Understanding Python’s Yield Keyword: A Comprehensive Guide
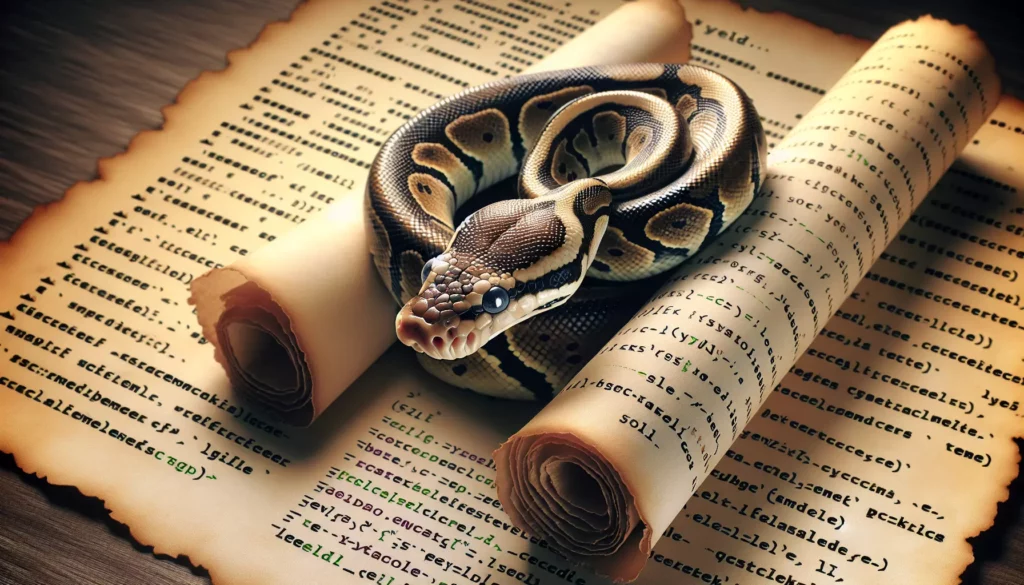
Python is a versatile and powerful programming language, known for its simplicity and readability. One of the features that contributes to its efficiency is the yield
keyword. In this comprehensive guide, we’ll explore the yield
keyword in depth, discussing its purpose, usage, and benefits in Python programming.
Table of Contents
- What is the Yield Keyword?
- How Yield Works
- Generators: The Power Behind Yield
- Advantages of Using Yield
- Common Use Cases for Yield
- Yield vs. Return: Understanding the Difference
- Advanced Yield Techniques
- Best Practices and Tips
- Common Pitfalls and How to Avoid Them
- Conclusion
1. What is the Yield Keyword?
The yield
keyword in Python is a special statement used in functions to define generator functions. When a function contains a yield
statement, it becomes a generator function. Instead of returning a value and terminating, a generator function returns a generator object that can be iterated over to retrieve values one at a time.
The primary purpose of yield
is to generate a sequence of values over time, rather than computing them all at once and storing them in memory. This makes it particularly useful for working with large datasets or infinite sequences.
2. How Yield Works
To understand how yield
works, let’s look at a simple example:
def countdown(n):
while n > 0:
yield n
n -= 1
for number in countdown(5):
print(number)
In this example, the countdown
function is a generator function. When called, it doesn’t execute the function body immediately. Instead, it returns a generator object. The function’s state is saved, and execution is paused.
When the generator is iterated over (in this case, using a for loop), the function resumes where it left off, executes until it encounters the next yield
statement, and then pauses again, returning the value specified in the yield
statement.
This process continues until the function completes or a StopIteration
exception is raised. In our example, the output would be:
5
4
3
2
1
3. Generators: The Power Behind Yield
Generators are the underlying mechanism that makes yield
possible. A generator is a special type of iterator that generates values on-the-fly instead of storing them all in memory. When you use yield
in a function, you’re creating a generator function.
Here are some key points about generators:
- Generators are memory-efficient because they generate values one at a time, rather than creating and storing an entire sequence in memory.
- They can represent infinite sequences, which would be impossible with regular functions that return lists.
- Generators are lazy, meaning they only compute values when requested, which can lead to performance improvements in certain scenarios.
- They can be used in any context where iterables are expected, such as for loops, list comprehensions, and the
sum()
function.
4. Advantages of Using Yield
Using yield
and generators offers several advantages:
- Memory Efficiency: Generators don’t store all values in memory, making them ideal for working with large datasets.
- Improved Performance: For large sequences, generators can be faster than creating and returning a list, especially when you don’t need all values at once.
- Simplicity: Generator functions can make code more readable and easier to understand, especially for complex iterations.
- Lazy Evaluation: Values are computed only when needed, which can be beneficial in certain scenarios.
- Infinite Sequences: Generators can represent infinite sequences, which is not possible with regular functions returning lists.
5. Common Use Cases for Yield
The yield
keyword has many practical applications in Python programming. Here are some common use cases:
5.1 Processing Large Files
When working with large files, using yield
can help you process the file line by line without loading the entire file into memory:
def read_large_file(file_path):
with open(file_path, 'r') as file:
for line in file:
yield line.strip()
for line in read_large_file('large_file.txt'):
print(line)
5.2 Implementing Custom Iterators
You can use yield
to create custom iterators for your own objects:
class Fibonacci:
def __init__(self, limit):
self.limit = limit
self.a, self.b = 0, 1
def __iter__(self):
return self
def __next__(self):
if self.a > self.limit:
raise StopIteration
result = self.a
self.a, self.b = self.b, self.a + self.b
return result
for num in Fibonacci(100):
print(num)
5.3 Generating Infinite Sequences
Yield is perfect for creating infinite sequences, which would be impossible with regular functions:
def infinite_sequence():
num = 0
while True:
yield num
num += 1
for i in infinite_sequence():
print(i)
if i > 100:
break
5.4 Pipelining Data Processing
You can use multiple generator functions to create a data processing pipeline:
def read_data(file_path):
with open(file_path, 'r') as file:
for line in file:
yield line.strip()
def process_data(lines):
for line in lines:
yield line.upper()
def write_data(processed_lines, output_file):
with open(output_file, 'w') as file:
for line in processed_lines:
file.write(line + '\n')
input_file = 'input.txt'
output_file = 'output.txt'
data = read_data(input_file)
processed_data = process_data(data)
write_data(processed_data, output_file)
6. Yield vs. Return: Understanding the Difference
While both yield
and return
are used to produce values from functions, they have significant differences:
- Function Type:
return
is used in regular functions, whileyield
is used in generator functions. - Execution: A function with
return
executes once and returns a value, terminating the function. A generator function withyield
pauses execution and resumes from where it left off when called again. - Memory Usage:
return
typically returns all data at once, which is stored in memory.yield
generates values one at a time, consuming less memory. - Iteration: Values from a regular function need to be stored in a data structure to be iterated over. Generator functions can be iterated over directly.
- State Preservation: Generator functions preserve their state between calls, while regular functions do not.
7. Advanced Yield Techniques
As you become more comfortable with yield
, you can explore some advanced techniques:
7.1 Yield From
The yield from
statement allows you to yield values from another iterator:
def generator1():
yield from range(3)
yield from 'abc'
for item in generator1():
print(item)
This will output:
0
1
2
a
b
c
7.2 Sending Values to Generators
You can send values back into a generator using the send()
method:
def echo_generator():
while True:
value = yield
print(f"Received: {value}")
gen = echo_generator()
next(gen) # Prime the generator
gen.send("Hello")
gen.send("World")
7.3 Using Generators as Coroutines
Generators can be used to implement coroutines for concurrent programming:
def coroutine():
while True:
x = yield
print('Received:', x)
c = coroutine()
next(c) # Prime the coroutine
c.send(10)
c.send(20)
8. Best Practices and Tips
To make the most of yield
and generators, consider these best practices:
- Use generators for large datasets: When working with large amounts of data, generators can significantly improve memory usage and performance.
- Combine generators with other itertools: Python’s
itertools
module provides many useful functions that work well with generators. - Use generator expressions for simple cases: For simple generators, you can use generator expressions, which are more concise than full generator functions.
- Be mindful of side effects: Remember that generator functions maintain state between calls, so be careful with side effects that might affect the generator’s behavior.
- Document your generator functions: Clearly document the purpose and behavior of your generator functions, including any side effects or assumptions about input.
9. Common Pitfalls and How to Avoid Them
While working with yield
and generators, be aware of these common pitfalls:
9.1 Exhausting Generators
Generators are exhausted after they’ve been fully iterated over. Trying to iterate over an exhausted generator will not yield any values:
def count_to_three():
yield 1
yield 2
yield 3
gen = count_to_three()
print(list(gen)) # [1, 2, 3]
print(list(gen)) # [] (generator is exhausted)
To avoid this, create a new generator object if you need to iterate multiple times.
9.2 Mixing Yield and Return
Using return
in a generator function will terminate the generator:
def mixed_generator():
yield 1
yield 2
return "Done"
yield 3 # This will never be reached
gen = mixed_generator()
print(list(gen)) # [1, 2]
If you need to return a final value, consider using StopIteration
exception with a value.
9.3 Forgetting to Prime Coroutines
When using generators as coroutines, don’t forget to prime them by calling next()
or send(None)
before sending values:
def coroutine():
while True:
x = yield
print(f"Received: {x}")
c = coroutine()
c.send("Hello") # This will raise TypeError
To avoid this, always prime your coroutines:
c = coroutine()
next(c) # Prime the coroutine
c.send("Hello") # Now it works
10. Conclusion
The yield
keyword is a powerful feature in Python that enables the creation of memory-efficient, lazy-evaluated sequences through generator functions. By using yield
, you can work with large datasets, create custom iterators, implement infinite sequences, and build efficient data processing pipelines.
Understanding the differences between yield
and return
, as well as mastering advanced techniques like yield from
and sending values to generators, will allow you to write more efficient and elegant Python code.
As with any programming concept, practice is key to mastering the use of yield
. Experiment with different scenarios, challenge yourself to solve problems using generators, and always keep in mind the best practices and potential pitfalls we’ve discussed.
By incorporating yield
and generators into your Python toolkit, you’ll be able to write more efficient, readable, and powerful code, tackling complex problems with ease and elegance.