Understanding Linked Lists and Their Variations: A Comprehensive Guide
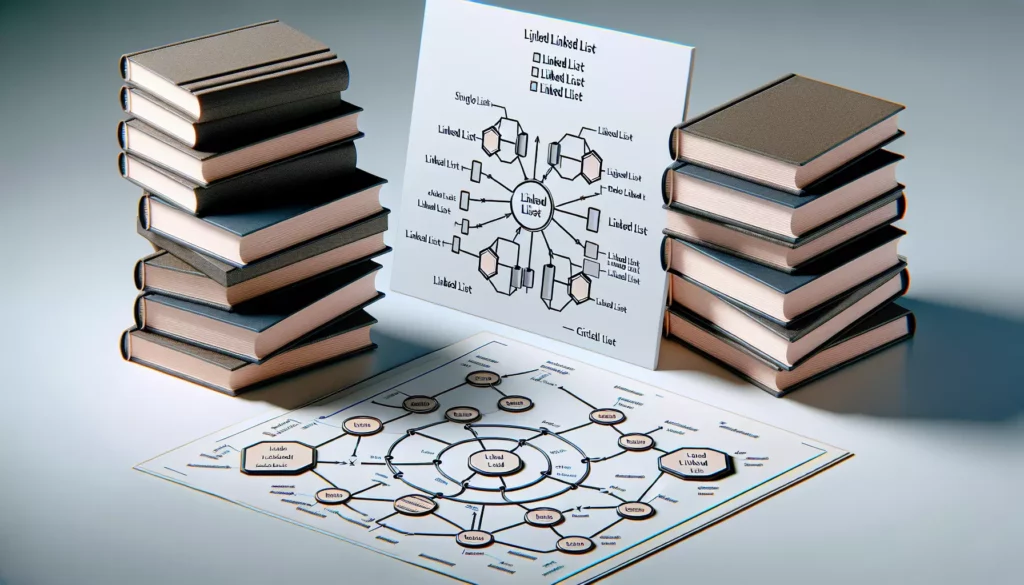
In the world of data structures, linked lists stand out as a fundamental and versatile concept. Whether you’re a beginner programmer or preparing for technical interviews at major tech companies, understanding linked lists is crucial. This comprehensive guide will delve into the intricacies of linked lists, their variations, and their practical applications in coding and algorithmic problem-solving.
What is a Linked List?
A linked list is a linear data structure where elements are stored in nodes. Each node contains a data field and a reference (or link) to the next node in the sequence. Unlike arrays, linked lists do not store elements in contiguous memory locations, allowing for more flexible memory allocation and management.
Basic Structure of a Linked List Node
In most programming languages, a linked list node can be represented as a simple class or struct:
class Node {
int data;
Node next;
public Node(int data) {
this.data = data;
this.next = null;
}
}
This basic structure allows us to create chains of nodes, forming the linked list.
Types of Linked Lists
There are several variations of linked lists, each with its own characteristics and use cases:
1. Singly Linked List
The most basic form of a linked list, where each node points to the next node in the sequence. The last node points to null, indicating the end of the list.
Head -> Node1 -> Node2 -> Node3 -> null
2. Doubly Linked List
In a doubly linked list, each node contains references to both the next and the previous nodes. This allows for bidirectional traversal but requires more memory.
null <- Node1 <-> Node2 <-> Node3 -> null
3. Circular Linked List
A circular linked list is similar to a singly linked list, but the last node points back to the first node, creating a circle.
Head -> Node1 -> Node2 -> Node3 ->
^ |
|___________________________|
4. Circular Doubly Linked List
This combines the features of doubly linked lists and circular linked lists. Each node has references to both the next and previous nodes, and the last node’s next pointer points to the first node, while the first node’s previous pointer points to the last node.
Operations on Linked Lists
Understanding how to perform basic operations on linked lists is crucial for mastering this data structure. Let’s explore some common operations:
1. Insertion
Inserting a new node into a linked list can be done at the beginning, end, or at a specific position.
Insertion at the Beginning
public void insertAtBeginning(int data) {
Node newNode = new Node(data);
newNode.next = head;
head = newNode;
}
Insertion at the End
public void insertAtEnd(int data) {
Node newNode = new Node(data);
if (head == null) {
head = newNode;
return;
}
Node current = head;
while (current.next != null) {
current = current.next;
}
current.next = newNode;
}
2. Deletion
Deleting a node from a linked list involves updating the references to bypass the node to be deleted.
public void deleteNode(int key) {
Node temp = head, prev = null;
if (temp != null && temp.data == key) {
head = temp.next;
return;
}
while (temp != null && temp.data != key) {
prev = temp;
temp = temp.next;
}
if (temp == null) return;
prev.next = temp.next;
}
3. Traversal
Traversing a linked list involves visiting each node in the list sequentially.
public void printList() {
Node current = head;
while (current != null) {
System.out.print(current.data + " -> ");
current = current.next;
}
System.out.println("null");
}
Advantages and Disadvantages of Linked Lists
Like any data structure, linked lists have their pros and cons:
Advantages:
- Dynamic size: Linked lists can grow or shrink in size during program execution.
- Efficient insertion and deletion: Adding or removing elements at the beginning of the list is O(1).
- Flexible memory allocation: Nodes can be stored anywhere in memory.
Disadvantages:
- No random access: Accessing an element by index requires traversing the list, making it O(n).
- Extra memory usage: Each node requires additional memory for storing the reference(s).
- Not cache-friendly: Nodes are not stored in contiguous memory locations, which can lead to more cache misses.
Common Interview Questions and Coding Challenges
Linked lists are a favorite topic in technical interviews, especially at major tech companies. Here are some common questions and challenges you might encounter:
1. Reverse a Linked List
This is a classic problem that tests your understanding of linked list manipulation.
public Node reverseList(Node head) {
Node prev = null;
Node current = head;
Node next = null;
while (current != null) {
next = current.next;
current.next = prev;
prev = current;
current = next;
}
return prev;
}
2. Detect a Cycle in a Linked List
This problem involves detecting if a linked list contains a cycle, often solved using Floyd’s Cycle-Finding Algorithm (also known as the “tortoise and hare” algorithm).
public boolean hasCycle(Node head) {
if (head == null || head.next == null) {
return false;
}
Node slow = head;
Node fast = head.next;
while (slow != fast) {
if (fast == null || fast.next == null) {
return false;
}
slow = slow.next;
fast = fast.next.next;
}
return true;
}
3. Find the Middle of a Linked List
This problem tests your ability to traverse a linked list efficiently.
public Node findMiddle(Node head) {
if (head == null) return null;
Node slow = head;
Node fast = head;
while (fast != null && fast.next != null) {
slow = slow.next;
fast = fast.next.next;
}
return slow;
}
Advanced Linked List Concepts
As you progress in your understanding of linked lists, you’ll encounter more advanced concepts and variations:
1. Skip Lists
Skip lists are a probabilistic data structure that allows for faster search within an ordered sequence of elements. They are built in layers, where the bottom layer is an ordinary linked list, and each higher layer acts as an “express lane” for the lists below, skipping over varying numbers of elements.
2. XOR Linked Lists
XOR linked lists are a memory-efficient variation of doubly linked lists. Instead of storing two pointers for the next and previous nodes, it stores a single pointer which is the XOR of the addresses of the previous and next nodes.
3. Self-Organizing Lists
Self-organizing lists are linked lists that reorder their elements based on access frequency. The goal is to improve average access time by moving frequently accessed elements closer to the front of the list.
Practical Applications of Linked Lists
Understanding the practical applications of linked lists can help you appreciate their importance in real-world scenarios:
1. Implementation of Other Data Structures
Linked lists serve as the foundation for implementing more complex data structures like stacks, queues, and hash tables.
2. Memory Management
Operating systems often use linked lists to manage free memory blocks, allowing for efficient allocation and deallocation of memory.
3. Music Player Playlists
Doubly linked lists are ideal for implementing music player playlists, allowing for easy navigation between songs in both directions.
4. Browser History
Web browsers often use linked lists to implement the back and forward navigation functionality.
5. Undo Functionality in Applications
Many applications use linked lists to implement undo/redo functionality, where each node represents a state of the application.
Optimizing Linked List Operations
As you become more proficient with linked lists, you’ll want to focus on optimizing your implementations for better performance:
1. Tail Pointers
Maintaining a tail pointer in addition to the head pointer can make insertions at the end of the list O(1) instead of O(n).
2. Sentinel Nodes
Using sentinel nodes (dummy nodes at the beginning and/or end of the list) can simplify edge cases and make your code cleaner.
3. Two-Pointer Technique
Many linked list problems can be solved efficiently using two pointers moving at different speeds or starting at different positions.
Linked Lists in Different Programming Languages
While the concept of linked lists remains the same, their implementation can vary across programming languages:
Java
Java provides the LinkedList class in its Collections framework, which implements the List and Deque interfaces.
import java.util.LinkedList;
LinkedList<String> list = new LinkedList<>();
list.add("Apple");
list.add("Banana");
list.addFirst("Orange");
Python
Python doesn’t have a built-in linked list class, but you can easily implement one:
class Node:
def __init__(self, data):
self.data = data
self.next = None
class LinkedList:
def __init__(self):
self.head = None
def append(self, data):
new_node = Node(data)
if not self.head:
self.head = new_node
return
last = self.head
while last.next:
last = last.next
last.next = new_node
C++
C++ provides the std::list container, which is typically implemented as a doubly-linked list:
#include <list>
#include <iostream>
std::list<int> myList;
myList.push_back(10);
myList.push_front(5);
for (int x : myList) {
std::cout << x << " ";
}
Conclusion
Linked lists are a fundamental data structure that play a crucial role in computer science and software development. From basic implementations to advanced variations, understanding linked lists is essential for any programmer aiming to excel in technical interviews and real-world programming challenges.
As you continue your journey in programming and prepare for interviews at major tech companies, remember that mastering linked lists is not just about memorizing implementations. It’s about understanding the underlying concepts, recognizing when to use them, and being able to apply that knowledge to solve complex problems efficiently.
Practice implementing different types of linked lists, solve coding challenges, and explore their applications in various scenarios. With dedication and consistent practice, you’ll not only become proficient in handling linked list problems but also develop a deeper understanding of data structures and algorithms as a whole.
Remember, the journey of learning never ends in the world of programming. Each problem you solve and each concept you master brings you one step closer to becoming a skilled and sought-after developer. Keep coding, keep learning, and embrace the challenges that come with mastering data structures like linked lists!