Understanding the Principles of Clean Code: A Comprehensive Guide
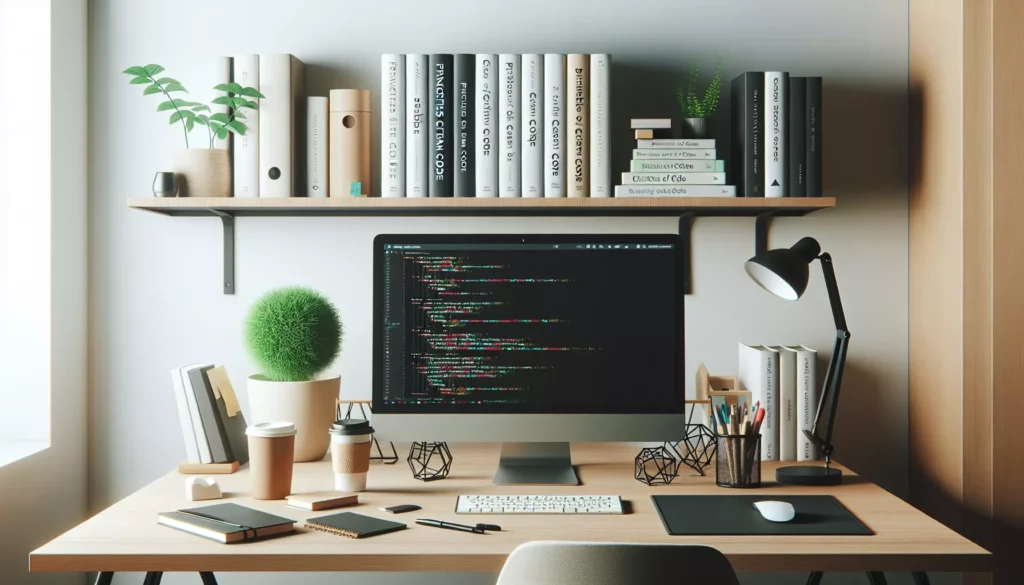
In the world of software development, writing code that works is just the beginning. As projects grow in size and complexity, the importance of writing clean, maintainable, and efficient code becomes paramount. This is where the principles of clean code come into play. In this comprehensive guide, we’ll explore what clean code is, why it matters, and how you can apply its principles to elevate your coding skills to the next level.
What is Clean Code?
Clean code is a set of practices and principles aimed at producing software that is easy to read, understand, and maintain. It goes beyond just making your code functional; it’s about crafting code that is elegant, efficient, and sustainable over time. Robert C. Martin, also known as “Uncle Bob,” popularized the concept in his book “Clean Code: A Handbook of Agile Software Craftsmanship.”
At its core, clean code is characterized by:
- Readability
- Simplicity
- Maintainability
- Testability
- Efficiency
Why is Clean Code Important?
You might wonder, “If my code works, why should I care about making it ‘clean’?” The answer lies in the long-term benefits that clean code brings to both individual developers and development teams:
- Improved Collaboration: Clean code is easier for other developers to understand and work with, facilitating smoother collaboration in team environments.
- Reduced Bugs: Well-structured, clean code is less prone to bugs and easier to debug when issues do arise.
- Easier Maintenance: As projects evolve, clean code is much easier to update, refactor, and maintain over time.
- Enhanced Productivity: While writing clean code might take a bit more time initially, it saves significant time and effort in the long run.
- Better Scalability: Clean code provides a solid foundation for scaling your project as it grows in size and complexity.
Key Principles of Clean Code
Let’s dive into some of the fundamental principles that guide the creation of clean code:
1. Meaningful Names
One of the most basic yet crucial aspects of clean code is using meaningful and intention-revealing names for variables, functions, classes, and other elements. Good names should:
- Be descriptive and self-explanatory
- Avoid abbreviations or cryptic shorthand
- Be pronounceable and searchable
- Follow consistent naming conventions
Example of poor naming:
int d; // elapsed time in days
void fp(String n) {
// Function to process name
// ...
}
Improved version with meaningful names:
int elapsedTimeInDays;
void processName(String fullName) {
// Function to process name
// ...
}
2. Functions Should Do One Thing
Functions should be small, focused, and do only one thing. This principle, known as the Single Responsibility Principle (SRP), makes code more modular, easier to understand, and simpler to test. When a function does more than one thing, it becomes harder to comprehend and maintain.
Example of a function doing multiple things:
void processAndSaveUserData(User user) {
// Validate user data
if (user.getName() == null || user.getEmail() == null) {
throw new IllegalArgumentException("Invalid user data");
}
// Format user data
String formattedName = user.getName().toUpperCase();
String formattedEmail = user.getEmail().toLowerCase();
// Save user to database
database.save(new User(formattedName, formattedEmail));
// Send welcome email
emailService.sendWelcomeEmail(user.getEmail());
}
Improved version with separate functions:
void processAndSaveUserData(User user) {
validateUserData(user);
User formattedUser = formatUserData(user);
saveUserToDatabase(formattedUser);
sendWelcomeEmail(user.getEmail());
}
void validateUserData(User user) {
if (user.getName() == null || user.getEmail() == null) {
throw new IllegalArgumentException("Invalid user data");
}
}
User formatUserData(User user) {
String formattedName = user.getName().toUpperCase();
String formattedEmail = user.getEmail().toLowerCase();
return new User(formattedName, formattedEmail);
}
void saveUserToDatabase(User user) {
database.save(user);
}
void sendWelcomeEmail(String email) {
emailService.sendWelcomeEmail(email);
}
3. DRY (Don’t Repeat Yourself)
The DRY principle advocates for reducing repetition in code. When you find yourself writing similar code in multiple places, it’s time to abstract that logic into a reusable function or class. This not only makes your code more maintainable but also reduces the chance of errors when updates are needed.
Example of repetitive code:
double calculateCircleArea(double radius) {
return 3.14159 * radius * radius;
}
double calculateCylinderVolume(double radius, double height) {
return 3.14159 * radius * radius * height;
}
Improved version applying DRY:
final double PI = 3.14159;
double calculateCircleArea(double radius) {
return PI * radius * radius;
}
double calculateCylinderVolume(double radius, double height) {
return calculateCircleArea(radius) * height;
}
4. Comments and Documentation
While clean code should be self-explanatory to a large extent, comments and documentation still play a crucial role. However, it’s important to use them judiciously:
- Use comments to explain why something is done, not what is being done.
- Keep comments up-to-date with code changes.
- Use clear and concise language in comments.
- Leverage documentation for APIs and complex algorithms.
Example of poor commenting:
// This function calculates the area of a circle
double calculateCircleArea(double radius) {
// Multiply pi by radius squared
return 3.14159 * radius * radius;
}
Improved version with meaningful comments:
/**
* Calculates the area of a circle.
*
* @param radius The radius of the circle
* @return The area of the circle
*/
double calculateCircleArea(double radius) {
// Using a simplified value of pi for demonstration purposes
// For more precise calculations, consider using Math.PI
return 3.14159 * radius * radius;
}
5. Error Handling
Proper error handling is a crucial aspect of clean code. It involves:
- Using exceptions for exceptional cases, not for control flow.
- Providing informative error messages.
- Catching and handling exceptions at the appropriate level.
- Avoiding empty catch blocks.
Example of poor error handling:
void processFile(String filename) {
try {
// Read and process file
} catch (Exception e) {
// Do nothing
}
}
Improved version with better error handling:
void processFile(String filename) throws IOException {
try {
// Read and process file
} catch (FileNotFoundException e) {
throw new IOException("File not found: " + filename, e);
} catch (IOException e) {
throw new IOException("Error processing file: " + filename, e);
}
}
6. Code Formatting and Structure
Consistent code formatting and structure contribute significantly to readability. This includes:
- Consistent indentation
- Proper use of whitespace
- Logical grouping of related code
- Keeping lines of code at a reasonable length
Many modern IDEs offer automatic formatting features that can help maintain consistent style across your codebase.
7. SOLID Principles
The SOLID principles are a set of five design principles that, when applied together, make software designs more understandable, flexible, and maintainable:
- Single Responsibility Principle (SRP)
- Open/Closed Principle (OCP)
- Liskov Substitution Principle (LSP)
- Interface Segregation Principle (ISP)
- Dependency Inversion Principle (DIP)
Let’s briefly explore each of these principles:
Single Responsibility Principle (SRP)
This principle states that a class should have only one reason to change. In other words, a class should have a single, well-defined responsibility.
Example violating SRP:
class User {
private String name;
private String email;
// User-related methods
public void saveToDatabase() {
// Logic to save user to database
}
public void sendEmail(String message) {
// Logic to send email
}
}
Improved version adhering to SRP:
class User {
private String name;
private String email;
// User-related methods
}
class UserRepository {
public void saveUser(User user) {
// Logic to save user to database
}
}
class EmailService {
public void sendEmail(String email, String message) {
// Logic to send email
}
}
Open/Closed Principle (OCP)
The OCP states that software entities (classes, modules, functions, etc.) should be open for extension but closed for modification. This means you should be able to extend a class’s behavior without modifying its existing code.
Example violating OCP:
class Rectangle {
private double width;
private double height;
// Constructor and getters/setters
}
class AreaCalculator {
public double calculateArea(Object shape) {
if (shape instanceof Rectangle) {
Rectangle rectangle = (Rectangle) shape;
return rectangle.getWidth() * rectangle.getHeight();
}
// Add more conditions for other shapes
return 0;
}
}
Improved version adhering to OCP:
interface Shape {
double calculateArea();
}
class Rectangle implements Shape {
private double width;
private double height;
// Constructor and getters/setters
@Override
public double calculateArea() {
return width * height;
}
}
class Circle implements Shape {
private double radius;
// Constructor and getter/setter
@Override
public double calculateArea() {
return Math.PI * radius * radius;
}
}
class AreaCalculator {
public double calculateArea(Shape shape) {
return shape.calculateArea();
}
}
Liskov Substitution Principle (LSP)
The LSP states that objects of a superclass should be replaceable with objects of its subclasses without affecting the correctness of the program. In other words, derived classes must be substitutable for their base classes.
Example violating LSP:
class Rectangle {
protected int width;
protected int height;
public void setWidth(int width) {
this.width = width;
}
public void setHeight(int height) {
this.height = height;
}
public int getArea() {
return width * height;
}
}
class Square extends Rectangle {
@Override
public void setWidth(int width) {
super.setWidth(width);
super.setHeight(width);
}
@Override
public void setHeight(int height) {
super.setWidth(height);
super.setHeight(height);
}
}
In this example, the Square class violates LSP because it changes the behavior of setWidth and setHeight methods, potentially breaking code that expects Rectangle behavior.
Improved version adhering to LSP:
interface Shape {
int getArea();
}
class Rectangle implements Shape {
protected int width;
protected int height;
public Rectangle(int width, int height) {
this.width = width;
this.height = height;
}
public int getArea() {
return width * height;
}
}
class Square implements Shape {
private int side;
public Square(int side) {
this.side = side;
}
public int getArea() {
return side * side;
}
}
Interface Segregation Principle (ISP)
The ISP states that no client should be forced to depend on methods it does not use. In other words, it’s better to have many smaller, specific interfaces rather than a few large, general-purpose interfaces.
Example violating ISP:
interface Worker {
void work();
void eat();
void sleep();
}
class Human implements Worker {
public void work() { /* ... */ }
public void eat() { /* ... */ }
public void sleep() { /* ... */ }
}
class Robot implements Worker {
public void work() { /* ... */ }
public void eat() { throw new UnsupportedOperationException(); }
public void sleep() { throw new UnsupportedOperationException(); }
}
Improved version adhering to ISP:
interface Workable {
void work();
}
interface Eatable {
void eat();
}
interface Sleepable {
void sleep();
}
class Human implements Workable, Eatable, Sleepable {
public void work() { /* ... */ }
public void eat() { /* ... */ }
public void sleep() { /* ... */ }
}
class Robot implements Workable {
public void work() { /* ... */ }
}
Dependency Inversion Principle (DIP)
The DIP states that high-level modules should not depend on low-level modules. Both should depend on abstractions. Additionally, abstractions should not depend on details; details should depend on abstractions.
Example violating DIP:
class LightBulb {
public void turnOn() {
// Turn on the light bulb
}
public void turnOff() {
// Turn off the light bulb
}
}
class Switch {
private LightBulb bulb;
public Switch() {
bulb = new LightBulb();
}
public void operate() {
// Toggle the light bulb
}
}
Improved version adhering to DIP:
interface Switchable {
void turnOn();
void turnOff();
}
class LightBulb implements Switchable {
public void turnOn() {
// Turn on the light bulb
}
public void turnOff() {
// Turn off the light bulb
}
}
class Switch {
private Switchable device;
public Switch(Switchable device) {
this.device = device;
}
public void operate() {
// Toggle the device
}
}
Tools and Practices for Maintaining Clean Code
While understanding the principles of clean code is crucial, implementing and maintaining these practices can be challenging. Fortunately, there are various tools and practices that can help you write and maintain clean code:
1. Code Linters
Code linters are tools that analyze your source code to flag programming errors, bugs, stylistic errors, and suspicious constructs. They can help enforce coding standards and identify potential issues before they become problems. Some popular linters include:
- ESLint for JavaScript
- Pylint for Python
- RuboCop for Ruby
- Checkstyle for Java
2. Automated Formatting Tools
These tools automatically format your code according to predefined style rules, ensuring consistency across your codebase. Examples include:
- Prettier for JavaScript, TypeScript, and more
- Black for Python
- gofmt for Go
3. Continuous Integration (CI)
CI systems can be configured to run linters, formatting checks, and automated tests every time code is pushed to the repository. This helps catch issues early and ensures that all code adheres to the project’s standards.
4. Code Reviews
Regular code reviews by peers can help maintain code quality, share knowledge, and catch issues that automated tools might miss. They also provide an opportunity for team members to learn from each other and discuss best practices.
5. Refactoring
Refactoring is the process of restructuring existing code without changing its external behavior. Regular refactoring helps keep code clean and manageable as projects evolve. Many IDEs offer automated refactoring tools that can assist in this process.
6. Documentation
While clean code should be largely self-documenting, maintaining up-to-date documentation for APIs, complex algorithms, and project architecture is still important. Tools like Javadoc, Sphinx, and Swagger can help generate and maintain documentation.
Challenges in Writing Clean Code
Despite its benefits, writing and maintaining clean code comes with its own set of challenges:
1. Time Constraints
In fast-paced development environments, there’s often pressure to deliver features quickly. This can lead to shortcuts and “quick fixes” that accumulate technical debt over time.
2. Legacy Code
Working with existing codebases that don’t follow clean code principles can be challenging. Refactoring legacy code takes time and carries the risk of introducing new bugs.
3. Team Alignment
Getting all team members to agree on and consistently apply clean code principles can be difficult, especially in larger teams or when working with developers of varying experience levels.
4. Overengineering
Sometimes, in an attempt to write clean code, developers may over-complicate simple solutions. It’s important to find the right balance between cleanliness and pragmatism.
5. Changing Requirements
As project requirements change, maintaining clean code can become challenging. What was once a clean, elegant solution may become convoluted as new features are added.
Conclusion
Clean code is not just a set of rules to follow; it’s a mindset and a commitment to craftsmanship in software development. By adhering to the principles of clean code, you can create software that is not only functional but also maintainable, scalable, and a joy to work with.
Remember that writing clean code is a skill that improves with practice. It requires constant learning, adaptation, and sometimes, tough decisions. But the long-term benefits—reduced bugs, improved collaboration, easier maintenance, and overall better software quality—make it a worthwhile endeavor.
As you continue your journey in software development, whether you’re preparing for technical interviews at top tech companies or working on personal projects, always strive to write clean, readable, and maintainable code. It’s an investment in your skills, your projects, and your career that will pay dividends for years to come.
Remember, the next person who reads your code might be you, six months from now. Write code that your future self will thank you for!