Understanding Microcontrollers and Electronics: Programming Arduino and Raspberry Pi for Hardware Projects
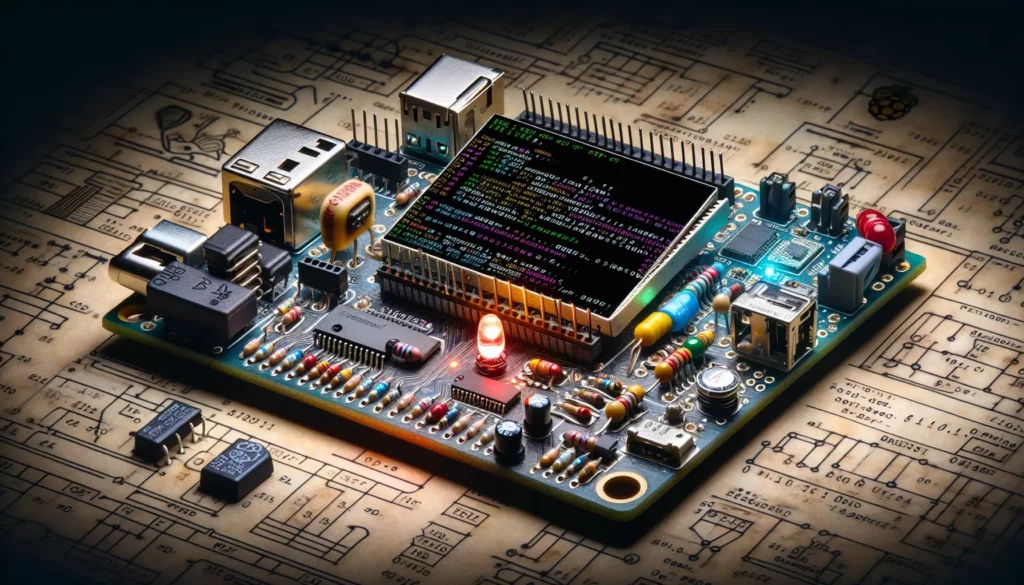
In the ever-evolving world of technology, the ability to program microcontrollers and work with electronics has become an increasingly valuable skill. Whether you’re a coding enthusiast, a budding engineer, or simply curious about how devices work, understanding microcontrollers and electronics can open up a whole new world of possibilities. In this comprehensive guide, we’ll explore the fascinating realm of microcontrollers, with a special focus on two popular platforms: Arduino and Raspberry Pi. We’ll delve into their capabilities, differences, and how you can use them to bring your hardware projects to life.
What Are Microcontrollers?
Before we dive into specific platforms, let’s start with the basics. A microcontroller is a small computer on a single integrated circuit. It contains a processor, memory, and programmable input/output peripherals. Microcontrollers are designed for embedded applications and are found in a wide range of devices, from simple toys to complex industrial machinery.
Key features of microcontrollers include:
- Low power consumption
- Compact size
- Integrated peripherals (timers, ADCs, DACs, etc.)
- Real-time capabilities
- Relatively low cost
Microcontrollers are the brains behind many “smart” devices and are essential components in the Internet of Things (IoT) ecosystem. They allow developers to create interactive, responsive hardware projects that can sense and react to their environment.
Introduction to Arduino
Arduino is an open-source electronics platform based on easy-to-use hardware and software. It’s designed for anyone making interactive projects, from hobbyists to professionals. Arduino boards can read inputs – light on a sensor, a finger on a button, or a Twitter message – and turn it into an output – activating a motor, turning on an LED, publishing something online.
Key Features of Arduino:
- Easy to learn and use, especially for beginners
- Cross-platform (runs on Windows, macOS, and Linux)
- Inexpensive compared to other microcontroller platforms
- Open-source and extensible software and hardware
- Large community and extensive documentation
Getting Started with Arduino
To begin working with Arduino, you’ll need:
- An Arduino board (e.g., Arduino Uno, Arduino Nano)
- The Arduino IDE (Integrated Development Environment)
- A USB cable to connect the board to your computer
- Basic electronic components (LEDs, resistors, breadboard, etc.)
Once you have these items, follow these steps:
- Download and install the Arduino IDE from the official website.
- Connect your Arduino board to your computer using the USB cable.
- Open the Arduino IDE and select your board type and port from the Tools menu.
- Write your code (called a “sketch” in Arduino terminology) or open an example sketch.
- Click the “Upload” button to compile and upload your code to the board.
Arduino Programming Basics
Arduino uses a simplified version of C++. Here’s a basic example of an Arduino sketch that blinks an LED:
const int ledPin = 13; // LED connected to digital pin 13
void setup() {
pinMode(ledPin, OUTPUT); // Set the pin as an output
}
void loop() {
digitalWrite(ledPin, HIGH); // Turn on the LED
delay(1000); // Wait for 1 second
digitalWrite(ledPin, LOW); // Turn off the LED
delay(1000); // Wait for 1 second
}
This sketch demonstrates the two essential functions in every Arduino program:
setup()
: Runs once when the board starts up or is resetloop()
: Runs continuously aftersetup()
completes
Introduction to Raspberry Pi
While Arduino is a microcontroller board, Raspberry Pi is a single-board computer. It’s a more powerful and versatile device that can run a full operating system, typically a version of Linux. Raspberry Pi was originally designed for education, but it has found its way into countless projects due to its capabilities and low cost.
Key Features of Raspberry Pi:
- Runs a full operating system (typically Raspberry Pi OS, based on Debian Linux)
- More powerful processor and more memory than typical microcontrollers
- Built-in Wi-Fi and Bluetooth on most models
- HDMI output for connecting to displays
- USB ports for connecting peripherals
- GPIO pins for interfacing with electronics
Getting Started with Raspberry Pi
To begin working with Raspberry Pi, you’ll need:
- A Raspberry Pi board (e.g., Raspberry Pi 4, Raspberry Pi Zero)
- A microSD card (8GB or larger) for the operating system
- A power supply
- A keyboard, mouse, and monitor (for initial setup)
- Optional: a case for the Raspberry Pi
To set up your Raspberry Pi:
- Download the Raspberry Pi Imager from the official website.
- Use the Imager to write the Raspberry Pi OS to your microSD card.
- Insert the microSD card into your Raspberry Pi.
- Connect the keyboard, mouse, and monitor.
- Power on the Raspberry Pi and follow the initial setup instructions.
Raspberry Pi Programming
Unlike Arduino, Raspberry Pi allows you to program in various languages. Python is particularly popular due to its simplicity and the availability of libraries for interfacing with hardware. Here’s a simple Python script that blinks an LED connected to GPIO pin 17:
import RPi.GPIO as GPIO
import time
GPIO.setmode(GPIO.BCM)
GPIO.setup(17, GPIO.OUT)
try:
while True:
GPIO.output(17, GPIO.HIGH) # Turn on the LED
time.sleep(1) # Wait for 1 second
GPIO.output(17, GPIO.LOW) # Turn off the LED
time.sleep(1) # Wait for 1 second
except KeyboardInterrupt:
GPIO.cleanup() # Clean up GPIO on CTRL+C exit
This script uses the RPi.GPIO library to control the GPIO pins. It sets up pin 17 as an output and then enters a loop that turns the LED on and off with a 1-second delay.
Choosing Between Arduino and Raspberry Pi
Both Arduino and Raspberry Pi have their strengths, and the choice between them depends on your project requirements. Here’s a comparison to help you decide:
Choose Arduino if:
- You need a simple, low-power device for a specific task
- Real-time operations are crucial (e.g., precise timing for motor control)
- You’re working on a beginner-level electronics project
- You need analog inputs (Raspberry Pi doesn’t have built-in analog inputs)
- Battery operation is important
Choose Raspberry Pi if:
- You need more processing power or memory
- Your project requires internet connectivity or networking
- You want to use a full operating system with a graphical interface
- You need to process complex data or run sophisticated algorithms
- You want to use the device as a small desktop computer
Advanced Topics and Project Ideas
Once you’re comfortable with the basics of Arduino or Raspberry Pi, you can explore more advanced topics and create exciting projects. Here are some ideas to inspire you:
For Arduino:
- Home Automation: Create a smart home system that controls lights, temperature, and appliances.
- Weather Station: Build a device that measures temperature, humidity, and pressure, and displays the data on an LCD screen.
- Robot Control: Design a simple robot with motors and sensors, controlled by Arduino.
- Musical Instrument: Create an electronic instrument using buttons, potentiometers, and a speaker.
- Plant Monitoring System: Develop a system that monitors soil moisture and automatically waters plants when needed.
For Raspberry Pi:
- Media Center: Turn your Raspberry Pi into a home media server using software like Kodi.
- Retro Gaming Console: Create a retro gaming machine using emulators.
- Network-Attached Storage (NAS): Build a personal cloud storage system.
- Home Security System: Develop a security camera with motion detection and remote viewing capabilities.
- Voice-Controlled Assistant: Create your own AI assistant using libraries like Google Assistant SDK or Amazon Alexa Voice Service.
Integrating Microcontrollers with Software Development
As you delve deeper into microcontroller programming, you’ll find that many of the skills you develop are transferable to other areas of software development. Here are some ways microcontroller programming can enhance your overall coding skills:
1. Low-Level Programming
Working with microcontrollers often involves programming close to the hardware level. This can give you a better understanding of how computers work at a fundamental level, which can be valuable in many areas of software development, including systems programming and optimization.
2. Real-Time Systems
Many microcontroller projects involve real-time operations, where timing is critical. Understanding how to write code that meets strict timing requirements can be valuable in fields like game development, audio/video processing, and financial trading systems.
3. Resource Optimization
Microcontrollers often have limited resources (memory, processing power), which forces you to write efficient code. These optimization skills can be applied to any software development project, especially in areas like mobile app development or embedded systems.
4. Hardware-Software Integration
Learning to interface software with hardware gives you a more holistic understanding of computer systems. This can be valuable in fields like IoT development, robotics, and computer engineering.
5. Practical Problem-Solving
Microcontroller projects often involve solving real-world problems, which can help develop your problem-solving skills and creativity. These skills are crucial in all areas of software development.
Advanced Programming Concepts for Microcontrollers
As you become more comfortable with basic microcontroller programming, you can explore more advanced concepts to create more sophisticated projects:
1. Interrupts
Interrupts allow your microcontroller to respond immediately to important events without constantly checking for them. This is crucial for real-time applications. Here’s a simple example of using an interrupt with Arduino:
const int buttonPin = 2;
const int ledPin = 13;
volatile int buttonState = LOW;
void setup() {
pinMode(ledPin, OUTPUT);
pinMode(buttonPin, INPUT_PULLUP);
attachInterrupt(digitalPinToInterrupt(buttonPin), buttonPressed, FALLING);
}
void loop() {
digitalWrite(ledPin, buttonState);
}
void buttonPressed() {
buttonState = !buttonState;
}
This code uses an interrupt to toggle an LED whenever a button is pressed, without needing to constantly check the button’s state in the main loop.
2. Communication Protocols
Understanding various communication protocols is essential for interfacing your microcontroller with other devices. Common protocols include:
- I2C (Inter-Integrated Circuit)
- SPI (Serial Peripheral Interface)
- UART (Universal Asynchronous Receiver/Transmitter)
Here’s an example of using I2C communication with Arduino to read data from a sensor:
#include <Wire.h>
void setup() {
Wire.begin();
Serial.begin(9600);
}
void loop() {
Wire.beginTransmission(0x68); // I2C address of the sensor
Wire.write(0x00); // Register to read
Wire.endTransmission(false);
Wire.requestFrom(0x68, 1); // Request 1 byte from the sensor
if (Wire.available()) {
byte data = Wire.read();
Serial.println(data);
}
delay(1000);
}
3. State Machines
State machines are a powerful way to organize code for complex behaviors. They’re particularly useful in embedded systems where you need to manage multiple modes of operation. Here’s a simple state machine example:
enum State {
IDLE,
ACTIVE,
COOLDOWN
};
State currentState = IDLE;
unsigned long stateTimer = 0;
void setup() {
// Setup code here
}
void loop() {
switch (currentState) {
case IDLE:
// IDLE state behavior
if (someCondition()) {
currentState = ACTIVE;
stateTimer = millis();
}
break;
case ACTIVE:
// ACTIVE state behavior
if (millis() - stateTimer > 5000) { // After 5 seconds
currentState = COOLDOWN;
stateTimer = millis();
}
break;
case COOLDOWN:
// COOLDOWN state behavior
if (millis() - stateTimer > 2000) { // After 2 seconds
currentState = IDLE;
}
break;
}
}
bool someCondition() {
// Check for condition to move from IDLE to ACTIVE
return false; // Placeholder
}
The Future of Microcontrollers and IoT
As we look to the future, microcontrollers are set to play an increasingly important role in our daily lives, particularly with the growth of the Internet of Things (IoT). Here are some trends to watch:
1. Edge Computing
With the increasing number of IoT devices, there’s a growing need for processing data closer to where it’s generated, rather than sending everything to the cloud. This is where edge computing comes in, and microcontrollers are at the heart of this trend.
2. AI and Machine Learning on Microcontrollers
As microcontrollers become more powerful, we’re seeing the emergence of TinyML (Tiny Machine Learning), which allows machine learning models to run on resource-constrained devices. This opens up possibilities for smart, responsive devices that can make decisions locally.
3. Energy Harvesting
Future microcontrollers may be able to harvest energy from their environment (light, heat, vibration), potentially eliminating the need for batteries in some applications.
4. Increased Integration
We’re likely to see more System-on-Chip (SoC) designs that integrate microcontrollers with wireless connectivity, sensors, and other components, making it easier to create connected devices.
Conclusion
Understanding microcontrollers and electronics is a valuable skill in today’s technology-driven world. Whether you choose to start with Arduino for its simplicity and extensive community support, or dive into Raspberry Pi for its versatility and computing power, you’re embarking on an exciting journey of discovery and creation.
Remember, the key to mastering microcontroller programming is practice. Start with simple projects, gradually increase complexity, and don’t be afraid to experiment. Each project will teach you something new and help you develop problem-solving skills that are valuable across all areas of programming.
As you progress, you’ll find that the skills you develop in microcontroller programming – understanding hardware-software interaction, optimizing for resource constraints, and thinking in terms of real-time systems – will enhance your capabilities as a programmer in any field.
So grab your Arduino or Raspberry Pi, fire up your IDE, and start bringing your ideas to life. The world of microcontrollers and electronics is waiting for you to explore, innovate, and create. Happy coding!