Understanding the Fundamentals: Why Basics Matter in Advanced Coding
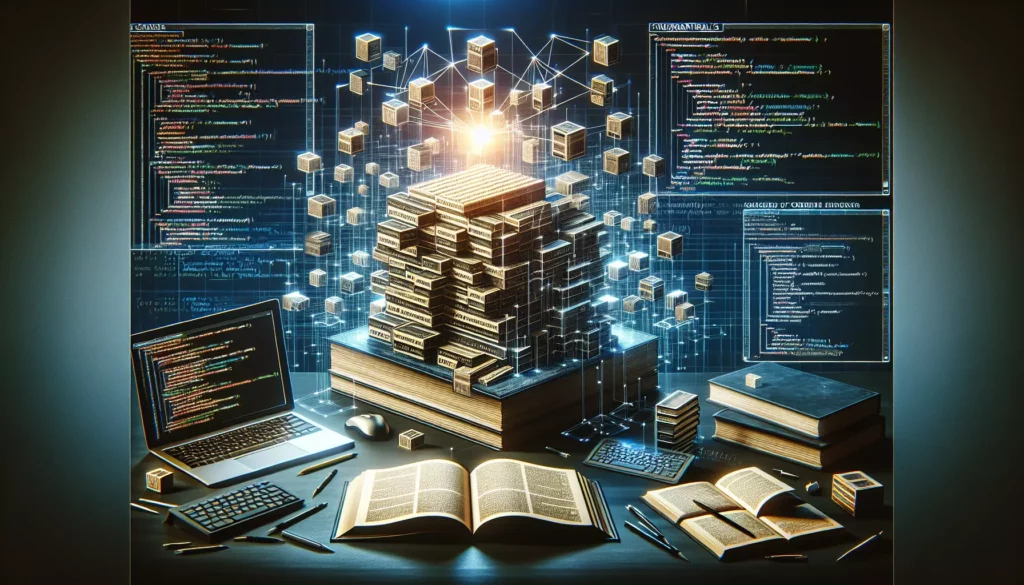
In the fast-paced world of technology, where new programming languages and frameworks seem to emerge overnight, it’s easy to get caught up in the excitement of learning the latest and greatest tools. However, amidst this constant evolution, one truth remains steadfast: a strong foundation in the basics is crucial for success in advanced coding. This article will explore why mastering the fundamentals is essential, even for those aiming to tackle complex algorithms or prepare for technical interviews at top tech companies.
The Building Blocks of Programming
Just as a skyscraper needs a solid foundation to reach great heights, a programmer’s skill set must be built upon a robust understanding of core concepts. These fundamental building blocks include:
- Variables and data types
- Control structures (if statements, loops)
- Functions and methods
- Basic data structures (arrays, lists, dictionaries)
- Object-oriented programming principles
- Basic algorithms and problem-solving techniques
These concepts form the bedrock upon which all advanced programming skills are built. Without a firm grasp of these basics, attempting to learn more complex topics can be like trying to run before learning to walk.
The Role of Fundamentals in Problem-Solving
One of the primary skills that separates great programmers from good ones is the ability to solve complex problems efficiently. This skill is not about knowing the most obscure language features or memorizing advanced algorithms. Instead, it’s about having a deep understanding of the basics and knowing how to apply them creatively.
Consider a common coding interview question: reversing a string. While there might be built-in functions to accomplish this in many languages, understanding how to do it manually reveals much more about a programmer’s capabilities:
def reverse_string(s):
reversed = ''
for char in s:
reversed = char + reversed
return reversed
print(reverse_string("Hello, World!")) # Output: !dlroW ,olleH
This simple function demonstrates an understanding of string manipulation, loops, and the concept of building a new string character by character. These are fundamental concepts that, when mastered, can be applied to solve much more complex problems.
Debugging and Troubleshooting
Another critical area where strong fundamentals shine is in debugging and troubleshooting. When faced with a bug in complex code, the ability to break down the problem into smaller, manageable parts is invaluable. This skill comes from a deep understanding of how basic programming constructs work.
For example, consider a scenario where a function is not producing the expected output. A programmer with solid fundamentals might approach the problem like this:
- Check the input parameters to ensure they are correct
- Use print statements or a debugger to trace the flow of the function
- Examine each step of the algorithm to identify where the logic might be flawed
- Test edge cases to ensure the function handles all scenarios correctly
This methodical approach, rooted in a strong grasp of basics, often leads to quicker resolution of issues compared to haphazard guessing or relying solely on trial and error.
Adaptability in a Changing Landscape
The tech industry is known for its rapid pace of change. New languages, frameworks, and tools are constantly emerging. In this environment, adaptability is a crucial skill for any programmer. Here’s where strong fundamentals become a superpower.
When you understand the core concepts of programming, learning a new language or framework becomes much easier. The syntax may change, but the underlying principles remain the same. For instance, if you understand how loops work in one language, you can quickly grasp how they function in another:
// JavaScript
for (let i = 0; i < 5; i++) {
console.log(i);
}
# Python
for i in range(5):
print(i)
// Java
for (int i = 0; i < 5; i++) {
System.out.println(i);
}
The syntax differs, but the fundamental concept of iteration remains constant across these languages. A programmer with strong basics can quickly adapt to new languages by mapping familiar concepts to new syntax.
Performance Optimization
As programs grow in complexity, performance becomes increasingly important. Optimizing code for speed and efficiency often requires a deep understanding of how computers process instructions at a fundamental level.
For example, consider the difference between two ways of summing numbers in a list:
def sum_list_1(numbers):
total = 0
for num in numbers:
total += num
return total
def sum_list_2(numbers):
return sum(numbers)
# Both functions will produce the same result, but sum_list_2 is generally faster
While both functions achieve the same result, sum_list_2
is typically faster because it uses a built-in function optimized for this specific operation. Understanding why this is the case requires knowledge of how loops work, how memory is accessed, and the benefits of using optimized built-in functions.
Code Readability and Maintenance
In professional settings, code is often read and modified by multiple developers over time. Writing clean, readable code is a skill that stems from a strong grasp of fundamentals. When you understand the basics well, you can express complex ideas in simple, clear code.
Consider these two functions that check if a number is prime:
def is_prime_1(n):
if n < 2:
return False
for i in range(2, int(n**0.5) + 1):
if n % i == 0:
return False
return True
def is_prime_2(n):
return n > 1 and all(n % i for i in range(2, int(n**0.5) + 1))
Both functions are correct, but is_prime_1
is more readable and easier to understand at a glance. It clearly shows the logic step by step, making it easier for other developers (or yourself in the future) to read and maintain the code.
Preparing for Technical Interviews
For those aiming to land jobs at top tech companies, often referred to as FAANG (Facebook, Amazon, Apple, Netflix, Google), a solid foundation in programming basics is absolutely crucial. These companies are known for their rigorous technical interviews, which often focus on fundamental computer science concepts and problem-solving skills.
Interviewers at these companies are less interested in your knowledge of specific frameworks or libraries. Instead, they want to see how you think, how you approach problems, and how well you understand core programming concepts. This is where a strong foundation in the basics truly shines.
Common topics in these interviews include:
- Data structures (arrays, linked lists, trees, graphs)
- Algorithms (sorting, searching, traversal)
- Time and space complexity analysis
- Object-oriented design
- System design (for more senior positions)
All of these topics build upon fundamental programming concepts. For instance, understanding how to implement and use a binary search tree requires a solid grasp of recursion, pointers (or references), and basic tree structures:
class Node:
def __init__(self, value):
self.value = value
self.left = None
self.right = None
class BinarySearchTree:
def __init__(self):
self.root = None
def insert(self, value):
if not self.root:
self.root = Node(value)
else:
self._insert_recursive(self.root, value)
def _insert_recursive(self, node, value):
if value < node.value:
if node.left is None:
node.left = Node(value)
else:
self._insert_recursive(node.left, value)
else:
if node.right is None:
node.right = Node(value)
else:
self._insert_recursive(node.right, value)
This implementation demonstrates an understanding of classes, recursion, and the binary search tree property. These are exactly the kind of fundamental skills that interviewers at top tech companies are looking for.
Continuous Learning and Growth
The journey of a programmer is one of continuous learning. As you progress in your career, you’ll encounter increasingly complex problems and systems. Having a strong foundation makes this ongoing learning process much smoother.
When you understand the basics deeply, you can more easily:
- Grasp new programming paradigms (e.g., functional programming)
- Understand and implement design patterns
- Learn about system architecture and scalability
- Explore advanced topics like machine learning and artificial intelligence
Each of these advanced areas builds upon fundamental programming concepts. For instance, understanding functional programming is much easier if you have a solid grasp of functions as first-class citizens in a language:
// JavaScript example of functions as first-class citizens
const numbers = [1, 2, 3, 4, 5];
// Using a function as an argument
const doubled = numbers.map(function(num) {
return num * 2;
});
// Using an arrow function
const squared = numbers.map(num => num ** 2);
console.log(doubled); // [2, 4, 6, 8, 10]
console.log(squared); // [1, 4, 9, 16, 25]
This example demonstrates how a strong understanding of functions and their properties in JavaScript can serve as a stepping stone to grasping functional programming concepts.
The Role of Practice and Application
While understanding the theory behind programming fundamentals is crucial, true mastery comes from consistent practice and application. This is where platforms like AlgoCademy play a vital role in a programmer’s journey.
AlgoCademy provides a structured environment for learners to:
- Practice coding problems that reinforce fundamental concepts
- Receive immediate feedback on their solutions
- Learn step-by-step problem-solving techniques
- Prepare for technical interviews with real-world coding challenges
By offering a combination of theoretical knowledge and practical application, such platforms help bridge the gap between understanding basics and applying them to solve complex problems.
Conclusion: Building a Strong Foundation for Long-Term Success
In the ever-evolving world of technology, the importance of strong programming fundamentals cannot be overstated. Whether you’re just starting your coding journey or preparing for interviews at top tech companies, a solid grasp of the basics will serve you well.
Remember:
- Fundamentals are the building blocks for all advanced concepts
- Strong basics enhance problem-solving and debugging skills
- A deep understanding of core concepts aids in adapting to new technologies
- Mastery of fundamentals is crucial for optimizing performance and writing maintainable code
- Technical interviews, especially at top companies, focus heavily on fundamental skills
- A strong foundation facilitates continuous learning and growth in your programming career
As you continue your journey in programming, whether through self-study, formal education, or platforms like AlgoCademy, always remember to reinforce and build upon your understanding of the basics. It’s these fundamental skills that will ultimately set you apart as a programmer and pave the way for long-term success in the field.
In the words of Bruce Lee, “I fear not the man who has practiced 10,000 kicks once, but I fear the man who has practiced one kick 10,000 times.” In programming, mastering the fundamentals is that one kick. Practice it, perfect it, and watch as it opens doors to endless possibilities in your coding journey.