Understanding Monads in Functional Programming: A Comprehensive Guide
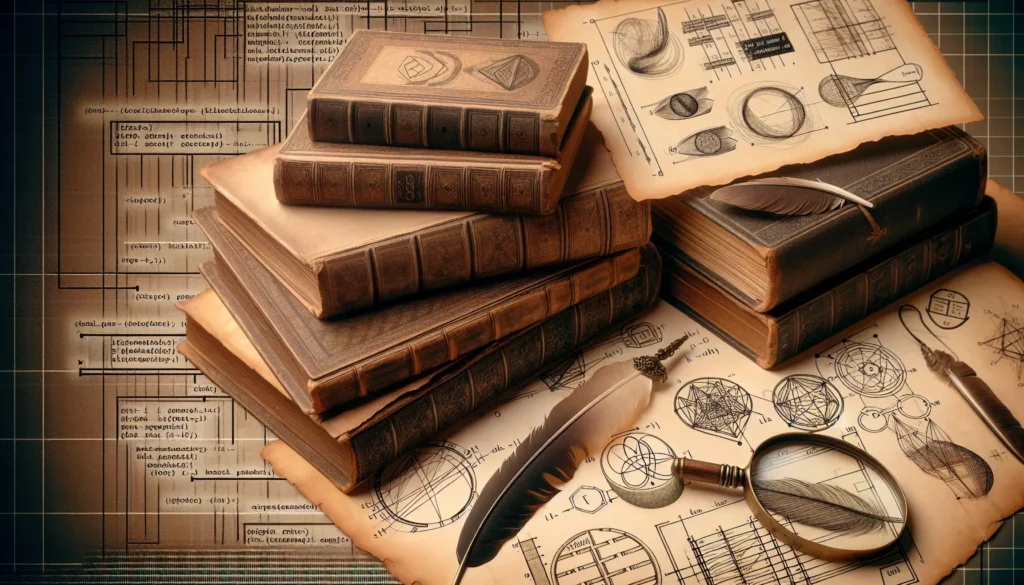
In the world of functional programming, few concepts are as powerful and often misunderstood as monads. Whether you’re a beginner just starting to explore functional programming or an experienced developer looking to deepen your understanding, this comprehensive guide will demystify monads and show you how they can enhance your code. By the end of this article, you’ll have a solid grasp of what monads are, how they work, and why they’re an essential tool in the functional programmer’s toolkit.
Table of Contents
- What Are Monads?
- Monad Laws
- Common Monads and Their Use Cases
- Implementing Monads in Different Languages
- Benefits of Using Monads
- Challenges and Common Misconceptions
- Real-World Examples of Monads in Action
- Advanced Topics in Monads
- Conclusion
1. What Are Monads?
At its core, a monad is a design pattern used in functional programming to handle side effects, manage complexity, and structure programs. It’s a way of wrapping values in a context, along with some specific operations that work with that context. While this definition might sound abstract, monads become more intuitive when we look at concrete examples.
To understand monads, let’s break them down into three key components:
- Type Constructor: This is a way to create a new type from an existing type. For example,
Maybe a
is a type constructor that creates a new type from the typea
. - Return Function: Also known as “unit” or “pure”, this function wraps a value in the monad’s context. For example,
Just 5
wraps the value 5 in the Maybe monad. - Bind Operation: This is a way to chain operations on monadic values. It’s often represented by the
>>=
operator in languages like Haskell.
Let’s look at a simple example using the Maybe monad in Haskell-like pseudocode:
-- Define a simple function that divides two numbers
safeDivide :: Double -> Double -> Maybe Double
safeDivide _ 0 = Nothing -- Return Nothing if dividing by zero
safeDivide x y = Just (x / y)
-- Use the Maybe monad to chain operations
result = Just 10 >>= (\x -> safeDivide x 2) >>= (\y -> safeDivide y 0)
-- result will be Nothing due to division by zero
In this example, the Maybe monad allows us to chain operations that might fail (division by zero) without explicitly checking for errors at each step. The bind operation (>>=
) takes care of propagating the Nothing value if any operation fails.
2. Monad Laws
For a type to be considered a monad, it must satisfy three laws. These laws ensure that monads behave consistently and predictably:
- Left Identity: return a >>= f ≡ f a
- Right Identity: m >>= return ≡ m
- Associativity: (m >>= f) >>= g ≡ m >>= (\x -> f x >>= g)
Let’s break these down:
Left Identity
This law states that if we take a value, wrap it in a monad using return
, and then feed it to a function f
using bind, it’s the same as just applying f
to the value directly.
-- Left Identity Example
return 5 >>= (\x -> Just (x * 2)) -- This should be equivalent to:
Just 10 -- The result of (\x -> Just (x * 2)) 5
Right Identity
This law says that if we have a monadic value and we bind it with the return
function, we should get back the original monadic value.
-- Right Identity Example
Just 5 >>= return -- This should be equivalent to:
Just 5 -- The original value
Associativity
This law ensures that the order of nesting when chaining monadic operations doesn’t matter.
-- Associativity Example
(Just 5 >>= (\x -> Just (x * 2))) >>= (\y -> Just (y + 1))
-- Should be equivalent to:
Just 5 >>= (\x -> (Just (x * 2) >>= (\y -> Just (y + 1))))
-- Both should result in:
Just 11
These laws might seem abstract, but they’re crucial for ensuring that monads behave consistently, allowing developers to reason about and compose monadic operations with confidence.
3. Common Monads and Their Use Cases
While there are many types of monads, some are more commonly used than others. Let’s explore a few of the most prevalent monads and their typical use cases:
Maybe Monad
The Maybe monad (also known as the Option monad in some languages) is used to handle computations that might not produce a value.
- Type:
Maybe a = Just a | Nothing
- Use Case: Handling potential null values or computations that might fail
findUser :: UserId -> Maybe User
updateEmail :: User -> Email -> Maybe User
result = findUser 123 >>= (\user -> updateEmail user "new@email.com")
List Monad
The List monad is used to represent computations with multiple possible results.
- Type:
[a]
(Built-in list type in many languages) - Use Case: Non-deterministic computations, generating combinations
-- Generate all possible pairs of elements from two lists
pairs = [1, 2, 3] >>= (\x -> ['a', 'b'] >>= (\y -> return (x, y)))
-- Result: [(1,'a'), (1,'b'), (2,'a'), (2,'b'), (3,'a'), (3,'b')]
IO Monad
The IO monad is used to handle input/output operations and other side effects in a pure functional context.
- Type:
IO a
- Use Case: Managing side effects, interacting with the outside world
main :: IO ()
main = do
putStrLn "Enter your name:"
name <- getLine
putStrLn ("Hello, " ++ name ++ "!")
State Monad
The State monad is used to carry state through a computation without explicitly passing it as a parameter.
- Type:
State s a
- Use Case: Managing mutable state in a functional way
import Control.Monad.State
type Stack = [Int]
pop :: State Stack Int
pop = do
(x:xs) <- get
put xs
return x
push :: Int -> State Stack ()
push x = do
xs <- get
put (x:xs)
-- Using the stack
stackManip :: State Stack Int
stackManip = do
push 3
push 5
pop
pop
-- Running the computation
runState stackManip [] -- Returns (3, [])
Reader Monad
The Reader monad is used to pass a shared environment to a series of computations.
- Type:
Reader r a
- Use Case: Dependency injection, configuration management
import Control.Monad.Reader
type Env = [(String, Int)]
lookupVar :: String -> Reader Env (Maybe Int)
lookupVar var = do
env <- ask
return (lookup var env)
computation :: Reader Env (Maybe Int)
computation = do
x <- lookupVar "x"
y <- lookupVar "y"
return $ (+) <$> x <*> y
-- Running the computation
runReader computation [('x', 10), ('y', 20)] -- Returns Just 30
These are just a few examples of common monads. Each serves a specific purpose and can greatly simplify code when used appropriately. As you become more comfortable with monads, you’ll start to recognize patterns in your code where they can be applied effectively.
4. Implementing Monads in Different Languages
While monads are most commonly associated with purely functional languages like Haskell, they can be implemented and used in many programming languages. Let’s look at how monads can be implemented in a few different languages:
Haskell
Haskell is the language where monads are most prevalent. Here’s how the Maybe monad is defined in Haskell:
data Maybe a = Nothing | Just a
instance Monad Maybe where
return = Just
Nothing >>= _ = Nothing
(Just x) >>= f = f x
Scala
Scala, being a hybrid functional-object-oriented language, has good support for monads. Here’s an implementation of the Maybe monad (called Option in Scala):
sealed trait Option[+A] {
def flatMap[B](f: A => Option[B]): Option[B] = this match {
case Some(x) => f(x)
case None => None
}
}
case class Some[+A](get: A) extends Option[A]
case object None extends Option[Nothing]
// Usage
val result = Some(3).flatMap(x => Some(x * 2)) // Some(6)
JavaScript
While JavaScript doesn’t have native support for monads, we can implement them using classes:
class Maybe {
constructor(value) {
this.value = value;
}
static of(value) {
return new Maybe(value);
}
isNothing() {
return this.value === null || this.value === undefined;
}
map(fn) {
return this.isNothing() ? this : Maybe.of(fn(this.value));
}
flatMap(fn) {
return this.isNothing() ? this : fn(this.value);
}
}
// Usage
const result = Maybe.of(3)
.flatMap(x => Maybe.of(x * 2))
.flatMap(x => Maybe.of(x + 1));
console.log(result); // Maybe { value: 7 }
Python
Python can implement monads using classes as well. Here’s a simple Maybe monad:
class Maybe:
def __init__(self, value):
self.value = value
@classmethod
def of(cls, value):
return cls(value)
def is_nothing(self):
return self.value is None
def map(self, fn):
return self if self.is_nothing() else Maybe.of(fn(self.value))
def flat_map(self, fn):
return self if self.is_nothing() else fn(self.value)
# Usage
result = Maybe.of(3) \
.flat_map(lambda x: Maybe.of(x * 2)) \
.flat_map(lambda x: Maybe.of(x + 1))
print(result.value) # 7
These implementations demonstrate that monads can be used in various programming languages, not just those traditionally associated with functional programming. While the syntax and exact implementation details may vary, the core concepts remain the same.
5. Benefits of Using Monads
Monads offer several significant benefits that can greatly improve code quality and maintainability:
Abstraction of Complex Operations
Monads provide a high level of abstraction for complex operations. They allow you to focus on the core logic of your computation without getting bogged down in the details of how that computation is managed.
Separation of Concerns
By encapsulating specific behaviors (like error handling or state management) within a monad, you can separate these concerns from your main business logic. This leads to cleaner, more modular code.
Composability
Monads are highly composable. You can chain monadic operations together to create complex computations from simpler ones. This composability is at the heart of functional programming and can lead to more reusable code.
Error Handling
Monads like Maybe or Either provide elegant ways to handle errors and exceptional cases without resorting to try-catch blocks or null checks throughout your code.
Side Effect Management
In purely functional languages, monads (like the IO monad) provide a way to handle side effects while maintaining referential transparency. This allows for easier reasoning about code behavior.
Code Consistency
Once you’re familiar with monads, they provide a consistent pattern for dealing with various programming challenges. This consistency can make code easier to understand and maintain.
6. Challenges and Common Misconceptions
While monads are powerful, they come with their own set of challenges and misconceptions:
Learning Curve
Monads can be difficult to understand initially, especially for developers coming from an imperative programming background. The abstract nature of monads and the functional programming concepts they rely on can take time to grasp fully.
Overuse
Sometimes developers, excited by the power of monads, try to use them everywhere. This can lead to unnecessarily complex code when simpler solutions would suffice.
“Monads are like burritos”
This common analogy, while well-intentioned, often leads to more confusion. Monads are a mathematical concept and are best understood in terms of their properties and use cases, not through strained analogies.
Performance Concerns
In some cases, excessive use of monads can lead to performance issues, especially in languages where they’re not natively supported. It’s important to profile your code and use monads judiciously.
Stacktrace Issues
In some implementations, extensive use of monads can lead to less helpful stacktraces when errors occur, making debugging more challenging.
7. Real-World Examples of Monads in Action
To better understand how monads are used in practice, let’s look at some real-world examples:
Database Queries with the Maybe Monad
Consider a scenario where you’re querying a database for user information:
getUserInfo :: UserId -> Maybe UserInfo
getAddress :: UserInfo -> Maybe Address
getZipCode :: Address -> Maybe ZipCode
getUserZipCode :: UserId -> Maybe ZipCode
getUserZipCode userId = do
userInfo <- getUserInfo userId
address <- getAddress userInfo
zipCode <- getZipCode address
return zipCode
Here, the Maybe monad allows us to chain these operations that might fail (e.g., if the user doesn’t exist or doesn’t have an address) without explicit null checks.
Asynchronous Operations with the Promise Monad
In JavaScript, Promises can be seen as a monad for handling asynchronous operations:
const fetchUserData = (userId) =>
fetch(`/api/users/${userId}`)
.then(response => response.json())
.then(user => fetch(`/api/posts?userId=${user.id}`))
.then(response => response.json())
.then(posts => ({user, posts}))
.catch(error => console.error('Error:', error));
fetchUserData(123).then(data => console.log(data));
The Promise monad allows us to chain asynchronous operations and handle errors in a clean, composable way.
State Management with the State Monad
The State monad can be useful for managing complex state in games or simulations:
import Control.Monad.State
type GameState = (Int, Int, Int) -- (score, level, lives)
increaseScore :: Int -> State GameState ()
increaseScore points = modify (\(s, l, v) -> (s + points, l, v))
levelUp :: State GameState ()
levelUp = modify (\(s, l, v) -> (s, l + 1, v))
loseLife :: State GameState ()
loseLife = modify (\(s, l, v) -> (s, l, v - 1))
playGame :: State GameState ()
playGame = do
increaseScore 100
levelUp
increaseScore 200
loseLife
increaseScore 300
initialState = (0, 1, 3)
finalState = execState playGame initialState
-- finalState will be (600, 2, 2)
This example shows how the State monad can manage complex state changes without explicitly passing state around.
8. Advanced Topics in Monads
As you become more comfortable with monads, there are several advanced topics you might want to explore:
Monad Transformers
Monad transformers allow you to combine multiple monads to create a new monad with the features of all of them. This is useful when you need the functionality of multiple monads in a single computation.
import Control.Monad.Trans.Maybe
import Control.Monad.Trans.State
type MyMonad = MaybeT (State Int)
computation :: MyMonad String
computation = do
count <- lift get
guard (count > 0)
lift $ put (count - 1)
return "Success"
runMyMonad :: MyMonad a -> Int -> (Maybe a, Int)
runMyMonad m s = runState (runMaybeT m) s
-- Usage
runMyMonad computation 1 -- (Just "Success", 0)
runMyMonad computation 0 -- (Nothing, 0)
Free Monads
Free monads are a way of building monads from any functor. They’re often used to create embedded domain-specific languages (EDSLs) and to separate the description of a computation from its interpreter.
{-# LANGUAGE DeriveFunctor #-}
import Control.Monad.Free
data TeletypeF a = PutStrLn String a | GetLine (String -> a) deriving Functor
type Teletype = Free TeletypeF
putStrLn' :: String -> Teletype ()
putStrLn' s = liftF $ PutStrLn s ()
getLine' :: Teletype String
getLine' = liftF $ GetLine id
program :: Teletype ()
program = do
putStrLn' "What's your name?"
name <- getLine'
putStrLn' $ "Hello, " ++ name ++ "!"
-- Interpreter
interpret :: Teletype a -> IO a
interpret (Pure r) = return r
interpret (Free (PutStrLn s next)) = putStrLn s >> interpret next
interpret (Free (GetLine f)) = getLine >>= (interpret . f)
-- Run the program
main = interpret program
Applicative Functors and Arrows
While not strictly about monads, understanding Applicative Functors and Arrows can broaden your understanding of functional programming patterns and how they relate to monads.
-- Applicative example
data User = User { name :: String, age :: Int }
userInfo :: Maybe String -> Maybe Int -> Maybe User
userInfo name age = User <$> name <*> age
-- Arrow example
import Control.Arrow
processUser :: (String -> String) -> (Int -> Int) -> User -> User
processUser nameFunc ageFunc = name *** age >>> arr (uncurry User)
where
name = nameFunc *** id
age = id *** ageFunc
Category Theory
For those interested in the mathematical foundations of monads, studying category theory can provide deeper insights. Monads are a concept from category theory that has been adapted for use in functional programming.
9. Conclusion
Monads are a powerful and flexible tool in the functional programmer’s toolkit. They provide a way to structure computations, handle side effects, and manage complexity in a clean and composable manner. While they can be challenging to grasp initially, understanding monads can significantly improve your ability to write clear, maintainable, and robust code.
As you continue your journey in functional programming, remember that monads are just one of many concepts you’ll encounter. Don’t get too caught up in trying to understand them perfectly right away. Instead, focus on practical applications and gradually build your intuition through experience.
Whether you’re using Haskell, Scala, JavaScript, or any other language, the principles of monads can be applied to improve your code. As you become more comfortable with monads, you’ll start to see opportunities to use them to simplify complex operations, manage state, handle errors, and more.
Keep exploring, keep coding, and most importantly, enjoy the process of learning and growing as a programmer. The world of functional programming has much to offer, and monads are just the beginning of an exciting journey into more expressive and powerful ways of writing software.