Understanding the Importance of Algorithms in Different Domains
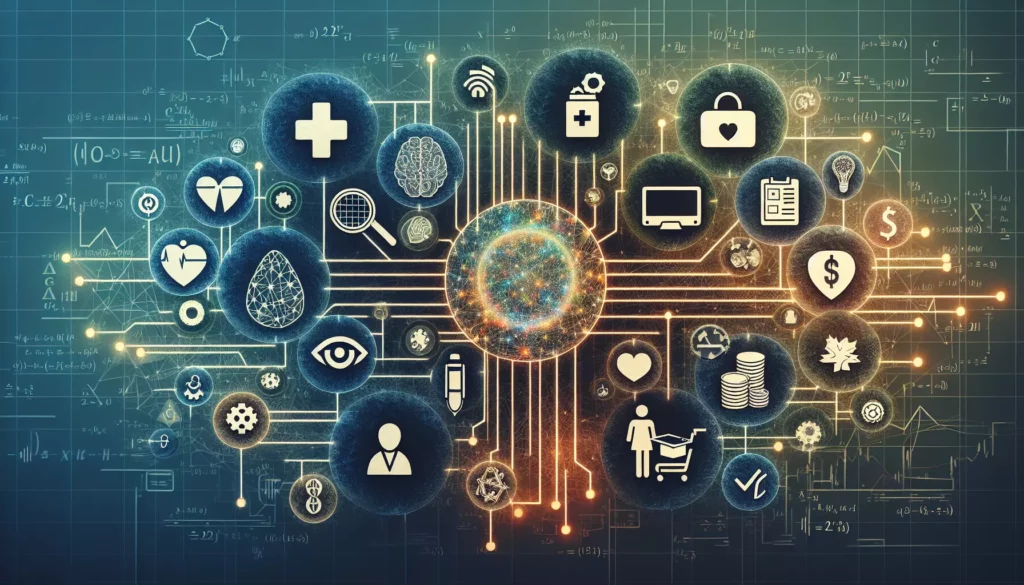
In today’s digital age, algorithms have become an integral part of our lives, influencing everything from the content we see on social media to the way we navigate through traffic. As the backbone of modern technology, algorithms play a crucial role in various domains, shaping the way we interact with the world around us. In this comprehensive guide, we’ll explore the significance of algorithms across different fields and why understanding them is essential for anyone looking to thrive in the tech-driven landscape.
What Are Algorithms?
Before diving into their importance, let’s first define what algorithms are. At its core, an algorithm is a step-by-step procedure or formula for solving a problem or accomplishing a task. In computer science, algorithms are the foundation of all software and are used to process data, make decisions, and automate complex processes.
Algorithms can be simple, like a recipe for baking a cake, or incredibly complex, like the ones used in artificial intelligence and machine learning. Regardless of their complexity, all algorithms share a common purpose: to efficiently solve problems and process information.
The Ubiquity of Algorithms in Modern Life
Algorithms are everywhere, often working behind the scenes to power the technologies we use daily. Here are just a few examples of how algorithms impact our lives:
- Search engines use algorithms to rank and display relevant results
- Social media platforms employ algorithms to curate personalized content feeds
- E-commerce websites utilize recommendation algorithms to suggest products
- GPS navigation systems use routing algorithms to find the fastest paths
- Streaming services rely on algorithms to recommend movies and TV shows
- Financial institutions use algorithms for fraud detection and risk assessment
As we can see, algorithms have permeated nearly every aspect of our digital lives, making them an essential subject of study for anyone interested in technology or looking to enter the tech industry.
The Importance of Algorithms in Different Domains
Now that we understand the prevalence of algorithms, let’s explore their importance across various domains:
1. Computer Science and Software Development
In the field of computer science and software development, algorithms are the foundation of everything we do. They are essential for:
- Efficient problem-solving: Algorithms help developers create efficient solutions to complex problems
- Optimizing performance: Well-designed algorithms can significantly improve the speed and efficiency of software applications
- Data structures: Algorithms work hand-in-hand with data structures to organize and manipulate data effectively
- Software architecture: Understanding algorithms is crucial for designing scalable and maintainable software systems
For aspiring software developers, a strong grasp of algorithms is essential for success in technical interviews and day-to-day work. Platforms like AlgoCademy provide invaluable resources for learning and practicing algorithmic problem-solving, helping developers prepare for interviews at top tech companies.
2. Artificial Intelligence and Machine Learning
The fields of artificial intelligence (AI) and machine learning (ML) are built on the foundation of advanced algorithms. In these domains, algorithms are used to:
- Train models on large datasets
- Recognize patterns and make predictions
- Optimize decision-making processes
- Enable natural language processing and computer vision
As AI and ML continue to evolve and impact various industries, understanding the underlying algorithms becomes increasingly important for professionals in these fields.
3. Data Science and Analytics
Data scientists and analysts rely heavily on algorithms to extract insights from large datasets. Algorithms are crucial for:
- Data cleaning and preprocessing
- Statistical analysis and hypothesis testing
- Predictive modeling and forecasting
- Data visualization and pattern recognition
By leveraging algorithms, data professionals can uncover valuable insights that drive business decisions and strategies.
4. Finance and Trading
The financial sector has been revolutionized by algorithmic trading and automated decision-making systems. In this domain, algorithms are used for:
- High-frequency trading
- Risk assessment and management
- Portfolio optimization
- Fraud detection and prevention
Understanding algorithms is crucial for financial professionals looking to stay competitive in an increasingly automated industry.
5. Healthcare and Bioinformatics
In healthcare and bioinformatics, algorithms play a vital role in:
- Analyzing medical images for diagnosis
- Predicting disease outbreaks and patient outcomes
- Drug discovery and development
- Genomic sequencing and analysis
As healthcare becomes more data-driven, the importance of algorithms in this field continues to grow.
6. Robotics and Automation
Algorithms are the brains behind robots and automated systems, enabling them to:
- Navigate and interact with their environment
- Perform complex tasks and make decisions
- Learn and adapt to new situations
- Collaborate with humans in various settings
As automation becomes more prevalent across industries, understanding the algorithms that power these systems is increasingly valuable.
The Role of Algorithmic Thinking in Problem-Solving
Beyond specific domains, the ability to think algorithmically is a valuable skill in itself. Algorithmic thinking involves:
- Breaking down complex problems into smaller, manageable steps
- Identifying patterns and creating generalizable solutions
- Optimizing processes for efficiency and scalability
- Considering edge cases and potential pitfalls
This approach to problem-solving is beneficial not only in technical fields but also in everyday life and business contexts. By developing algorithmic thinking skills, individuals can become more effective problem-solvers and decision-makers.
Learning Algorithms: Where to Start
For those interested in learning more about algorithms, there are numerous resources available:
- Online platforms: Websites like AlgoCademy offer interactive tutorials and coding challenges to help learners develop their algorithmic skills
- Books: Classic texts like “Introduction to Algorithms” by Cormen, Leiserson, Rivest, and Stein provide comprehensive coverage of algorithmic concepts
- MOOCs: Many universities offer free online courses on algorithms through platforms like Coursera and edX
- Coding bootcamps: Intensive programs that often include algorithm study as part of their curriculum
- Practice websites: Platforms like LeetCode and HackerRank offer coding challenges to help you apply algorithmic concepts
When learning algorithms, it’s essential to combine theoretical understanding with practical implementation. Platforms like AlgoCademy offer a balanced approach, providing both conceptual explanations and hands-on coding exercises.
Common Algorithms Every Programmer Should Know
While the field of algorithms is vast, there are some fundamental algorithms that every programmer should be familiar with:
1. Sorting Algorithms
Sorting algorithms are used to arrange data in a specific order. Some common sorting algorithms include:
- Bubble Sort
- Insertion Sort
- Merge Sort
- Quick Sort
- Heap Sort
Here’s a simple implementation of the Bubble Sort algorithm in Python:
def bubble_sort(arr):
n = len(arr)
for i in range(n):
for j in range(0, n - i - 1):
if arr[j] > arr[j + 1]:
arr[j], arr[j + 1] = arr[j + 1], arr[j]
return arr
# Example usage
unsorted_list = [64, 34, 25, 12, 22, 11, 90]
sorted_list = bubble_sort(unsorted_list)
print(sorted_list)
2. Searching Algorithms
Searching algorithms are used to find specific items within a dataset. Common searching algorithms include:
- Linear Search
- Binary Search
- Depth-First Search (DFS)
- Breadth-First Search (BFS)
Here’s an implementation of the Binary Search algorithm in Java:
public class BinarySearch {
public static int binarySearch(int[] arr, int target) {
int left = 0;
int right = arr.length - 1;
while (left <= right) {
int mid = left + (right - left) / 2;
if (arr[mid] == target) {
return mid;
} else if (arr[mid] < target) {
left = mid + 1;
} else {
right = mid - 1;
}
}
return -1; // Target not found
}
public static void main(String[] args) {
int[] sortedArray = {1, 3, 5, 7, 9, 11, 13, 15};
int target = 7;
int result = binarySearch(sortedArray, target);
if (result != -1) {
System.out.println("Element found at index: " + result);
} else {
System.out.println("Element not found in the array");
}
}
}
3. Graph Algorithms
Graph algorithms are used to solve problems related to networks and relationships. Some important graph algorithms include:
- Dijkstra’s Algorithm (shortest path)
- Kruskal’s Algorithm (minimum spanning tree)
- Floyd-Warshall Algorithm (all-pairs shortest path)
- Topological Sort
4. Dynamic Programming
Dynamic programming is a technique used to solve complex problems by breaking them down into simpler subproblems. It’s often used in optimization problems and can significantly improve the efficiency of algorithms.
5. Greedy Algorithms
Greedy algorithms make locally optimal choices at each step with the hope of finding a global optimum. They are often used in optimization problems where finding an exact solution is impractical.
The Future of Algorithms
As technology continues to advance, the importance of algorithms is only expected to grow. Some emerging trends in the field of algorithms include:
- Quantum algorithms: With the development of quantum computers, new algorithms are being designed to take advantage of quantum properties
- Explainable AI: As AI systems become more complex, there’s a growing need for algorithms that can explain their decision-making processes
- Edge computing algorithms: With the rise of IoT devices, algorithms that can run efficiently on low-power devices are becoming increasingly important
- Federated learning: Algorithms that can train machine learning models across decentralized devices while maintaining privacy
Staying up-to-date with these developments will be crucial for professionals in tech-related fields.
Conclusion
Understanding algorithms is no longer just a requirement for computer scientists and software developers. As algorithms continue to shape our digital world, having a solid grasp of algorithmic concepts is becoming increasingly valuable across various domains. Whether you’re a student looking to enter the tech industry, a professional seeking to enhance your problem-solving skills, or simply someone interested in understanding the technology that surrounds us, investing time in learning about algorithms can pay significant dividends.
Platforms like AlgoCademy offer an excellent starting point for those looking to develop their algorithmic thinking and coding skills. By providing interactive tutorials, coding challenges, and AI-powered assistance, these resources make it easier than ever to embark on the journey of mastering algorithms.
Remember, the goal isn’t just to memorize specific algorithms but to develop a problem-solving mindset that can be applied to a wide range of challenges. As you continue to learn and practice, you’ll find that algorithmic thinking becomes a powerful tool in your personal and professional life, opening up new opportunities and enabling you to tackle complex problems with confidence.
So, whether you’re preparing for a technical interview at a top tech company or simply looking to enhance your analytical skills, don’t underestimate the importance of algorithms. Embrace the challenge, start learning, and unlock the potential that comes with mastering this fundamental aspect of modern technology.