Understanding Real-Time Web with WebSockets: Building Live Applications
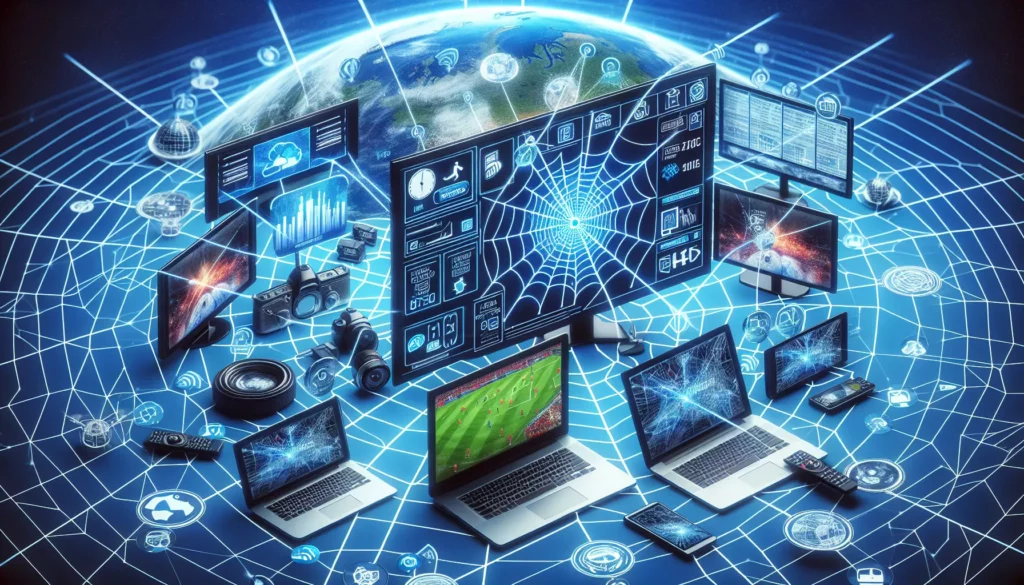
In today’s fast-paced digital world, users expect instant updates and real-time interactions from web applications. Whether it’s a chat application, live sports scores, or collaborative editing tools, the demand for real-time functionality has never been higher. This is where WebSockets come into play, revolutionizing the way we build and interact with web applications. In this comprehensive guide, we’ll dive deep into the world of WebSockets, exploring how they work, their benefits, and how to implement them in your projects.
What are WebSockets?
WebSockets are a protocol that enables full-duplex, bidirectional communication between a client (typically a web browser) and a server over a single TCP connection. Unlike traditional HTTP requests, which follow a request-response model, WebSockets allow for continuous, real-time data exchange without the need for polling or long-polling techniques.
The WebSocket protocol was standardized by the IETF as RFC 6455 in 2011, and it has since become a crucial technology for building real-time web applications. WebSockets provide a persistent connection that remains open, allowing both the client and server to send messages to each other at any time.
How do WebSockets Work?
The WebSocket connection lifecycle consists of several stages:
- Handshake: The client initiates a WebSocket connection by sending an HTTP upgrade request to the server.
- Upgrade: If the server supports WebSockets, it responds with an HTTP 101 status code, indicating that the protocol is switching from HTTP to WebSocket.
- Data Transfer: Once the connection is established, both the client and server can send messages to each other at any time.
- Closing: Either the client or server can initiate the closing of the WebSocket connection.
Here’s a simple example of how a WebSocket connection is established in JavaScript:
const socket = new WebSocket('ws://example.com/socketserver');
socket.onopen = function(event) {
console.log('WebSocket connection established');
};
socket.onmessage = function(event) {
console.log('Received message:', event.data);
};
socket.onclose = function(event) {
console.log('WebSocket connection closed');
};
// Sending a message
socket.send('Hello, WebSocket!');
Benefits of Using WebSockets
WebSockets offer several advantages over traditional HTTP communication:
- Real-time Updates: WebSockets enable instant data transmission, making them ideal for applications that require live updates.
- Reduced Latency: Since the connection remains open, there’s no need for repeated handshakes, resulting in lower latency compared to polling techniques.
- Bi-directional Communication: Both the client and server can initiate communication, allowing for more dynamic interactions.
- Efficiency: WebSockets reduce overhead by eliminating the need for multiple HTTP requests, leading to better performance and lower server load.
- Scalability: WebSockets can handle a large number of concurrent connections more efficiently than traditional HTTP.
Use Cases for WebSockets
WebSockets are particularly useful in scenarios where real-time updates and low-latency communication are crucial. Some common use cases include:
- Chat Applications: Instant messaging and group chat features benefit greatly from the real-time nature of WebSockets.
- Live Feeds: Social media feeds, news tickers, and sports score updates can provide instant information to users.
- Collaborative Tools: Real-time document editing, whiteboarding, and project management tools can leverage WebSockets for seamless collaboration.
- Gaming: Multiplayer online games can use WebSockets for real-time player interactions and game state updates.
- Financial Applications: Stock tickers and trading platforms require up-to-the-second data, making WebSockets an ideal choice.
- IoT (Internet of Things): WebSockets can facilitate real-time communication between connected devices and central servers.
Implementing WebSockets in Your Projects
Now that we understand the basics of WebSockets, let’s explore how to implement them in your projects. We’ll look at both client-side and server-side implementations using popular technologies.
Client-Side Implementation (JavaScript)
Modern web browsers provide native support for WebSockets through the WebSocket API. Here’s a more detailed example of how to use WebSockets in a client-side JavaScript application:
// Establish a WebSocket connection
const socket = new WebSocket('ws://example.com/socketserver');
// Connection opened
socket.addEventListener('open', (event) => {
console.log('WebSocket connection established');
socket.send('Hello, Server!');
});
// Listen for messages
socket.addEventListener('message', (event) => {
console.log('Message from server:', event.data);
});
// Connection closed
socket.addEventListener('close', (event) => {
console.log('WebSocket connection closed');
});
// Error handling
socket.addEventListener('error', (event) => {
console.error('WebSocket error:', event);
});
// Function to send messages
function sendMessage(message) {
if (socket.readyState === WebSocket.OPEN) {
socket.send(message);
} else {
console.error('WebSocket is not open. Unable to send message.');
}
}
This example demonstrates how to establish a WebSocket connection, handle various events (open, message, close, and error), and send messages to the server.
Server-Side Implementation (Node.js)
On the server side, you’ll need to implement a WebSocket server to handle incoming connections and messages. Let’s look at an example using Node.js and the popular `ws` library:
First, install the `ws` package:
npm install ws
Now, create a simple WebSocket server:
const WebSocket = require('ws');
const wss = new WebSocket.Server({ port: 8080 });
wss.on('connection', (ws) => {
console.log('New client connected');
ws.on('message', (message) => {
console.log('Received message:', message);
// Echo the message back to the client
ws.send(`Server received: ${message}`);
});
ws.on('close', () => {
console.log('Client disconnected');
});
// Send a welcome message
ws.send('Welcome to the WebSocket server!');
});
console.log('WebSocket server is running on ws://localhost:8080');
This server listens for WebSocket connections on port 8080, handles incoming messages, and sends responses back to the clients.
Best Practices for Working with WebSockets
When implementing WebSockets in your applications, consider the following best practices:
- Error Handling: Implement robust error handling on both the client and server sides to gracefully manage connection issues and unexpected errors.
- Reconnection Logic: Implement automatic reconnection logic on the client side to handle temporary disconnections or network issues.
- Message Validation: Validate incoming messages on the server to ensure they conform to your expected format and contain valid data.
- Security: Use WSS (WebSocket Secure) for encrypted connections, especially when dealing with sensitive data.
- Rate Limiting: Implement rate limiting on the server to prevent abuse and ensure fair usage of resources.
- Scalability: Design your WebSocket architecture to handle a large number of concurrent connections, possibly using load balancers and multiple server instances.
- Heartbeats: Implement heartbeat mechanisms to detect and close stale connections, freeing up server resources.
- Fallback Mechanisms: Provide fallback options (e.g., long-polling) for clients that don’t support WebSockets or in environments where WebSockets are blocked.
WebSocket Libraries and Frameworks
While the native WebSocket API is powerful, there are several libraries and frameworks that can simplify WebSocket implementation and provide additional features. Here are some popular options:
Client-Side Libraries
- Socket.IO: A widely-used library that provides real-time, bidirectional event-based communication. It automatically falls back to other techniques when WebSockets are not available.
- SockJS: A JavaScript library that provides a WebSocket-like object. It offers various fallback options for browsers that don’t support WebSockets.
- Pusher: A hosted solution that provides real-time functionality through WebSockets, with client libraries for various platforms.
Server-Side Libraries
- ws: A simple and fast WebSocket client and server implementation for Node.js.
- Socket.IO: The server-side component of the Socket.IO library, which can be used with Node.js.
- WebSocket-Node: A WebSocket client and server implementation for Node.js, fully compatible with the WebSocket API in web browsers.
Advanced WebSocket Concepts
As you become more comfortable with WebSockets, you may want to explore some advanced concepts and techniques:
1. WebSocket Subprotocols
WebSocket subprotocols allow you to define custom protocols on top of the WebSocket connection. This can be useful for implementing specific messaging formats or behaviors. Here’s an example of how to use subprotocols:
// Client-side
const socket = new WebSocket('ws://example.com/socketserver', ['json', 'xml']);
// Server-side (Node.js with 'ws' library)
const WebSocket = require('ws');
const wss = new WebSocket.Server({ port: 8080 });
wss.on('connection', (ws, req) => {
const protocol = req.headers['sec-websocket-protocol'];
if (protocol === 'json') {
ws.protocol = 'json';
// Handle JSON messages
} else if (protocol === 'xml') {
ws.protocol = 'xml';
// Handle XML messages
}
});
2. Binary Data Transfer
WebSockets support sending binary data in addition to text. This can be useful for transferring files, images, or other non-text data efficiently. Here’s how you can send and receive binary data:
// Sending binary data (client-side)
const buffer = new ArrayBuffer(4);
const view = new Int32Array(buffer);
view[0] = 42;
socket.send(buffer);
// Receiving binary data (client-side)
socket.onmessage = function(event) {
if (event.data instanceof ArrayBuffer) {
const view = new Int32Array(event.data);
console.log('Received binary data:', view[0]);
}
};
// Sending binary data (server-side, Node.js with 'ws')
const binaryData = Buffer.from([1, 2, 3, 4]);
ws.send(binaryData);
3. WebSocket Compression
WebSocket compression (also known as permessage-deflate) can significantly reduce the amount of data transferred over the wire, especially for text-based messages. Many WebSocket libraries support compression out of the box. Here’s an example of enabling compression with the ‘ws’ library:
const WebSocket = require('ws');
const wss = new WebSocket.Server({
port: 8080,
perMessageDeflate: {
zlibDeflateOptions: {
// See zlib defaults.
chunkSize: 1024,
memLevel: 7,
level: 3
},
zlibInflateOptions: {
chunkSize: 10 * 1024
},
// Other options, see the ws documentation
clientNoContextTakeover: true,
serverNoContextTakeover: true,
serverMaxWindowBits: 10,
concurrencyLimit: 10,
threshold: 1024 // Only compress messages larger than this size
}
});
4. WebSocket Authentication
Implementing authentication for WebSocket connections is crucial for securing your application. One common approach is to use token-based authentication. Here’s a basic example:
// Client-side
const token = 'your-auth-token';
const socket = new WebSocket(`ws://example.com/socketserver?token=${token}`);
// Server-side (Node.js with 'ws')
const WebSocket = require('ws');
const url = require('url');
const wss = new WebSocket.Server({ port: 8080 });
wss.on('connection', (ws, req) => {
const { query } = url.parse(req.url, true);
const token = query.token;
if (!isValidToken(token)) {
ws.close(1008, 'Invalid token');
return;
}
// Proceed with the authenticated connection
});
function isValidToken(token) {
// Implement your token validation logic here
return token === 'valid-token';
}
Scaling WebSocket Applications
As your WebSocket application grows, you’ll need to consider scalability to handle a large number of concurrent connections. Here are some strategies for scaling WebSocket applications:
1. Load Balancing
Use a load balancer to distribute WebSocket connections across multiple server instances. Ensure that your load balancer supports WebSocket connections and sticky sessions if required.
2. Horizontal Scaling
Deploy multiple WebSocket server instances behind a load balancer to handle increased traffic. Use a shared storage solution (e.g., Redis) to maintain state across instances.
3. Message Queues
Implement message queues (e.g., RabbitMQ, Apache Kafka) to handle message distribution between server instances and ensure reliable message delivery.
4. Microservices Architecture
Consider breaking down your application into microservices, with dedicated services for WebSocket connections and message processing.
5. Connection Pooling
Implement connection pooling to efficiently manage and reuse WebSocket connections, reducing the overhead of establishing new connections.
Monitoring and Debugging WebSocket Applications
Proper monitoring and debugging are essential for maintaining healthy WebSocket applications. Here are some tips and tools to help you monitor and debug your WebSocket implementations:
1. Logging
Implement comprehensive logging for both client and server-side WebSocket events. Log connection establishments, closures, errors, and message exchanges to help diagnose issues.
2. WebSocket Inspection Tools
Use browser developer tools to inspect WebSocket traffic. Most modern browsers provide built-in WebSocket debugging capabilities in their Network tab.
3. Monitoring Tools
Utilize application performance monitoring (APM) tools that support WebSocket monitoring, such as New Relic, Datadog, or AppDynamics.
4. Custom Metrics
Implement custom metrics to track important WebSocket-related data, such as the number of active connections, message rates, and connection durations.
5. Testing Tools
Use WebSocket testing tools like Postman or wscat to simulate client connections and test your WebSocket server’s behavior.
Conclusion
WebSockets have revolutionized the way we build real-time web applications, enabling seamless, bidirectional communication between clients and servers. By understanding the core concepts, implementing best practices, and leveraging advanced techniques, you can create powerful, efficient, and scalable real-time applications that provide engaging user experiences.
As you continue to explore and work with WebSockets, remember to stay updated on the latest developments in the field, as new tools, libraries, and best practices are constantly emerging. With the knowledge gained from this guide, you’re well-equipped to tackle real-time web development challenges and create innovative applications that push the boundaries of what’s possible on the web.
Happy coding, and may your WebSocket connections always remain open and responsive!