Understanding Pull Requests in GitHub: A Comprehensive Guide
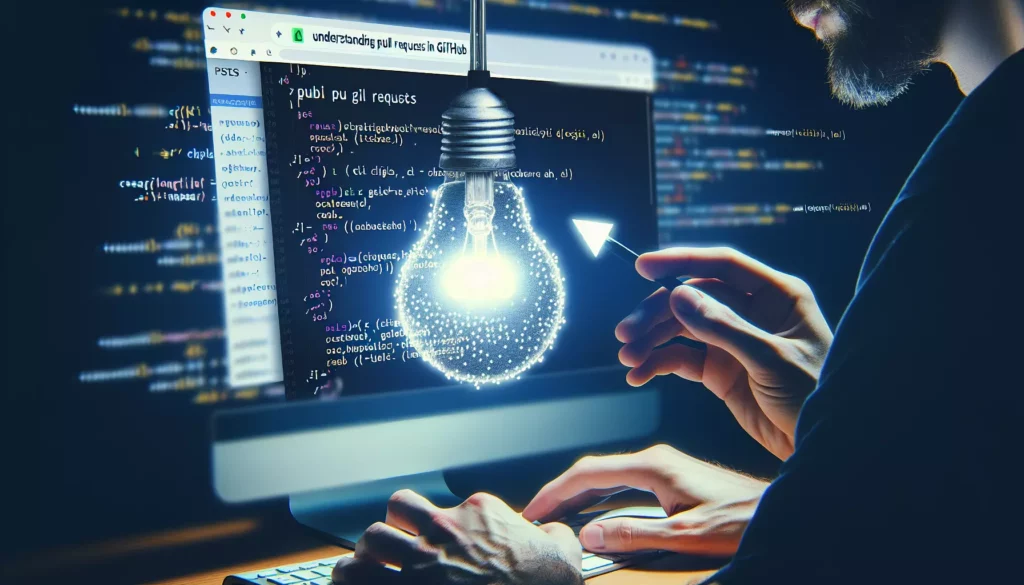
In the world of collaborative software development, GitHub has become an indispensable platform for developers to work together on projects, share code, and contribute to open-source initiatives. One of the most powerful features of GitHub is the Pull Request (PR) system. Whether you’re a beginner just starting your coding journey or an experienced developer preparing for technical interviews at major tech companies, understanding pull requests is crucial for effective collaboration and code management.
In this comprehensive guide, we’ll dive deep into the concept of pull requests, exploring their importance, how they work, and best practices for using them effectively. By the end of this article, you’ll have a solid grasp of pull requests and be ready to leverage them in your own projects or contribute to others.
Table of Contents
- What is a Pull Request?
- Why Use Pull Requests?
- Anatomy of a Pull Request
- Creating a Pull Request
- Reviewing a Pull Request
- Merging a Pull Request
- Best Practices for Pull Requests
- Advanced Techniques and Features
- Common Issues and How to Resolve Them
- Pull Requests in Technical Interviews
- Conclusion
1. What is a Pull Request?
A pull request is a feature in Git-based version control systems, particularly popularized by GitHub, that allows developers to propose changes to a codebase. It’s essentially a way to notify team members that you’ve pushed a new feature or bug fix to a branch in the repository and that you’d like these changes to be reviewed and merged into the main branch.
The term “pull request” comes from the idea that you’re asking the project maintainers to “pull” your changes into their repository. It’s a collaborative tool that facilitates code review, discussion, and eventual integration of new code into the main project.
2. Why Use Pull Requests?
Pull requests offer several benefits that make them an essential part of modern software development workflows:
- Code Review: PRs provide a structured way for team members to review code changes, catch bugs, and suggest improvements before they’re merged into the main codebase.
- Collaboration: They create a space for discussion about the proposed changes, allowing team members to ask questions, provide feedback, and collaborate on solutions.
- Quality Control: By requiring reviews and approvals before merging, PRs help maintain code quality and consistency across the project.
- Documentation: Pull requests serve as a record of changes and discussions, which can be valuable for understanding the evolution of the codebase over time.
- Continuous Integration: Many teams integrate automated testing and continuous integration processes with pull requests to ensure that proposed changes don’t break existing functionality.
3. Anatomy of a Pull Request
A typical pull request consists of several key components:
- Title: A brief, descriptive summary of the changes being proposed.
- Description: A more detailed explanation of the changes, often including the reason for the changes and any potential impacts.
- Base and Compare Branches: The base branch is where you want your changes to be merged, while the compare branch contains your changes.
- Commits: The individual commits that make up the proposed changes.
- Files Changed: A list of all files that have been modified, added, or deleted in the pull request.
- Diff: A line-by-line comparison of the changes made to each file.
- Comments: A section for reviewers to leave feedback, ask questions, or suggest changes.
- Labels: Optional tags that can be added to categorize or provide additional context for the pull request.
- Reviewers: Team members assigned to review the changes.
- Status Checks: Results of automated tests or other checks that run against the proposed changes.
4. Creating a Pull Request
Creating a pull request typically involves the following steps:
- Fork the Repository: If you’re contributing to a project you don’t have direct write access to, start by forking the repository to your own GitHub account.
- Create a New Branch: Always create a new branch for your changes rather than working directly on the main branch.
- Make Your Changes: Implement your feature or fix the bug in your new branch, committing your changes as you go.
- Push Your Branch: Push your new branch with the changes to your fork on GitHub.
- Open the Pull Request: Go to the original repository on GitHub and click the “New Pull Request” button.
- Select Branches: Choose the base branch (usually main or master) and your new branch as the compare branch.
- Fill in Details: Add a title and description for your pull request, explaining what changes you’ve made and why.
- Create the Pull Request: Click “Create Pull Request” to submit it for review.
Here’s a simple example of creating a pull request using the GitHub CLI:
$ git checkout -b feature/new-feature
$ git add .
$ git commit -m "Add new feature"
$ git push origin feature/new-feature
$ gh pr create --title "Add new feature" --body "This PR adds a new feature that..."
5. Reviewing a Pull Request
Reviewing pull requests is a critical part of the development process. Here’s how to effectively review a PR:
- Understand the Context: Read the PR description and any linked issues to understand the purpose of the changes.
- Review the Code: Go through the changed files, looking for potential bugs, code style issues, and areas for improvement.
- Run the Code: If possible, check out the branch locally and run the code to ensure it works as expected.
- Provide Feedback: Leave constructive comments on specific lines of code or on the PR as a whole.
- Suggest Changes: Use GitHub’s suggestion feature to propose specific code changes.
- Approve or Request Changes: Once you’ve completed your review, either approve the PR, request changes, or leave your review as a comment.
Here’s an example of how to review a pull request using the GitHub CLI:
$ gh pr checkout 123
$ gh pr review 123 --approve --body "Looks good! Great work on this feature."
6. Merging a Pull Request
Once a pull request has been approved and all discussions have been resolved, it’s time to merge the changes into the main branch. Here are the steps typically involved in merging a PR:
- Final Review: Do a final check to ensure all comments have been addressed and all checks have passed.
- Choose Merge Method: GitHub offers three merge options:
- Create a merge commit
- Squash and merge
- Rebase and merge
- Merge the PR: Click the “Merge pull request” button and confirm the action.
- Delete the Branch: After merging, you can delete the source branch if it’s no longer needed.
Here’s how to merge a pull request using the GitHub CLI:
$ gh pr merge 123 --merge --delete-branch
7. Best Practices for Pull Requests
To make the most of pull requests and ensure smooth collaboration, consider these best practices:
- Keep PRs Small and Focused: Large PRs are harder to review and more likely to introduce bugs. Aim for smaller, more focused changes.
- Write Clear Descriptions: Provide context, explain your approach, and highlight any important changes or considerations.
- Use Templates: Create PR templates to ensure consistency and prompt contributors to include necessary information.
- Link Related Issues: Connect your PR to related issues to provide more context and track progress.
- Be Responsive: Address comments and feedback promptly to keep the review process moving.
- Use Draft PRs: If your work is still in progress, create a draft PR to signal that it’s not yet ready for review.
- Update Regularly: If the base branch has moved forward, rebase your branch to keep it up to date.
- Write Good Commit Messages: Clear, concise commit messages make it easier to understand the history of changes.
- Include Tests: Add or update tests to cover your changes and prevent regressions.
- Review Your Own PR First: Before requesting reviews, do a self-review to catch any obvious issues or improvements.
8. Advanced Techniques and Features
As you become more comfortable with pull requests, you can explore some advanced techniques and features:
8.1 GitHub Actions
GitHub Actions allow you to automate workflows triggered by pull request events. You can set up actions to run tests, linters, or even deploy preview environments automatically when a PR is opened or updated.
Here’s a simple example of a GitHub Actions workflow that runs tests on pull requests:
<!-- .github/workflows/pr-tests.yml -->
name: PR Tests
on:
pull_request:
branches: [ main ]
jobs:
test:
runs-on: ubuntu-latest
steps:
- uses: actions/checkout@v2
- name: Set up Node.js
uses: actions/setup-node@v2
with:
node-version: '14'
- name: Install dependencies
run: npm ci
- name: Run tests
run: npm test
8.2 Branch Protection Rules
You can set up branch protection rules to enforce certain conditions before a PR can be merged. This might include requiring a certain number of approvals, passing status checks, or requiring a linear commit history.
8.3 Code Owners
The CODEOWNERS file allows you to specify individuals or teams that are responsible for certain parts of the codebase. These code owners will be automatically requested for review when a PR touches their areas of responsibility.
8.4 PR Labeling
Use labels to categorize PRs (e.g., “bug”, “enhancement”, “documentation”) and automate processes based on these labels.
8.5 PR Previews
For web projects, you can set up systems to automatically deploy a preview of your changes, making it easier for reviewers to see the impact of your work.
9. Common Issues and How to Resolve Them
Even with best practices in place, you may encounter some common issues when working with pull requests. Here’s how to handle them:
9.1 Merge Conflicts
Merge conflicts occur when the base branch has changed in a way that conflicts with your changes. To resolve:
- Fetch the latest changes from the base branch
- Merge or rebase the base branch into your feature branch
- Resolve conflicts manually in conflicting files
- Commit the resolved changes and push to update the PR
9.2 Failed CI Checks
If your PR fails automated checks:
- Review the CI logs to understand what failed
- Fix the issues locally and push the changes
- If the failure is a false positive, communicate this in the PR comments
9.3 Stale PRs
For PRs that have been open for a long time:
- Rebase the PR on the latest base branch
- Ping reviewers or request new reviews
- Consider closing the PR if it’s no longer relevant
9.4 Too Many Changes
If your PR has grown too large:
- Consider splitting it into multiple smaller PRs
- Use commit history to tell a clear story of your changes
- Provide a detailed description and potentially a video walkthrough
10. Pull Requests in Technical Interviews
Understanding pull requests is not just important for day-to-day development work; it’s also a topic that may come up in technical interviews, especially for roles at major tech companies. Here are some ways pull requests might be relevant in an interview context:
10.1 Collaboration Scenarios
You might be asked how you would handle certain collaboration scenarios using pull requests. For example:
- How would you handle a situation where a colleague disagrees with your approach in a PR?
- What steps would you take if you found a critical bug in a PR that’s about to be merged?
- How would you structure a large feature implementation using multiple PRs?
10.2 Code Review Skills
Your ability to review code effectively might be assessed. This could involve:
- Being given a sample PR and asked to provide a review
- Discussing your approach to code reviews and what you look for
- Explaining how you would provide constructive feedback on a PR
10.3 Git and GitHub Knowledge
You might be asked about Git commands and GitHub features related to pull requests, such as:
- How to create a PR from the command line
- The difference between merging, rebasing, and squashing when closing a PR
- How to resolve merge conflicts
10.4 Best Practices
Interviewers might ask about best practices for pull requests, including:
- What information you include in a PR description
- How you ensure the quality of your PRs before submitting them for review
- Your strategy for breaking down large features into manageable PRs
10.5 Continuous Integration/Continuous Deployment (CI/CD)
You might be asked about how pull requests fit into a CI/CD pipeline, including:
- How to set up automated tests for PRs
- Strategies for deploying code from PRs to staging environments
- How to handle PRs in a trunk-based development model
To prepare for these types of questions, make sure you have a solid understanding of Git, GitHub features, and best practices for code review and collaboration. Be ready to discuss real-world examples from your experience working with pull requests.
11. Conclusion
Pull requests are a fundamental part of modern software development, enabling collaboration, code review, and quality control. By understanding how to create, review, and manage pull requests effectively, you’ll be better equipped to contribute to projects and collaborate with other developers.
Remember that the key to successful pull requests lies not just in the technical aspects, but also in clear communication, responsiveness, and a willingness to learn and improve. Whether you’re preparing for a technical interview or working on your next big project, mastering the art of pull requests will serve you well in your development career.
As you continue to work with pull requests, you’ll develop your own preferences and workflows. Stay curious, keep learning, and don’t hesitate to explore advanced features and integrations that can make your development process even more efficient and effective.
Happy coding, and may your pull requests always be small, focused, and swiftly merged!