Understanding Inheritance in Object-Oriented Programming: A Comprehensive Guide
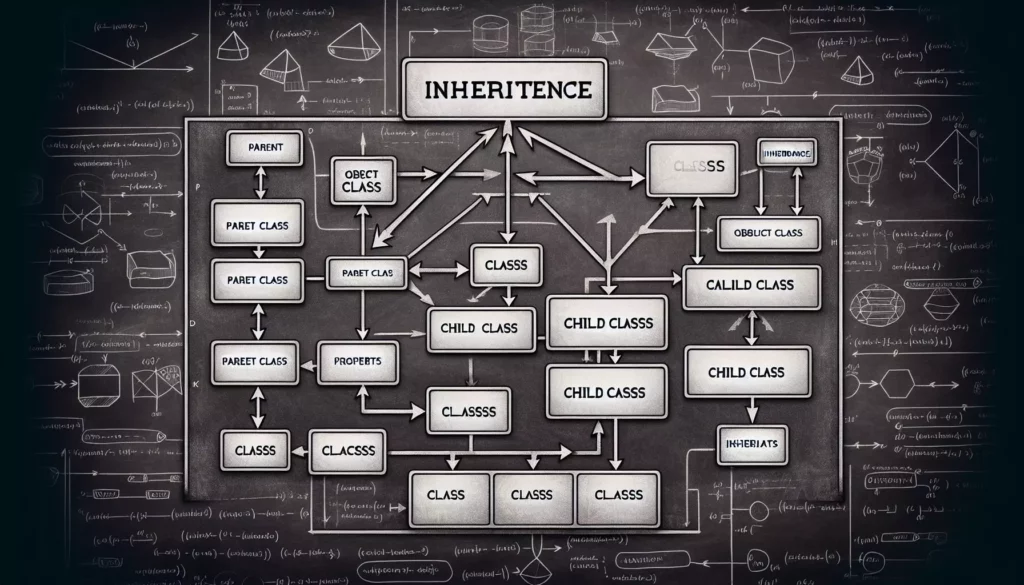
In the world of programming, object-oriented programming (OOP) stands out as a powerful paradigm that allows developers to create efficient, modular, and reusable code. One of the key pillars of OOP is inheritance, a concept that plays a crucial role in structuring and organizing code. In this comprehensive guide, we’ll dive deep into inheritance, exploring its fundamentals, benefits, and practical applications in various programming languages.
Table of Contents
- What is Inheritance?
- Benefits of Inheritance
- Types of Inheritance
- Inheritance in Different Programming Languages
- Best Practices and Common Pitfalls
- Real-World Examples of Inheritance
- Inheritance vs. Composition
- Advanced Concepts in Inheritance
- Conclusion
1. What is Inheritance?
Inheritance is a fundamental concept in object-oriented programming that allows a new class to be based on an existing class. The new class, known as the derived class or subclass, inherits properties and methods from the existing class, called the base class or superclass. This mechanism enables code reuse and establishes a hierarchical relationship between classes.
To understand inheritance better, let’s break it down into its core components:
- Base Class (Superclass): The existing class that serves as a blueprint for derived classes.
- Derived Class (Subclass): A new class that inherits properties and methods from the base class.
- Inheritance Relationship: The “is-a” relationship between the derived class and the base class.
- Inherited Members: Properties and methods that are passed down from the base class to the derived class.
Here’s a simple example to illustrate the concept of inheritance:
// Base class
class Animal {
constructor(name) {
this.name = name;
}
speak() {
console.log(`${this.name} makes a sound.`);
}
}
// Derived class
class Dog extends Animal {
bark() {
console.log(`${this.name} barks.`);
}
}
// Usage
const myDog = new Dog('Buddy');
myDog.speak(); // Output: Buddy makes a sound.
myDog.bark(); // Output: Buddy barks.
In this example, Dog
is a derived class that inherits from the Animal
base class. The Dog
class automatically gains the name
property and the speak()
method from the Animal
class, while also defining its own bark()
method.
2. Benefits of Inheritance
Inheritance offers several advantages that make it a powerful tool in object-oriented programming:
- Code Reusability: Inheritance allows developers to reuse existing code, reducing redundancy and promoting a DRY (Don’t Repeat Yourself) approach to programming.
- Hierarchical Classification: It enables the creation of a clear and logical hierarchy of classes, making it easier to understand and organize complex systems.
- Extensibility: New functionality can be added to existing classes without modifying the original code, promoting easier maintenance and updates.
- Polymorphism: Inheritance facilitates polymorphism, allowing objects of different classes to be treated as objects of a common base class.
- Overriding: Derived classes can override methods from the base class, providing specialized implementations while maintaining a consistent interface.
These benefits contribute to creating more maintainable, scalable, and efficient code structures in object-oriented programming.
3. Types of Inheritance
Inheritance can be implemented in various ways, depending on the programming language and the specific requirements of the system. Here are the main types of inheritance:
3.1 Single Inheritance
Single inheritance is the simplest and most common form of inheritance. In this type, a derived class inherits from only one base class.
class Animal {
// Base class implementation
}
class Dog extends Animal {
// Derived class implementation
}
3.2 Multiple Inheritance
Multiple inheritance allows a derived class to inherit from two or more base classes. This type of inheritance is supported in some programming languages like C++, but not in others like Java or C#.
class Mammal {
// Mammal class implementation
}
class Swimmer {
// Swimmer class implementation
}
class Dolphin : public Mammal, public Swimmer {
// Dolphin class inherits from both Mammal and Swimmer
}
3.3 Multilevel Inheritance
Multilevel inheritance involves a chain of inheritance where a derived class becomes the base class for another derived class.
class Animal {
// Animal class implementation
}
class Mammal extends Animal {
// Mammal class implementation
}
class Dog extends Mammal {
// Dog class implementation
}
3.4 Hierarchical Inheritance
In hierarchical inheritance, multiple derived classes inherit from a single base class.
class Animal {
// Animal class implementation
}
class Dog extends Animal {
// Dog class implementation
}
class Cat extends Animal {
// Cat class implementation
}
3.5 Hybrid Inheritance
Hybrid inheritance is a combination of two or more types of inheritance. It’s less common but can be useful in complex scenarios.
class A {
// Class A implementation
}
class B extends A {
// Class B implementation
}
class C extends A {
// Class C implementation
}
class D extends B, C {
// Class D implements hybrid inheritance
}
Understanding these types of inheritance is crucial for designing effective class hierarchies and choosing the most appropriate inheritance structure for your specific programming needs.
4. Inheritance in Different Programming Languages
While the concept of inheritance is universal in object-oriented programming, its implementation can vary across different programming languages. Let’s explore how inheritance is handled in some popular languages:
4.1 Java
Java supports single inheritance for classes but allows multiple inheritance through interfaces.
public class Animal {
// Animal class implementation
}
public class Dog extends Animal {
// Dog class implementation
}
public interface Swimmer {
// Swimmer interface
}
public class Labrador extends Dog implements Swimmer {
// Labrador class implementation
}
4.2 Python
Python supports multiple inheritance, allowing a class to inherit from multiple base classes.
class Animal:
# Animal class implementation
class Swimmer:
# Swimmer class implementation
class Dolphin(Animal, Swimmer):
# Dolphin class inherits from both Animal and Swimmer
4.3 C++
C++ supports multiple inheritance, allowing a class to inherit from multiple base classes.
class Animal {
// Animal class implementation
};
class Swimmer {
// Swimmer class implementation
};
class Dolphin : public Animal, public Swimmer {
// Dolphin class inherits from both Animal and Swimmer
};
4.4 C#
C# supports single inheritance for classes but allows multiple inheritance through interfaces, similar to Java.
public class Animal
{
// Animal class implementation
}
public interface ISwimmer
{
// Swimmer interface
}
public class Dolphin : Animal, ISwimmer
{
// Dolphin class implementation
}
4.5 JavaScript (ES6+)
JavaScript implements prototype-based inheritance but provides a class syntax for more traditional OOP-style inheritance.
class Animal {
// Animal class implementation
}
class Dog extends Animal {
// Dog class implementation
}
Understanding these language-specific implementations is crucial for effectively applying inheritance in your chosen programming environment.
5. Best Practices and Common Pitfalls
While inheritance is a powerful tool, it’s important to use it judiciously and be aware of potential pitfalls. Here are some best practices and common issues to watch out for:
Best Practices:
- Favor Composition Over Inheritance: In many cases, composition (has-a relationship) can be more flexible and maintainable than inheritance (is-a relationship).
- Use Inheritance for “is-a” Relationships: Ensure that the derived class truly is a more specific type of the base class.
- Keep the Inheritance Hierarchy Shallow: Deep inheritance hierarchies can become difficult to understand and maintain.
- Follow the Liskov Substitution Principle: Derived classes should be substitutable for their base classes without affecting the correctness of the program.
- Use Abstract Classes and Interfaces: These can provide a clear contract for derived classes and promote loose coupling.
Common Pitfalls:
- Overuse of Inheritance: Relying too heavily on inheritance can lead to inflexible and tightly coupled designs.
- Breaking Encapsulation: Inheritance can potentially break encapsulation if not designed carefully.
- The Diamond Problem: In multiple inheritance, ambiguity can arise when two base classes implement the same method.
- Tight Coupling: Inheritance can create tight coupling between base and derived classes, making changes more difficult.
- Incorrect Abstraction: Poor design of the base class can lead to derived classes that don’t fit the abstraction well.
By following these best practices and being aware of the potential pitfalls, you can leverage inheritance effectively in your object-oriented designs.
6. Real-World Examples of Inheritance
To better understand how inheritance is applied in practical scenarios, let’s explore some real-world examples:
6.1 Vehicle Hierarchy
class Vehicle {
constructor(make, model) {
this.make = make;
this.model = model;
}
startEngine() {
console.log(`The ${this.make} ${this.model}'s engine is starting.`);
}
}
class Car extends Vehicle {
drive() {
console.log(`The ${this.make} ${this.model} is being driven.`);
}
}
class Motorcycle extends Vehicle {
wheelie() {
console.log(`The ${this.make} ${this.model} is doing a wheelie!`);
}
}
// Usage
const myCar = new Car('Toyota', 'Corolla');
myCar.startEngine(); // Inherited method
myCar.drive(); // Car-specific method
const myMotorcycle = new Motorcycle('Harley-Davidson', 'Street 750');
myMotorcycle.startEngine(); // Inherited method
myMotorcycle.wheelie(); // Motorcycle-specific method
In this example, Car
and Motorcycle
inherit common properties and methods from the Vehicle
class while adding their own specific functionalities.
6.2 Employee Management System
class Employee {
constructor(name, id) {
this.name = name;
this.id = id;
}
getDetails() {
return `Name: ${this.name}, ID: ${this.id}`;
}
}
class Manager extends Employee {
constructor(name, id, department) {
super(name, id);
this.department = department;
}
getDetails() {
return `${super.getDetails()}, Department: ${this.department}`;
}
}
class Developer extends Employee {
constructor(name, id, programmingLanguage) {
super(name, id);
this.programmingLanguage = programmingLanguage;
}
getDetails() {
return `${super.getDetails()}, Language: ${this.programmingLanguage}`;
}
}
// Usage
const manager = new Manager('Alice', 'M001', 'IT');
console.log(manager.getDetails());
const developer = new Developer('Bob', 'D001', 'JavaScript');
console.log(developer.getDetails());
This example demonstrates how inheritance can be used to create a hierarchical structure for different types of employees in a company, with each type having its own specific attributes and behaviors.
6.3 Shape Hierarchy for a Graphics Application
class Shape {
constructor(color) {
this.color = color;
}
draw() {
console.log(`Drawing a ${this.color} shape.`);
}
}
class Circle extends Shape {
constructor(color, radius) {
super(color);
this.radius = radius;
}
draw() {
console.log(`Drawing a ${this.color} circle with radius ${this.radius}.`);
}
}
class Rectangle extends Shape {
constructor(color, width, height) {
super(color);
this.width = width;
this.height = height;
}
draw() {
console.log(`Drawing a ${this.color} rectangle with width ${this.width} and height ${this.height}.`);
}
}
// Usage
const circle = new Circle('red', 5);
circle.draw();
const rectangle = new Rectangle('blue', 10, 20);
rectangle.draw();
This example illustrates how inheritance can be used in a graphics application to create a hierarchy of shapes, each with its own specific properties and drawing behavior.
These real-world examples demonstrate how inheritance can be applied to model complex systems and relationships between different entities in a program.
7. Inheritance vs. Composition
While inheritance is a powerful feature of object-oriented programming, it’s not always the best solution for every design problem. Composition is another fundamental concept in OOP that often provides a more flexible alternative to inheritance. Let’s compare these two approaches:
Inheritance:
- Represents an “is-a” relationship
- Allows code reuse through a class hierarchy
- Supports polymorphism
- Can lead to tight coupling between classes
- May result in a rigid class hierarchy
Composition:
- Represents a “has-a” relationship
- Allows code reuse by combining objects
- Provides more flexibility in designing relationships between classes
- Promotes loose coupling
- Easier to modify and extend
Here’s an example to illustrate the difference:
// Inheritance approach
class Animal {
makeSound() {
console.log('Some generic animal sound');
}
}
class Dog extends Animal {
makeSound() {
console.log('Woof!');
}
}
// Composition approach
class Animal {
constructor(sound) {
this.sound = sound;
}
makeSound() {
console.log(this.sound);
}
}
class Dog {
constructor() {
this.animal = new Animal('Woof!');
}
bark() {
this.animal.makeSound();
}
}
// Usage
const inheritanceDog = new Dog();
inheritanceDog.makeSound(); // Output: Woof!
const compositionDog = new Dog();
compositionDog.bark(); // Output: Woof!
In the inheritance approach, Dog
is tightly coupled to Animal
. If we want to change the sound-making behavior, we need to modify the inheritance hierarchy. In the composition approach, we can easily change the sound by passing a different sound to the Animal
constructor, providing more flexibility.
The choice between inheritance and composition depends on the specific requirements of your system. As a general guideline, favor composition over inheritance when possible, as it often leads to more flexible and maintainable code.
8. Advanced Concepts in Inheritance
As you delve deeper into object-oriented programming and inheritance, you’ll encounter several advanced concepts that build upon the basic principles. Let’s explore some of these concepts:
8.1 Abstract Classes
Abstract classes are classes that cannot be instantiated and are meant to be subclassed. They often contain abstract methods that must be implemented by their subclasses.
abstract class Shape {
abstract calculateArea(): number;
display(): void {
console.log(`The area is ${this.calculateArea()}`);
}
}
class Circle extends Shape {
constructor(private radius: number) {
super();
}
calculateArea(): number {
return Math.PI * this.radius * this.radius;
}
}
// Usage
const circle = new Circle(5);
circle.display(); // Output: The area is 78.53981633974483
8.2 Interfaces
Interfaces define a contract that classes must adhere to. They specify a set of methods that a class must implement.
interface Drawable {
draw(): void;
}
class Square implements Drawable {
draw(): void {
console.log('Drawing a square');
}
}
class Circle implements Drawable {
draw(): void {
console.log('Drawing a circle');
}
}
// Usage
function renderShape(shape: Drawable) {
shape.draw();
}
renderShape(new Square()); // Output: Drawing a square
renderShape(new Circle()); // Output: Drawing a circle
8.3 Mixins
Mixins are a way of adding methods to a class without using inheritance. They’re particularly useful in languages that don’t support multiple inheritance.
// Mixin
const SwimMixin = {
swim() {
console.log(`${this.name} is swimming.`);
}
};
class Animal {
constructor(name) {
this.name = name;
}
}
class Fish extends Animal {}
Object.assign(Fish.prototype, SwimMixin);
class Duck extends Animal {}
Object.assign(Duck.prototype, SwimMixin);
// Usage
const nemo = new Fish('Nemo');
nemo.swim(); // Output: Nemo is swimming.
const donald = new Duck('Donald');
donald.swim(); // Output: Donald is swimming.
8.4 Method Overriding and the super Keyword
Method overriding allows a subclass to provide a specific implementation of a method that is already defined in its superclass. The super
keyword is used to call methods on the superclass.
class Animal {
makeSound() {
console.log('Some generic animal sound');
}
}
class Dog extends Animal {
makeSound() {
super.makeSound(); // Call the superclass method
console.log('Woof!');
}
}
// Usage
const dog = new Dog();
dog.makeSound();
// Output:
// Some generic animal sound
// Woof!
8.5 Final Classes and Methods
Some languages support the concept of final classes (which cannot be subclassed) and final methods (which cannot be overridden). This is useful for preventing unintended modifications to critical parts of your code.
// Example in Java
public final class ImmutableClass {
// This class cannot be subclassed
}
public class SomeClass {
public final void criticalMethod() {
// This method cannot be overridden
}
}
These advanced concepts provide powerful tools for creating more sophisticated and robust object-oriented designs. As you become more comfortable with basic inheritance, exploring these concepts will allow you to create more flexible, maintainable, and efficient code structures.
9. Conclusion
Inheritance is a fundamental concept in object-oriented programming that allows developers to create hierarchical relationships between classes, promoting code reuse and establishing a clear organization of code. Throughout this comprehensive guide, we’ve explored the various aspects of inheritance, from its basic principles to advanced concepts and real-world applications.
Key takeaways from this guide include:
- Understanding the core concept of inheritance and its role in OOP
- Recognizing the benefits of inheritance, such as code reusability and extensibility
- Exploring different types of inheritance and their implementations across various programming languages
- Learning best practices and common pitfalls to avoid when using inheritance
- Examining real-world examples of inheritance in action
- Comparing inheritance with composition and understanding when to use each approach
- Delving into advanced concepts like abstract classes, interfaces, and method overriding
As you continue to develop your programming skills, remember that inheritance is just one tool in the object-oriented programming toolbox. While it’s a powerful concept, it’s essential to use it judiciously and in combination with other OOP principles and design patterns to create robust, maintainable, and efficient software systems.
By mastering inheritance and understanding its nuances, you’ll be better equipped to design and implement complex software solutions, tackle coding challenges, and excel in technical interviews, particularly when targeting positions at major tech companies.
Continue to practice applying inheritance in your projects, explore its implementation in different programming languages, and stay updated with evolving best practices in software design. With a solid grasp of inheritance and other OOP concepts, you’ll be well-prepared to tackle the challenges of modern software development and advance your career in the tech industry.