Understanding the Basics of Discrete Mathematics
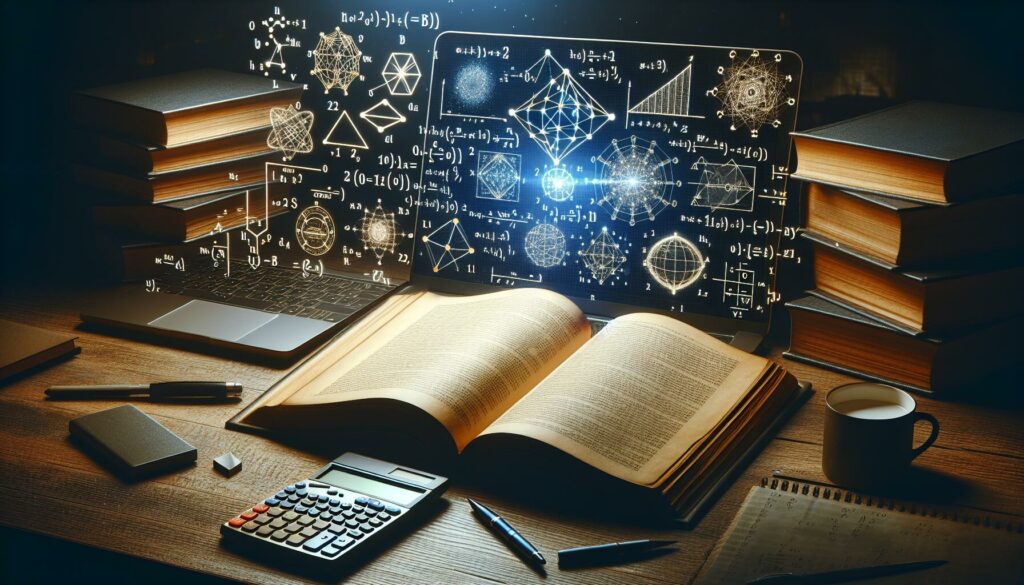
In the world of computer science and programming, discrete mathematics plays a crucial role. It forms the foundation for many concepts in algorithms, data structures, and computational theory. For aspiring programmers and those preparing for technical interviews at top tech companies like FAANG (Facebook, Amazon, Apple, Netflix, Google), a solid understanding of discrete mathematics is essential. In this comprehensive guide, we’ll explore the fundamental concepts of discrete mathematics and their applications in computer science.
What is Discrete Mathematics?
Discrete mathematics is a branch of mathematics that deals with objects that can assume only distinct, separate values. Unlike continuous mathematics, which deals with continuous, smooth functions, discrete mathematics focuses on structures that are countable or otherwise distinct and separable. This makes it particularly relevant to computer science, where we deal with discrete data and finite processes.
Some key areas of discrete mathematics include:
- Set Theory
- Logic
- Number Theory
- Combinatorics
- Graph Theory
- Probability
Let’s dive into each of these areas and explore their relevance to programming and algorithm design.
Set Theory
Set theory is the foundation of mathematics and is crucial in computer science. A set is a collection of distinct objects, called elements or members of the set. In programming, sets are often used to represent collections of data with no duplicates.
Key Concepts in Set Theory:
- Set operations: union, intersection, difference, complement
- Subsets and supersets
- Power sets
- Cartesian products
In Python, sets can be implemented using the built-in set
data type:
# Creating sets
A = {1, 2, 3, 4}
B = {3, 4, 5, 6}
# Set operations
union = A | B
intersection = A & B
difference = A - B
print(f"Union: {union}")
print(f"Intersection: {intersection}")
print(f"Difference: {difference}")
Understanding set theory is crucial for solving problems related to data organization, efficient searching, and eliminating duplicates in algorithms.
Logic
Logic is the study of reasoning and argumentation. In computer science, it forms the basis for boolean algebra, which is fundamental to digital circuit design and programming constructs like conditional statements and loops.
Key Concepts in Logic:
- Propositional logic
- Predicate logic
- Truth tables
- Logical connectives (AND, OR, NOT, XOR)
- Implication and equivalence
In programming, logical operations are used extensively in control flow statements:
def is_even_and_positive(num):
return num > 0 and num % 2 == 0
print(is_even_and_positive(4)) # True
print(is_even_and_positive(-2)) # False
Understanding logic helps in writing correct and efficient conditional statements, designing complex algorithms, and debugging code.
Number Theory
Number theory is the study of integers and their properties. It has numerous applications in computer science, especially in cryptography and algorithm design.
Key Concepts in Number Theory:
- Prime numbers and factorization
- Greatest Common Divisor (GCD) and Least Common Multiple (LCM)
- Modular arithmetic
- Congruences
- Euler’s totient function
Here’s an example of implementing the Euclidean algorithm to find the GCD of two numbers:
def gcd(a, b):
while b != 0:
a, b = b, a % b
return a
print(gcd(48, 18)) # Output: 6
Number theory is essential for developing efficient algorithms for tasks like primality testing, factorization, and in cryptographic applications.
Combinatorics
Combinatorics is the study of counting, arrangement, and combination of objects. It’s crucial for analyzing algorithm efficiency and solving problems related to probability and optimization.
Key Concepts in Combinatorics:
- Permutations and combinations
- Binomial coefficients
- Inclusion-exclusion principle
- Generating functions
- Recurrence relations
Here’s a Python function to calculate the number of combinations:
def combinations(n, r):
from math import factorial
return factorial(n) // (factorial(r) * factorial(n - r))
print(combinations(5, 2)) # Output: 10
Combinatorics is particularly useful in analyzing the time and space complexity of algorithms, especially those involving searching and sorting.
Graph Theory
Graph theory is the study of graphs, which are mathematical structures used to model pairwise relations between objects. It has numerous applications in computer science, including network analysis, pathfinding algorithms, and data organization.
Key Concepts in Graph Theory:
- Vertices and edges
- Directed and undirected graphs
- Graph traversal (DFS, BFS)
- Shortest path algorithms (Dijkstra’s, Bellman-Ford)
- Minimum spanning trees
- Graph coloring
Here’s a simple implementation of a graph and a depth-first search (DFS) traversal:
class Graph:
def __init__(self):
self.graph = {}
def add_edge(self, u, v):
if u not in self.graph:
self.graph[u] = []
self.graph[u].append(v)
def dfs(self, start, visited=None):
if visited is None:
visited = set()
visited.add(start)
print(start, end=' ')
for neighbor in self.graph.get(start, []):
if neighbor not in visited:
self.dfs(neighbor, visited)
# Example usage
g = Graph()
g.add_edge(0, 1)
g.add_edge(0, 2)
g.add_edge(1, 2)
g.add_edge(2, 0)
g.add_edge(2, 3)
g.add_edge(3, 3)
print("DFS starting from vertex 2:")
g.dfs(2)
Graph theory is essential for solving problems related to network flows, social network analysis, and optimization problems like the traveling salesman problem.
Probability
Probability theory is the study of random phenomena. In computer science, it’s crucial for analyzing algorithms, especially randomized algorithms, and in machine learning and artificial intelligence.
Key Concepts in Probability:
- Sample spaces and events
- Probability distributions
- Conditional probability
- Bayes’ theorem
- Random variables
- Expected value and variance
Here’s a simple example of calculating probability:
import random
def coin_flip_experiment(n):
heads = 0
for _ in range(n):
if random.choice(['H', 'T']) == 'H':
heads += 1
return heads / n
# Simulate 10000 coin flips
probability_of_heads = coin_flip_experiment(10000)
print(f"Probability of getting heads: {probability_of_heads:.4f}")
Probability is essential in algorithm analysis, especially for randomized algorithms, and in fields like machine learning and cryptography.
Applications in Computer Science and Programming
The concepts of discrete mathematics have numerous applications in computer science and programming. Here are some examples:
- Algorithm Analysis: Combinatorics and probability theory are used to analyze the time and space complexity of algorithms.
- Data Structures: Set theory and graph theory form the basis for many data structures like sets, trees, and graphs.
- Cryptography: Number theory is fundamental to many encryption algorithms.
- Database Design: Set theory is used in relational algebra, which forms the theoretical basis of relational databases.
- Artificial Intelligence: Logic and probability theory are crucial in AI and machine learning algorithms.
- Network Design: Graph theory is essential for designing and analyzing computer networks.
- Compiler Design: Automata theory, a branch of discrete mathematics, is used in lexical analysis and parsing.
Preparing for Technical Interviews
For those preparing for technical interviews at top tech companies, a strong foundation in discrete mathematics is invaluable. Here are some tips:
- Practice Problem Solving: Solve problems that involve discrete mathematics concepts. Platforms like LeetCode, HackerRank, and AlgoCademy offer many such problems.
- Understand the Underlying Theory: Don’t just memorize formulas. Understand the reasoning behind mathematical concepts and how they apply to computer science.
- Implement Algorithms: Implement common algorithms related to graph theory, number theory, and combinatorics. This will help you understand how these concepts are applied in practice.
- Analyze Time and Space Complexity: Practice analyzing the efficiency of your solutions using big O notation.
- Study Data Structures: Understand how discrete mathematics concepts are used in various data structures.
- Review Probability: Many interview questions involve probability and expected value calculations.
Conclusion
Discrete mathematics is a vast and fascinating field that forms the backbone of computer science. From set theory to graph theory, from logic to probability, these concepts are essential for anyone looking to excel in programming and algorithm design. By mastering these fundamentals, you’ll be better equipped to tackle complex problems, design efficient algorithms, and excel in technical interviews at top tech companies.
Remember, the key to mastering discrete mathematics is practice and application. As you learn these concepts, try to apply them to real-world programming problems. Use platforms like AlgoCademy to practice implementing algorithms and data structures based on these mathematical foundations. With time and practice, you’ll find that discrete mathematics becomes an invaluable tool in your programming toolkit, helping you to think more logically, solve problems more efficiently, and write better code.
As you continue your journey in computer science and programming, keep exploring the beautiful world of discrete mathematics. It’s not just about solving abstract problems; it’s about developing a way of thinking that will serve you well throughout your career in technology. Happy coding!