Understanding MVC: A Comprehensive Guide to the Model-View-Controller Architecture
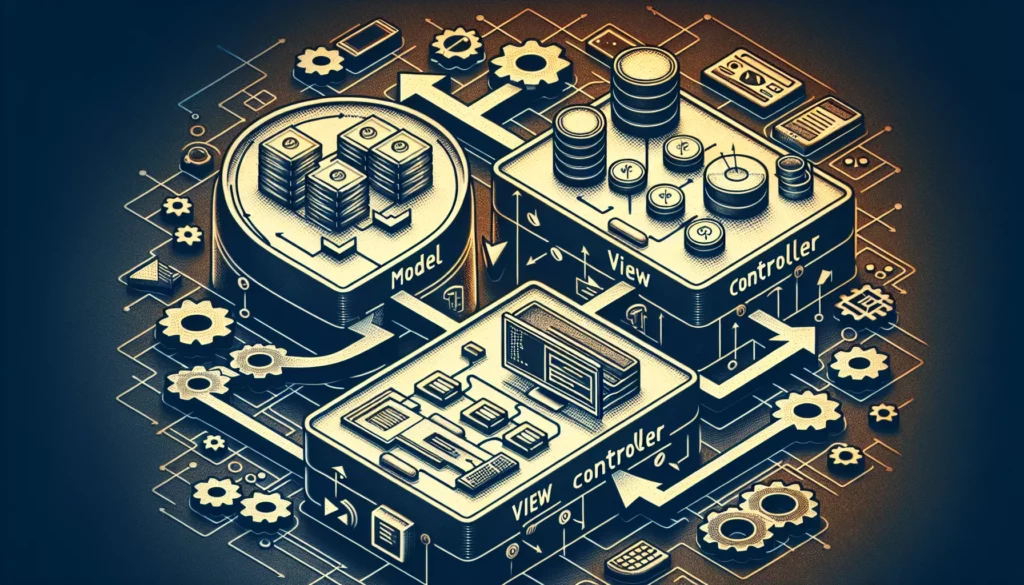
In the world of software development, architectural patterns play a crucial role in organizing code and creating maintainable applications. One of the most widely used and influential patterns is the Model-View-Controller (MVC) architecture. Whether you’re a beginner programmer or an experienced developer looking to refine your skills, understanding MVC is essential for building robust and scalable applications.
In this comprehensive guide, we’ll dive deep into the MVC pattern, exploring its components, benefits, and practical applications. By the end of this article, you’ll have a solid grasp of MVC and be well-equipped to implement it in your own projects.
Table of Contents
- What is MVC?
- Components of MVC
- How MVC Works
- Benefits of MVC
- MVC vs. Other Architectural Patterns
- Implementing MVC in Different Programming Languages
- Best Practices for Using MVC
- Common Challenges and Solutions in MVC
- Real-World Examples of MVC Applications
- The Future of MVC
1. What is MVC?
Model-View-Controller (MVC) is an architectural pattern that separates an application into three main logical components: the Model, the View, and the Controller. Each of these components is built to handle specific development aspects of an application. MVC is one of the most frequently used industry-standard web development frameworks to create scalable and extensible projects.
The concept of MVC was introduced by Trygve Reenskaug in 1979 while working on Smalltalk at Xerox PARC. Since then, it has evolved and been adapted to various programming languages and frameworks, becoming a cornerstone of modern web development.
2. Components of MVC
Let’s break down the three main components of the MVC architecture:
Model
The Model represents the data and business logic of the application. It is responsible for managing the data, logic, and rules of the application. Some key points about the Model:
- It is independent of the user interface.
- It directly manages the data, logic, and rules of the application.
- It can have multiple views.
- When a model changes, it typically notifies its observers (usually views) that a change has occurred.
View
The View is responsible for presenting the data to the user. It defines how the application’s data should be displayed. Key aspects of the View include:
- It represents the visualization of the data that the model contains.
- It is responsible for presenting data to the user in various formats and layouts.
- Multiple views can exist for a single model for different purposes.
- A view can be any output representation of data, such as a chart, diagram, or table.
Controller
The Controller acts as an intermediary between the Model and the View. It receives user input and makes calls to model objects and the view to perform appropriate actions. The Controller:
- Accepts input from the user and instructs the model and view to perform actions based on that input.
- Sends commands to the model to update its state.
- Sends commands to the view to change the presentation of the model.
- Is often described as the “glue” between the model and view.
3. How MVC Works
The MVC pattern follows a cycle of interactions:
- The user interacts with the View (e.g., clicking a button or submitting a form).
- The View notifies the Controller about the user’s action.
- The Controller processes the user’s request, which may involve updating the Model.
- If the Model is updated, it notifies the View of the changes.
- The View requests the updated data from the Model and refreshes itself.
- The updated View is presented to the user.
This cycle ensures a clear separation of concerns and allows for efficient data flow and user interaction handling.
4. Benefits of MVC
The MVC architecture offers several advantages that make it a popular choice among developers:
Separation of Concerns
MVC separates the application logic into three distinct components, making the code more organized and easier to maintain. This separation allows developers to work on different parts of the application independently.
Reusability
Because the components are separate, they can be reused in other projects or parts of the same project. For example, a Model can be used with different Views, or a View can be used with different Controllers.
Scalability
The modular nature of MVC makes it easier to scale applications. New features can be added by creating new Models, Views, and Controllers without significantly impacting existing code.
Easier Collaboration
MVC allows different team members to work on different components simultaneously. For example, a front-end developer can work on the View while a back-end developer works on the Model.
Better Code Organization
MVC provides a clear structure for the application, making it easier for developers to understand and navigate the codebase.
Faster Development Process
With clear separations and defined roles for each component, developers can work more efficiently, leading to faster development cycles.
5. MVC vs. Other Architectural Patterns
While MVC is widely used, it’s not the only architectural pattern available. Let’s compare MVC with some other popular patterns:
MVC vs. MVP (Model-View-Presenter)
MVP is similar to MVC, but the Presenter assumes some of the responsibilities of the Controller. In MVP, the Presenter contains the UI business logic for the View and is responsible for updating the View with data from the Model.
MVC vs. MVVM (Model-View-ViewModel)
MVVM introduces a ViewModel, which acts as a binder between the View and the Model. The ViewModel exposes data and commands that the View can bind to, providing a more robust separation between the View and the Model.
MVC vs. Flux
Flux, developed by Facebook, is an application architecture for building client-side web applications. Unlike MVC, Flux enforces unidirectional data flow, which can make it easier to reason about state changes in complex applications.
6. Implementing MVC in Different Programming Languages
MVC can be implemented in various programming languages and frameworks. Let’s look at some examples:
MVC in Java (Spring Framework)
Spring MVC is a popular framework for building web applications in Java. Here’s a basic example of how MVC components might look in a Spring application:
Model:
public class User {
private String name;
private String email;
// Getters and setters
}
Controller:
@Controller
public class UserController {
@GetMapping("/user")
public String getUser(Model model) {
User user = new User();
user.setName("John Doe");
user.setEmail("john@example.com");
model.addAttribute("user", user);
return "user-view";
}
}
View (user-view.html):
<!DOCTYPE html>
<html xmlns:th="http://www.thymeleaf.org">
<head>
<title>User Details</title>
</head>
<body>
<h1>User Details</h1>
<p>Name: <span th:text="${user.name}"></span></p>
<p>Email: <span th:text="${user.email}"></span></p>
</body>
</html>
MVC in Python (Django)
Django follows the MVT (Model-View-Template) pattern, which is similar to MVC. Here’s a basic example:
Model (models.py):
from django.db import models
class User(models.Model):
name = models.CharField(max_length=100)
email = models.EmailField()
View (views.py):
from django.shortcuts import render
from .models import User
def user_detail(request):
user = User.objects.get(id=1)
return render(request, 'user_detail.html', {'user': user})
Template (user_detail.html):
<!DOCTYPE html>
<html>
<head>
<title>User Details</title>
</head>
<body>
<h1>User Details</h1>
<p>Name: {{ user.name }}</p>
<p>Email: {{ user.email }}</p>
</body>
</html>
MVC in JavaScript (Express.js)
While Express.js doesn’t enforce MVC out of the box, you can structure your application to follow the MVC pattern:
Model (user.js):
class User {
constructor(name, email) {
this.name = name;
this.email = email;
}
static getUser() {
return new User('John Doe', 'john@example.com');
}
}
module.exports = User;
Controller (userController.js):
const User = require('../models/user');
exports.getUser = (req, res) => {
const user = User.getUser();
res.render('user', { user });
};
View (user.ejs):
<!DOCTYPE html>
<html>
<head>
<title>User Details</title>
</head>
<body>
<h1>User Details</h1>
<p>Name: <%= user.name %></p>
<p>Email: <%= user.email %></p>
</body>
</html>
7. Best Practices for Using MVC
To make the most of the MVC pattern, consider the following best practices:
Keep Components Separate
Maintain a clear separation between Models, Views, and Controllers. Avoid putting business logic in Views or data access in Controllers.
Use Thin Controllers
Controllers should be as thin as possible, delegating complex operations to the Model or service layers. This makes controllers easier to test and maintain.
Make Models Rich
Models should contain the bulk of the business logic. This ensures that the logic is reusable across different parts of the application.
Keep Views Simple
Views should be responsible only for displaying data. Avoid putting complex logic or data manipulation in views.
Use View Models
For complex views, consider using view models to prepare data specifically for that view. This can help keep your models focused on business logic rather than presentation concerns.
Implement Proper Error Handling
Implement robust error handling in your controllers to manage exceptions and provide meaningful feedback to users.
Use Dependency Injection
Use dependency injection to decouple your components and make your code more testable and maintainable.
8. Common Challenges and Solutions in MVC
While MVC offers many benefits, it also comes with its own set of challenges. Here are some common issues and their solutions:
Challenge: Tight Coupling
Solution: Use interfaces and dependency injection to reduce coupling between components. This allows for easier testing and maintenance.
Challenge: Fat Controllers
Solution: Move business logic to the Model or create service layers. Keep controllers focused on handling requests and delegating work.
Challenge: Complex Views
Solution: Use view models or presenters to prepare data for complex views. This keeps your views simple and your models focused on business logic.
Challenge: Handling AJAX Requests
Solution: Create separate API controllers or endpoints for handling AJAX requests. This keeps your regular controllers clean and focused on serving full page requests.
Challenge: Managing State
Solution: Use a state management library (like Redux for JavaScript applications) to manage complex application states outside of the MVC pattern.
9. Real-World Examples of MVC Applications
Many popular web applications and frameworks use the MVC pattern. Here are a few examples:
Ruby on Rails
Ruby on Rails is a web application framework that follows the MVC pattern. It’s known for its convention over configuration approach, which makes it easy to build web applications quickly.
ASP.NET MVC
Microsoft’s ASP.NET MVC is a web application framework that implements the MVC pattern for building dynamic websites with .NET and C#.
Laravel (PHP)
Laravel is a PHP web application framework that follows the MVC architectural pattern. It’s known for its elegant syntax and powerful features.
Express.js with MVC Structure
While Express.js doesn’t enforce MVC, many developers structure their Express applications using the MVC pattern for better organization and maintainability.
10. The Future of MVC
As web development continues to evolve, so does the MVC pattern. Here are some trends and potential future directions for MVC:
Microservices Architecture
As applications grow more complex, there’s a trend towards breaking them down into microservices. MVC principles can still apply within each microservice, but the overall architecture may become more distributed.
Serverless Architecture
Serverless computing is gaining popularity. While it doesn’t directly replace MVC, it may influence how we structure our applications, potentially leading to more emphasis on the Model and View components with less need for traditional Controllers.
Component-Based Architectures
Frameworks like React and Vue.js have popularized component-based architectures. These can work alongside MVC, with components often serving as specialized Views.
AI and Machine Learning Integration
As AI and machine learning become more prevalent in web applications, we may see new patterns emerge for integrating these technologies with traditional MVC structures.
Continued Evolution
MVC will likely continue to evolve, with new variations and adaptations emerging to meet the changing needs of web development. However, its core principles of separation of concerns and modular design are likely to remain relevant for years to come.
Conclusion
The Model-View-Controller (MVC) pattern has stood the test of time, proving to be a robust and flexible architecture for building web applications. By separating concerns into distinct components, MVC promotes code organization, reusability, and maintainability.
As you continue your journey in software development, understanding and implementing MVC will be a valuable skill. Whether you’re building a simple web application or a complex enterprise system, the principles of MVC can help you create more organized, scalable, and maintainable code.
Remember, while MVC provides a solid foundation, it’s not a one-size-fits-all solution. As you gain experience, you’ll learn when to strictly adhere to MVC principles and when to adapt them to best suit your project’s needs. The key is to understand the core concepts and apply them thoughtfully to create efficient and effective software solutions.