Understanding Scope: Local vs. Global Variables in Programming
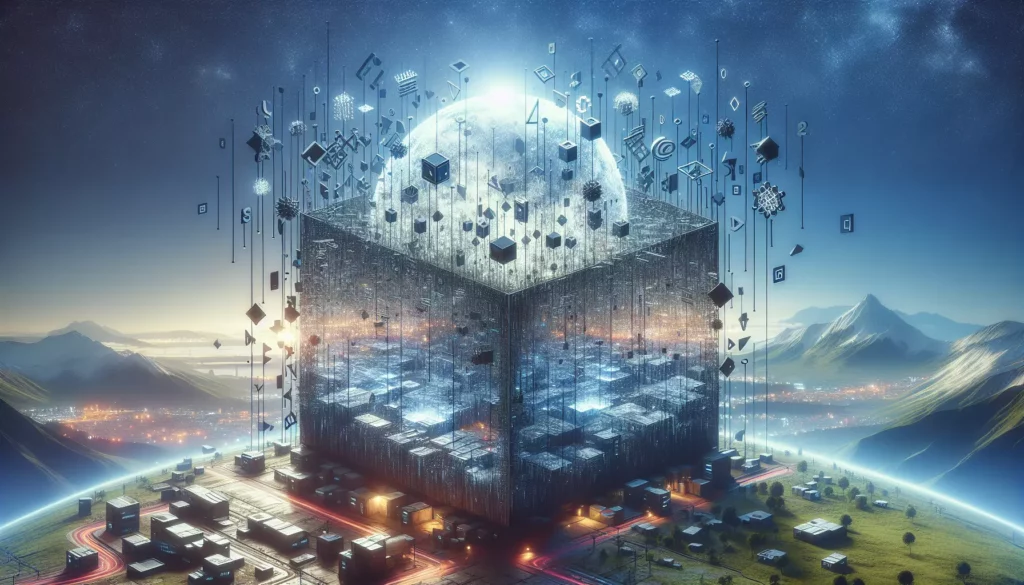
When diving into the world of programming, one of the fundamental concepts you’ll encounter is the idea of scope. Scope refers to the visibility and accessibility of variables within different parts of your code. Two primary types of scope you’ll work with are local and global scope, which determine where variables can be accessed and modified. Understanding the difference between local and global variables is crucial for writing clean, efficient, and bug-free code.
In this comprehensive guide, we’ll explore the concepts of local and global variables, their characteristics, use cases, and best practices. We’ll also dive into some advanced topics related to scope and provide practical examples to solidify your understanding. By the end of this article, you’ll have a strong grasp of how to effectively use local and global variables in your programming projects.
Table of Contents
- What is Scope?
- Local Variables
- Global Variables
- Key Differences Between Local and Global Variables
- Best Practices for Using Local and Global Variables
- Advanced Topics in Scope
- Practical Examples
- Common Pitfalls and How to Avoid Them
- Conclusion
1. What is Scope?
Before we dive into the specifics of local and global variables, let’s first understand what scope means in programming. Scope refers to the region of a program where a variable is visible and can be accessed. It determines where you can use a variable and where it’s not available.
The concept of scope is crucial because it helps in:
- Organizing and structuring code
- Preventing naming conflicts
- Controlling access to variables
- Managing memory efficiently
Different programming languages may have slightly different rules for scope, but the general principles remain similar. In this article, we’ll focus on the most common scoping rules found in popular languages like Python, JavaScript, Java, and C++.
2. Local Variables
Local variables are variables that are defined within a specific function or block of code. They have a limited scope and can only be accessed within the function or block where they are declared. Once the function or block finishes executing, the local variables are destroyed, and their memory is freed up.
Characteristics of Local Variables:
- Limited Visibility: Local variables are only visible and accessible within the function or block where they are defined.
- Lifetime: They exist only for the duration of the function or block execution.
- Memory Efficiency: Local variables are created when the function is called and destroyed when it returns, making them memory-efficient.
- Name Reusability: You can use the same variable name in different functions without conflict, as each instance is separate and local to its function.
Example of Local Variables:
Here’s a simple example in Python to illustrate local variables:
def greet(name):
message = f"Hello, {name}!" # Local variable
print(message)
greet("Alice")
# print(message) # This would raise an error because 'message' is not accessible here
In this example, message
is a local variable within the greet
function. It can’t be accessed outside the function.
3. Global Variables
Global variables are variables that are defined outside of any function or class and have a global scope. This means they can be accessed from any part of the program, including within functions and classes.
Characteristics of Global Variables:
- Wide Visibility: Global variables can be accessed from any part of the program.
- Lifetime: They exist for the entire duration of the program execution.
- Shared State: Global variables can be used to share data between different functions and modules.
- Potential for Conflicts: Since they can be accessed and modified from anywhere, there’s a higher risk of naming conflicts and unintended modifications.
Example of Global Variables:
Here’s an example in Python demonstrating global variables:
count = 0 # Global variable
def increment():
global count
count += 1
print(f"Count is now: {count}")
increment()
increment()
print(f"Final count: {count}")
In this example, count
is a global variable that can be accessed and modified within the increment
function using the global
keyword.
4. Key Differences Between Local and Global Variables
Understanding the differences between local and global variables is crucial for writing efficient and maintainable code. Here are the key distinctions:
Aspect | Local Variables | Global Variables |
---|---|---|
Scope | Limited to the function or block where defined | Accessible throughout the entire program |
Lifetime | Exist only during function execution | Exist for the entire program runtime |
Memory Usage | More efficient, memory allocated only when needed | Less efficient, occupy memory for the entire program duration |
Naming Conflicts | Less likely, as names are isolated to their function | More likely, as names are shared across the program |
Code Organization | Promote modular and encapsulated code | Can lead to interdependencies and harder-to-maintain code |
Testing and Debugging | Easier to test and debug, as effects are localized | Can be harder to track changes and side effects |
5. Best Practices for Using Local and Global Variables
While both local and global variables have their place in programming, it’s important to use them judiciously. Here are some best practices to follow:
For Local Variables:
- Prefer Local Variables: Use local variables whenever possible. They make your code more modular and easier to understand.
- Descriptive Names: Use clear, descriptive names for your variables to enhance code readability.
- Minimize Scope: Keep the scope of variables as small as possible. Declare them close to where they’re used.
- Avoid Shadowing: Be cautious not to use the same name for local variables as global ones to prevent confusion.
For Global Variables:
- Use Sparingly: Limit the use of global variables. They should be used only when absolutely necessary.
- Constant Values: Use global variables for constant values that don’t change during program execution.
- Clear Naming: Use clear, distinctive names for global variables to avoid confusion with local variables.
- Documentation: Clearly document the purpose and usage of global variables in your code.
- Consider Alternatives: Before using a global variable, consider if there’s a better way to achieve the same result, such as passing parameters or using object-oriented design.
6. Advanced Topics in Scope
As you delve deeper into programming, you’ll encounter more advanced concepts related to scope. Let’s explore some of these topics:
6.1 Lexical Scope
Lexical scope, also known as static scope, is a scope resolution mechanism where the scope of a variable is determined by its location in the source code. This means that an inner function has access to variables in its outer (enclosing) function.
Example in JavaScript:
function outerFunction() {
let outerVar = "I'm from outer function";
function innerFunction() {
console.log(outerVar); // Can access outerVar
}
innerFunction();
}
outerFunction();
6.2 Closure
A closure is a function that has access to variables in its lexical scope, even when the function is executed outside that scope. Closures are powerful for creating private variables and maintaining state.
Example in JavaScript:
function createCounter() {
let count = 0;
return function() {
count++;
return count;
}
}
const counter = createCounter();
console.log(counter()); // 1
console.log(counter()); // 2
6.3 Block Scope
Block scope refers to variables that are only accessible within a specific block of code, typically denoted by curly braces {}. Languages like JavaScript (with let
and const
) and C++ support block scope.
Example in JavaScript:
if (true) {
let blockVar = "I'm block-scoped";
console.log(blockVar); // Works
}
// console.log(blockVar); // Would throw an error
6.4 Function Scope
Function scope means that variables declared within a function are only accessible within that function and any nested functions.
Example in JavaScript:
function exampleFunction() {
var functionScopedVar = "I'm function-scoped";
console.log(functionScopedVar); // Works
}
// console.log(functionScopedVar); // Would throw an error
6.5 Module Scope
In languages that support modules (like Python or JavaScript with ES6 modules), variables can have module scope, meaning they’re only accessible within the module they’re defined in.
Example in Python:
# module.py
module_var = "I'm module-scoped"
def module_function():
print(module_var)
# main.py
import module
module.module_function() # Works
# print(module.module_var) # Would raise an AttributeError
7. Practical Examples
Let’s look at some practical examples to solidify our understanding of local and global variables in different programming languages.
7.1 Python Example
global_var = "I'm global"
def example_function():
local_var = "I'm local"
print(global_var) # Accessing global variable
print(local_var) # Accessing local variable
def nested_function():
print(global_var) # Can access global
print(local_var) # Can access outer function's local variable
nested_function()
example_function()
print(global_var) # Works
# print(local_var) # Would raise a NameError
7.2 JavaScript Example
let globalVar = "I'm global";
function exampleFunction() {
let localVar = "I'm local";
console.log(globalVar); // Accessing global variable
console.log(localVar); // Accessing local variable
if (true) {
let blockVar = "I'm block-scoped";
console.log(blockVar); // Works
}
// console.log(blockVar); // Would throw a ReferenceError
}
exampleFunction();
console.log(globalVar); // Works
// console.log(localVar); // Would throw a ReferenceError
7.3 Java Example
public class ScopeExample {
static String globalVar = "I'm global";
public static void main(String[] args) {
System.out.println(globalVar); // Accessing global variable
String localVar = "I'm local";
System.out.println(localVar); // Accessing local variable
{
String blockVar = "I'm block-scoped";
System.out.println(blockVar); // Works
}
// System.out.println(blockVar); // Would not compile
}
}
8. Common Pitfalls and How to Avoid Them
When working with local and global variables, there are several common pitfalls that programmers often encounter. Being aware of these issues can help you write more robust and maintainable code.
8.1 Unintended Global Variables
Pitfall: In some languages like JavaScript, forgetting to declare a variable with var
, let
, or const
inside a function can accidentally create a global variable.
Solution: Always use proper variable declarations and enable strict mode in JavaScript to catch these errors.
"use strict";
function example() {
localVar = "Oops, I'm accidentally global!"; // This will throw an error in strict mode
}
// Instead, use:
function correctExample() {
let localVar = "I'm properly local";
}
8.2 Shadowing
Pitfall: Shadowing occurs when a local variable has the same name as a global variable, potentially leading to confusion and bugs.
Solution: Use distinct names for local and global variables. If you need to access a global variable within a function that has a local variable with the same name, use language-specific techniques (like the global
keyword in Python).
x = 10 # Global variable
def example():
x = 20 # Local variable shadowing the global x
print(x) # Prints 20
example()
print(x) # Prints 10
8.3 Overuse of Global Variables
Pitfall: Excessive use of global variables can make code harder to understand, test, and maintain.
Solution: Minimize the use of global variables. Instead, pass necessary data as parameters to functions or use object-oriented design principles.
// Instead of:
let globalCounter = 0;
function incrementCounter() {
globalCounter++;
}
// Prefer:
function createCounter() {
let count = 0;
return function() {
count++;
return count;
};
}
const counter = createCounter();
console.log(counter()); // 1
console.log(counter()); // 2
8.4 Modifying Global Variables in Functions
Pitfall: Modifying global variables within functions can lead to unexpected side effects and make code harder to debug.
Solution: If you must modify a global variable, clearly document this behavior. Consider using function parameters and return values instead.
// Instead of:
let total = 0;
function addToTotal(value) {
total += value;
}
// Prefer:
function addToTotal(currentTotal, value) {
return currentTotal + value;
}
let total = 0;
total = addToTotal(total, 5);
total = addToTotal(total, 10);
8.5 Circular Dependencies
Pitfall: When using global variables across multiple modules, circular dependencies can occur, leading to complex and hard-to-maintain code.
Solution: Restructure your code to avoid circular dependencies. Use dependency injection or a centralized configuration module if necessary.
9. Conclusion
Understanding the concept of scope and the differences between local and global variables is fundamental to writing clean, efficient, and maintainable code. Local variables provide encapsulation and memory efficiency, while global variables offer a way to share data across different parts of your program. However, the use of global variables should be limited and carefully considered due to their potential drawbacks.
As you continue your journey in programming, remember these key points:
- Prefer local variables whenever possible to keep your code modular and easier to understand.
- Use global variables sparingly and only when necessary.
- Be aware of the scope rules in the programming language you’re using.
- Practice good naming conventions to avoid confusion between local and global variables.
- Understand advanced concepts like lexical scope, closures, and block scope to write more sophisticated code.
By mastering the use of local and global variables, you’ll be better equipped to tackle complex programming challenges and write code that is both efficient and maintainable. As you apply these concepts in your projects, you’ll develop a deeper appreciation for the importance of scope in programming and how it contributes to the overall quality of your code.
Remember, the journey to becoming a proficient programmer is ongoing, and understanding scope is just one step along the way. Continue to practice, explore, and challenge yourself with new programming concepts and techniques. Happy coding!