Understanding Sorting Algorithms for Coding Interviews: A Comprehensive Guide
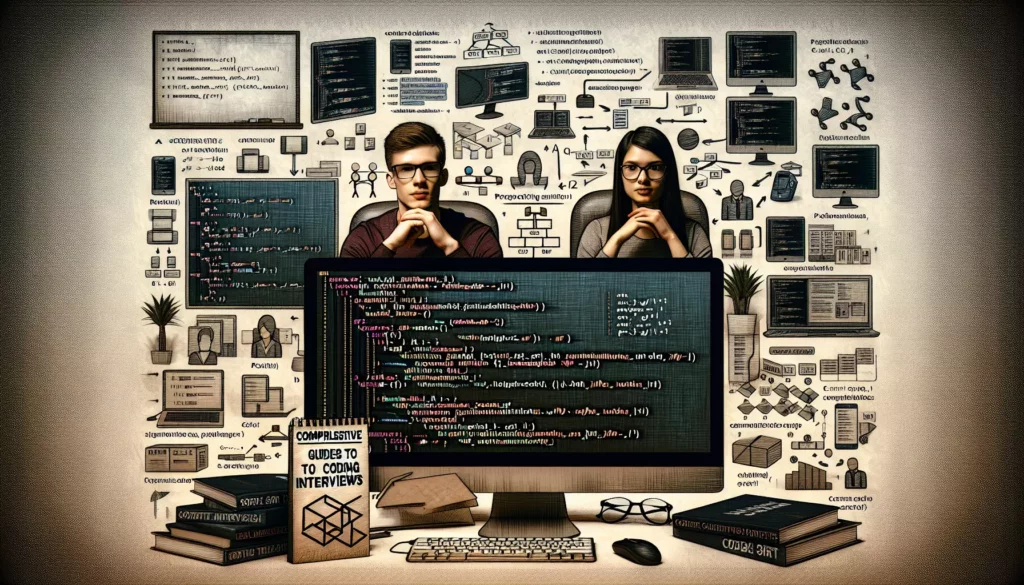
When preparing for coding interviews, particularly for positions at major tech companies like FAANG (Facebook, Amazon, Apple, Netflix, Google), understanding sorting algorithms is crucial. These algorithms form the backbone of many complex computational problems and are often used to assess a candidate’s problem-solving skills and algorithmic thinking. In this comprehensive guide, we’ll dive deep into the world of sorting algorithms, exploring their implementations, time complexities, and practical applications.
Why Sorting Algorithms Matter in Coding Interviews
Sorting algorithms are fundamental to computer science and play a significant role in coding interviews for several reasons:
- Efficiency assessment: Interviewers use sorting problems to evaluate how well candidates can optimize algorithms for time and space complexity.
- Problem-solving skills: Implementing sorting algorithms requires logical thinking and the ability to break down complex problems into manageable steps.
- Algorithm design: Understanding sorting algorithms helps in designing efficient solutions for a wide range of problems beyond just sorting.
- Foundation for other algorithms: Many advanced algorithms and data structures build upon the principles used in sorting algorithms.
Common Sorting Algorithms
Let’s explore some of the most frequently discussed sorting algorithms in coding interviews:
1. Bubble Sort
Bubble Sort is a simple sorting algorithm that repeatedly steps through the list, compares adjacent elements, and swaps them if they’re in the wrong order.
Implementation:
def bubble_sort(arr):
n = len(arr)
for i in range(n):
for j in range(0, n - i - 1):
if arr[j] > arr[j + 1]:
arr[j], arr[j + 1] = arr[j + 1], arr[j]
return arr
Time Complexity:
- Best Case: O(n) – when the array is already sorted
- Average Case: O(n^2)
- Worst Case: O(n^2)
Space Complexity:
O(1) – Bubble Sort is an in-place sorting algorithm
2. Selection Sort
Selection Sort divides the input list into two parts: a sorted portion at the left end and an unsorted portion at the right end. It repeatedly selects the smallest element from the unsorted portion and moves it to the sorted portion.
Implementation:
def selection_sort(arr):
n = len(arr)
for i in range(n):
min_idx = i
for j in range(i + 1, n):
if arr[j] < arr[min_idx]:
min_idx = j
arr[i], arr[min_idx] = arr[min_idx], arr[i]
return arr
Time Complexity:
- Best Case: O(n^2)
- Average Case: O(n^2)
- Worst Case: O(n^2)
Space Complexity:
O(1) – Selection Sort is an in-place sorting algorithm
3. Insertion Sort
Insertion Sort builds the final sorted array one item at a time. It iterates through the input elements, growing the sorted array with each iteration. It is much less efficient on large lists than more advanced algorithms such as QuickSort, HeapSort, or Merge Sort.
Implementation:
def insertion_sort(arr):
for i in range(1, len(arr)):
key = arr[i]
j = i - 1
while j >= 0 and key < arr[j]:
arr[j + 1] = arr[j]
j -= 1
arr[j + 1] = key
return arr
Time Complexity:
- Best Case: O(n) – when the array is already sorted
- Average Case: O(n^2)
- Worst Case: O(n^2)
Space Complexity:
O(1) – Insertion Sort is an in-place sorting algorithm
4. Merge Sort
Merge Sort is an efficient, stable, and comparison-based sorting algorithm. Most implementations produce a stable sort, meaning that the order of equal elements is the same in the input and output. Merge Sort is a divide and conquer algorithm that was invented by John von Neumann in 1945.
Implementation:
def merge_sort(arr):
if len(arr) > 1:
mid = len(arr) // 2
L = arr[:mid]
R = arr[mid:]
merge_sort(L)
merge_sort(R)
i = j = k = 0
while i < len(L) and j < len(R):
if L[i] < R[j]:
arr[k] = L[i]
i += 1
else:
arr[k] = R[j]
j += 1
k += 1
while i < len(L):
arr[k] = L[i]
i += 1
k += 1
while j < len(R):
arr[k] = R[j]
j += 1
k += 1
return arr
Time Complexity:
- Best Case: O(n log n)
- Average Case: O(n log n)
- Worst Case: O(n log n)
Space Complexity:
O(n) – Merge Sort requires additional space for merging
5. QuickSort
QuickSort is a highly efficient sorting algorithm and is based on partitioning of array of data into smaller arrays. A large array is partitioned into two arrays one of which holds values smaller than the specified value, say pivot, based on which the partition is made and another array holds values greater than the pivot value.
Implementation:
def partition(arr, low, high):
i = (low - 1)
pivot = arr[high]
for j in range(low, high):
if arr[j] <= pivot:
i = i + 1
arr[i], arr[j] = arr[j], arr[i]
arr[i + 1], arr[high] = arr[high], arr[i + 1]
return (i + 1)
def quick_sort(arr, low, high):
if low < high:
pi = partition(arr, low, high)
quick_sort(arr, low, pi - 1)
quick_sort(arr, pi + 1, high)
return arr
# Usage
arr = [10, 7, 8, 9, 1, 5]
n = len(arr)
sorted_arr = quick_sort(arr, 0, n - 1)
Time Complexity:
- Best Case: O(n log n)
- Average Case: O(n log n)
- Worst Case: O(n^2) – rare, happens when the pivot is always the smallest or largest element
Space Complexity:
O(log n) – QuickSort is an in-place sorting algorithm, but it requires space on the call stack for recursion
Comparing Sorting Algorithms
When preparing for coding interviews, it’s essential to understand the strengths and weaknesses of each sorting algorithm. Here’s a quick comparison:
Algorithm | Time Complexity (Best) | Time Complexity (Average) | Time Complexity (Worst) | Space Complexity | Stability |
---|---|---|---|---|---|
Bubble Sort | O(n) | O(n^2) | O(n^2) | O(1) | Stable |
Selection Sort | O(n^2) | O(n^2) | O(n^2) | O(1) | Not Stable |
Insertion Sort | O(n) | O(n^2) | O(n^2) | O(1) | Stable |
Merge Sort | O(n log n) | O(n log n) | O(n log n) | O(n) | Stable |
QuickSort | O(n log n) | O(n log n) | O(n^2) | O(log n) | Not Stable |
Advanced Sorting Algorithms
While the algorithms mentioned above are commonly discussed in coding interviews, it’s beneficial to be aware of some advanced sorting algorithms:
1. HeapSort
HeapSort is a comparison-based sorting algorithm that uses a binary heap data structure. It’s similar to Selection Sort where we first find the minimum element and place it at the beginning. We repeat the same process for the remaining elements.
Key Points:
- Time Complexity: O(n log n) for all cases
- Space Complexity: O(1)
- Not stable, but in-place
2. Counting Sort
Counting Sort is an integer sorting algorithm that operates by counting the number of objects that possess distinct key values, and applying prefix sum on those counts to determine the positions of each key value in the output sequence.
Key Points:
- Time Complexity: O(n + k) where n is the number of elements and k is the range of input
- Space Complexity: O(n + k)
- Stable and efficient for small ranges of input
3. Radix Sort
Radix Sort is a non-comparative sorting algorithm that sorts data with integer keys by grouping the keys by individual digits that share the same significant position and value.
Key Points:
- Time Complexity: O(d * (n + k)) where d is the number of digits, n is the number of elements, and k is the range of input
- Space Complexity: O(n + k)
- Stable and efficient for fixed-length integer keys
Practical Applications of Sorting Algorithms
Understanding sorting algorithms isn’t just about passing coding interviews. These algorithms have numerous practical applications in real-world software development:
- Database Management Systems: Sorting is crucial for efficient data retrieval and indexing in databases.
- Search Algorithms: Many search algorithms require sorted data to function efficiently.
- Data Analysis: Sorting is often a preprocessing step in data analysis and visualization tasks.
- Computer Graphics: Sorting is used in rendering pipelines for determining the order of drawing objects.
- Operating Systems: Process scheduling and memory management often involve sorting algorithms.
- Network Routing: Sorting is used in routing algorithms to determine the best path for data packets.
Tips for Mastering Sorting Algorithms in Coding Interviews
To excel in coding interviews when it comes to sorting algorithms, consider the following tips:
- Understand the basics: Make sure you have a solid grasp of the fundamental sorting algorithms like Bubble Sort, Insertion Sort, and Selection Sort.
- Focus on efficient algorithms: Pay special attention to Merge Sort and QuickSort, as they are often the most efficient and commonly used in practice.
- Analyze time and space complexity: Be prepared to discuss the time and space complexity of different sorting algorithms in various scenarios.
- Practice implementation: Implement sorting algorithms from scratch to ensure you understand their inner workings.
- Consider edge cases: Think about how different algorithms perform with already sorted arrays, reverse sorted arrays, or arrays with many duplicate elements.
- Know when to use built-in functions: In real-world scenarios, it’s often best to use language-provided sorting functions. Be prepared to explain when and why you would choose a built-in function over implementing your own algorithm.
- Understand stability: Know which algorithms are stable and why stability matters in certain applications.
- Practice problem variations: Solve problems that involve sorting as part of a larger algorithm or data structure.
Common Interview Questions Related to Sorting Algorithms
To help you prepare, here are some common interview questions related to sorting algorithms:
- Implement QuickSort and explain its average-case time complexity.
- How would you sort a large file with limited RAM?
- Explain the difference between a stable and unstable sorting algorithm. When does stability matter?
- How would you modify Merge Sort to count the number of inversions in an array?
- Implement a sorting algorithm that works in O(n) time complexity.
- How would you sort an array of 0s, 1s, and 2s in linear time?
- Explain how you would implement an external sort.
- How would you sort a linked list using Merge Sort?
- Implement a hybrid sorting algorithm that combines Insertion Sort and Merge Sort.
- How would you sort a nearly sorted array efficiently?
Conclusion
Mastering sorting algorithms is a crucial step in preparing for coding interviews, especially for positions at major tech companies. By understanding the mechanics, time and space complexities, and practical applications of various sorting algorithms, you’ll be well-equipped to tackle a wide range of interview questions and real-world programming challenges.
Remember, the key to success in coding interviews is not just memorizing algorithms, but understanding their underlying principles and being able to apply them to solve diverse problems. Practice implementing these algorithms, analyze their performance characteristics, and work on problems that require sorting as part of their solution.
As you continue your journey in mastering algorithms and data structures, don’t forget to explore other important topics such as searching algorithms, graph algorithms, and dynamic programming. With dedication and consistent practice, you’ll be well on your way to acing your coding interviews and building a strong foundation for your software engineering career.