Understanding System Design Questions in Technical Interviews: A Comprehensive Guide
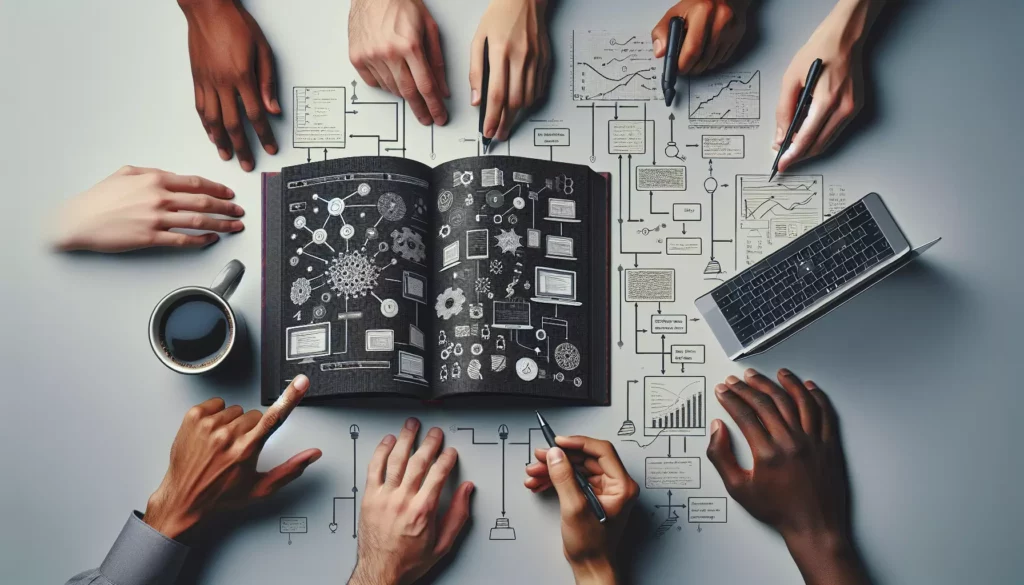
In the competitive landscape of tech industry job interviews, particularly for positions at major companies like FAANG (Facebook, Amazon, Apple, Netflix, Google), system design questions have become an integral part of the evaluation process. These questions assess a candidate’s ability to think at scale, make architectural decisions, and design robust, efficient systems. For many aspiring software engineers and even experienced professionals, system design interviews can be daunting. This comprehensive guide aims to demystify system design questions, provide strategies for tackling them, and offer insights into what interviewers are really looking for.
What Are System Design Questions?
System design questions are open-ended problems that require candidates to design a large-scale distributed system. These questions typically involve creating high-level architectures for real-world applications or services. Some common examples include:
- Design a social media platform like Facebook
- Create a file storage and sharing service similar to Dropbox
- Develop a video streaming platform like YouTube
- Design a ride-sharing application like Uber
- Build a global chat application like WhatsApp
The goal of these questions is not to produce a perfect, detailed implementation, but rather to demonstrate your ability to think through complex problems, make trade-offs, and communicate your ideas effectively.
Why Are System Design Questions Important?
System design questions serve several purposes in the interview process:
- Assessing scalability thinking: They evaluate your ability to design systems that can handle millions or even billions of users.
- Testing problem-solving skills: These questions require you to break down complex problems into manageable components.
- Evaluating communication skills: Your ability to explain your thoughts and decisions clearly is crucial.
- Gauging technical knowledge: They test your understanding of various technologies, protocols, and architectural patterns.
- Simulating real-world scenarios: These questions often mirror actual challenges that companies face.
Key Components of System Design
To effectively answer system design questions, it’s essential to understand the key components that make up large-scale systems:
1. Load Balancing
Load balancers distribute incoming network traffic across multiple servers to ensure no single server becomes overwhelmed. This improves the reliability and availability of applications.
// Simple pseudo-code for a round-robin load balancer
class LoadBalancer {
private List<Server> servers;
private int currentIndex = 0;
public Server getNextServer() {
Server server = servers.get(currentIndex);
currentIndex = (currentIndex + 1) % servers.size();
return server;
}
}
2. Caching
Caching involves storing copies of frequently accessed data in a fast-access storage layer. This reduces the load on databases and improves response times.
// Example of using a cache in Python
import redis
cache = redis.Redis(host='localhost', port=6379)
def get_user_data(user_id):
# Try to get data from cache
cached_data = cache.get(user_id)
if cached_data:
return cached_data
# If not in cache, fetch from database
data = fetch_from_database(user_id)
# Store in cache for future requests
cache.set(user_id, data, ex=3600) # expire in 1 hour
return data
3. Database Sharding
Sharding is a method of splitting and storing a single logical dataset in multiple databases. This allows for horizontal scaling of the database tier.
// Pseudo-code for a simple sharding strategy
function getDatabaseShard(userId) {
return userId % NUMBER_OF_SHARDS;
}
function storeUser(user) {
shardId = getDatabaseShard(user.id);
database = getDatabaseConnection(shardId);
database.insert(user);
}
4. Content Delivery Networks (CDNs)
CDNs are distributed networks of servers that deliver content to users based on their geographic location, improving load times and reducing bandwidth costs.
5. Microservices Architecture
Microservices architecture involves designing an application as a collection of loosely coupled services, each running in its own process and communicating via lightweight mechanisms.
// Example of a microservice in Node.js
const express = require('express');
const app = express();
app.get('/api/users', (req, res) => {
// Logic to fetch users
res.json({ users: [/* user data */] });
});
app.listen(3000, () => {
console.log('User microservice running on port 3000');
});
Approach to Solving System Design Questions
When faced with a system design question in an interview, follow these steps to structure your approach:
1. Clarify Requirements
Begin by asking questions to understand the scope and constraints of the system you’re designing. Some key questions to consider:
- What are the core features required?
- What is the expected scale (number of users, data volume)?
- What are the performance requirements (latency, throughput)?
- Are there any specific technical constraints or preferences?
2. Sketch the High-Level Design
Start with a basic outline of the system’s architecture. This might include:
- Client (web, mobile, etc.)
- Load balancers
- Application servers
- Databases
- Caching layers
- Any other necessary components
3. Deep Dive into Core Components
Identify the most critical components of the system and discuss them in more detail. This might involve:
- Explaining the data model
- Discussing API design
- Detailing the caching strategy
- Describing how data is sharded or partitioned
4. Address Scalability
Discuss how the system will handle growth. This could include:
- Horizontal scaling of application servers
- Database replication and sharding
- Caching strategies
- Use of CDNs for content delivery
5. Identify and Resolve Bottlenecks
Consider potential issues that could arise as the system scales and how to address them. This might involve:
- Optimizing database queries
- Implementing asynchronous processing for time-consuming tasks
- Using message queues to decouple system components
// Example of using a message queue in Python with RabbitMQ
import pika
connection = pika.BlockingConnection(pika.ConnectionParameters('localhost'))
channel = connection.channel()
channel.queue_declare(queue='task_queue', durable=True)
def process_task(task):
# Time-consuming task processing logic here
print(f"Processing task: {task}")
def callback(ch, method, properties, body):
task = body.decode()
process_task(task)
ch.basic_ack(delivery_tag=method.delivery_tag)
channel.basic_qos(prefetch_count=1)
channel.basic_consume(queue='task_queue', on_message_callback=callback)
print('Waiting for messages. To exit press CTRL+C')
channel.start_consuming()
Common Pitfalls to Avoid
When answering system design questions, be wary of these common mistakes:
1. Diving into Details Too Quickly
Don’t jump into specific implementation details before establishing the high-level architecture. Start broad and then narrow down.
2. Ignoring Scalability
Always consider how your design will handle growth. A solution that works for 100 users might fail completely with 1 million users.
3. Overlooking Trade-offs
Every design decision involves trade-offs. Be prepared to discuss the pros and cons of your choices.
4. Not Asking Clarifying Questions
Don’t make assumptions about the requirements. Ask questions to ensure you understand the problem fully.
5. Failing to Justify Design Decisions
Be prepared to explain the reasoning behind your architectural choices.
Advanced Topics in System Design
As you become more comfortable with basic system design concepts, consider exploring these advanced topics:
1. Consistent Hashing
Consistent hashing is a technique used in distributed systems to minimize the number of keys that need to be remapped when a hash table is resized. It’s particularly useful in distributed caching systems.
import hashlib
class ConsistentHash:
def __init__(self, nodes, virtual_nodes=100):
self.nodes = nodes
self.virtual_nodes = virtual_nodes
self.ring = {}
self._build_ring()
def _build_ring(self):
for node in self.nodes:
for i in range(self.virtual_nodes):
key = self._hash(f"{node}:{i}")
self.ring[key] = node
def _hash(self, key):
return hashlib.md5(key.encode()).hexdigest()
def get_node(self, key):
if not self.ring:
return None
hash_key = self._hash(key)
for node_key in sorted(self.ring.keys()):
if node_key > hash_key:
return self.ring[node_key]
return self.ring[sorted(self.ring.keys())[0]]
# Usage
nodes = ['node1', 'node2', 'node3']
ch = ConsistentHash(nodes)
print(ch.get_node('object_key'))
2. CAP Theorem
The CAP theorem states that it is impossible for a distributed data store to simultaneously provide more than two out of the following three guarantees:
- Consistency: Every read receives the most recent write or an error
- Availability: Every request receives a (non-error) response
- Partition tolerance: The system continues to operate despite network partitions
Understanding the CAP theorem is crucial when designing distributed systems and making decisions about data consistency and availability.
3. Event-Driven Architecture
Event-driven architecture is a software design pattern in which decoupled components can asynchronously publish and subscribe to events.
// Simple event emitter in JavaScript
class EventEmitter {
constructor() {
this.listeners = {};
}
on(event, callback) {
if (!this.listeners[event]) {
this.listeners[event] = [];
}
this.listeners[event].push(callback);
}
emit(event, data) {
if (this.listeners[event]) {
this.listeners[event].forEach(callback => callback(data));
}
}
}
// Usage
const emitter = new EventEmitter();
emitter.on('userCreated', user => console.log(`New user created: ${user.name}`));
emitter.emit('userCreated', { name: 'John Doe' });
4. CQRS (Command Query Responsibility Segregation)
CQRS is an architectural pattern that separates read and write operations for a data store. This can lead to more scalable and performant systems, especially when read and write workloads have different characteristics.
5. Eventual Consistency
Eventual consistency is a consistency model used in distributed computing to achieve high availability. It states that if no new updates are made to a given data item, eventually all accesses to that item will return the last updated value.
Real-World System Design Examples
To better understand how these concepts come together in practice, let’s look at high-level designs for a few real-world systems:
1. Designing a URL Shortener (like bit.ly)
Key components:
- Load Balancer
- Application Servers
- Database (for storing long URL to short URL mappings)
- Cache (for frequently accessed URLs)
Considerations:
- How to generate unique short URLs
- Handling high read traffic
- Analytics and tracking
2. Designing a News Feed System (like Facebook’s News Feed)
Key components:
- User Service
- Post Service
- News Feed Generation Service
- Notification Service
- Database (for user data, posts, etc.)
- Cache (for news feed items)
- Message Queue (for asynchronous processing)
Considerations:
- Efficient feed generation algorithms
- Real-time updates
- Handling large volumes of data
3. Designing a Distributed File Storage System (like Dropbox)
Key components:
- Client Application
- Load Balancer
- Metadata Database
- File Storage Servers
- Synchronization Service
- Notification Service
Considerations:
- Efficient file chunking and de-duplication
- Handling large file uploads and downloads
- Ensuring data consistency across devices
- Implementing file versioning and conflict resolution
Preparing for System Design Interviews
To excel in system design interviews, consider the following preparation strategies:
1. Study Existing Systems
Analyze popular services and platforms to understand their architectures. Many companies publish engineering blogs that provide insights into their system designs.
2. Practice Regularly
Solve system design problems regularly. Start with simpler systems and gradually move to more complex ones.
3. Learn from Others
Participate in online forums, attend tech talks, or join study groups to learn from others’ experiences and perspectives.
4. Stay Updated
Keep abreast of new technologies, architectural patterns, and industry best practices.
5. Develop a Structured Approach
Create a personal framework for tackling system design questions. This could include a checklist of components to consider or a step-by-step process for breaking down problems.
Conclusion
System design questions are a crucial part of technical interviews, especially for senior positions and at major tech companies. They assess a candidate’s ability to think at scale, make architectural decisions, and communicate complex ideas effectively. By understanding the key components of distributed systems, following a structured approach to problem-solving, and staying informed about current technologies and best practices, you can significantly improve your performance in system design interviews.
Remember, there’s rarely a single “correct” answer to system design questions. The goal is to demonstrate your thought process, your ability to make and justify design decisions, and your understanding of the trade-offs involved in building large-scale systems. With practice and preparation, you can develop the skills needed to confidently tackle even the most challenging system design questions in your technical interviews.