Understanding Real-Time Operating Systems (RTOS): Programming for Immediate Processing
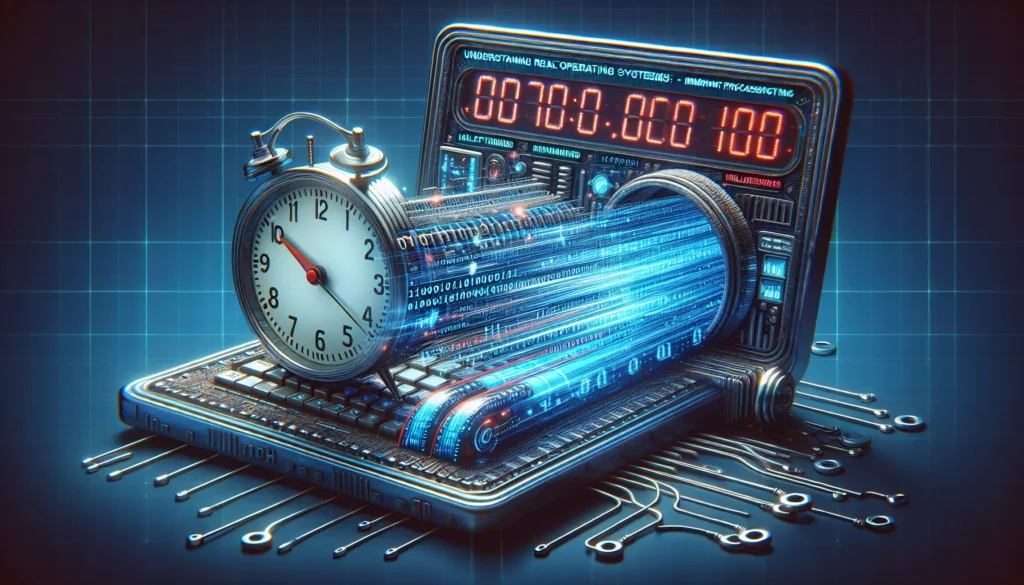
In the world of computing, timing is everything. While most operating systems we use daily, like Windows or macOS, are designed for general-purpose computing, there are specialized systems where precise timing and immediate response are crucial. This is where Real-Time Operating Systems (RTOS) come into play. In this comprehensive guide, we’ll dive deep into the world of RTOS, exploring their importance, characteristics, and how to program systems that require immediate processing.
What is a Real-Time Operating System (RTOS)?
A Real-Time Operating System (RTOS) is a specialized operating system designed to handle time-critical tasks with precise timing constraints. Unlike general-purpose operating systems, an RTOS guarantees that certain operations will be completed within specified time limits. This predictability is crucial for applications where a delayed response could lead to system failure or even catastrophic consequences.
Key Characteristics of RTOS:
- Deterministic Timing: RTOS ensures that tasks are completed within predetermined time constraints.
- Priority-based Scheduling: Tasks are assigned priorities, and the highest priority task always runs first.
- Minimal Interrupt Latency: RTOS responds to interrupts quickly and predictably.
- Preemptive Multitasking: Higher priority tasks can interrupt and take control from lower priority tasks.
- Reliability: RTOS is designed to be highly reliable and fault-tolerant.
Types of Real-Time Systems
Real-time systems are generally categorized into two main types:
1. Hard Real-Time Systems
In hard real-time systems, meeting deadlines is absolutely critical. Failure to complete a task within its specified time frame can result in system failure or even catastrophic consequences. Examples include:
- Aircraft control systems
- Medical equipment (e.g., pacemakers)
- Industrial control systems
- Automotive systems (e.g., airbag deployment)
2. Soft Real-Time Systems
Soft real-time systems have less stringent timing requirements. While they aim to meet deadlines, occasional missed deadlines won’t result in critical failure. However, the system’s overall performance may degrade. Examples include:
- Multimedia streaming
- Online gaming
- Virtual reality applications
- Telecommunication systems
Key Components of an RTOS
To understand how to program for RTOS, it’s essential to familiarize yourself with its key components:
1. Kernel
The kernel is the core of the RTOS, responsible for managing system resources and providing essential services. It handles task scheduling, inter-task communication, and memory management.
2. Scheduler
The scheduler determines which task should run next based on priority and timing constraints. It ensures that high-priority tasks are executed promptly.
3. Task Management
RTOS provides mechanisms for creating, deleting, and managing tasks (also called threads). Each task has its own priority and execution context.
4. Inter-Task Communication
RTOS offers various mechanisms for tasks to communicate and synchronize with each other, such as semaphores, mutexes, and message queues.
5. Memory Management
Efficient memory allocation and deallocation are crucial in RTOS. Many RTOSes provide specialized memory management techniques to ensure predictable behavior.
6. Interrupt Handling
RTOS must be able to handle hardware and software interrupts quickly and predictably to maintain real-time responsiveness.
Programming for RTOS: Best Practices and Considerations
When developing applications for RTOS, there are several best practices and considerations to keep in mind:
1. Choose the Right RTOS
Select an RTOS that meets your specific requirements in terms of performance, supported hardware, and development tools. Popular RTOS options include:
- FreeRTOS
- VxWorks
- QNX
- RT-Thread
- Zephyr
2. Understand Task Priorities
Properly assigning task priorities is crucial in RTOS programming. Higher priority tasks should be reserved for time-critical operations, while less critical tasks can have lower priorities.
3. Minimize Task Switching
Excessive task switching can lead to increased overhead and reduced system performance. Design your tasks to minimize context switches where possible.
4. Use Appropriate Synchronization Mechanisms
Choose the right synchronization primitives (e.g., semaphores, mutexes) for inter-task communication and resource sharing. Be aware of potential issues like priority inversion and deadlocks.
5. Optimize Memory Usage
Efficiently manage memory to avoid fragmentation and ensure predictable allocation times. Consider using static allocation where possible for critical tasks.
6. Handle Interrupts Carefully
Keep interrupt service routines (ISRs) short and efficient. Use deferred processing techniques to handle longer operations outside of the ISR context.
7. Implement Robust Error Handling
Design your system to handle errors gracefully without compromising real-time performance. Implement appropriate error detection and recovery mechanisms.
8. Use RTOS-Specific APIs
Leverage the APIs provided by your chosen RTOS for task management, synchronization, and timing functions instead of relying on standard library functions.
Example: Creating a Simple RTOS Task
Let’s look at a simple example of creating and running a task in FreeRTOS, one of the most popular open-source RTOS options:
#include "FreeRTOS.h"
#include "task.h"
void vTaskFunction(void *pvParameters)
{
for (;;)
{
// Task code goes here
printf("Hello from RTOS task!\n");
vTaskDelay(pdMS_TO_TICKS(1000)); // Delay for 1 second
}
}
int main(void)
{
// Create the task
xTaskCreate(
vTaskFunction, // Function that implements the task
"ExampleTask", // Text name for the task
configMINIMAL_STACK_SIZE, // Stack size
NULL, // Parameter passed into the task
tskIDLE_PRIORITY + 1,// Priority
NULL // Task handle
);
// Start the scheduler
vTaskStartScheduler();
// Will not get here unless there is insufficient RAM
for (;;);
}
In this example, we create a simple task that prints a message every second. The xTaskCreate
function is used to create the task, specifying its function, name, stack size, priority, and other parameters. The vTaskStartScheduler
function starts the RTOS scheduler, which begins executing tasks based on their priorities.
Challenges in RTOS Programming
While RTOS provides powerful capabilities for real-time applications, it also presents unique challenges:
1. Determinism vs. Performance
Balancing the need for deterministic behavior with overall system performance can be challenging. Techniques that improve average-case performance may introduce unpredictability in worst-case scenarios.
2. Resource Constraints
Many RTOS applications run on embedded systems with limited resources. Efficient use of CPU, memory, and power is crucial.
3. Debugging Complexity
Debugging real-time systems can be more complex than general-purpose applications due to timing-sensitive behavior and potential race conditions.
4. Priority Inversion
Priority inversion occurs when a high-priority task is indirectly preempted by a lower-priority task. Proper use of priority inheritance protocols is necessary to mitigate this issue.
5. Interrupt Handling
Balancing the need for quick interrupt response with the overhead of context switching can be challenging.
Advanced RTOS Concepts
As you delve deeper into RTOS programming, you’ll encounter more advanced concepts:
1. Rate Monotonic Scheduling (RMS)
RMS is a priority assignment algorithm for periodic tasks in real-time systems. It assigns priorities based on task periods, with shorter periods receiving higher priorities.
2. Earliest Deadline First (EDF) Scheduling
EDF is a dynamic scheduling algorithm that prioritizes tasks based on their absolute deadlines. It can achieve higher CPU utilization than static priority schemes in some cases.
3. Time Partitioning
In safety-critical systems, time partitioning is used to isolate tasks and ensure that the failure of one task doesn’t affect others. This is common in avionics systems.
4. Formal Verification
For critical real-time systems, formal methods are used to mathematically prove that timing constraints are met under all possible scenarios.
RTOS in the Context of Modern Computing
While RTOS has traditionally been associated with embedded systems, its principles are becoming increasingly relevant in other areas of computing:
1. Internet of Things (IoT)
Many IoT devices require real-time capabilities for sensor data processing and actuation. RTOS principles are often applied in IoT firmware development.
2. Autonomous Vehicles
Self-driving cars rely heavily on real-time systems for sensor fusion, decision making, and control. RTOS concepts are crucial for ensuring safe and timely responses.
3. Edge Computing
As computation moves closer to data sources in edge computing scenarios, real-time processing becomes more important for applications like industrial automation and smart cities.
4. 5G and Beyond
Next-generation telecommunication systems require ultra-low latency and high reliability, driving the need for real-time capabilities in network infrastructure.
Conclusion
Understanding and programming Real-Time Operating Systems is a crucial skill in today’s interconnected world of smart devices, autonomous systems, and time-critical applications. By mastering RTOS concepts and best practices, developers can create robust, efficient, and responsive systems that meet the demanding requirements of real-time applications.
As we’ve explored in this guide, RTOS programming requires a different mindset compared to general-purpose application development. It demands careful consideration of timing constraints, resource management, and system behavior under various scenarios. However, with the right knowledge and tools, developers can harness the power of RTOS to create innovative solutions that push the boundaries of what’s possible in real-time computing.
Whether you’re working on embedded systems, IoT devices, or cutting-edge autonomous technologies, the principles of RTOS will serve as a solid foundation for building reliable and efficient real-time applications. As technology continues to evolve, the importance of real-time systems is only set to grow, making RTOS skills an invaluable asset for any developer looking to stay at the forefront of the industry.
Remember, mastering RTOS is not just about learning a new set of APIs or programming techniques. It’s about developing a deep understanding of system behavior, timing analysis, and the intricate dance of tasks and interrupts that make up a real-time system. With practice and experience, you’ll be well-equipped to tackle the exciting challenges that lie at the intersection of hardware and software in the world of real-time computing.