Understanding np.mean: A Comprehensive Guide to Calculating Averages in NumPy
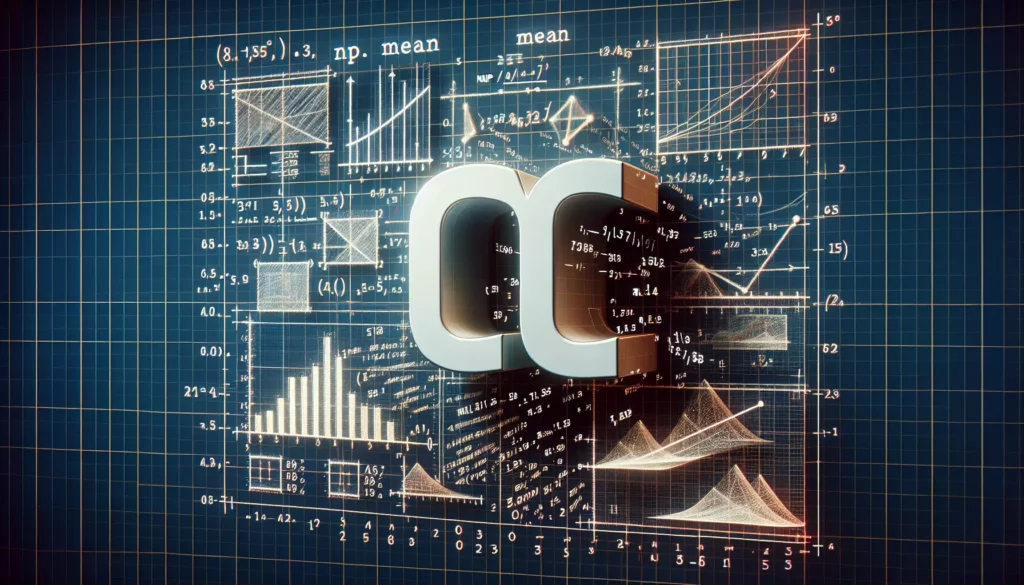
When working with numerical data in Python, calculating the mean (average) of values is one of the most common operations. NumPy, Python’s powerful numerical computing library, offers an efficient and versatile function for this purpose: np.mean()
. Whether you’re analyzing scientific data, developing machine learning models, or simply processing lists of numbers, understanding how to use np.mean()
effectively is an essential skill for any programmer.
In this comprehensive guide, we’ll explore the ins and outs of np.mean()
, from basic usage to advanced applications, complete with practical examples that will enhance your data analysis capabilities.
What is np.mean()?
np.mean()
is a NumPy function that calculates the arithmetic mean of elements in an array. The arithmetic mean is the sum of all values divided by the number of values. It’s a fundamental statistical measure that represents the central tendency of a dataset.
Basic Usage of np.mean()
Before diving into the details, let’s make sure NumPy is installed and imported:
import numpy as np
The simplest use case for np.mean()
is calculating the average of a one-dimensional array:
# Create a simple array
arr = np.array([1, 2, 3, 4, 5])
# Calculate the mean
mean_value = np.mean(arr)
print(mean_value) # Output: 3.0
In this example, np.mean()
adds all the values (1+2+3+4+5=15) and divides by the number of elements (5), resulting in 3.0.
The Syntax of np.mean()
The full syntax of the np.mean()
function is:
numpy.mean(a, axis=None, dtype=None, out=None, keepdims=<no value>, *, where=<no value>)
Let’s break down these parameters:
- a: The input array or object that can be converted to an array.
- axis: The axis along which to compute the mean. By default (None), the mean of the flattened array is computed.
- dtype: The type to use in computing the mean. By default, the data type of the input array is used.
- out: Alternative output array to place the result. Must be of the same shape and buffer length as the expected output.
- keepdims: If set to True, the reduced axes are left in the result as dimensions with size one.
- where: Elements to include in the mean calculation.
Working with Multi-dimensional Arrays
One of the strengths of np.mean()
is its ability to work with multi-dimensional arrays. Let’s see how to calculate means across different dimensions:
2D Array Example
# Create a 2D array
arr_2d = np.array([[1, 2, 3],
[4, 5, 6],
[7, 8, 9]])
# Calculate the mean of the entire array
overall_mean = np.mean(arr_2d)
print(f"Overall mean: {overall_mean}") # Output: 5.0
# Calculate the mean along rows (axis=1)
row_means = np.mean(arr_2d, axis=1)
print(f"Row means: {row_means}") # Output: [2. 5. 8.]
# Calculate the mean along columns (axis=0)
column_means = np.mean(arr_2d, axis=0)
print(f"Column means: {column_means}") # Output: [4. 5. 6.]
In this example:
- The overall mean (5.0) is calculated by taking the average of all nine values.
- The row means [2. 5. 8.] represent the average of each row.
- The column means [4. 5. 6.] represent the average of each column.
3D Array Example
For higher-dimensional arrays, the axis parameter becomes even more important:
# Create a 3D array (2x3x2)
arr_3d = np.array([[[1, 2], [3, 4], [5, 6]],
[[7, 8], [9, 10], [11, 12]]])
# Mean along the first axis (axis=0)
mean_axis0 = np.mean(arr_3d, axis=0)
print("Mean along axis 0:")
print(mean_axis0)
# Output:
# [[ 4. 5.]
# [ 6. 7.]
# [ 8. 9.]]
# Mean along the second axis (axis=1)
mean_axis1 = np.mean(arr_3d, axis=1)
print("Mean along axis 1:")
print(mean_axis1)
# Output:
# [[ 3. 4.]
# [ 9. 10.]]
# Mean along the third axis (axis=2)
mean_axis2 = np.mean(arr_3d, axis=2)
print("Mean along axis 2:")
print(mean_axis2)
# Output:
# [[ 1.5 3.5 5.5]
# [ 7.5 9.5 11.5]]
Handling NaN Values
When working with real-world data, you might encounter missing values represented as NaN (Not a Number). The standard np.mean()
function will return NaN if any value in the array is NaN:
# Array with NaN values
arr_with_nan = np.array([1, 2, np.nan, 4, 5])
# Regular mean
regular_mean = np.mean(arr_with_nan)
print(f"Regular mean: {regular_mean}") # Output: nan
To handle NaN values, NumPy provides np.nanmean()
, which ignores NaN values when computing the mean:
# Using nanmean to ignore NaN values
nan_mean = np.nanmean(arr_with_nan)
print(f"Mean ignoring NaNs: {nan_mean}") # Output: 3.0
Weighted Mean Calculation
Sometimes, not all values in your data should contribute equally to the mean. In such cases, you can use np.average()
to calculate a weighted mean:
# Values
values = np.array([10, 20, 30, 40, 50])
# Weights (importance of each value)
weights = np.array([0.1, 0.2, 0.3, 0.3, 0.1])
# Calculate weighted mean
weighted_mean = np.average(values, weights=weights)
print(f"Weighted mean: {weighted_mean}") # Output: 30.0
# For comparison, the regular mean
regular_mean = np.mean(values)
print(f"Regular mean: {regular_mean}") # Output: 30.0
In this example, the weighted mean gives more importance to the middle values (20, 30, 40) and less to the extremes (10, 50), resulting in a different value than the regular mean.
Performance Considerations
NumPy’s np.mean()
is highly optimized and much faster than Python’s built-in functions for large arrays. Let’s compare the performance:
import time
# Create a large array
large_array = np.random.rand(1000000)
list_version = large_array.tolist()
# Time NumPy's mean
start = time.time()
numpy_mean = np.mean(large_array)
numpy_time = time.time() - start
# Time Python's built-in mean
start = time.time()
python_mean = sum(list_version) / len(list_version)
python_time = time.time() - start
print(f"NumPy mean: {numpy_mean:.6f}, Time: {numpy_time:.6f} seconds")
print(f"Python mean: {python_mean:.6f}, Time: {python_time:.6f} seconds")
print(f"NumPy is {python_time/numpy_time:.1f}x faster")
You’ll typically see that NumPy’s implementation is many times faster than the pure Python approach, especially for large arrays.
Practical Applications
Let’s explore some common applications of np.mean()
in data analysis and machine learning:
Image Processing
In image processing, np.mean()
can be used to calculate the average pixel intensity:
# Assuming we have an image as a 2D numpy array
image = np.array([[50, 60, 70],
[80, 90, 100],
[110, 120, 130]])
# Calculate average pixel value
avg_intensity = np.mean(image)
print(f"Average pixel intensity: {avg_intensity}") # Output: 90.0
# Calculate average intensity per row (useful for row-based analysis)
row_intensities = np.mean(image, axis=1)
print(f"Row intensities: {row_intensities}") # Output: [ 60. 90. 120.]
Feature Normalization in Machine Learning
np.mean()
is often used in feature normalization, a common preprocessing step in machine learning:
# Sample feature data
features = np.array([[1, 2, 3],
[4, 5, 6],
[7, 8, 9]])
# Calculate mean for each feature (column)
feature_means = np.mean(features, axis=0)
print(f"Feature means: {feature_means}") # Output: [4. 5. 6.]
# Normalize features by subtracting the mean (centering)
normalized_features = features - feature_means
print("Normalized features:")
print(normalized_features)
# Output:
# [[-3. -3. -3.]
# [ 0. 0. 0.]
# [ 3. 3. 3.]]
Moving Average Calculation
You can use np.mean()
to implement a simple moving average:
# Time series data
time_series = np.array([10, 12, 15, 18, 20, 22, 25, 28, 30])
# Function to calculate moving average
def moving_average(data, window_size):
result = np.zeros(len(data) - window_size + 1)
for i in range(len(result)):
result[i] = np.mean(data[i:i+window_size])
return result
# Calculate 3-point moving average
ma3 = moving_average(time_series, 3)
print(f"3-point moving average: {ma3}")
# Output: [12.33333333 15. 17.66666667 20. 22.33333333 25. 27.66666667]
Common Mistakes and Best Practices
Empty Arrays
Be careful when calculating the mean of empty arrays:
# Empty array
empty_array = np.array([])
try:
mean_value = np.mean(empty_array)
print(f"Mean of empty array: {mean_value}")
except Exception as e:
print(f"Error: {e}")
NumPy will return NaN for empty arrays, which is different from some other programming languages that might raise errors.
Precision Issues
For very large arrays with small values, precision can be an issue. You can specify a higher precision data type:
# Array with small values
small_values = np.array([1e-10, 2e-10, 3e-10])
# Calculate mean with default precision
default_mean = np.mean(small_values)
print(f"Default precision mean: {default_mean}")
# Calculate mean with higher precision
high_precision_mean = np.mean(small_values, dtype=np.float64)
print(f"High precision mean: {high_precision_mean}")
Axis Parameter Confusion
One common source of confusion is the axis parameter. Remember:
- axis=0 computes the mean along the first axis (down columns)
- axis=1 computes the mean along the second axis (across rows)
- axis=None flattens the array first (default)
It’s always a good practice to check your results with small test cases if you’re unsure about the behavior.
Alternative Mean Calculations in NumPy
Besides np.mean()
, NumPy offers several other functions for calculating various types of means:
# Geometric mean (nth root of the product of all values)
from scipy import stats # Required for geometric mean
geometric_mean = stats.gmean([1, 2, 3, 4, 5])
print(f"Geometric mean: {geometric_mean}") # Output: ~2.61
# Harmonic mean (reciprocal of the arithmetic mean of the reciprocals)
harmonic_mean = stats.hmean([1, 2, 3, 4, 5])
print(f"Harmonic mean: {harmonic_mean}") # Output: ~2.19
# Weighted mean using np.average
weighted_mean = np.average([1, 2, 3, 4, 5], weights=[5, 4, 3, 2, 1])
print(f"Weighted mean: {weighted_mean}") # Output: 2.33...
Conclusion
np.mean()
is a versatile and powerful function for calculating averages in NumPy. Its ability to work with arrays of any dimension, handle different data types, and compute means along specific axes makes it an essential tool for data analysis, scientific computing, and machine learning.
By understanding the various parameters and use cases of np.mean()
, you can effectively analyze your data and extract meaningful insights. Whether you’re preprocessing features for a machine learning model, analyzing experimental results, or simply working with lists of numbers, mastering np.mean()
will enhance your programming toolkit.
Remember that for special cases like handling NaN values or calculating weighted means, NumPy provides specialized functions that build upon the foundation of np.mean()
. As you continue to work with numerical data in Python, you’ll find that the principles learned here apply to many other statistical functions in the NumPy ecosystem.
Happy coding, and may your means be meaningful!