Understanding the += Operator in Python: A Comprehensive Guide
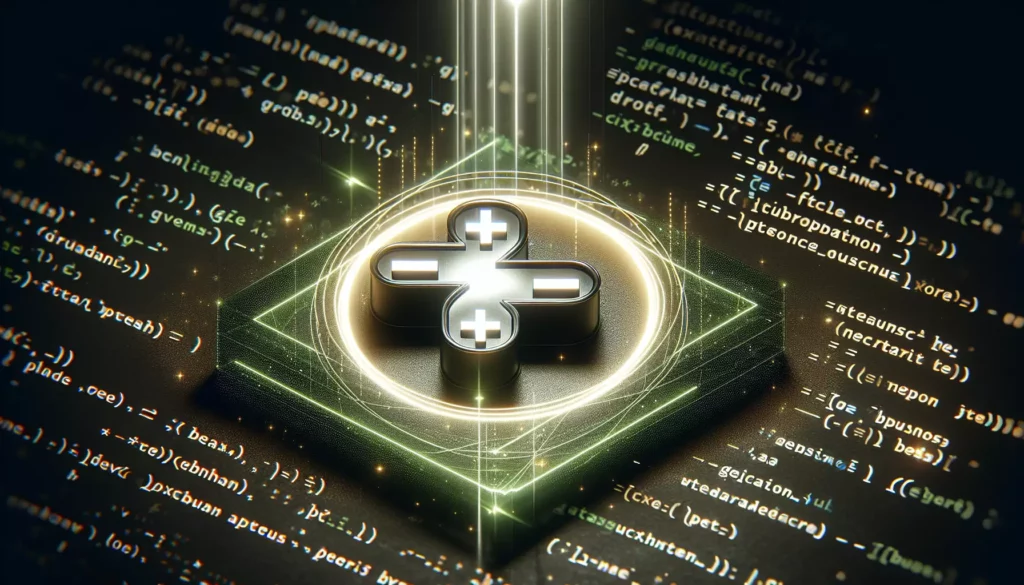
Python is known for its simplicity and readability, and one of the features that contributes to this is its use of concise operators. Among these, the += operator stands out as a particularly useful shorthand. In this comprehensive guide, we’ll dive deep into the meaning, usage, and intricacies of the += operator in Python.
What Does += Mean in Python?
The += operator in Python is a compound assignment operator. It combines addition and assignment into a single operation. Essentially, a += b
is shorthand for a = a + b
. This operator is not unique to Python and is found in many programming languages, but its implementation in Python has some interesting nuances.
Basic Usage of +=
Let’s start with a simple example to illustrate how += works:
x = 5
x += 3
print(x) # Output: 8
In this example, we start with x = 5
. Then, x += 3
adds 3 to the current value of x and assigns the result back to x. So, x becomes 8.
+= with Different Data Types
One of the powerful aspects of += in Python is that it works with various data types, not just numbers. Let’s explore how it behaves with different types:
1. Integers and Floats
We’ve already seen how += works with integers. It works similarly with floats:
y = 3.14
y += 2.86
print(y) # Output: 6.0
2. Strings
With strings, += concatenates:
greeting = "Hello, "
greeting += "World!"
print(greeting) # Output: Hello, World!
3. Lists
For lists, += extends the list:
fruits = ["apple", "banana"]
fruits += ["cherry", "date"]
print(fruits) # Output: ['apple', 'banana', 'cherry', 'date']
4. Tuples
With tuples, += creates a new tuple:
coordinates = (1, 2)
coordinates += (3, 4)
print(coordinates) # Output: (1, 2, 3, 4)
The Magic Behind +=: The __iadd__ Method
In Python, the += operator is implemented using the __iadd__
special method. When you use +=, Python looks for this method in the object’s class. If it’s not found, Python falls back to the __add__
method.
Here’s a simple class that implements __iadd__
:
class Counter:
def __init__(self, value=0):
self.value = value
def __iadd__(self, other):
self.value += other
return self
counter = Counter(5)
counter += 3
print(counter.value) # Output: 8
In this example, counter += 3
calls the __iadd__
method, which adds 3 to the counter’s value.
Performance Considerations
The += operator can have performance benefits, especially when working with large data structures. For mutable objects like lists, += modifies the object in-place, which is more efficient than creating a new object and reassigning it.
import timeit
def using_plus_equals():
lst = []
for i in range(1000):
lst += [i]
def using_extend():
lst = []
for i in range(1000):
lst.extend([i])
print(timeit.timeit(using_plus_equals, number=10000))
print(timeit.timeit(using_extend, number=10000))
You’ll find that using_plus_equals()
and using_extend()
have similar performance, as += for lists is implemented using the extend method internally.
Common Pitfalls and Gotchas
While += is generally straightforward, there are some situations where it might not behave as you expect:
1. Immutable Objects
For immutable objects like strings, integers, and tuples, += creates a new object. This can lead to unexpected behavior in certain situations:
def modify_tuple(t):
t += (4, 5)
print("Inside function:", t)
original = (1, 2, 3)
modify_tuple(original)
print("Outside function:", original)
# Output:
# Inside function: (1, 2, 3, 4, 5)
# Outside function: (1, 2, 3)
In this example, the original tuple remains unchanged because += created a new tuple inside the function.
2. Unexpected Type Coercion
Be careful when using += with different types:
x = 5
x += 3.14
print(x) # Output: 8.14
y = "5"
y += 3 # This will raise a TypeError
In the first case, x is converted to a float. In the second case, you can’t add an integer to a string, so it raises an error.
+= in Loops
The += operator is particularly useful in loops. Here’s an example of calculating the sum of numbers:
numbers = [1, 2, 3, 4, 5]
sum = 0
for num in numbers:
sum += num
print(sum) # Output: 15
This is more concise and often more readable than writing sum = sum + num
in each iteration.
+= vs. extend() for Lists
For lists, += is equivalent to the extend() method:
list1 = [1, 2, 3]
list2 = [4, 5, 6]
# Using +=
list1 += list2
print(list1) # Output: [1, 2, 3, 4, 5, 6]
# Using extend()
list1 = [1, 2, 3]
list1.extend(list2)
print(list1) # Output: [1, 2, 3, 4, 5, 6]
Both approaches modify the original list in-place and have similar performance characteristics.
+= in Multithreaded Environments
When working with multiple threads, be cautious with +=. It’s not an atomic operation, which means it’s not thread-safe:
import threading
counter = 0
def increment():
global counter
for _ in range(100000):
counter += 1
threads = []
for _ in range(5):
thread = threading.Thread(target=increment)
threads.append(thread)
thread.start()
for thread in threads:
thread.join()
print(counter) # Output may be less than 500000
In this example, the final value of counter may be less than expected due to race conditions. For thread-safe operations, consider using the threading.Lock class or the multiprocessing module.
+= in Functional Programming
While += is convenient, it’s worth noting that it’s a stateful operation. In functional programming paradigms, where immutability is preferred, you might want to avoid += in favor of creating new objects:
def add_to_list(lst, item):
return lst + [item]
my_list = [1, 2, 3]
my_list = add_to_list(my_list, 4)
print(my_list) # Output: [1, 2, 3, 4]
This approach creates a new list instead of modifying the existing one, which can be beneficial in certain contexts.
+= with Custom Objects
You can define how += behaves with your custom objects by implementing the __iadd__ method. Here’s a more complex example:
class Vector:
def __init__(self, x, y):
self.x = x
self.y = y
def __iadd__(self, other):
if isinstance(other, Vector):
self.x += other.x
self.y += other.y
elif isinstance(other, (int, float)):
self.x += other
self.y += other
else:
raise TypeError("Unsupported operand type")
return self
def __str__(self):
return f"Vector({self.x}, {self.y})"
v1 = Vector(1, 2)
v2 = Vector(3, 4)
v1 += v2
print(v1) # Output: Vector(4, 6)
v1 += 5
print(v1) # Output: Vector(9, 11)
This Vector class supports += with both other Vector objects and scalar values.
+= in Python 2 vs Python 3
The behavior of += is generally consistent between Python 2 and Python 3, but there are some differences to be aware of, particularly with division:
# Python 2
x = 5
x += 2.0 # x becomes 7.0 (float)
# Python 3
x = 5
x += 2.0 # x becomes 7.0 (float)
# The difference is more apparent with division
# Python 2
x = 5
x /= 2 # x becomes 2 (integer division)
# Python 3
x = 5
x /= 2 # x becomes 2.5 (float division)
In Python 3, division always returns a float, while in Python 2, it depends on the operands.
Conclusion
The += operator in Python is a powerful and versatile tool that can make your code more concise and readable. It works across various data types, from simple numbers to complex custom objects. Understanding its behavior with different types, its implementation through the __iadd__ method, and its performance characteristics can help you use it more effectively in your Python programs.
While += is generally straightforward, it’s important to be aware of potential pitfalls, especially when working with immutable objects or in multithreaded environments. By mastering the nuances of +=, you can write more efficient and expressive Python code.
Remember, like many features in Python, += is designed to make the common case easy while still allowing for more complex use cases. Whether you’re a beginner or an experienced Python developer, understanding += thoroughly will undoubtedly enhance your Python programming skills.