Understanding Suffix Trees and Arrays: Advanced Data Structures for String Processing
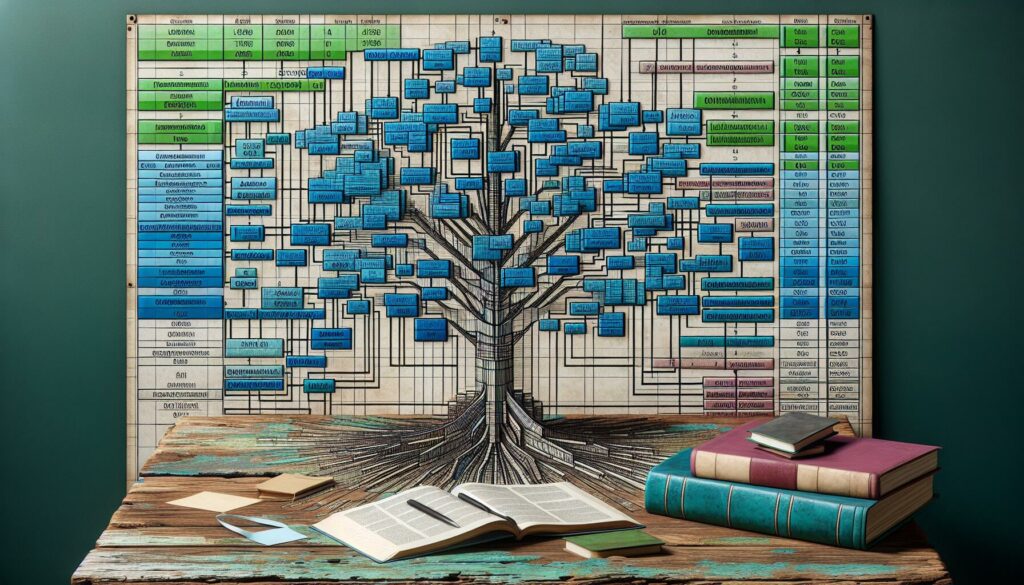
In the world of computer science and algorithmic problem-solving, efficient string processing is a crucial skill. Whether you’re preparing for technical interviews at top tech companies or working on complex software projects, understanding advanced data structures like suffix trees and suffix arrays can give you a significant edge. These powerful tools enable fast pattern matching, substring searches, and various other string-related operations. In this comprehensive guide, we’ll dive deep into suffix trees and arrays, exploring their structure, construction, applications, and implementation details.
What are Suffix Trees?
A suffix tree is a compressed trie-like data structure that represents all suffixes of a given string. It provides a space-efficient way to store and query substrings, making it an invaluable tool for various string processing tasks. The key features of a suffix tree include:
- Each internal node (except the root) has at least two children
- Each edge is labeled with a non-empty substring of the original string
- No two edges starting from a node can have edge labels beginning with the same character
- The concatenation of edge labels from the root to a leaf node represents a suffix of the original string
Construction of Suffix Trees
There are several algorithms for constructing suffix trees, with varying time and space complexities. One of the most well-known is Ukkonen’s algorithm, which builds the suffix tree in O(n) time and space for a string of length n. Here’s a high-level overview of the process:
- Start with an empty tree and incrementally add suffixes
- Use “active points” and “suffix links” to efficiently navigate the tree during construction
- Implement “trick” techniques to handle edge-case scenarios and maintain linear time complexity
While the full implementation of Ukkonen’s algorithm is beyond the scope of this article, here’s a simplified example of a suffix tree node structure in Python:
class SuffixTreeNode:
def __init__(self):
self.children = {}
self.suffix_link = None
self.start = -1
self.end = -1
self.suffix_index = -1
class SuffixTree:
def __init__(self, text):
self.text = text
self.root = SuffixTreeNode()
self.build_tree()
def build_tree(self):
# Implementation of Ukkonen's algorithm goes here
pass
Applications of Suffix Trees
Suffix trees have numerous applications in string processing and bioinformatics. Some of the key use cases include:
- Substring Search: Find if a pattern exists in a text in O(m) time, where m is the length of the pattern.
- Longest Common Substring: Find the longest substring common to two or more strings.
- Longest Repeated Substring: Find the longest substring that appears more than once in a text.
- Palindrome Detection: Identify all palindromic substrings in a given string.
- Genome Analysis: Perform various operations on DNA sequences, such as finding repeats or comparing genomes.
What are Suffix Arrays?
A suffix array is a sorted array of all suffixes of a string. It provides functionality similar to suffix trees but with a simpler structure and often lower space requirements. The key features of a suffix array include:
- It’s an integer array storing the starting indices of sorted suffixes
- Often accompanied by a Longest Common Prefix (LCP) array for enhanced functionality
- Requires O(n) space for a string of length n
Construction of Suffix Arrays
There are several algorithms for constructing suffix arrays, ranging from simple O(n^2 log n) approaches to more complex linear-time algorithms. One popular method is the SA-IS (Suffix Array Induced Sorting) algorithm, which achieves O(n) time complexity. Here’s a basic implementation of a naive suffix array construction in Python:
def build_suffix_array(text):
suffixes = [(text[i:], i) for i in range(len(text))]
suffixes.sort()
return [index for _, index in suffixes]
# Example usage
text = "banana"
sa = build_suffix_array(text)
print(sa) # Output: [5, 3, 1, 0, 4, 2]
This simple implementation has O(n^2 log n) time complexity due to the sorting step. For large strings, more efficient algorithms like SA-IS should be used.
Applications of Suffix Arrays
Suffix arrays have many of the same applications as suffix trees, often with lower space requirements. Some key use cases include:
- Pattern Matching: Find all occurrences of a pattern in a text in O(m log n + occ) time, where m is the pattern length, n is the text length, and occ is the number of occurrences.
- Longest Common Prefix: Compute the longest common prefix between any two suffixes efficiently.
- Burrows-Wheeler Transform: Construct the BWT, which is useful in data compression and bioinformatics.
- Longest Repeated Substring: Find the longest substring that appears multiple times in the text.
- String Compression: Identify repeated substrings for efficient compression algorithms.
Comparison: Suffix Trees vs. Suffix Arrays
Both suffix trees and suffix arrays are powerful data structures for string processing, but they have some key differences:
Aspect | Suffix Trees | Suffix Arrays |
---|---|---|
Space Complexity | O(n) but with a larger constant factor | O(n) with a smaller constant factor |
Construction Time | O(n) using advanced algorithms | O(n) using advanced algorithms |
Ease of Implementation | More complex | Generally simpler |
Query Time | Often O(m) for pattern matching | Often O(m log n) for pattern matching |
Flexibility | More flexible for complex string operations | Less flexible but sufficient for many tasks |
The choice between suffix trees and suffix arrays often depends on the specific requirements of your application, such as space constraints, query patterns, and implementation complexity.
Advanced Topics and Optimizations
As you delve deeper into suffix trees and arrays, you’ll encounter various advanced topics and optimizations that can further enhance their performance and applicability:
1. Compressed Suffix Trees
Compressed suffix trees aim to reduce the space requirements of traditional suffix trees while maintaining their functionality. Techniques like using sparse suffix trees or implementing the tree using compressed data structures can significantly reduce memory usage.
2. FM-Index
The FM-Index (Full-text index in Minute space) is a compressed full-text index based on the Burrows-Wheeler Transform. It combines the functionality of suffix arrays with the compression benefits of the BWT, allowing for efficient pattern matching in compressed space.
3. Wavelet Trees
Wavelet trees are versatile data structures that can be used to enhance the functionality of suffix arrays. They allow for efficient rank and select operations, which can speed up various string processing tasks.
4. Suffix Trays
Suffix trays are a hybrid data structure that combines elements of both suffix trees and suffix arrays. They aim to provide a balance between the space efficiency of suffix arrays and the query efficiency of suffix trees.
5. Parallel and External Memory Algorithms
For processing extremely large strings or datasets that don’t fit in main memory, parallel algorithms and external memory techniques have been developed for both suffix tree and suffix array construction and querying.
Implementing Suffix Trees and Arrays in Practice
When it comes to implementing suffix trees and arrays in real-world applications, there are several considerations and best practices to keep in mind:
1. Choose the Right Data Structure
Carefully evaluate whether a suffix tree or suffix array is more appropriate for your specific use case. Consider factors like space constraints, query patterns, and the need for additional operations like longest common prefix computations.
2. Use Existing Libraries
For production use, consider using well-tested libraries that implement suffix trees and arrays. Some popular options include:
- SDSL (Succinct Data Structure Library) for C++
- Suffix Tree module in BioPython for bioinformatics applications
- Java String Processing Library (JSP) for Java implementations
3. Optimize for Memory Usage
When working with large strings, memory usage can become a bottleneck. Consider techniques like:
- Using integer identifiers instead of storing actual substrings in suffix tree edges
- Implementing lazy loading for suffix array construction
- Using bit-level optimizations to reduce memory footprint
4. Handling Large Alphabets
If your application deals with strings over large alphabets (e.g., Unicode text), consider alphabet reduction techniques or specialized data structures designed for large alphabets.
5. Testing and Validation
Implement thorough testing for your suffix tree or array implementation. Use both small, hand-crafted examples and large, randomly generated strings to ensure correctness and performance.
Real-world Applications and Case Studies
To better understand the practical impact of suffix trees and arrays, let’s explore some real-world applications and case studies:
1. Bioinformatics: Genome Assembly and Analysis
In the field of bioinformatics, suffix trees and arrays play a crucial role in various genomic analyses:
- Genome Assembly: Suffix trees are used in de novo genome assembly algorithms to efficiently identify overlapping DNA fragments.
- Read Mapping: Suffix arrays and FM-indexes are employed in tools like Bowtie and BWA for fast alignment of DNA sequencing reads to reference genomes.
- Repeat Detection: Identifying repetitive sequences in genomes, which is crucial for understanding genome structure and evolution.
2. Information Retrieval: Full-Text Search Engines
Suffix arrays and related data structures are used in full-text search engines to enable fast substring searches:
- Inverted Indexes: While not directly a suffix array, inverted indexes used in search engines share some similarities and can be enhanced with suffix array techniques.
- Phrase Queries: Suffix arrays can speed up phrase searches in large document collections.
3. Data Compression
Suffix trees and arrays contribute to various data compression techniques:
- LZ77 Compression: Suffix trees can be used to implement efficient LZ77 compression, which forms the basis of popular algorithms like DEFLATE (used in ZIP files).
- Burrows-Wheeler Transform: Suffix arrays are integral to constructing the BWT, which is used in compression algorithms like bzip2.
4. Plagiarism Detection
Suffix data structures can be employed in plagiarism detection systems:
- Document Comparison: Quickly identify common substrings between documents to detect potential plagiarism.
- Source Code Analysis: Detect code duplication and potential copyright infringements in software projects.
Future Directions and Research
The field of string processing and suffix-based data structures continues to evolve. Some exciting areas of ongoing research and future directions include:
1. Compressed Text Indexing
Developing even more space-efficient index structures that can operate directly on compressed text without full decompression.
2. Streaming and Online Algorithms
Creating suffix structures that can be efficiently updated as new text is appended, enabling real-time processing of data streams.
3. Approximate String Matching
Extending suffix-based data structures to handle fuzzy matching and error-tolerant string searches more efficiently.
4. Parallel and Distributed Processing
Developing algorithms and data structures optimized for modern hardware architectures, including multi-core CPUs, GPUs, and distributed systems.
5. Integration with Machine Learning
Exploring ways to combine suffix-based structures with machine learning techniques for enhanced text analysis and natural language processing tasks.
Conclusion
Suffix trees and suffix arrays are fundamental data structures in the world of string processing and algorithmic problem-solving. Their ability to efficiently handle complex string operations makes them invaluable tools for a wide range of applications, from bioinformatics to information retrieval and data compression.
As you progress in your coding journey and prepare for technical interviews, especially at top tech companies, a deep understanding of these data structures can set you apart. They not only demonstrate your grasp of advanced algorithms but also showcase your ability to tackle complex, real-world problems efficiently.
Remember that mastering these concepts requires practice and hands-on implementation. Try to implement basic versions of suffix trees and arrays, experiment with different construction algorithms, and apply them to various string processing problems. As you gain confidence, explore the more advanced topics and optimizations we’ve discussed.
The field of string processing is constantly evolving, with new techniques and optimizations being developed. Stay curious, keep learning, and don’t hesitate to dive into research papers and advanced resources to deepen your knowledge. With a solid understanding of suffix trees and arrays, you’ll be well-equipped to tackle a wide range of string-related challenges in your coding career.