Understanding Radix Sort and Its Applications
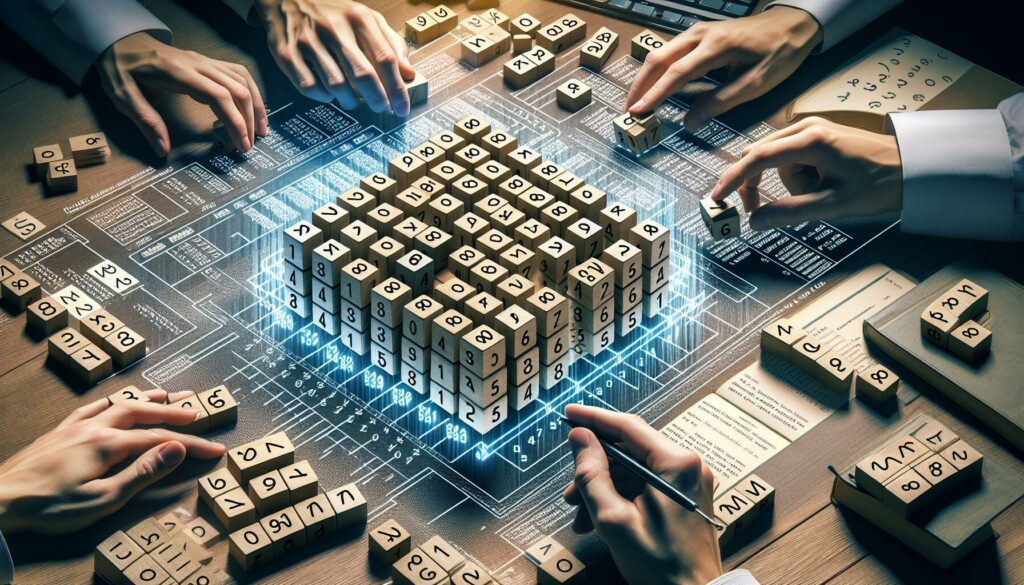
In the world of computer science and algorithm design, sorting algorithms play a crucial role in organizing and managing data efficiently. While popular algorithms like Quicksort and Mergesort are widely known, there’s another powerful sorting technique that deserves attention: Radix Sort. In this comprehensive guide, we’ll dive deep into Radix Sort, exploring its mechanics, implementation, time complexity, and real-world applications.
What is Radix Sort?
Radix Sort is a non-comparative integer sorting algorithm that sorts data with integer keys by grouping the keys by individual digits sharing the same significant position and value. Unlike comparison-based sorting algorithms, Radix Sort doesn’t compare elements directly. Instead, it exploits the fact that information about the size of a number is encoded in the number of digits.
The name “Radix” comes from the way the algorithm processes each digit position. The radix is the base of the number system being used. For example, in the decimal system, the radix is 10, while in the binary system, it’s 2.
How Radix Sort Works
Radix Sort operates by processing the digits of the numbers from least significant to most significant (or vice versa). Here’s a step-by-step breakdown of the algorithm:
- Find the maximum element in the array to determine the number of digits in the largest number.
- For each digit position, from least significant to most significant:
- Sort the elements based on the current digit using a stable sorting algorithm (usually Counting Sort).
- Collect the elements in order after sorting.
- Repeat step 2 until all digit positions have been processed.
The key to Radix Sort’s efficiency is the use of a stable sorting algorithm for each digit. This ensures that the relative order of elements with the same digit value is preserved.
Implementing Radix Sort
Let’s implement Radix Sort in Python to better understand its mechanics:
def counting_sort(arr, exp):
n = len(arr)
output = [0] * n
count = [0] * 10
for i in range(n):
index = arr[i] // exp
count[index % 10] += 1
for i in range(1, 10):
count[i] += count[i - 1]
i = n - 1
while i >= 0:
index = arr[i] // exp
output[count[index % 10] - 1] = arr[i]
count[index % 10] -= 1
i -= 1
for i in range(n):
arr[i] = output[i]
def radix_sort(arr):
max_num = max(arr)
exp = 1
while max_num // exp > 0:
counting_sort(arr, exp)
exp *= 10
# Example usage
arr = [170, 45, 75, 90, 802, 24, 2, 66]
radix_sort(arr)
print("Sorted array:", arr)
In this implementation, we use Counting Sort as the stable sorting algorithm for each digit. The counting_sort
function sorts the array based on a specific digit position, while the radix_sort
function orchestrates the overall sorting process.
Time and Space Complexity
The time complexity of Radix Sort is O(d * (n + k)), where:
- d is the number of digits in the maximum element
- n is the number of elements in the array
- k is the range of values for each digit (e.g., 10 for decimal system)
When d is constant and k is significantly smaller than n, the time complexity can be simplified to O(n). This makes Radix Sort linear time in practice for many common scenarios.
The space complexity of Radix Sort is O(n + k), as it requires additional space for the output array and the count array used in Counting Sort.
Advantages of Radix Sort
- Linear Time Complexity: For many practical scenarios, Radix Sort achieves linear time complexity, making it faster than comparison-based sorting algorithms for large datasets.
- Stable Sorting: Radix Sort is a stable sorting algorithm, meaning it preserves the relative order of equal elements.
- Efficient for Fixed-Length Integer Keys: When dealing with integers or strings of fixed length, Radix Sort can be particularly efficient.
Limitations of Radix Sort
- Limited to Integer or String Keys: Radix Sort is primarily designed for sorting integers or strings and may not be directly applicable to other data types.
- Overhead for Small Datasets: For small datasets, the overhead of multiple passes and additional memory usage may make Radix Sort less efficient than simpler algorithms.
- Sensitivity to Data Distribution: The performance of Radix Sort can be affected by the distribution of data, particularly when there’s a large range of values.
Applications of Radix Sort
Radix Sort finds applications in various domains where efficient sorting of integers or strings is required:
1. Sorting Large Files
When dealing with large files containing integer or string data, Radix Sort can be an efficient choice. Its linear time complexity makes it suitable for processing massive datasets, such as log files or database records.
2. String Sorting
Radix Sort can be adapted to sort strings efficiently. By treating each character as a digit, we can sort strings lexicographically. This is particularly useful in applications like:
- Dictionary sorting
- URL sorting in web crawlers
- Organizing file names in file systems
3. Integer Sorting in Databases
Database systems often need to sort large volumes of integer data, such as user IDs, timestamps, or numerical attributes. Radix Sort can be employed to optimize these sorting operations, especially when dealing with fixed-width integer fields.
4. Network Routing Tables
In computer networking, routing tables often contain IP addresses that need to be sorted efficiently. Radix Sort can be used to organize these addresses, facilitating faster lookup and routing decisions.
5. Sorting in Graphics Applications
Computer graphics applications, such as rendering engines, may use Radix Sort to efficiently sort polygons or pixels based on their depth or other attributes. This can help in implementing algorithms like painter’s algorithm for hidden surface removal.
6. Numerical Analysis
In scientific computing and numerical analysis, Radix Sort can be useful for sorting floating-point numbers. By converting floating-point values to integers while preserving their order, Radix Sort can be applied to achieve efficient sorting.
Optimizing Radix Sort
While the basic implementation of Radix Sort is already efficient, there are several optimizations that can further improve its performance:
1. Hybrid Approach
For small subarrays or when the number of digits is small, it may be more efficient to switch to a comparison-based sorting algorithm like Insertion Sort. This hybrid approach can reduce the overhead of multiple passes for small datasets.
2. Parallelization
Radix Sort can be parallelized to take advantage of multi-core processors. Each digit position can be processed independently, allowing for concurrent sorting of different digit positions.
3. In-place Sorting
While the basic implementation uses additional space, it’s possible to implement Radix Sort in-place, reducing the space complexity to O(1). However, this often comes at the cost of increased time complexity.
4. Adaptive Radix Sort
By analyzing the input data distribution, an adaptive version of Radix Sort can dynamically adjust its behavior. For example, it can skip unnecessary passes when certain digit positions are not significant for the given dataset.
Comparison with Other Sorting Algorithms
To better understand the strengths and weaknesses of Radix Sort, let’s compare it with some popular sorting algorithms:
Radix Sort vs. Quicksort
- Time Complexity: Radix Sort: O(d * (n + k)), Quicksort: O(n log n) on average
- Space Complexity: Radix Sort: O(n + k), Quicksort: O(log n) for the recursive call stack
- Stability: Radix Sort is stable, Quicksort is not stable in its basic form
- In-place Sorting: Radix Sort typically requires additional space, while Quicksort can be implemented in-place
Radix Sort can outperform Quicksort for large datasets with a limited range of values, but Quicksort is more versatile and often preferred for general-purpose sorting.
Radix Sort vs. Mergesort
- Time Complexity: Radix Sort: O(d * (n + k)), Mergesort: O(n log n)
- Space Complexity: Radix Sort: O(n + k), Mergesort: O(n)
- Stability: Both are stable sorting algorithms
- Adaptability: Mergesort is more adaptable to different data types and can be easily parallelized
Radix Sort can be faster than Mergesort for integer sorting, but Mergesort is more flexible and efficient for a wider range of data types.
Radix Sort vs. Counting Sort
- Time Complexity: Radix Sort: O(d * (n + k)), Counting Sort: O(n + k)
- Space Complexity: Radix Sort: O(n + k), Counting Sort: O(k)
- Range of Values: Radix Sort can handle a larger range of values more efficiently
- Applicability: Counting Sort is limited to integers with a small range, while Radix Sort can handle larger ranges and can be adapted for string sorting
Radix Sort can be seen as a generalization of Counting Sort, allowing it to handle a wider range of values efficiently.
Implementing Radix Sort for String Sorting
To illustrate the versatility of Radix Sort, let’s implement a version that can sort strings lexicographically:
def counting_sort_strings(arr, position):
n = len(arr)
output = [""] * n
count = [0] * 256 # Assuming ASCII characters
for s in arr:
if position < len(s):
count[ord(s[position])] += 1
else:
count[0] += 1 # Treat shorter strings as if padded with null characters
for i in range(1, 256):
count[i] += count[i - 1]
for i in range(n - 1, -1, -1):
if position < len(arr[i]):
char = ord(arr[i][position])
else:
char = 0
output[count[char] - 1] = arr[i]
count[char] -= 1
for i in range(n):
arr[i] = output[i]
def radix_sort_strings(arr):
max_len = max(len(s) for s in arr)
for position in range(max_len - 1, -1, -1):
counting_sort_strings(arr, position)
# Example usage
strings = ["apple", "banana", "cherry", "date", "elderberry", "fig", "grape"]
radix_sort_strings(strings)
print("Sorted strings:", strings)
This implementation sorts strings from the least significant character (rightmost) to the most significant character (leftmost). It handles strings of different lengths by treating shorter strings as if they were padded with null characters.
Radix Sort in Practice: A Case Study
To better understand the practical applications of Radix Sort, let’s consider a real-world scenario: sorting a large dataset of IP addresses.
Imagine you’re working on a network analysis tool that needs to process millions of IP addresses from log files. The goal is to sort these IP addresses efficiently to identify patterns, detect anomalies, or generate reports.
Here’s how we can implement Radix Sort for IP addresses:
def ip_to_int(ip):
return int(".".join(["%03d" % int(x) for x in ip.split(".")]))
def int_to_ip(num):
return ".".join([str(int(num // (256 ** i) % 256)) for i in range(3, -1, -1)])
def counting_sort_ip(arr, exp):
n = len(arr)
output = [0] * n
count = [0] * 256
for i in range(n):
index = (arr[i] // exp) % 256
count[index] += 1
for i in range(1, 256):
count[i] += count[i - 1]
i = n - 1
while i >= 0:
index = (arr[i] // exp) % 256
output[count[index] - 1] = arr[i]
count[index] -= 1
i -= 1
for i in range(n):
arr[i] = output[i]
def radix_sort_ip(arr):
max_num = max(arr)
exp = 1
while max_num // exp > 0:
counting_sort_ip(arr, exp)
exp *= 256
# Example usage
ip_addresses = [
"192.168.0.1",
"10.0.0.1",
"172.16.0.1",
"192.168.1.1",
"10.10.10.10",
"8.8.8.8"
]
# Convert IP addresses to integers
ip_ints = [ip_to_int(ip) for ip in ip_addresses]
# Sort the integer representations
radix_sort_ip(ip_ints)
# Convert back to IP address strings
sorted_ips = [int_to_ip(num) for num in ip_ints]
print("Sorted IP addresses:", sorted_ips)
In this implementation:
- We convert IP addresses to integer representations to facilitate sorting.
- We apply Radix Sort to these integer representations, treating each byte of the IP address as a digit.
- After sorting, we convert the integers back to IP address strings.
This approach allows us to efficiently sort large numbers of IP addresses, which can be crucial for tasks like:
- Identifying the most frequent IP addresses in log files
- Detecting unusual patterns or potential security threats
- Organizing network traffic data for analysis
- Optimizing routing tables in network devices
Conclusion
Radix Sort is a powerful and efficient sorting algorithm that shines in specific scenarios, particularly when dealing with integer or string data. Its linear time complexity makes it an attractive choice for large datasets with a limited range of values.
Key takeaways about Radix Sort include:
- It’s a non-comparative sorting algorithm that sorts data digit by digit.
- It achieves linear time complexity in many practical scenarios.
- It’s particularly efficient for sorting integers, strings, and other data that can be represented as sequences of digits or characters.
- It finds applications in various domains, including file sorting, string processing, and network data analysis.
- While it has some limitations, such as being less flexible than comparison-based sorts, it can significantly outperform other algorithms in its specialized domain.
As you continue your journey in algorithm design and implementation, keep Radix Sort in your toolbox. Understanding when and how to apply it can lead to significant performance improvements in your applications, especially when dealing with large-scale data processing tasks.
Remember, the key to becoming a proficient programmer is not just knowing individual algorithms, but understanding their strengths, weaknesses, and appropriate use cases. Radix Sort is a perfect example of how a specialized algorithm can offer substantial benefits when applied in the right context.