Understanding React’s Virtual DOM: A Deep Dive into Efficient UI Updates
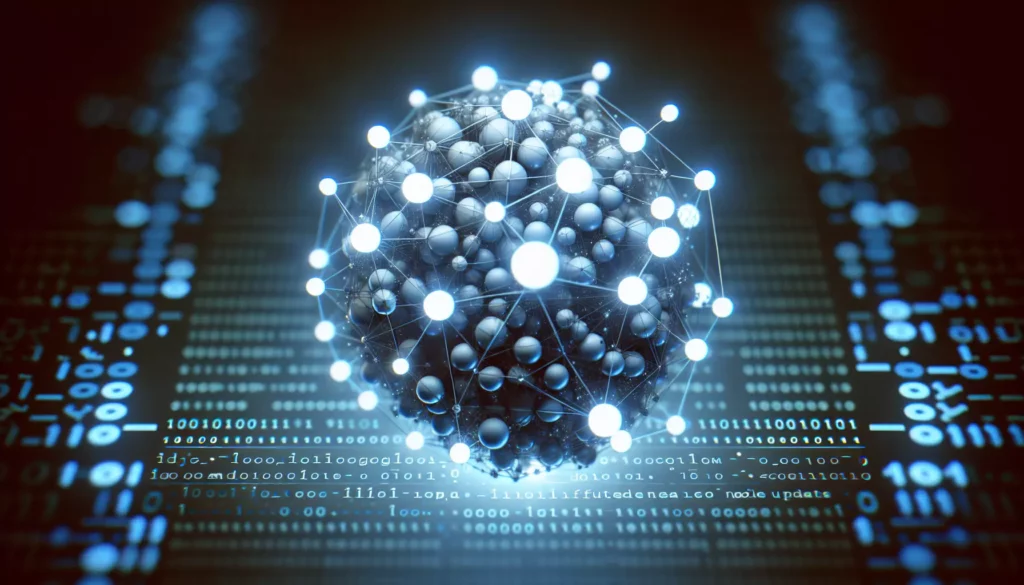
In the world of web development, efficiency and performance are paramount. As applications grow in complexity, managing the Document Object Model (DOM) becomes increasingly challenging. This is where React, a popular JavaScript library for building user interfaces, shines with its innovative approach to DOM manipulation through the Virtual DOM. In this comprehensive guide, we’ll explore the concept of React’s Virtual DOM, its benefits, and how it contributes to the overall performance of web applications.
What is the Virtual DOM?
Before we dive into the Virtual DOM, let’s briefly recap what the DOM is. The Document Object Model (DOM) is a programming interface for HTML and XML documents. It represents the structure of a document as a tree-like hierarchy of objects, where each object represents a part of the document, such as an element, attribute, or text node.
The Virtual DOM, on the other hand, is a lightweight copy of the actual DOM. It’s a JavaScript object that mimics the structure of the DOM but exists entirely in memory. React uses this virtual representation to perform operations and comparisons before making any changes to the actual DOM.
How Does the Virtual DOM Work?
The process of updating the UI using React’s Virtual DOM can be broken down into several steps:
- Initial Render: When a React component is first rendered, React creates a virtual DOM representation of the entire UI.
- State or Props Change: When a component’s state or props change, React creates a new virtual DOM tree reflecting these changes.
- Diffing: React then compares the new virtual DOM with the previous one, identifying the differences (or “diffs”) between them.
- Reconciliation: Based on the identified differences, React determines the most efficient way to update the actual DOM.
- Batch Update: Finally, React applies all the necessary changes to the real DOM in a single batch, minimizing the number of DOM manipulations.
Benefits of the Virtual DOM
The Virtual DOM offers several advantages that contribute to React’s popularity and efficiency:
1. Performance Optimization
By minimizing direct manipulation of the DOM, React reduces the number of expensive DOM operations. This leads to improved performance, especially in complex applications with frequent updates.
2. Cross-platform Compatibility
The Virtual DOM abstraction allows React to be used in various environments beyond just web browsers. This is why React can be used to develop mobile applications (React Native) and even desktop applications.
3. Declarative API
React’s use of the Virtual DOM allows developers to write more declarative code. Instead of manually specifying DOM updates, developers can simply describe how the UI should look at any given point, and React takes care of the updates.
4. Improved Developer Experience
The Virtual DOM simplifies the mental model for developers. They can think in terms of how the UI should look rather than the specific steps needed to get there, leading to more intuitive and maintainable code.
Virtual DOM in Action: A Simple Example
Let’s look at a simple example to illustrate how the Virtual DOM works in practice. Consider a basic React component that displays a counter:
import React, { useState } from 'react';
function Counter() {
const [count, setCount] = useState(0);
return (
<div>
<p>Count: {count}</p>
<button onClick={() => setCount(count + 1)}>Increment</button>
</div>
);
}
In this example, when the user clicks the “Increment” button, here’s what happens behind the scenes:
- The
setCount
function is called, updating the component’s state. - React creates a new Virtual DOM tree representing the updated UI.
- React compares this new Virtual DOM tree with the previous one.
- React identifies that only the text content of the
<p>
element needs to change. - React efficiently updates only that specific part of the actual DOM.
This process ensures that only the necessary changes are made to the DOM, avoiding unnecessary re-renders and improving performance.
The Diffing Algorithm
At the heart of React’s Virtual DOM implementation is its diffing algorithm. This algorithm is responsible for identifying the differences between the new and old Virtual DOM trees. Understanding how this algorithm works can provide insights into optimizing React applications:
1. Element Type Comparison
React first compares the root elements. If the element types are different, it assumes the entire subtree has changed and rebuilds it from scratch.
2. Props Comparison
For elements of the same type, React looks at the attributes and updates only the changed attributes.
3. Child Reconciliation
When dealing with lists of children, React uses keys to efficiently track which items have been added, removed, or modified.
4. Component Lifecycle
For custom components, React calls lifecycle methods like componentDidUpdate
or effects in function components to handle side effects of updates.
Keys in React: Optimizing List Rendering
When rendering lists of elements in React, using keys is crucial for optimizing the diffing process. Keys help React identify which items in a list have changed, been added, or been removed. Here’s an example:
function TodoList({ todos }) {
return (
<ul>
{todos.map((todo) => (
<li key={todo.id}>{todo.text}</li>
))}
</ul>
);
}
By providing a unique key
prop to each list item, React can efficiently update the list when items are added, removed, or reordered.
Common Misconceptions about the Virtual DOM
While the Virtual DOM is a powerful concept, there are some common misconceptions about it:
1. The Virtual DOM is Always Faster
While the Virtual DOM generally leads to performance improvements, it’s not always faster than direct DOM manipulation. In simple applications or for one-time renders, the overhead of the Virtual DOM might not be necessary.
2. The Virtual DOM Eliminates the Need for Optimization
Although the Virtual DOM helps optimize updates, developers still need to be mindful of performance. Unnecessary re-renders or deep component trees can still impact performance.
3. The Virtual DOM is Unique to React
While React popularized the concept, other libraries and frameworks have implemented similar virtual DOM concepts, such as Vue.js and Ember.js.
Best Practices for Working with React’s Virtual DOM
To make the most of React’s Virtual DOM, consider these best practices:
1. Use the shouldComponentUpdate Method
For class components, implementing shouldComponentUpdate
can prevent unnecessary re-renders:
class MyComponent extends React.Component {
shouldComponentUpdate(nextProps, nextState) {
return nextProps.value !== this.props.value;
}
render() {
return <div>{this.props.value}</div>;
}
}
2. Use React.memo for Function Components
For function components, React.memo
can be used to achieve similar optimization:
const MyComponent = React.memo(function MyComponent({ value }) {
return <div>{value}</div>;
});
3. Avoid Inline Function Definitions in Render
Inline function definitions can lead to unnecessary re-renders. Instead, define functions outside the render method:
// Avoid
<button onClick={() => this.handleClick()}>Click me</button>
// Prefer
handleClick = () => {
// handle click
}
render() {
return <button onClick={this.handleClick}>Click me</button>;
}
4. Use Immutable Data Structures
Immutable data structures can help React more efficiently determine when to re-render components:
// Instead of modifying an array directly
this.state.items.push(newItem);
// Create a new array
this.setState(prevState => ({
items: [...prevState.items, newItem]
}));
The Future of Virtual DOM
As web development evolves, so does the concept of the Virtual DOM. React’s team is continuously working on improvements and new features:
1. Concurrent Mode
React’s experimental Concurrent Mode aims to make React apps more responsive by rendering component trees without blocking the main thread.
2. Server Components
React Server Components is a new feature that allows components to be rendered on the server, reducing the amount of JavaScript sent to the client.
3. Incremental Static Regeneration
Frameworks like Next.js are leveraging React’s architecture to enable incremental static regeneration, combining the benefits of static site generation with dynamic content.
Conclusion
React’s Virtual DOM is a powerful abstraction that has revolutionized the way we build user interfaces for the web. By providing a layer between the developer’s code and the actual DOM manipulations, React offers a more efficient and developer-friendly approach to building complex, interactive web applications.
Understanding the Virtual DOM and its underlying mechanisms not only helps in writing more efficient React code but also provides insights into modern web development practices. As you continue your journey in web development, keep exploring the depths of React and its ecosystem – there’s always more to learn and discover in this ever-evolving field.
Remember, while the Virtual DOM is a crucial part of React’s efficiency, it’s just one piece of the puzzle. Combining this knowledge with other best practices in state management, component design, and performance optimization will help you build truly outstanding web applications.
Happy coding, and may your React applications be forever smooth and performant!