Understanding Recursion vs. Iteration Trade-offs: A Comprehensive Guide
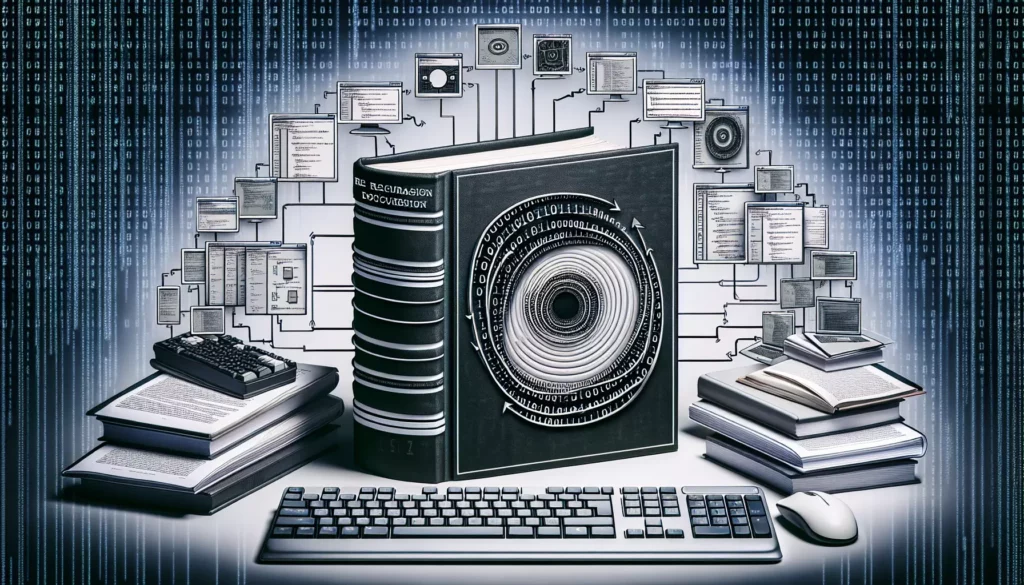
When it comes to solving problems in computer science and programming, two fundamental approaches often come into play: recursion and iteration. Both methods can be used to tackle similar problems, but they differ significantly in their implementation and performance characteristics. In this comprehensive guide, we’ll dive deep into the world of recursion and iteration, exploring their strengths, weaknesses, and the trade-offs involved in choosing between them.
What is Recursion?
Recursion is a problem-solving technique where a function calls itself to solve a smaller instance of the same problem. It’s based on the principle of breaking down a complex problem into smaller, more manageable subproblems. The recursive approach continues until it reaches a base case, which is a simple enough scenario that can be solved directly without further recursion.
Key Characteristics of Recursion:
- Self-referential: The function calls itself.
- Base case: A condition that stops the recursion.
- Recursive case: The part where the function calls itself with a modified input.
- Stack-based: Utilizes the call stack to manage function calls.
Example of a Recursive Function:
Let’s look at a classic example of recursion: calculating the factorial of a number.
def factorial(n):
if n == 0 or n == 1: # Base case
return 1
else: # Recursive case
return n * factorial(n - 1)
print(factorial(5)) # Output: 120
What is Iteration?
Iteration, on the other hand, is a problem-solving approach that uses loops to repeat a set of instructions until a specific condition is met. It’s a more straightforward and often more intuitive way to solve problems, especially for those new to programming.
Key Characteristics of Iteration:
- Loop-based: Uses constructs like for or while loops.
- State variables: Maintains state through variables that change with each iteration.
- Termination condition: A condition that ends the loop.
- Sequential execution: Processes instructions in a linear fashion.
Example of an Iterative Function:
Here’s the same factorial calculation implemented iteratively:
def factorial_iterative(n):
result = 1
for i in range(1, n + 1):
result *= i
return result
print(factorial_iterative(5)) # Output: 120
Trade-offs Between Recursion and Iteration
Now that we’ve covered the basics of both approaches, let’s dive into the trade-offs between recursion and iteration. Understanding these trade-offs is crucial for making informed decisions about which approach to use in different scenarios.
1. Memory Usage
Recursion: Each recursive call adds a new frame to the call stack, which can lead to high memory usage for deeply nested recursions. This can potentially result in a stack overflow for very large inputs.
Iteration: Generally uses less memory as it doesn’t rely on the call stack for each repetition. It maintains state using variables, which typically consume less memory than stack frames.
Trade-off: Iteration is often more memory-efficient, especially for large inputs or deep recursions. However, some algorithms (like tree traversals) may be more naturally expressed using recursion, potentially leading to cleaner, more maintainable code at the cost of higher memory usage.
2. Performance
Recursion: Can be slower due to the overhead of function calls and stack management. However, some compilers can optimize tail-recursive functions to be as efficient as loops.
Iteration: Generally faster as it avoids the overhead of function calls and stack operations. It’s more predictable in terms of performance.
Trade-off: Iteration often performs better, especially for simple problems. However, for certain complex problems (like traversing tree-like data structures), recursion might lead to more elegant and potentially faster solutions.
3. Code Readability and Maintainability
Recursion: Can lead to more concise and elegant code for problems that have a naturally recursive structure (e.g., tree traversals, divide-and-conquer algorithms).
Iteration: Often results in more straightforward, easy-to-understand code for simpler problems. It can be more intuitive for programmers, especially beginners.
Trade-off: The choice between recursion and iteration can significantly impact code readability and maintainability. Recursive solutions might be more elegant for certain problems, while iterative solutions might be clearer for others. The best choice often depends on the specific problem and the team’s familiarity with both approaches.
4. Stack Overflow Risk
Recursion: Prone to stack overflow errors for large inputs or deep recursions, as each recursive call adds to the call stack.
Iteration: Generally not susceptible to stack overflow errors, as it doesn’t rely on the call stack for repetition.
Trade-off: Iteration is safer when dealing with large inputs or when the depth of recursion is unpredictable. However, some languages and compilers support tail-call optimization, which can mitigate this risk for certain types of recursive functions.
5. Problem Suitability
Recursion: Well-suited for problems with a recursive nature, such as tree traversals, graph algorithms, and divide-and-conquer strategies.
Iteration: Better for linear processes, simple repetitive tasks, and problems where state needs to be explicitly managed.
Trade-off: The nature of the problem often dictates which approach is more suitable. Choosing the wrong approach can lead to unnecessarily complex or inefficient solutions.
When to Use Recursion
Despite some of its drawbacks, recursion can be the better choice in certain scenarios:
- Tree-like Data Structures: For traversing or manipulating trees, graphs, or nested data structures, recursion often leads to more intuitive and cleaner code.
- Divide-and-Conquer Algorithms: Problems that can be broken down into smaller, similar subproblems (like quicksort or merge sort) are naturally suited to recursive solutions.
- Backtracking Algorithms: For problems involving exhaustive searches with backtracking (e.g., solving Sudoku), recursion can provide a more elegant solution.
- Mathematical Definitions: When the problem is defined recursively (like factorial or Fibonacci sequences), a recursive implementation can closely mirror the mathematical definition.
- Simplicity and Elegance: In cases where a recursive solution is significantly more concise and easier to understand than an iterative one.
Example: Tree Traversal
Here’s an example of in-order traversal of a binary tree using recursion:
class TreeNode:
def __init__(self, val=0, left=None, right=None):
self.val = val
self.left = left
self.right = right
def inorder_traversal(root):
if root:
inorder_traversal(root.left)
print(root.val, end=' ')
inorder_traversal(root.right)
# Example usage
root = TreeNode(1)
root.left = TreeNode(2)
root.right = TreeNode(3)
root.left.left = TreeNode(4)
root.left.right = TreeNode(5)
inorder_traversal(root) # Output: 4 2 5 1 3
When to Use Iteration
Iteration is often the preferred choice in many scenarios:
- Simple Repetitive Tasks: For straightforward loops or repetitions, iteration is usually more intuitive and efficient.
- Large Inputs: When dealing with large datasets or deep recursions where stack overflow is a concern, iteration is safer.
- Performance-Critical Code: In scenarios where every bit of performance matters, iteration often has the edge due to less overhead.
- Explicit State Management: When you need fine-grained control over the state at each step of the process.
- Beginner-Friendly Code: For educational purposes or when working with less experienced developers, iterative solutions are often easier to understand and debug.
Example: Finding the Maximum Element in an Array
Here’s an iterative approach to finding the maximum element in an array:
def find_max(arr):
if not arr:
return None
max_val = arr[0]
for num in arr[1:]:
if num > max_val:
max_val = num
return max_val
# Example usage
numbers = [3, 7, 2, 9, 1, 5]
print(find_max(numbers)) # Output: 9
Hybrid Approaches: Combining Recursion and Iteration
In some cases, a hybrid approach that combines both recursion and iteration can provide the best of both worlds. This approach can leverage the elegance of recursion while mitigating some of its drawbacks.
Example: Iterative Deepening Depth-First Search
Iterative Deepening Depth-First Search (IDDFS) is a graph traversal algorithm that combines the space efficiency of depth-first search with the completeness of breadth-first search. It uses iteration to control the depth of recursion:
def iddfs(graph, start, goal, max_depth):
for depth in range(max_depth):
if dfs(graph, start, goal, depth):
return True
return False
def dfs(graph, node, goal, depth):
if depth == 0 and node == goal:
return True
if depth > 0:
for neighbor in graph[node]:
if dfs(graph, neighbor, goal, depth - 1):
return True
return False
# Example usage
graph = {
'A': ['B', 'C'],
'B': ['D', 'E'],
'C': ['F'],
'D': [],
'E': ['F'],
'F': []
}
print(iddfs(graph, 'A', 'F', 3)) # Output: True
In this example, the outer function iddfs
uses iteration to control the maximum depth, while the inner function dfs
uses recursion to perform the actual depth-first search.
Performance Optimization Techniques
When working with recursion and iteration, there are several optimization techniques that can help improve performance:
1. Tail Recursion Optimization
Tail recursion is a special case of recursion where the recursive call is the last operation in the function. Some compilers can optimize tail-recursive functions to be as efficient as loops:
def factorial_tail(n, acc=1):
if n == 0:
return acc
return factorial_tail(n - 1, n * acc)
print(factorial_tail(5)) # Output: 120
2. Memoization
Memoization is a technique used to store the results of expensive function calls and return the cached result when the same inputs occur again. It can significantly improve the performance of recursive functions:
def fibonacci(n, memo={}):
if n in memo:
return memo[n]
if n <= 1:
return n
result = fibonacci(n-1, memo) + fibonacci(n-2, memo)
memo[n] = result
return result
print(fibonacci(100)) # Output: 354224848179261915075
3. Generator Functions
Generator functions can be used to create iterators, which can be more memory-efficient than recursion for certain problems:
def fibonacci_generator():
a, b = 0, 1
while True:
yield a
a, b = b, a + b
fib = fibonacci_generator()
for _ in range(10):
print(next(fib), end=' ')
# Output: 0 1 1 2 3 5 8 13 21 34
Conclusion
The choice between recursion and iteration is not always clear-cut. It depends on various factors including the nature of the problem, performance requirements, code readability, and the specific constraints of your project. Here are some key takeaways:
- Recursion often leads to more elegant solutions for problems with a naturally recursive structure, but it can be less efficient in terms of memory usage and performance for large inputs.
- Iteration is generally more efficient and safer in terms of memory usage, but it may result in more complex code for certain types of problems.
- Consider using recursion for tree-like structures, divide-and-conquer algorithms, and problems with a clear recursive definition.
- Opt for iteration when dealing with simple repetitive tasks, large inputs, or when performance is critical.
- Hybrid approaches can sometimes offer the best of both worlds, combining the elegance of recursion with the efficiency of iteration.
- Always consider the specific requirements of your project, including performance needs, code maintainability, and the expertise of your team when making the choice.
By understanding the trade-offs between recursion and iteration, you can make informed decisions that lead to more efficient, readable, and maintainable code. Remember, the best approach often depends on the specific problem at hand, so always be ready to analyze and choose the most appropriate solution for each unique situation.