Understanding Inheritance in Object-Oriented Programming: A Comprehensive Guide
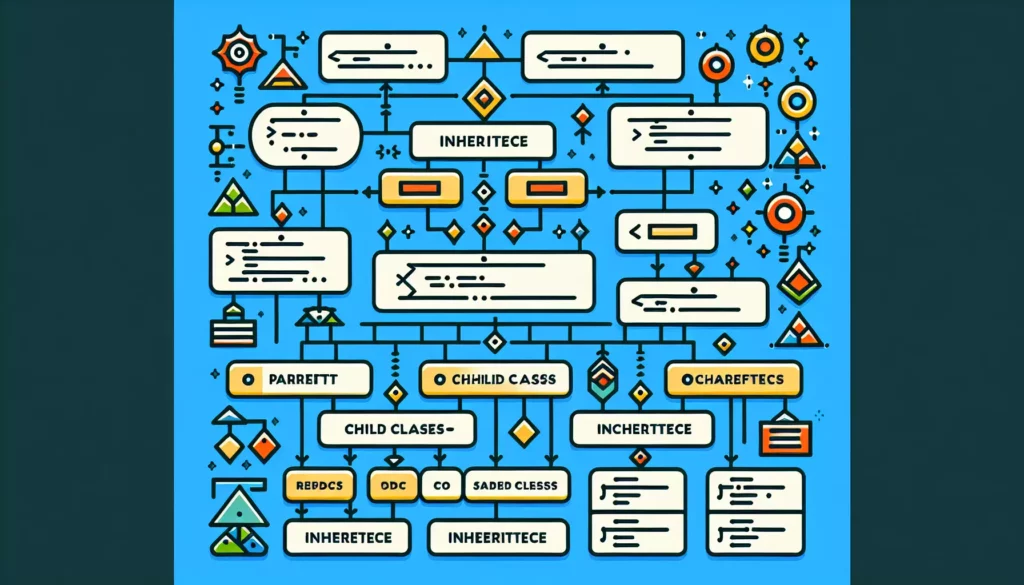
In the world of programming, object-oriented programming (OOP) stands as a paradigm that has revolutionized how we structure and design software. Among its core principles, inheritance plays a crucial role in creating efficient, reusable, and organized code. Whether you’re a beginner just starting your coding journey or an experienced developer looking to solidify your understanding, this comprehensive guide will delve deep into the concept of inheritance in OOP.
Table of Contents
- What is Inheritance?
- The Importance of Inheritance in OOP
- Types of Inheritance
- Implementing Inheritance in Different Programming Languages
- Benefits of Using Inheritance
- Challenges and Considerations with Inheritance
- Best Practices for Using Inheritance
- Real-World Examples of Inheritance
- Inheritance vs. Composition
- Common Interview Questions on Inheritance
- Conclusion
1. What is Inheritance?
Inheritance is a fundamental concept in object-oriented programming that allows a new class to be based on an existing class. The new class, known as the derived class or subclass, inherits properties and methods from the existing class, called the base class or superclass. This mechanism enables code reuse and establishes a relationship between classes that mirrors real-world hierarchies.
To understand inheritance better, let’s break it down with an analogy:
Imagine a family tree. Just as children inherit traits from their parents, in OOP, a subclass inherits characteristics (properties and methods) from its parent class. This inheritance can span multiple generations, creating a hierarchy of classes.
2. The Importance of Inheritance in OOP
Inheritance is not just a feature of OOP; it’s a cornerstone that supports several key objectives:
- Code Reusability: Inheritance allows developers to reuse code from existing classes, reducing redundancy and promoting efficiency.
- Hierarchical Classification: It helps in organizing classes into a hierarchical structure, reflecting real-world relationships between objects.
- Extensibility: New functionality can be added to existing code without modifying it, adhering to the open-closed principle of SOLID.
- Polymorphism: Inheritance is the foundation for polymorphism, allowing objects of different classes to be treated as objects of a common base class.
3. Types of Inheritance
Inheritance comes in various forms, each serving different purposes in software design:
3.1 Single Inheritance
In single inheritance, a subclass inherits from only one superclass. This is the simplest and most common form of inheritance.
3.2 Multiple Inheritance
Multiple inheritance allows a subclass to inherit from more than one superclass. While powerful, it can lead to complexities like the “diamond problem” and is not supported in all programming languages.
3.3 Multilevel Inheritance
This type involves a chain of inheritance where a subclass becomes the superclass for another class, creating a hierarchy of classes.
3.4 Hierarchical Inheritance
In hierarchical inheritance, multiple subclasses inherit from a single superclass, creating a tree-like structure.
3.5 Hybrid Inheritance
Hybrid inheritance is a combination of two or more types of inheritance, often seen in complex system designs.
4. Implementing Inheritance in Different Programming Languages
While the concept of inheritance is universal in OOP, its implementation can vary across programming languages. Let’s look at how inheritance is implemented in some popular languages:
4.1 Java
In Java, inheritance is implemented using the extends
keyword for classes and the implements
keyword for interfaces.
public class Animal {
// Base class
}
public class Dog extends Animal {
// Subclass inheriting from Animal
}
4.2 Python
Python uses parentheses to denote inheritance, and supports multiple inheritance.
class Animal:
# Base class
pass
class Dog(Animal):
# Subclass inheriting from Animal
pass
4.3 C++
C++ uses a colon to denote inheritance and supports multiple inheritance.
class Animal {
// Base class
};
class Dog : public Animal {
// Subclass inheriting from Animal
};
4.4 JavaScript (ES6+)
JavaScript implements inheritance through prototypes, but ES6 introduced a more familiar class-based syntax using the extends
keyword.
class Animal {
// Base class
}
class Dog extends Animal {
// Subclass inheriting from Animal
}
5. Benefits of Using Inheritance
Inheritance offers numerous advantages that contribute to better software design and development:
- Code Reusability: Inheritance allows developers to reuse code from existing classes, reducing redundancy and promoting efficiency. This not only saves time but also reduces the chances of errors that might occur when rewriting similar code.
- Modularity: By organizing code into a hierarchy of classes, inheritance promotes a modular approach to software design. This makes the code easier to understand, maintain, and modify.
- Extensibility: New functionality can be added to existing code without modifying it, adhering to the open-closed principle of SOLID. This makes it easier to extend the capabilities of a system without risking the stability of existing code.
- Polymorphism: Inheritance is the foundation for polymorphism, allowing objects of different classes to be treated as objects of a common base class. This enables more flexible and dynamic code.
- Improved Organization: Inheritance helps in creating a logical structure for your code, mirroring real-world relationships between objects. This can make the overall design of a system more intuitive and easier to understand.
- Code Maintainability: With proper use of inheritance, changes made to a base class automatically propagate to all its subclasses, reducing the effort required for maintenance and updates.
6. Challenges and Considerations with Inheritance
While inheritance is a powerful tool, it’s not without its challenges. Here are some considerations to keep in mind:
6.1 Tight Coupling
Inheritance can create tight coupling between classes, making the system less flexible and harder to modify. Changes in the base class can have far-reaching effects on all subclasses.
6.2 The Fragile Base Class Problem
Modifications to the base class can potentially break functionality in subclasses, a phenomenon known as the “fragile base class problem.”
6.3 Complexity in Large Hierarchies
As class hierarchies grow, they can become complex and difficult to understand, especially with multiple inheritance.
6.4 Overuse of Inheritance
Overreliance on inheritance can lead to inflexible designs. Sometimes, composition or interfaces might be more appropriate solutions.
6.5 The Diamond Problem
In languages that support multiple inheritance, the diamond problem can occur when a class inherits from two classes that have a common ancestor, leading to ambiguity.
7. Best Practices for Using Inheritance
To make the most of inheritance while avoiding its pitfalls, consider these best practices:
- Follow the “Is-A” Relationship: Use inheritance only when there’s a clear “is-a” relationship between the subclass and superclass. For example, a “Dog” is an “Animal”.
- Favor Composition Over Inheritance: When in doubt, prefer composition over inheritance. This can lead to more flexible and less tightly coupled designs.
- Keep the Hierarchy Shallow: Avoid deep inheritance hierarchies. A good rule of thumb is to keep it no more than three levels deep.
- Use Abstract Classes and Interfaces: These can provide a cleaner way to define shared behavior without the tight coupling of concrete inheritance.
- Override with Care: When overriding methods, ensure that the new implementation doesn’t violate the expectations set by the base class (Liskov Substitution Principle).
- Document the Inheritance Design: Clearly document the purpose and design of your inheritance hierarchy to help other developers understand and maintain the code.
8. Real-World Examples of Inheritance
Let’s explore some real-world scenarios where inheritance is commonly used:
8.1 Vehicle Hierarchy
A classic example is a vehicle hierarchy:
class Vehicle {
void start() { /* ... */ }
void stop() { /* ... */ }
}
class Car extends Vehicle {
void accelerate() { /* ... */ }
}
class ElectricCar extends Car {
void charge() { /* ... */ }
}
8.2 Shape Hierarchy in Graphics Programming
In graphics programming, shapes often form an inheritance hierarchy:
abstract class Shape {
abstract double area();
abstract double perimeter();
}
class Circle extends Shape {
double radius;
double area() { return Math.PI * radius * radius; }
double perimeter() { return 2 * Math.PI * radius; }
}
class Rectangle extends Shape {
double width;
double height;
double area() { return width * height; }
double perimeter() { return 2 * (width + height); }
}
8.3 Employee Management System
In an employee management system, different types of employees can inherit from a base Employee class:
class Employee {
String name;
int id;
double calculateSalary() { /* ... */ }
}
class Manager extends Employee {
List<Employee> team;
double calculateBonus() { /* ... */ }
}
class Developer extends Employee {
String programmingLanguage;
void code() { /* ... */ }
}
9. Inheritance vs. Composition
While inheritance is powerful, it’s not always the best solution. Composition offers an alternative approach to code reuse and object relationships.
Inheritance
- Represents an “is-a” relationship
- Allows for code reuse through extending base classes
- Can lead to tight coupling between classes
- Supports polymorphism directly
Composition
- Represents a “has-a” relationship
- Achieves code reuse by containing other objects
- Provides more flexibility and loose coupling
- Can be more complex to implement but often results in more maintainable code
Here’s an example comparing inheritance and composition:
// Inheritance
class Car extends Vehicle {
void drive() { /* ... */ }
}
// Composition
class Car {
private Engine engine;
private Wheels wheels;
void drive() {
engine.start();
wheels.rotate();
}
}
The choice between inheritance and composition depends on the specific requirements of your system and the relationships between your objects.
10. Common Interview Questions on Inheritance
Inheritance is a popular topic in technical interviews. Here are some common questions you might encounter:
- What is inheritance and why is it important in OOP?
Answer: Inheritance is a mechanism where a new class is derived from an existing class, inheriting its properties and methods. It’s important because it promotes code reuse, establishes a hierarchical relationship between classes, and supports polymorphism. - What is the difference between single inheritance and multiple inheritance?
Answer: Single inheritance is when a class inherits from only one superclass. Multiple inheritance is when a class can inherit from more than one superclass. Not all programming languages support multiple inheritance due to its potential complexities. - Explain the concept of method overriding in inheritance.
Answer: Method overriding occurs when a subclass provides a specific implementation for a method that is already defined in its superclass. This allows the subclass to change or extend the behavior of the inherited method. - What is the ‘super’ keyword used for in inheritance?
Answer: The ‘super’ keyword is used to refer to the superclass. It can be used to call the superclass constructor or to access superclass methods that have been overridden in the subclass. - Can you explain the concept of the “diamond problem” in multiple inheritance?
Answer: The diamond problem occurs in multiple inheritance when a class inherits from two classes that have a common ancestor. This can lead to ambiguity about which version of a method to use if it’s defined in multiple parent classes.
11. Conclusion
Inheritance is a powerful feature of object-oriented programming that allows for code reuse, hierarchical classification of objects, and supports polymorphism. While it offers numerous benefits, it’s important to use inheritance judiciously, considering its potential drawbacks and alternatives like composition.
As you continue your journey in programming, remember that mastering concepts like inheritance is crucial for writing efficient, maintainable, and scalable code. Whether you’re preparing for technical interviews or building complex software systems, a solid understanding of inheritance will serve you well.
Keep practicing, exploring real-world applications, and considering the trade-offs between inheritance and other design patterns. With time and experience, you’ll develop the intuition to know when and how to best apply inheritance in your projects.
Happy coding!