Understanding the Basics of CAP Theorem for System Design
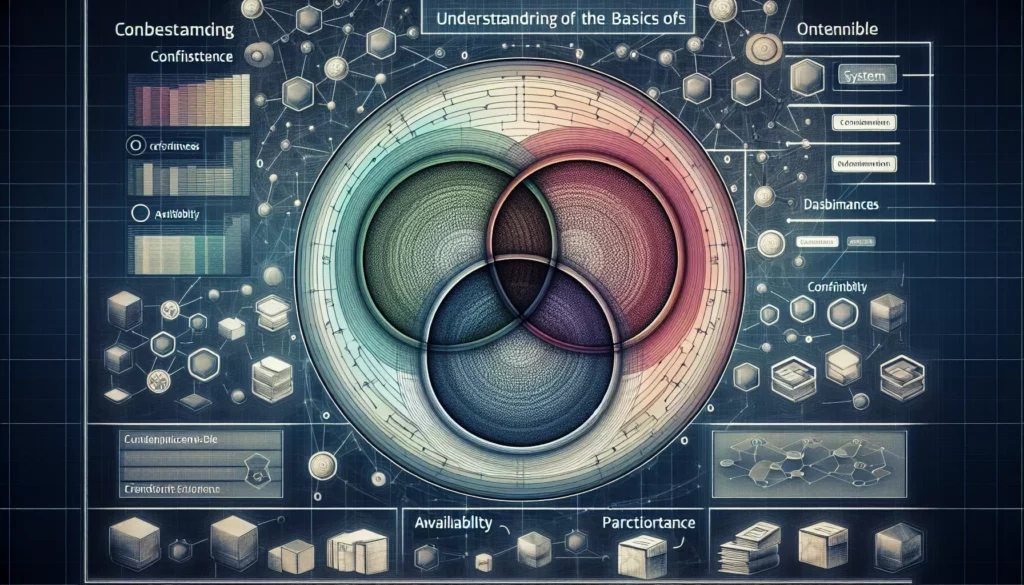
In the world of distributed systems and database design, the CAP theorem stands as a fundamental principle that guides architects and developers in making crucial decisions. As we dive into the intricacies of this theorem, it’s important to understand its significance in the context of modern software engineering, particularly for those preparing for technical interviews at major tech companies.
What is the CAP Theorem?
The CAP theorem, also known as Brewer’s theorem after computer scientist Eric Brewer, states that it is impossible for a distributed data store to simultaneously provide more than two out of the following three guarantees:
- Consistency (C): Every read receives the most recent write or an error.
- Availability (A): Every request receives a (non-error) response, without the guarantee that it contains the most recent write.
- Partition tolerance (P): The system continues to operate despite an arbitrary number of messages being dropped (or delayed) by the network between nodes.
In essence, the theorem posits that in the presence of a network partition, one has to choose between consistency and availability.
Breaking Down the Components
Consistency
Consistency in the CAP theorem refers to all nodes seeing the same data at the same time. When a write operation is performed on a consistent system, all subsequent read operations should reflect that write, regardless of which node in the distributed system the read operation is performed on.
For example, if we update a user’s profile picture in a social media application, a consistent system would ensure that this update is immediately visible to all other users, no matter which server they’re connected to.
Availability
Availability means that every request to the non-failing node in the system receives a response, without the guarantee that it contains the most recent version of the information. In other words, the system remains operational and responsive at all times, even in the face of failures.
In our social media example, an available system would ensure that users can always access their profiles and post updates, even if some servers are down or experiencing issues.
Partition Tolerance
Partition tolerance is the ability of the system to continue operating despite arbitrary partitioning due to network failures. In a distributed system, nodes may become unreachable due to network issues, and partition tolerance ensures that the system can handle such situations.
For a large-scale social media platform, partition tolerance would mean that the service remains functional even if communication between data centers in different geographic regions is temporarily lost.
The Trade-offs: CP, AP, and CA Systems
According to the CAP theorem, a distributed system can only guarantee two of the three properties at any given time. This leads to three possible combinations:
CP (Consistency and Partition Tolerance)
CP systems prioritize consistency and partition tolerance over availability. In the event of a network partition, the system will shut down the inconsistent nodes to maintain consistency across the available nodes.
Example: A banking system that prioritizes consistent account balances across all nodes, even if it means some nodes become temporarily unavailable during network issues.
Implementing a CP System
Here’s a simplified example of how you might implement a CP system using a distributed lock:
import threading
class CPSystem:
def __init__(self):
self.data = {}
self.lock = threading.Lock()
def write(self, key, value):
with self.lock:
self.data[key] = value
# Simulate writing to all nodes
print(f"Writing {key}:{value} to all nodes")
def read(self, key):
with self.lock:
# Simulate reading from any available node
return self.data.get(key, None)
# Usage
cp_system = CPSystem()
cp_system.write("user_1", "John Doe")
print(cp_system.read("user_1")) # Output: John Doe
In this example, the lock ensures that all write operations are consistent across all nodes before allowing any read operations.
AP (Availability and Partition Tolerance)
AP systems prioritize availability and partition tolerance over consistency. These systems will return the most recent version of the data available on a node, which might not be the latest version across all nodes.
Example: A content delivery network (CDN) that prioritizes serving content quickly, even if it means some users might see slightly outdated versions of a website.
Implementing an AP System
Here’s a simplified example of an AP system using eventual consistency:
import threading
import time
class APSystem:
def __init__(self):
self.data = {}
self.update_queue = []
threading.Thread(target=self.background_sync, daemon=True).start()
def write(self, key, value):
self.data[key] = value
self.update_queue.append((key, value))
print(f"Writing {key}:{value} to local node")
def read(self, key):
return self.data.get(key, None)
def background_sync(self):
while True:
if self.update_queue:
key, value = self.update_queue.pop(0)
# Simulate writing to other nodes
print(f"Syncing {key}:{value} to other nodes")
time.sleep(1) # Sync every second
# Usage
ap_system = APSystem()
ap_system.write("user_1", "Jane Doe")
print(ap_system.read("user_1")) # Output: Jane Doe
# Note: The background sync happens asynchronously
In this AP system, writes are immediately available on the local node, while a background process handles eventual consistency across all nodes.
CA (Consistency and Availability)
CA systems prioritize consistency and availability but cannot tolerate network partitions. In practice, CA systems are rare in distributed environments because network partitions are essentially unavoidable.
Example: A single-node database system that doesn’t need to handle network partitions but provides both consistency and availability.
Implementing a CA System
Here’s a simplified example of a CA system (note that this is not truly distributed):
import threading
class CASystem:
def __init__(self):
self.data = {}
self.lock = threading.Lock()
def write(self, key, value):
with self.lock:
self.data[key] = value
print(f"Writing {key}:{value}")
def read(self, key):
with self.lock:
return self.data.get(key, None)
# Usage
ca_system = CASystem()
ca_system.write("user_1", "Alice")
print(ca_system.read("user_1")) # Output: Alice
This CA system provides consistency and availability but would not work in a distributed environment with network partitions.
Practical Implications of CAP Theorem
Understanding the CAP theorem is crucial for system designers and developers, especially when working on large-scale distributed systems. Here are some practical implications:
1. Choosing the Right Database
Different databases are designed with different CAP priorities:
- CP databases (e.g., MongoDB, HBase) prioritize consistency and partition tolerance.
- AP databases (e.g., Cassandra, CouchDB) prioritize availability and partition tolerance.
- CA databases (e.g., traditional RDBMSs like MySQL, PostgreSQL) work well in single-node setups but struggle with partitions in distributed environments.
2. Designing for Scale
As systems grow and become more distributed, partition tolerance becomes increasingly important. This often leads to a choice between consistency and availability in large-scale systems.
3. Understanding Business Requirements
The choice between CP and AP often depends on business requirements:
- Financial systems often prioritize consistency (CP) to ensure accurate transactions.
- Social media platforms might prioritize availability (AP) to ensure users can always access the service, even if they occasionally see outdated information.
4. Implementing Eventual Consistency
Many modern distributed systems use eventual consistency as a way to balance the CAP trade-offs. This approach allows for high availability while providing consistency over time.
Beyond CAP: PACELC Theorem
While the CAP theorem provides a fundamental understanding of distributed systems, it doesn’t cover all scenarios. The PACELC theorem extends CAP by considering system behavior both in the presence of partitions and in the absence of partitions:
- If there is a partition (P), a system must choose between availability (A) and consistency (C).
- Else (E), when the system is running normally in the absence of partitions, the system can choose between latency (L) and consistency (C).
This extension helps in understanding system design choices in more nuanced scenarios, particularly when network partitions are not present.
Implementing CAP-Aware Systems
When designing systems with CAP theorem in mind, consider the following strategies:
1. Use of Quorum-Based Systems
Quorum-based systems can help balance consistency and availability. For example, in a system with 5 nodes, you might require a write to be acknowledged by 3 nodes before considering it successful. This provides a level of consistency while maintaining some availability in the face of node failures.
2. Implementing Version Vectors
Version vectors can help track the state of data across distributed nodes, allowing systems to detect and resolve conflicts:
class VersionVector:
def __init__(self):
self.vector = {}
def update(self, node_id):
self.vector[node_id] = self.vector.get(node_id, 0) + 1
def merge(self, other_vector):
for node_id, version in other_vector.vector.items():
self.vector[node_id] = max(self.vector.get(node_id, 0), version)
def is_concurrent(self, other_vector):
return not (self < other_vector or other_vector < self)
def __lt__(self, other_vector):
return all(self.vector.get(k, 0) <= other_vector.vector.get(k, 0)
for k in set(self.vector) | set(other_vector.vector))
# Usage
v1 = VersionVector()
v2 = VersionVector()
v1.update("node1")
v2.update("node2")
print(v1.is_concurrent(v2)) # Output: True
v1.merge(v2)
print(v1.vector) # Output: {'node1': 1, 'node2': 1}
3. Implementing a Distributed Lock
For systems prioritizing consistency, a distributed lock can ensure that only one node can modify data at a time:
import threading
import time
class DistributedLock:
def __init__(self):
self.lock = threading.Lock()
self.owner = None
def acquire(self, node_id, timeout=5):
start_time = time.time()
while time.time() - start_time < timeout:
if self.lock.acquire(blocking=False):
self.owner = node_id
return True
time.sleep(0.1)
return False
def release(self, node_id):
if self.owner == node_id:
self.owner = None
self.lock.release()
return True
return False
# Usage
lock = DistributedLock()
def node_operation(node_id):
if lock.acquire(node_id):
print(f"Node {node_id} acquired the lock")
time.sleep(1) # Simulate some work
lock.release(node_id)
print(f"Node {node_id} released the lock")
else:
print(f"Node {node_id} failed to acquire the lock")
# Simulate multiple nodes trying to acquire the lock
threads = [threading.Thread(target=node_operation, args=(i,)) for i in range(3)]
for t in threads:
t.start()
for t in threads:
t.join()
CAP Theorem in Technical Interviews
For those preparing for technical interviews, especially at major tech companies, understanding the CAP theorem is crucial. Here are some tips for discussing CAP in interviews:
- Understand the trade-offs: Be prepared to discuss why you might choose CP over AP or vice versa in different scenarios.
- Real-world examples: Familiarize yourself with how popular systems (e.g., databases, cloud services) implement CAP trade-offs.
- System design questions: In system design interviews, consider CAP when making architectural decisions and be ready to justify your choices.
- Coding implementations: Practice implementing simple distributed systems that demonstrate CAP principles.
- Beyond CAP: Show your depth of knowledge by discussing related concepts like PACELC or eventual consistency.
Conclusion
The CAP theorem is a fundamental concept in distributed systems design that helps engineers make informed decisions about consistency, availability, and partition tolerance. By understanding the trade-offs involved, developers can create more robust and scalable systems that meet specific business requirements.
As you continue your journey in software engineering and prepare for technical interviews, remember that the CAP theorem is not just theoretical knowledge. It has practical implications in the design and implementation of real-world systems. By mastering this concept and its applications, you’ll be better equipped to tackle complex distributed systems challenges and excel in technical discussions with potential employers.
Keep practicing, implementing, and exploring the nuances of CAP in various scenarios. This deep understanding will not only help you in interviews but also in your future role as a software engineer working on large-scale, distributed systems.