Understanding Network Programming Concepts for Technical Interviews
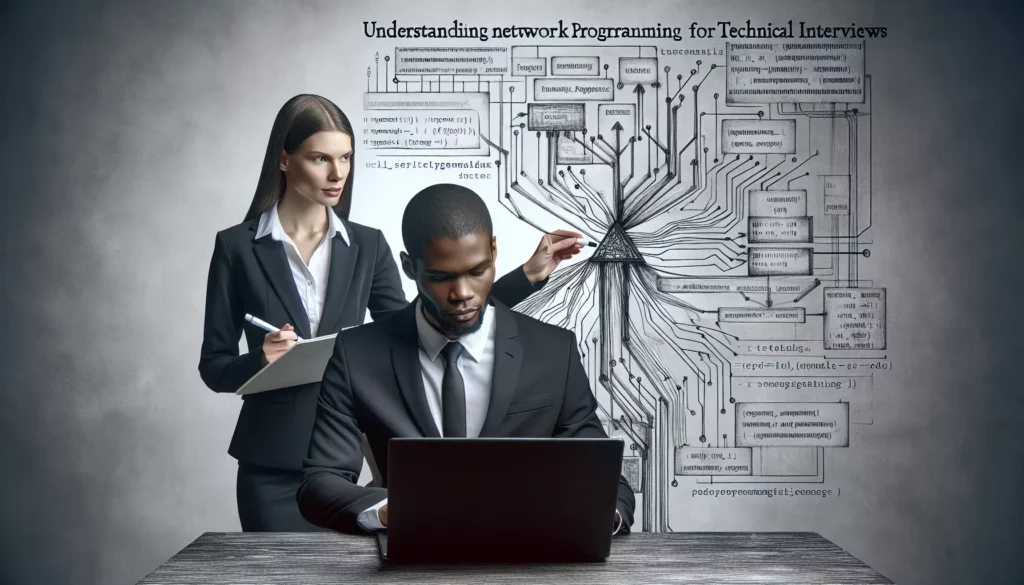
In today’s interconnected world, network programming has become an essential skill for software developers. Whether you’re preparing for a technical interview at a major tech company or looking to enhance your coding skills, understanding network programming concepts is crucial. This comprehensive guide will dive deep into the fundamental principles of network programming, common protocols, and practical implementation techniques that are often discussed in technical interviews.
1. Introduction to Network Programming
Network programming involves writing code that enables communication between different computers or devices over a network. It’s the foundation of many applications we use daily, from web browsers to messaging apps and online gaming platforms. Before we delve into specific concepts, let’s understand why network programming is important:
- Enables distributed computing and resource sharing
- Facilitates real-time communication between devices
- Powers the internet and cloud-based services
- Essential for building scalable and robust applications
2. Key Networking Concepts
2.1 OSI Model
The Open Systems Interconnection (OSI) model is a conceptual framework that describes how data is transmitted between two devices on a network. It consists of seven layers:
- Physical Layer
- Data Link Layer
- Network Layer
- Transport Layer
- Session Layer
- Presentation Layer
- Application Layer
Understanding the OSI model is crucial for grasping how different networking protocols operate and interact with each other.
2.2 TCP/IP Model
The TCP/IP model is a simplified version of the OSI model and is more commonly used in practice. It consists of four layers:
- Network Access Layer
- Internet Layer
- Transport Layer
- Application Layer
Most network programming tasks focus on the Transport and Application layers of the TCP/IP model.
2.3 IP Addresses and Ports
IP addresses are unique identifiers assigned to devices on a network. They come in two versions: IPv4 (e.g., 192.168.0.1) and IPv6 (e.g., 2001:0db8:85a3:0000:0000:8a2e:0370:7334). Ports are numerical identifiers that allow multiple network services to run on a single IP address. Together, IP addresses and ports form the foundation of network communication.
2.4 Sockets
Sockets are the primary interface for network programming. They provide an endpoint for sending and receiving data across a network. In most programming languages, sockets are implemented as objects or file descriptors that represent a network connection.
3. Common Network Protocols
3.1 TCP (Transmission Control Protocol)
TCP is a connection-oriented protocol that provides reliable, ordered, and error-checked delivery of data. It’s widely used for applications that require high reliability, such as web browsing, email, and file transfer. Key features of TCP include:
- Connection establishment through a three-way handshake
- Flow control and congestion control mechanisms
- Retransmission of lost packets
- In-order delivery of data
3.2 UDP (User Datagram Protocol)
UDP is a connectionless protocol that provides a simple, unreliable datagram service. It’s often used for applications that prioritize speed over reliability, such as online gaming, video streaming, and VoIP. Key features of UDP include:
- No connection establishment or termination
- No guarantee of packet delivery or order
- Lower overhead and latency compared to TCP
- Support for broadcast and multicast communication
3.3 HTTP (Hypertext Transfer Protocol)
HTTP is an application-layer protocol used for transmitting hypermedia documents, such as HTML. It forms the foundation of data communication on the World Wide Web. Key concepts related to HTTP include:
- Request-response model
- HTTP methods (GET, POST, PUT, DELETE, etc.)
- Status codes (200 OK, 404 Not Found, etc.)
- Headers and body structure
- RESTful API design principles
3.4 WebSocket
WebSocket is a protocol that enables full-duplex, bidirectional communication between a client and a server over a single TCP connection. It’s commonly used for real-time applications, such as chat systems and live updates. Key features of WebSocket include:
- Persistent connection
- Low latency communication
- Support for both text and binary data
- Cross-origin communication
4. Network Programming in Practice
4.1 Socket Programming
Socket programming is the foundation of network communication in most programming languages. Here’s a simple example of a TCP server and client in Python:
TCP Server:
import socket
server_socket = socket.socket(socket.AF_INET, socket.SOCK_STREAM)
server_socket.bind(('localhost', 8000))
server_socket.listen(1)
print("Server listening on localhost:8000")
while True:
client_socket, address = server_socket.accept()
print(f"Connection from {address}")
data = client_socket.recv(1024).decode()
print(f"Received: {data}")
response = f"Server received: {data}"
client_socket.send(response.encode())
client_socket.close()
TCP Client:
import socket
client_socket = socket.socket(socket.AF_INET, socket.SOCK_STREAM)
client_socket.connect(('localhost', 8000))
message = "Hello, server!"
client_socket.send(message.encode())
response = client_socket.recv(1024).decode()
print(f"Server response: {response}")
client_socket.close()
This example demonstrates the basic flow of socket programming: creating a socket, establishing a connection, sending and receiving data, and closing the connection.
4.2 HTTP Requests
Making HTTP requests is a common task in network programming. Here’s an example using Python’s requests library:
import requests
# GET request
response = requests.get('https://api.example.com/data')
print(f"Status code: {response.status_code}")
print(f"Response content: {response.json()}")
# POST request
data = {'key': 'value'}
response = requests.post('https://api.example.com/create', json=data)
print(f"Status code: {response.status_code}")
print(f"Response content: {response.json()}")
This example shows how to make GET and POST requests to a REST API and handle the responses.
4.3 WebSocket Communication
Here’s a simple example of WebSocket communication using the websockets library in Python:
WebSocket Server:
import asyncio
import websockets
async def echo(websocket, path):
async for message in websocket:
print(f"Received: {message}")
await websocket.send(f"Echo: {message}")
start_server = websockets.serve(echo, "localhost", 8765)
asyncio.get_event_loop().run_until_complete(start_server)
asyncio.get_event_loop().run_forever()
WebSocket Client:
import asyncio
import websockets
async def hello():
uri = "ws://localhost:8765"
async with websockets.connect(uri) as websocket:
await websocket.send("Hello WebSocket!")
response = await websocket.recv()
print(f"Received: {response}")
asyncio.get_event_loop().run_until_complete(hello())
This example demonstrates how to set up a WebSocket server that echoes messages back to clients, and a client that sends a message and receives the response.
5. Advanced Network Programming Concepts
5.1 Asynchronous I/O
Asynchronous I/O is a programming pattern that allows handling multiple network connections concurrently without using multiple threads. It’s particularly useful for building scalable network applications. Here’s an example using Python’s asyncio library:
import asyncio
async def handle_client(reader, writer):
data = await reader.read(100)
message = data.decode()
addr = writer.get_extra_info('peername')
print(f"Received {message} from {addr}")
response = f"Echo: {message}"
writer.write(response.encode())
await writer.drain()
writer.close()
async def main():
server = await asyncio.start_server(
handle_client, '127.0.0.1', 8888)
addr = server.sockets[0].getsockname()
print(f'Serving on {addr}')
async with server:
await server.serve_forever()
asyncio.run(main())
This example shows an asynchronous TCP server that can handle multiple clients concurrently using a single thread.
5.2 Non-blocking I/O
Non-blocking I/O allows a program to continue execution while waiting for I/O operations to complete. It’s often used in conjunction with event loops to handle multiple connections efficiently. Here’s an example using Python’s selectors module:
import selectors
import socket
selector = selectors.DefaultSelector()
def accept(sock, mask):
conn, addr = sock.accept()
print(f"Connection from {addr}")
conn.setblocking(False)
selector.register(conn, selectors.EVENT_READ, read)
def read(conn, mask):
data = conn.recv(1000)
if data:
print(f"Received: {data.decode()}")
conn.send(f"Echo: {data.decode()}".encode())
else:
print("Closing connection")
selector.unregister(conn)
conn.close()
sock = socket.socket()
sock.bind(('localhost', 1234))
sock.listen(100)
sock.setblocking(False)
selector.register(sock, selectors.EVENT_READ, accept)
while True:
events = selector.select()
for key, mask in events:
callback = key.data
callback(key.fileobj, mask)
This example demonstrates a non-blocking server that can handle multiple connections using an event loop and callbacks.
5.3 Network Security
Network security is a crucial aspect of network programming. Some important security concepts to consider include:
- SSL/TLS encryption for secure communication
- Authentication and authorization mechanisms
- Input validation and sanitization to prevent injection attacks
- Firewalls and network segmentation
- Secure protocols (HTTPS, SFTP, etc.)
Here’s an example of creating an HTTPS server in Python using the ssl module:
import http.server
import ssl
httpd = http.server.HTTPServer(('localhost', 4443), http.server.SimpleHTTPRequestHandler)
ssl_context = ssl.SSLContext(ssl.PROTOCOL_TLS_SERVER)
ssl_context.load_cert_chain(certfile='server.crt', keyfile='server.key')
httpd.socket = ssl_context.wrap_socket(httpd.socket, server_side=True)
print("Server running on https://localhost:4443")
httpd.serve_forever()
This example sets up a simple HTTPS server using a self-signed certificate. In a production environment, you would use a certificate from a trusted Certificate Authority.
6. Common Interview Questions and Coding Challenges
When preparing for technical interviews that focus on network programming, it’s essential to be familiar with common questions and coding challenges. Here are some examples:
6.1 Conceptual Questions
- Explain the difference between TCP and UDP. When would you use one over the other?
- What is the purpose of the three-way handshake in TCP?
- How does DNS resolution work?
- Explain the concept of port forwarding.
- What is the difference between IPv4 and IPv6?
- How does a load balancer work?
- Explain the concept of network address translation (NAT).
- What is the difference between a proxy server and a reverse proxy?
6.2 Coding Challenges
- Implement a simple HTTP server that can handle GET and POST requests.
- Create a chat application using WebSockets.
- Write a program that scans open ports on a given IP address.
- Implement a basic DNS resolver.
- Create a file transfer program using TCP sockets.
- Implement a simple load balancer that distributes requests across multiple servers.
- Write a program that simulates network packet loss and retransmission.
- Implement a basic network protocol for a specific use case (e.g., a distributed key-value store).
7. Best Practices and Tips for Network Programming
To excel in network programming and perform well in technical interviews, consider the following best practices and tips:
- Error Handling: Always implement proper error handling and logging in your network code. Network operations are prone to various issues, such as connection timeouts, dropped packets, and unexpected disconnections.
- Security: Prioritize security in your network applications. Use encryption, implement proper authentication and authorization, and follow secure coding practices to prevent common vulnerabilities.
- Performance Optimization: Be mindful of performance when writing network code. Use appropriate data structures, implement caching where necessary, and consider techniques like connection pooling for database-driven applications.
- Scalability: Design your network applications with scalability in mind. Use asynchronous programming techniques, implement proper load balancing, and consider distributed architectures for large-scale systems.
- Testing: Thoroughly test your network code, including edge cases and error scenarios. Implement unit tests, integration tests, and load tests to ensure your application behaves correctly under various conditions.
- Documentation: Write clear and comprehensive documentation for your network code, including API references, usage examples, and deployment instructions.
- Stay Updated: Keep up with the latest developments in network protocols, security best practices, and performance optimization techniques.
- Practice: Regularly work on network programming projects and coding challenges to hone your skills and prepare for technical interviews.
Conclusion
Network programming is a vast and complex field that plays a crucial role in modern software development. By understanding the fundamental concepts, mastering common protocols, and gaining hands-on experience with practical implementations, you’ll be well-prepared for technical interviews and real-world network programming challenges.
Remember that network programming is not just about writing code; it’s about understanding the underlying principles of computer networks and how different components interact. As you continue to learn and practice, focus on building a strong foundation in networking concepts, and always consider security, performance, and scalability in your implementations.
With dedication and consistent practice, you’ll be well-equipped to tackle network programming questions in technical interviews and contribute to building robust, scalable, and efficient networked applications in your future career.