introduction to machine learning concepts
- Week 3-4: Python for data science and machine learning
- Week 5-6: Implementing basic machine learning models
- Week 7-8: Integrating machine learning models into applications
Challenges Faced by Tech Leads
While the role of a Tech Lead is rewarding, it comes with its own set of challenges:
Balancing Technical Work and Leadership
Tech Leads often struggle to find the right balance between hands-on coding and leadership responsibilities. They need to contribute technically while also guiding the team and managing projects.
Managing Different Personalities
Development teams often consist of individuals with diverse personalities and working styles. Tech Leads must be able to manage these differences effectively to maintain team harmony and productivity.
Keeping Up with Rapid Technological Changes
The fast-paced nature of the tech industry means that Tech Leads must constantly update their knowledge and skills to remain effective in their role.
Dealing with Ambiguity
Tech Leads often have to make decisions with incomplete information or unclear requirements. They need to be comfortable with ambiguity and able to guide their team through uncertain situations.
Managing Stakeholder Expectations
Tech Leads must balance the expectations of various stakeholders, including management, clients, and team members. This often involves negotiating timelines, resources, and technical decisions.
The Impact of Tech Leads on Development Teams
Effective Tech Leads can have a significant positive impact on their teams and organizations:
Improved Code Quality and Technical Excellence
By setting high standards and providing guidance, Tech Leads help ensure that their team produces high-quality, maintainable code. This leads to more robust and reliable software products.
Enhanced Team Productivity
Through effective project management and team leadership, Tech Leads can significantly boost their team’s productivity. They help remove obstacles, streamline processes, and ensure that team members are working on the right tasks at the right time.
Fostering Innovation
Tech Leads encourage their teams to explore new technologies and approaches, fostering a culture of innovation. This can lead to breakthrough solutions and keep the organization at the forefront of technological advancements.
Better Alignment with Business Goals
By bridging the gap between technical teams and business stakeholders, Tech Leads ensure that technical decisions and development efforts align with overall business objectives.
Career Development for Team Members
Tech Leads play a crucial role in mentoring and developing the skills of their team members. This not only improves the team’s capabilities but also helps in retention of talented developers.
Preparing for a Tech Lead Role
For developers aspiring to become Tech Leads, here are some steps to prepare for the role:
Develop a Broad Technical Skill Set
While it’s important to have deep expertise in certain areas, Tech Leads should also have a broad understanding of various technologies and development practices. This might involve:
- Learning multiple programming languages
- Gaining experience with different frameworks and tools
- Understanding various architectural patterns and their trade-offs
- Familiarizing yourself with DevOps practices and tools
Gain Project Management Experience
Look for opportunities to manage small projects or take on leadership roles within your current team. This could include:
- Volunteering to lead a sprint or a specific feature development
- Organizing and running team meetings
- Creating project plans and timelines
- Managing dependencies and coordinating with other teams
Improve Your Communication Skills
Effective communication is crucial for Tech Leads. You can improve these skills by:
- Practicing presenting technical concepts to non-technical audiences
- Writing clear and concise documentation
- Participating in or leading code reviews
- Engaging in discussions and debates about technical decisions
Develop Your Leadership Skills
Leadership skills are essential for Tech Leads. You can develop these by:
- Mentoring junior developers
- Taking on more responsibilities in your current role
- Reading books on leadership and management
- Attending leadership workshops or courses
Stay Updated with Industry Trends
Tech Leads need to stay ahead of technological trends. You can do this by:
- Following tech blogs and news sites
- Attending conferences and meetups
- Participating in online tech communities
- Experimenting with new technologies in side projects
Conclusion
The role of a Tech Lead is multifaceted and challenging, requiring a unique blend of technical expertise, leadership skills, and business acumen. Tech Leads play a crucial role in guiding development teams, ensuring technical excellence, and driving innovation within their organizations.
As the tech industry continues to evolve, the importance of effective Tech Leads will only grow. For developers aspiring to this role, focusing on broadening technical skills, developing leadership abilities, and staying current with industry trends will be key to success.
Whether you’re currently in a Tech Lead position, aspiring to become one, or working with Tech Leads, understanding the complexities and responsibilities of this role is crucial for success in the modern software development landscape.