Understanding String Interning and Its Impact on Performance
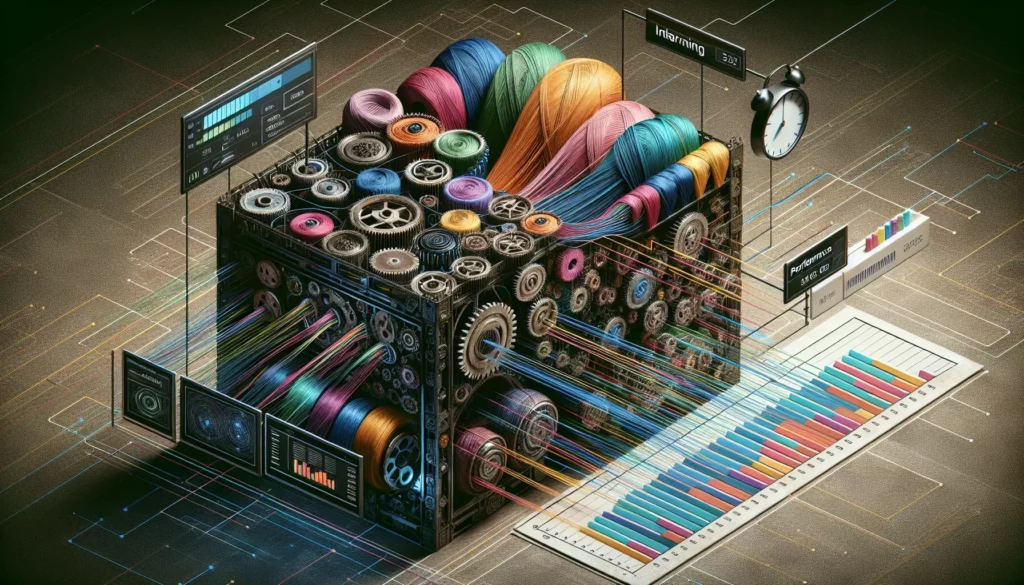
In the world of programming, particularly when working with languages like Java, C#, and Python, you might have come across the concept of string interning. This powerful feature can significantly impact your code’s performance and memory usage, yet it’s often overlooked or misunderstood. In this comprehensive guide, we’ll dive deep into string interning, exploring what it is, how it works, and why it matters for efficient coding.
What is String Interning?
String interning is a method of storing only one copy of each distinct string value in memory. Interned strings are stored in a special memory area, often called the “string pool” or “intern pool”. When you create a string, the system checks if an identical string already exists in the pool. If it does, instead of creating a new object, it returns a reference to the existing string.
This process can lead to significant memory savings and performance improvements, especially in applications that deal with large amounts of textual data or perform frequent string comparisons.
How String Interning Works
To understand string interning better, let’s break down the process:
- When a string is created, the system checks the string pool.
- If an identical string is found, a reference to that existing string is returned.
- If no match is found, the new string is added to the pool and a reference is returned.
Here’s a simple example in Java:
String s1 = "Hello";
String s2 = "Hello";
String s3 = new String("Hello");
System.out.println(s1 == s2); // true
System.out.println(s1 == s3); // false
System.out.println(s1 == s3.intern()); // true
In this example, s1
and s2
refer to the same interned string. s3
is a new string object, but s3.intern()
returns the interned version, which is the same as s1
and s2
.
String Interning in Different Languages
Java
In Java, string literals are automatically interned. The String.intern()
method allows you to manually intern strings created at runtime.
String s1 = "Hello"; // Automatically interned
String s2 = new String("Hello").intern(); // Manually interned
System.out.println(s1 == s2); // true
C#
C# also interns string literals automatically. You can use the String.Intern()
method to manually intern strings.
string s1 = "Hello"; // Automatically interned
string s2 = string.Intern("Hello"); // Manually interned
Console.WriteLine(object.ReferenceEquals(s1, s2)); // True
Python
Python interns some strings automatically, particularly short strings and identifiers. However, the behavior can be implementation-dependent.
s1 = "Hello"
s2 = "Hello"
print(s1 is s2) # True (usually, but not guaranteed)
s3 = "Hello, World!"
s4 = "Hello, World!"
print(s3 is s4) # May be True or False, depending on implementation
Benefits of String Interning
1. Memory Efficiency
By storing only one copy of each unique string, string interning can significantly reduce memory usage, especially in applications that deal with large amounts of repetitive text data.
2. Faster String Comparisons
When strings are interned, comparing them becomes as simple as comparing references, which is much faster than comparing the contents of the strings.
String s1 = "Hello".intern();
String s2 = "Hello".intern();
// This comparison is now just a reference comparison
if (s1 == s2) {
System.out.println("Strings are equal");
}
3. Improved Performance in Collections
Data structures like HashSet
and HashMap
can benefit from interned strings, as they can use reference equality instead of equals()
for comparisons.
Potential Drawbacks and Considerations
1. Increased Startup Time
Interning strings takes time, which can increase the startup time of your application, especially if you’re interning a large number of strings.
2. Memory Pressure on the Permanent Generation (Java)
In older versions of Java (before Java 8), interned strings were stored in the Permanent Generation, which had a fixed size. This could lead to OutOfMemoryError
if too many strings were interned.
3. Risk of Memory Leaks
Interned strings stay in memory for the lifetime of the application. If you intern too many unique strings, it can lead to increased memory usage over time.
Best Practices for String Interning
1. Use for Frequently Compared Strings
Intern strings that you’ll be comparing frequently. This is particularly useful for things like enum values, constants, or frequently used identifiers.
2. Be Cautious with User Input
Be careful about interning strings from user input or other external sources. This could potentially be used as a vector for denial-of-service attacks by forcing your application to store a large number of unique strings.
3. Profile Before Optimizing
Always profile your application before deciding to manually intern strings. The benefits of interning depend on your specific use case, and in some scenarios, the overhead might outweigh the benefits.
4. Use Language-Specific Features
Take advantage of language-specific features. For example, in Java, you can use the -XX:+UseStringDeduplication
JVM option to automatically deduplicate strings in the heap.
Advanced Topics in String Interning
String Interning and Garbage Collection
In modern Java versions (since Java 7), interned strings are no longer stored in the Permanent Generation but in the regular heap. This means they can be garbage collected if there are no more references to them.
public class StringInternGC {
public static void main(String[] args) {
for (int i = 0; i < 100000; i++) {
String.valueOf(i).intern();
}
System.gc();
// Many of these interned strings can now be garbage collected
}
}
String Interning in Distributed Systems
In distributed systems, string interning can be used to reduce network traffic. By sending interned string identifiers instead of full string contents, you can significantly reduce the amount of data transferred between nodes.
Custom String Interning Implementations
For more control over the interning process, you can implement your own string interning mechanism. This can be useful if you want to limit the number of interned strings or implement a custom eviction policy.
import java.util.WeakHashMap;
public class CustomStringInterner {
private final WeakHashMap<String, String> pool = new WeakHashMap<>();
public String intern(String s) {
String existing = pool.get(s);
if (existing == null) {
pool.put(s, s);
return s;
}
return existing;
}
}
String Interning in Real-World Scenarios
Database Query Optimization
In database systems, string interning can be used to optimize query processing. By interning column names and frequently used string values, databases can perform faster comparisons and reduce memory usage.
XML and JSON Processing
When parsing large XML or JSON documents, interning repeated element or property names can lead to significant memory savings and faster processing.
Compiler and Interpreter Design
Compilers and interpreters often use string interning to efficiently store and compare identifiers, keywords, and literals in the source code.
Benchmarking String Interning
To truly understand the impact of string interning on your specific application, it’s crucial to benchmark. Here’s a simple benchmark in Java that compares the performance of interned and non-interned strings:
public class StringInternBenchmark {
private static final int ITERATIONS = 10_000_000;
public static void main(String[] args) {
// Prepare strings
String[] strings = new String[ITERATIONS];
for (int i = 0; i < ITERATIONS; i++) {
strings[i] = "String" + (i % 1000);
}
// Benchmark non-interned strings
long start = System.nanoTime();
for (int i = 0; i < ITERATIONS; i++) {
strings[i].equals("String" + (i % 1000));
}
long nonInternedTime = System.nanoTime() - start;
// Intern strings
for (int i = 0; i < ITERATIONS; i++) {
strings[i] = strings[i].intern();
}
// Benchmark interned strings
start = System.nanoTime();
for (int i = 0; i < ITERATIONS; i++) {
strings[i] == ("String" + (i % 1000)).intern();
}
long internedTime = System.nanoTime() - start;
System.out.println("Non-interned time: " + nonInternedTime / 1_000_000 + " ms");
System.out.println("Interned time: " + internedTime / 1_000_000 + " ms");
}
}
This benchmark compares the time taken to perform equality checks on interned and non-interned strings. Run this on your system to see the performance difference in your specific environment.
String Interning and Security
While string interning can bring performance benefits, it’s important to consider its security implications, especially in web applications.
Denial of Service (DoS) Attacks
If an attacker can control the strings being interned, they might be able to cause the application to intern a large number of unique strings, potentially exhausting memory. This is particularly relevant when interning user input or data from untrusted sources.
Timing Attacks
In some cases, the time difference between comparing interned and non-interned strings could potentially be used in timing attacks. While this is a relatively obscure attack vector, it’s worth being aware of in high-security contexts.
Future of String Interning
As programming languages and runtime environments evolve, so does the implementation and importance of string interning. Here are some trends and potential future developments:
Automatic Optimization
Future language implementations may include more sophisticated automatic string interning and deduplication strategies, reducing the need for manual intervention.
Integration with Other Optimizations
String interning may become more tightly integrated with other optimizations like escape analysis and JIT compilation, leading to even better performance in hot code paths.
Distributed String Interning
In distributed systems and microservices architectures, we might see the development of distributed string interning mechanisms to optimize cross-service communication.
Conclusion
String interning is a powerful technique that can significantly improve the performance and memory efficiency of applications that work with large amounts of string data. By understanding how it works and when to use it, you can write more efficient code and optimize your applications for better performance.
However, like any optimization technique, it should be used judiciously. Always measure the impact in your specific use case, be aware of the potential drawbacks, and consider the security implications, especially when working with user input or in web applications.
As you continue your journey in software development, keep string interning in your toolbox of optimization techniques. It’s particularly valuable when preparing for technical interviews or when working on performance-critical applications. Remember, understanding low-level optimizations like this can set you apart as a developer and help you create more efficient, scalable software systems.