Understanding the Basics of Compiler and Interpreter Design
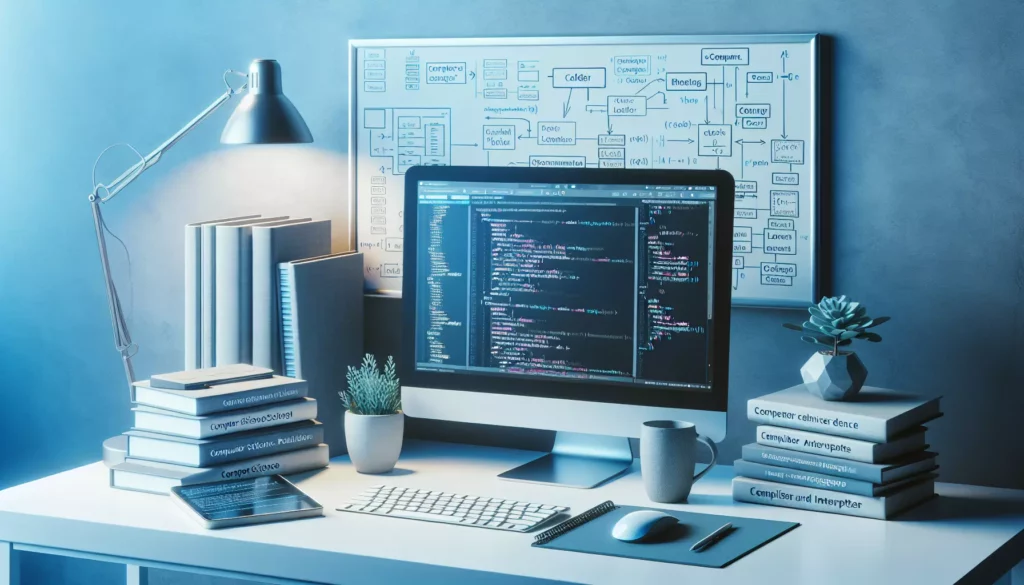
In the world of programming and software development, compilers and interpreters play a crucial role in translating human-readable code into machine-executable instructions. As aspiring programmers and computer scientists, understanding the fundamentals of compiler and interpreter design is essential for developing a deeper comprehension of how programming languages work and how our code is ultimately executed by computers. In this comprehensive guide, we’ll explore the basics of compiler and interpreter design, their differences, and their importance in the field of computer science.
What are Compilers and Interpreters?
Before delving into the design aspects, let’s first define what compilers and interpreters are:
Compilers
A compiler is a program that translates source code written in a high-level programming language (like C++, Java, or Rust) into machine code or an intermediate code that can be executed directly by a computer’s processor. The compilation process typically occurs before runtime, creating an executable file that can be run multiple times without needing to recompile.
Interpreters
An interpreter, on the other hand, is a program that directly executes instructions written in a programming or scripting language without previously compiling them into machine language. Interpreters read, analyze, and execute the source code line by line at runtime.
The Compilation Process
The compilation process typically involves several stages, each performing a specific task in transforming source code into executable machine code. Let’s examine these stages:
1. Lexical Analysis
The first stage of compilation is lexical analysis, also known as scanning or tokenization. In this phase, the compiler breaks down the source code into a sequence of tokens. Tokens are the smallest units of meaning in a programming language, such as keywords, identifiers, literals, and operators.
Here’s a simple example of how lexical analysis might work:
// Source code
int x = 5 + 3;
// Tokens
[INT] [IDENTIFIER:x] [ASSIGN] [NUMBER:5] [PLUS] [NUMBER:3] [SEMICOLON]
2. Syntax Analysis
The next stage is syntax analysis, also called parsing. During this phase, the compiler takes the sequence of tokens produced by the lexical analyzer and checks if they form a valid structure according to the grammar rules of the programming language. The output of this stage is typically an Abstract Syntax Tree (AST), which represents the hierarchical structure of the program.
For example, the AST for the previous code snippet might look like this:
ASSIGN
/ \
x ADDITION
/ \
5 3
3. Semantic Analysis
After syntax analysis, the compiler performs semantic analysis. This stage involves checking for semantic errors, such as type mismatches, undeclared variables, or invalid operations. It also enriches the AST with type information and performs type checking.
4. Intermediate Code Generation
Once the semantic analysis is complete, the compiler generates an intermediate representation of the code. This intermediate code is usually closer to machine code but is not specific to any particular machine architecture. Common forms of intermediate code include Three-Address Code (TAC) or Quadruples.
An example of Three-Address Code for our previous snippet might look like:
t1 = 5 + 3
x = t1
5. Code Optimization
The optimization phase aims to improve the intermediate code to make it more efficient. This can involve various techniques such as constant folding, dead code elimination, loop unrolling, and many others. The goal is to produce code that runs faster or uses less memory without changing its behavior.
6. Code Generation
The final stage of compilation is code generation. Here, the optimized intermediate code is translated into the target machine code or assembly language. This stage takes into account the specific architecture of the target machine, including its instruction set and memory model.
The Interpretation Process
While compilers translate the entire source code into machine code before execution, interpreters execute the code directly, line by line. The interpretation process generally involves the following steps:
1. Parsing
Similar to compilation, the interpreter first parses the source code to create an internal representation, often an AST.
2. Intermediate Representation (Optional)
Some interpreters may generate an intermediate representation of the code, such as bytecode, which can be more efficiently executed than the original source code.
3. Execution
The interpreter then executes the code directly from the AST or intermediate representation. This is done line by line or statement by statement, translating each instruction into machine code and executing it immediately.
Key Differences Between Compilers and Interpreters
Understanding the differences between compilers and interpreters is crucial for grasping their design principles:
- Execution Speed: Compiled programs generally run faster than interpreted ones because the translation to machine code is done ahead of time.
- Development Speed: Interpreted languages often allow for faster development and easier debugging, as changes can be made and tested immediately without a separate compilation step.
- Portability: Interpreted languages are often more portable, as the same source code can run on different platforms without recompilation.
- Error Detection: Compilers can catch more errors at compile-time, while interpreters may only detect certain errors at runtime.
- Memory Usage: Compiled programs typically use less memory at runtime, as they don’t need to carry the overhead of an interpreter.
Design Considerations for Compilers
When designing a compiler, several key aspects need to be considered:
1. Lexical Analysis Design
The lexical analyzer, or scanner, is typically implemented using finite automata. Regular expressions are often used to define the patterns for different tokens. Tools like Lex or Flex can be used to generate scanners automatically from these specifications.
Here’s a simple example of how token patterns might be defined:
DIGIT [0-9]
LETTER [a-zA-Z]
IDENTIFIER {LETTER}({LETTER}|{DIGIT})*
NUMBER {DIGIT}+
WHITESPACE [ \t\n]+
2. Parser Design
Parsers are often designed using context-free grammars (CFGs) and implemented using techniques like recursive descent parsing or shift-reduce parsing. Tools like Yacc or Bison can generate parsers from grammar specifications.
A simple grammar for arithmetic expressions might look like this:
expression : term
| expression '+' term
| expression '-' term
term : factor
| term '*' factor
| term '/' factor
factor : NUMBER
| '(' expression ')'
3. Symbol Table Design
The symbol table is a crucial data structure in compiler design. It stores information about identifiers in the program, such as their types, scopes, and memory locations. Efficient implementation of symbol tables (often using hash tables) is important for good compiler performance.
4. Intermediate Code Representation
Choosing an appropriate intermediate representation is important for optimization and code generation. Common choices include abstract syntax trees (ASTs), three-address code, or static single assignment (SSA) form.
5. Optimization Techniques
Implementing effective optimization techniques can significantly improve the performance of the generated code. This includes local optimizations (like constant folding), global optimizations (like dead code elimination), and loop optimizations.
6. Code Generation Strategies
The code generator must be designed to efficiently map the intermediate representation to the target machine’s instruction set. This involves instruction selection, register allocation, and handling of machine-specific details.
Design Considerations for Interpreters
When designing an interpreter, several factors come into play:
1. Parsing and AST Construction
Like compilers, interpreters need to parse the source code. However, the emphasis is often on creating an efficient in-memory representation that can be quickly traversed during execution.
2. Execution Model
Interpreters can use different execution models, such as:
- Tree-walking interpreters that directly execute the AST
- Bytecode interpreters that first compile to an intermediate bytecode
- Just-In-Time (JIT) compilers that dynamically compile frequently executed code paths
3. Memory Management
Efficient memory management is crucial for interpreters, especially for managing dynamic allocation and garbage collection.
4. Error Handling and Debugging
Interpreters need robust error handling mechanisms and often provide interactive debugging capabilities.
5. Performance Optimization
While interpreters are generally slower than compiled code, various techniques can be employed to improve performance, such as:
- Caching of frequently used values or computations
- Inline caching for method dispatch in object-oriented languages
- Optimization of common code patterns
Practical Examples
To better understand the concepts of compiler and interpreter design, let’s look at some practical examples:
Simple Compiler Example
Here’s a very basic example of how you might start implementing a simple compiler in Python:
import re
# Lexical analysis
def tokenize(code):
token_patterns = [
('NUMBER', r'\d+'),
('PLUS', r'\+'),
('MINUS', r'-'),
('MULTIPLY', r'\*'),
('DIVIDE', r'/'),
('LPAREN', r'\('),
('RPAREN', r'\)'),
('WHITESPACE', r'\s+')
]
tokens = []
while code:
for token_type, pattern in token_patterns:
match = re.match(pattern, code)
if match:
if token_type != 'WHITESPACE':
tokens.append((token_type, match.group(0)))
code = code[match.end():]
break
else:
raise ValueError(f"Invalid token: {code[0]}")
return tokens
# Syntax analysis (very simple for this example)
def parse(tokens):
# This is a placeholder for a real parser
return tokens
# Code generation (very simple for this example)
def generate_code(ast):
# This would typically generate machine code or assembly
# Here we're just returning a string representation
return ' '.join(token[1] for token in ast if token[0] != 'WHITESPACE')
# Main compilation function
def compile(source_code):
tokens = tokenize(source_code)
ast = parse(tokens)
target_code = generate_code(ast)
return target_code
# Example usage
source = "3 + 4 * (2 - 1)"
result = compile(source)
print(f"Compiled result: {result}")
This is a very simplified example and doesn’t include many of the complexities of a real compiler, but it illustrates the basic stages of compilation.
Simple Interpreter Example
Here’s a basic example of a simple interpreter for arithmetic expressions:
class Interpreter:
def __init__(self, text):
self.text = text
self.pos = 0
self.current_token = None
def error(self):
raise Exception('Error parsing input')
def get_next_token(self):
text = self.text
if self.pos > len(text) - 1:
return None
current_char = text[self.pos]
if current_char.isdigit():
self.pos += 1
return int(current_char)
if current_char == '+':
self.pos += 1
return '+'
if current_char == '-':
self.pos += 1
return '-'
self.error()
def eat(self, token_type):
if self.current_token == token_type:
self.current_token = self.get_next_token()
else:
self.error()
def expr(self):
self.current_token = self.get_next_token()
result = self.current_token
self.eat(self.current_token)
while self.current_token in ('+', '-'):
op = self.current_token
self.eat(op)
if op == '+':
result += self.current_token
else:
result -= self.current_token
self.eat(self.current_token)
return result
# Example usage
interpreter = Interpreter("3+5-2")
result = interpreter.expr()
print(f"Result: {result}")
This interpreter can handle simple addition and subtraction of single-digit numbers. It demonstrates the basic concept of parsing and interpreting code on-the-fly.
Conclusion
Understanding the basics of compiler and interpreter design is fundamental for any serious programmer or computer scientist. These concepts not only provide insight into how programming languages work but also form the foundation for many advanced topics in computer science, such as program analysis, optimization, and language design.
As you progress in your programming journey, you’ll find that a solid grasp of these concepts will enhance your ability to write efficient code, debug complex issues, and even create your own programming languages or domain-specific languages.
Remember, the field of compiler and interpreter design is vast and complex, with many advanced topics that we haven’t covered here. If you’re interested in diving deeper, consider exploring topics like type systems, static analysis, advanced optimization techniques, and just-in-time compilation.
By mastering these concepts, you’ll be well-equipped to tackle complex programming challenges and contribute to the ever-evolving field of computer science and software development. Whether you’re preparing for technical interviews at top tech companies or simply aiming to become a more proficient programmer, a strong foundation in compiler and interpreter design will serve you well throughout your career.