Understanding the Call Stack and Stack Trace: Essential Tools for Debugging and Code Execution
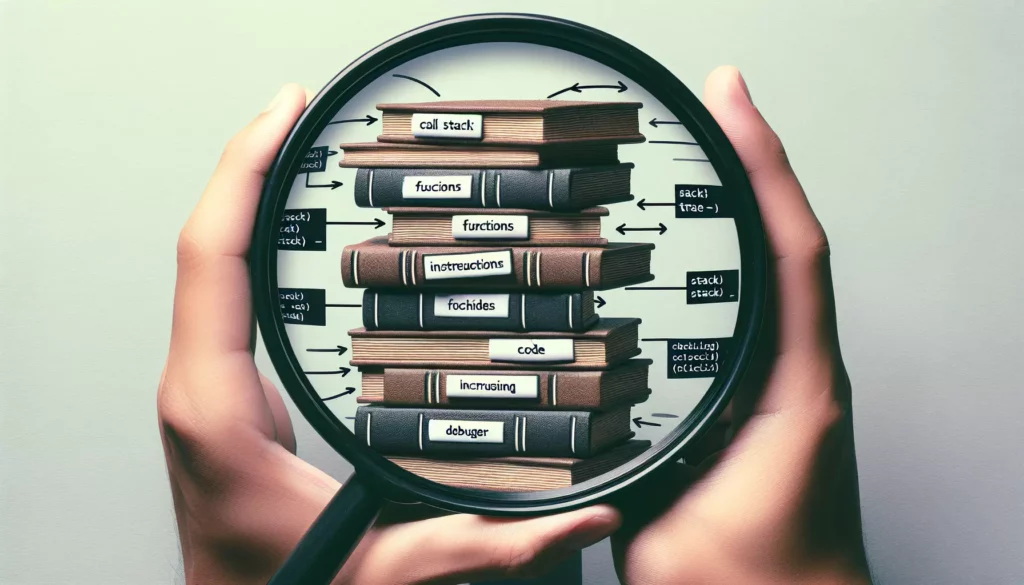
As you progress in your coding journey, you’ll encounter situations where understanding how your program executes becomes crucial. Two fundamental concepts that play a vital role in this understanding are the call stack and stack trace. These concepts are not only essential for debugging but also for grasping how your code behaves during runtime. In this comprehensive guide, we’ll dive deep into the call stack and stack trace, exploring their significance, functionality, and how they can be leveraged to enhance your programming skills.
What is the Call Stack?
The call stack, also known as the program stack or execution stack, is a critical data structure used by programming languages to manage the execution of function calls in a program. It keeps track of active subroutines (functions or methods) and handles the flow of execution as functions are called and return.
To understand the call stack better, let’s break down its key characteristics:
- Last-In-First-Out (LIFO) Structure: The call stack operates on a LIFO principle, meaning the most recently added item is the first one to be removed.
- Function Call Management: When a function is called, a new frame is pushed onto the stack, containing information about the function’s local variables, parameters, and return address.
- Memory Allocation: Each function call allocates memory on the stack for its local variables and parameters.
- Execution Context: The call stack maintains the current execution context of the program, allowing it to resume execution at the correct point after a function returns.
How the Call Stack Works
To illustrate how the call stack works, let’s consider a simple example in Python:
def function_a():
print("Executing function A")
function_b()
print("Back in function A")
def function_b():
print("Executing function B")
function_c()
print("Back in function B")
def function_c():
print("Executing function C")
function_a()
When this program runs, the call stack evolves as follows:
- Initially, the stack is empty.
function_a()
is called, and a frame for it is pushed onto the stack.- Inside
function_a()
,function_b()
is called, and a frame for it is pushed onto the stack. - Inside
function_b()
,function_c()
is called, and a frame for it is pushed onto the stack. function_c()
executes and completes, so its frame is popped off the stack.- Execution returns to
function_b()
, which completes, and its frame is popped off the stack. - Finally, execution returns to
function_a()
, which completes, and its frame is popped off the stack. - The stack is now empty, and the program terminates.
Understanding Stack Traces
A stack trace, also known as a stack backtrace or call stack trace, is a report of the active stack frames at a certain point in time during the execution of a program. It’s essentially a snapshot of the call stack at a specific moment, usually when an exception or error occurs.
Stack traces are invaluable tools for debugging, as they provide crucial information about:
- The sequence of function calls that led to the current point of execution
- The line numbers in the source code where each function call occurred
- The values of local variables and function parameters at the time of the error
Anatomy of a Stack Trace
Let’s examine a typical stack trace in Python:
Traceback (most recent call last):
File "example.py", line 13, in <module>
function_a()
File "example.py", line 3, in function_a
function_b()
File "example.py", line 7, in function_b
function_c()
File "example.py", line 11, in function_c
raise Exception("An error occurred in function C")
Exception: An error occurred in function C
This stack trace provides the following information:
- The error originated in
function_c()
on line 11 of the file “example.py”. - The error was an Exception with the message “An error occurred in function C”.
- The sequence of function calls that led to the error:
function_a()
calledfunction_b()
, which calledfunction_c()
. - The line numbers where each function call occurred.
Importance of Call Stack and Stack Trace in Debugging
Understanding the call stack and being able to interpret stack traces are crucial skills for effective debugging. Here’s why:
1. Identifying the Source of Errors
Stack traces pinpoint the exact location where an error occurred, saving developers significant time in locating the problematic code. Instead of manually searching through the entire codebase, you can jump directly to the relevant line and function.
2. Understanding Program Flow
By examining the call stack, you can trace the sequence of function calls that led to a particular point in your program. This is especially useful when debugging complex applications with multiple layers of function calls.
3. Debugging Recursive Functions
For recursive functions, the call stack helps visualize the depth of recursion and identify potential issues like infinite recursion or excessive stack usage.
4. Memory Management Insights
Understanding the call stack provides insights into how memory is allocated and deallocated during program execution. This knowledge is crucial for optimizing memory usage and preventing memory-related errors.
5. Improving Code Quality
Analyzing stack traces can reveal patterns in your code that may need refactoring. For example, if you frequently see deep call stacks, it might indicate that your code could benefit from simplification or restructuring.
Advanced Concepts Related to Call Stack and Stack Trace
As you delve deeper into programming and software development, you’ll encounter more advanced concepts related to the call stack and stack traces. Let’s explore some of these:
1. Stack Overflow
A stack overflow occurs when the call stack exceeds its maximum size. This typically happens in scenarios involving deep recursion or when a large number of nested function calls occur. For example:
def recursive_function(n):
if n == 0:
return
recursive_function(n - 1)
recursive_function(1000000) # This will likely cause a stack overflow
Understanding stack overflows is crucial for writing efficient and stable code, especially when dealing with recursive algorithms or deep call hierarchies.
2. Tail Call Optimization
Tail call optimization is a technique used by some programming languages and compilers to optimize recursive functions. When a function call is the last operation in another function (known as a tail call), the compiler can optimize it to reuse the current stack frame instead of adding a new one. This can prevent stack overflow in certain recursive scenarios.
For example, consider this factorial function:
def factorial(n, acc=1):
if n == 0:
return acc
return factorial(n - 1, n * acc) # Tail call
In languages that support tail call optimization, this function could theoretically handle arbitrarily large inputs without causing a stack overflow.
3. Asynchronous Programming and the Call Stack
In asynchronous programming models, such as those used in JavaScript or Python’s asyncio, the relationship between the call stack and program execution becomes more complex. Asynchronous operations often involve callback functions or promises, which can lead to non-linear execution flows.
For instance, consider this JavaScript example:
function asyncOperation() {
console.log("Start of async operation");
setTimeout(() => {
console.log("Async operation complete");
}, 1000);
console.log("End of async operation function");
}
asyncOperation();
console.log("After calling asyncOperation");
The output of this code might be:
Start of async operation
End of async operation function
After calling asyncOperation
Async operation complete
Understanding how the call stack interacts with the event loop and task queue in asynchronous environments is crucial for debugging and optimizing asynchronous code.
4. Call Stack Sampling and Profiling
In performance optimization, call stack sampling is a technique used to identify performance bottlenecks in a program. By periodically sampling the call stack during program execution, developers can build a statistical profile of where the program spends most of its time.
Many profiling tools use call stack sampling to generate flame graphs, which provide a visual representation of the call stack over time, helping identify performance hotspots.
5. Stack Unwinding and Exception Handling
When an exception is thrown, the process of finding an appropriate exception handler involves “unwinding” the stack. The runtime system pops frames off the stack, looking for a catch block that can handle the exception. Understanding this process is crucial for implementing robust error handling in your programs.
For example, in C++:
void function_c() {
throw std::runtime_error("Error in function C");
}
void function_b() {
function_c();
}
void function_a() {
try {
function_b();
} catch (const std::exception& e) {
std::cout << "Caught exception: " << e.what() << std::endl;
}
}
int main() {
function_a();
return 0;
}
In this example, the exception thrown in function_c()
will unwind the stack through function_b()
until it’s caught in function_a()
.
Best Practices for Working with Call Stacks and Stack Traces
To effectively leverage your understanding of call stacks and stack traces, consider the following best practices:
1. Use Meaningful Function Names
Clear, descriptive function names make stack traces more readable and help quickly identify the purpose of each function in the call stack.
2. Implement Proper Error Handling
Use try-catch blocks (or equivalent constructs in your language) to handle exceptions at appropriate levels of your application. This helps create more informative stack traces and prevents unhandled exceptions from crashing your program.
3. Log Stack Traces
When catching exceptions, log the full stack trace along with any relevant context. This practice is invaluable for debugging production issues where you might not have direct access to the running application.
4. Use Debugging Tools
Familiarize yourself with debugging tools provided by your IDE or development environment. These tools often provide interactive ways to explore the call stack during program execution.
5. Monitor Stack Usage
In resource-constrained environments or when working with recursive algorithms, monitor stack usage to prevent stack overflow errors. Consider iterative solutions or tail-call optimization where appropriate.
6. Understand Language-Specific Behavior
Different programming languages handle call stacks and stack traces differently. Familiarize yourself with the specifics of your primary programming language(s) to interpret stack information accurately.
Conclusion
The call stack and stack trace are fundamental concepts in programming that play crucial roles in program execution and debugging. By understanding how the call stack manages function calls and how stack traces provide insights into program behavior, you can become a more effective developer and debugger.
As you continue to develop your programming skills, remember that mastering these concepts is an ongoing process. Practice interpreting stack traces, experiment with different debugging techniques, and always strive to write code that is not only functional but also easy to debug and maintain.
Whether you’re preparing for technical interviews at top tech companies or simply aiming to improve your coding skills, a solid grasp of call stacks and stack traces will serve you well throughout your programming career. Keep exploring, keep learning, and use these powerful tools to their full potential in your journey as a software developer.