Understanding the Event Loop in JavaScript: A Comprehensive Guide
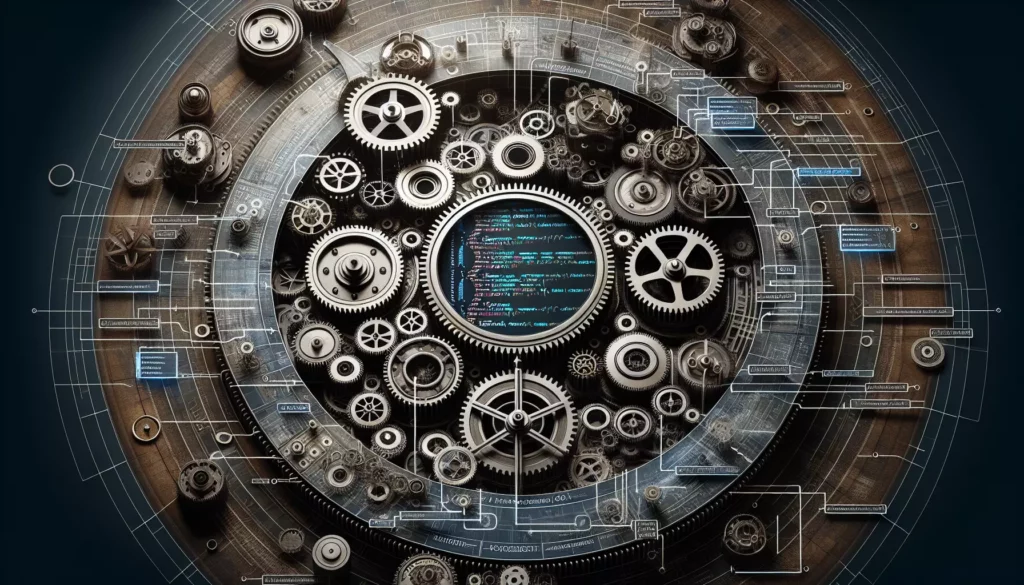
JavaScript is known for its asynchronous nature, which allows it to handle multiple operations simultaneously without blocking the execution of code. At the heart of this asynchronous behavior lies the Event Loop, a crucial mechanism that enables JavaScript to manage concurrency and maintain responsiveness in applications. In this comprehensive guide, we’ll dive deep into the Event Loop, exploring its inner workings, components, and how it impacts JavaScript’s execution model.
Table of Contents
- What is the Event Loop?
- The JavaScript Runtime Environment
- The Call Stack
- Web APIs and the Callback Queue
- The Event Loop in Action
- Microtasks vs. Macrotasks
- Optimizing for the Event Loop
- Common Pitfalls and Best Practices
- The Event Loop in Node.js
- Conclusion
1. What is the Event Loop?
The Event Loop is a fundamental concept in JavaScript that allows the language to perform non-blocking operations despite being single-threaded. It’s the mechanism that enables JavaScript to handle asynchronous callbacks, promises, and other deferred actions efficiently.
At its core, the Event Loop continuously checks if there are any tasks in the queue that need to be executed. It operates on a simple principle: it takes the first task from the queue, executes it, and then repeats the process with the next task. This loop continues as long as there are tasks to process.
2. The JavaScript Runtime Environment
To understand the Event Loop fully, we need to first grasp the components of the JavaScript runtime environment:
- Heap: This is where memory allocation happens for variables and objects.
- Call Stack: This is where function calls are stacked and executed.
- Web APIs: These are provided by the browser and include functionality like DOM manipulation, AJAX requests, and timers.
- Callback Queue: This is where callback functions wait to be executed.
- Event Loop: This constantly checks if the Call Stack is empty and moves callbacks from the Queue to the Stack when it is.
3. The Call Stack
The Call Stack is a data structure that records where in the program we are. When we call a function, it’s pushed onto the stack. When we return from a function, it’s popped off the stack.
Let’s look at a simple example:
function multiply(a, b) {
return a * b;
}
function square(n) {
return multiply(n, n);
}
function printSquare(n) {
var squared = square(n);
console.log(squared);
}
printSquare(4);
Here’s how the Call Stack would look during execution:
- Push
printSquare(4)
onto the stack - Push
square(4)
onto the stack - Push
multiply(4, 4)
onto the stack - Execute
multiply(4, 4)
, pop it off the stack - Return to
square(4)
, pop it off the stack - Execute
console.log(squared)
inprintSquare
- Pop
printSquare(4)
off the stack
4. Web APIs and the Callback Queue
JavaScript in the browser has access to Web APIs provided by the browser environment. These APIs include functions for making HTTP requests (XMLHttpRequest or fetch), manipulating the DOM, and setting timers (setTimeout or setInterval).
When you use these APIs, the browser takes care of the heavy lifting, and once it’s done, it pushes any callback function you’ve provided onto the Callback Queue.
Here’s an example using setTimeout
:
console.log('Start');
setTimeout(function() {
console.log('Timeout callback');
}, 0);
console.log('End');
The output will be:
Start
End
Timeout callback
Even though we set the timeout to 0 milliseconds, the callback function is still pushed to the Callback Queue and has to wait for the Call Stack to be empty before it can be executed.
5. The Event Loop in Action
Now that we understand the components, let’s see how the Event Loop ties everything together:
- The Event Loop constantly checks if the Call Stack is empty.
- If the Call Stack is empty, it looks at the Callback Queue.
- If there’s a callback in the Queue, it pushes it onto the Call Stack for execution.
- This process repeats, creating a loop.
Here’s a more complex example to illustrate this:
console.log('Script start');
setTimeout(function() {
console.log('setTimeout');
}, 0);
Promise.resolve().then(function() {
console.log('Promise 1');
}).then(function() {
console.log('Promise 2');
});
console.log('Script end');
The output will be:
Script start
Script end
Promise 1
Promise 2
setTimeout
This output order demonstrates how the Event Loop prioritizes tasks and manages the execution flow.
6. Microtasks vs. Macrotasks
The Event Loop actually manages two types of tasks: microtasks and macrotasks.
- Macrotasks: Include setTimeout, setInterval, setImmediate, I/O operations, UI rendering
- Microtasks: Include Promise callbacks, process.nextTick (in Node.js), MutationObserver
The Event Loop prioritizes microtasks over macrotasks. After each macrotask, the Event Loop will empty the microtask queue before moving on to the next macrotask.
Here’s an example to illustrate this:
console.log('Script start');
setTimeout(function() {
console.log('setTimeout 1');
}, 0);
Promise.resolve().then(function() {
console.log('Promise 1');
}).then(function() {
console.log('Promise 2');
});
setTimeout(function() {
console.log('setTimeout 2');
}, 0);
console.log('Script end');
The output will be:
Script start
Script end
Promise 1
Promise 2
setTimeout 1
setTimeout 2
Notice how both Promises (microtasks) are executed before the setTimeout callbacks (macrotasks).
7. Optimizing for the Event Loop
Understanding the Event Loop can help you write more efficient JavaScript code. Here are some optimization tips:
- Avoid long-running tasks: Long-running synchronous tasks can block the Event Loop, making your application unresponsive. Break these tasks into smaller chunks or use Web Workers for heavy computations.
- Use asynchronous operations: Whenever possible, use asynchronous versions of functions to avoid blocking the main thread.
- Be mindful of microtasks: While microtasks are prioritized, an excessive number of microtasks can delay macrotasks indefinitely. This is known as “microtask starvation.”
- Optimize DOM manipulation: Batch DOM updates to reduce the number of repaints and reflows, which can be expensive operations.
8. Common Pitfalls and Best Practices
When working with the Event Loop, there are several common pitfalls to avoid:
Callback Hell
Nesting multiple callbacks can lead to code that’s hard to read and maintain, often referred to as “callback hell.” Consider this example:
getData(function(a) {
getMoreData(a, function(b) {
getEvenMoreData(b, function(c) {
getFinalData(c, function(d) {
console.log(d);
});
});
});
});
To avoid this, you can use Promises or async/await syntax:
async function getAllData() {
const a = await getData();
const b = await getMoreData(a);
const c = await getEvenMoreData(b);
const d = await getFinalData(c);
console.log(d);
}
getAllData();
Blocking the Event Loop
Long-running synchronous operations can block the Event Loop, making your application unresponsive. For example:
function longRunningOperation() {
for (let i = 0; i < 1000000000; i++) {
// Do something computationally expensive
}
}
longRunningOperation();
console.log('This will be delayed');
Instead, consider breaking the operation into smaller chunks or using Web Workers for heavy computations:
function longRunningOperation(start, end) {
if (start >= end) return;
// Do a chunk of work
for (let i = start; i < Math.min(start + 1000000, end); i++) {
// Do something computationally expensive
}
setTimeout(() => longRunningOperation(start + 1000000, end), 0);
}
longRunningOperation(0, 1000000000);
console.log('This will not be delayed');
9. The Event Loop in Node.js
While the core concept of the Event Loop is the same in Node.js as it is in browsers, there are some differences in implementation and additional phases in Node.js’s Event Loop:
- Timers: Executes callbacks scheduled by setTimeout() and setInterval()
- Pending callbacks: Executes I/O callbacks deferred to the next loop iteration
- Idle, prepare: Used internally
- Poll: Retrieves new I/O events; executes I/O related callbacks
- Check: Executes setImmediate() callbacks
- Close callbacks: Executes close callbacks, e.g., socket.on(‘close’, …)
Node.js also introduces process.nextTick(), which adds callbacks to the microtask queue, similar to Promises.
console.log('Start');
setTimeout(() => console.log('Timeout 1'), 0);
setImmediate(() => console.log('Immediate 1'));
process.nextTick(() => console.log('NextTick 1'));
Promise.resolve().then(() => console.log('Promise 1'));
console.log('End');
The output will typically be:
Start
End
NextTick 1
Promise 1
Timeout 1
Immediate 1
Understanding these phases can help you optimize Node.js applications and avoid potential issues with the Event Loop.
10. Conclusion
The Event Loop is a fundamental concept in JavaScript that enables its asynchronous, non-blocking behavior. By understanding how the Event Loop works, you can write more efficient and responsive JavaScript code, both in the browser and in Node.js environments.
Key takeaways include:
- The Event Loop continuously checks the Call Stack and Callback Queue, moving callbacks to the Stack when it’s empty.
- Web APIs handle time-consuming operations, freeing up the main thread.
- Microtasks (like Promise callbacks) are prioritized over macrotasks (like setTimeout callbacks).
- Long-running synchronous operations can block the Event Loop and should be avoided.
- Asynchronous programming patterns like Promises and async/await can help manage complex asynchronous operations.
As you continue to develop your JavaScript skills, keep the Event Loop in mind. It will help you understand why your code behaves the way it does and how to optimize it for better performance. Whether you’re building interactive web applications or scalable backend services with Node.js, a solid grasp of the Event Loop is essential for any serious JavaScript developer.