Understanding Inheritance and Polymorphism in Object-Oriented Programming
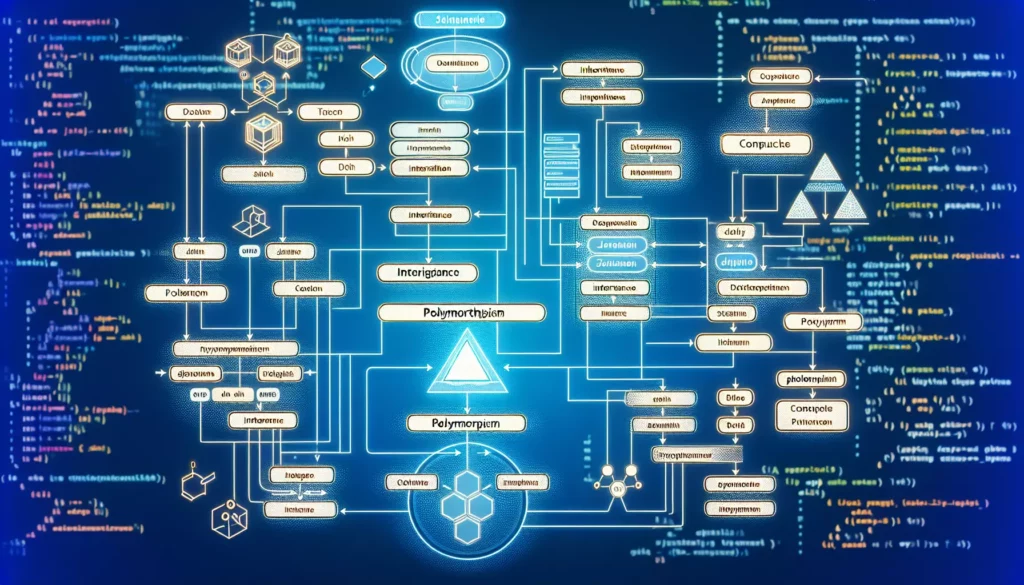
Object-Oriented Programming (OOP) is a fundamental paradigm in modern software development, and two of its core concepts are inheritance and polymorphism. These powerful features allow developers to create more efficient, reusable, and maintainable code. In this comprehensive guide, we’ll dive deep into inheritance and polymorphism, exploring their definitions, benefits, and practical applications in various programming languages.
What is Inheritance?
Inheritance is a mechanism in OOP that allows a new class to be based on an existing class. The new class, called the derived or child class, inherits properties and methods from the existing class, known as the base or parent class. This concept promotes code reusability and establishes a hierarchical relationship between classes.
Key Benefits of Inheritance:
- Code Reusability: Inherit common properties and methods from parent classes
- Hierarchical Classification: Organize classes in a logical structure
- Method Overriding: Modify inherited methods to suit specific needs
- Extensibility: Easily add new features to existing classes
Types of Inheritance:
- Single Inheritance: A derived class inherits from a single base class
- Multiple Inheritance: A derived class inherits from multiple base classes (supported in some languages like C++)
- Multilevel Inheritance: A derived class inherits from another derived class
- Hierarchical Inheritance: Multiple derived classes inherit from a single base class
- Hybrid Inheritance: A combination of two or more types of inheritance
Example of Inheritance in Python:
class Animal:
def __init__(self, name):
self.name = name
def speak(self):
pass
class Dog(Animal):
def speak(self):
return f"{self.name} says Woof!"
class Cat(Animal):
def speak(self):
return f"{self.name} says Meow!"
dog = Dog("Buddy")
cat = Cat("Whiskers")
print(dog.speak()) # Output: Buddy says Woof!
print(cat.speak()) # Output: Whiskers says Meow!
In this example, both Dog
and Cat
classes inherit from the Animal
class, demonstrating single inheritance.
Understanding Polymorphism
Polymorphism, derived from Greek words meaning “many forms,” is a concept in OOP that allows objects of different classes to be treated as objects of a common base class. It enables a single interface to represent different underlying forms (data types or classes).
Key Benefits of Polymorphism:
- Flexibility: Write code that can work with objects of multiple types
- Extensibility: Easily add new classes without modifying existing code
- Simplification: Reduce complex switch or if-else statements
- Interface-based Programming: Program to interfaces rather than concrete implementations
Types of Polymorphism:
- Compile-time Polymorphism (Static Binding):
- Method Overloading: Multiple methods with the same name but different parameters
- Operator Overloading: Giving special meanings to operators for user-defined types
- Runtime Polymorphism (Dynamic Binding):
- Method Overriding: Redefining a method in a derived class that is already defined in the base class
Example of Polymorphism in Java:
interface Shape {
double getArea();
}
class Circle implements Shape {
private double radius;
public Circle(double radius) {
this.radius = radius;
}
@Override
public double getArea() {
return Math.PI * radius * radius;
}
}
class Rectangle implements Shape {
private double width;
private double height;
public Rectangle(double width, double height) {
this.width = width;
this.height = height;
}
@Override
public double getArea() {
return width * height;
}
}
public class Main {
public static void printArea(Shape shape) {
System.out.println("Area: " + shape.getArea());
}
public static void main(String[] args) {
Shape circle = new Circle(5);
Shape rectangle = new Rectangle(4, 6);
printArea(circle); // Output: Area: 78.53981633974483
printArea(rectangle); // Output: Area: 24.0
}
}
In this example, both Circle
and Rectangle
implement the Shape
interface, demonstrating polymorphism through the common getArea()
method.
The Relationship Between Inheritance and Polymorphism
Inheritance and polymorphism are closely related concepts in OOP. Inheritance provides a mechanism for creating hierarchical relationships between classes, while polymorphism allows objects of these related classes to be treated uniformly. Together, they enable powerful design patterns and promote code flexibility and reusability.
Key Relationships:
- Inheritance as a Foundation: Inheritance establishes the “is-a” relationship necessary for polymorphism
- Method Overriding: Inheritance allows method overriding, which is a form of runtime polymorphism
- Interface Implementation: Interfaces, a form of inheritance, enable polymorphism through common method signatures
- Abstract Classes: Combine inheritance and polymorphism by providing a common interface with some implemented methods
Practical Applications of Inheritance and Polymorphism
Understanding how to apply inheritance and polymorphism in real-world scenarios is crucial for effective object-oriented design. Let’s explore some common applications and design patterns that leverage these concepts.
1. GUI Frameworks
GUI frameworks extensively use inheritance and polymorphism to create flexible and extensible user interface components.
abstract class UIComponent {
protected int x, y, width, height;
public abstract void draw();
public abstract void handleClick();
}
class Button extends UIComponent {
private String label;
public Button(String label) {
this.label = label;
}
@Override
public void draw() {
// Draw button with label
}
@Override
public void handleClick() {
// Handle button click event
}
}
class Checkbox extends UIComponent {
private boolean isChecked;
@Override
public void draw() {
// Draw checkbox
}
@Override
public void handleClick() {
isChecked = !isChecked;
// Update checkbox state
}
}
2. Game Development
Game engines often use inheritance and polymorphism to manage different types of game objects and their behaviors.
abstract class GameObject {
protected float x, y;
protected float velocityX, velocityY;
public abstract void update();
public abstract void render();
}
class Player extends GameObject {
@Override
public void update() {
// Update player position based on input
}
@Override
public void render() {
// Render player sprite
}
}
class Enemy extends GameObject {
@Override
public void update() {
// Update enemy AI and position
}
@Override
public void render() {
// Render enemy sprite
}
}
3. Plugin Systems
Plugin architectures often rely on inheritance and polymorphism to allow for extensibility and modularity.
interface Plugin {
void initialize();
void execute();
void shutdown();
}
class AudioPlugin implements Plugin {
@Override
public void initialize() {
// Set up audio system
}
@Override
public void execute() {
// Process audio
}
@Override
public void shutdown() {
// Clean up audio resources
}
}
class VideoPlugin implements Plugin {
@Override
public void initialize() {
// Set up video system
}
@Override
public void execute() {
// Process video
}
@Override
public void shutdown() {
// Clean up video resources
}
}
Best Practices for Using Inheritance and Polymorphism
While inheritance and polymorphism are powerful tools, they can be misused, leading to complex and hard-to-maintain code. Here are some best practices to follow:
1. Favor Composition Over Inheritance
While inheritance is useful, it can sometimes lead to tight coupling between classes. Consider using composition (has-a relationship) instead of inheritance (is-a relationship) when appropriate.
2. Use the Liskov Substitution Principle
Ensure that objects of a superclass can be replaced with objects of its subclasses without affecting the correctness of the program.
3. Keep the Inheritance Hierarchy Shallow
Deep inheritance hierarchies can become difficult to understand and maintain. Try to keep your inheritance tree no more than 2-3 levels deep.
4. Use Interfaces for Multiple Inheritance
In languages that don’t support multiple inheritance (like Java), use interfaces to achieve similar functionality without the complexities of multiple inheritance.
5. Override with Care
When overriding methods, ensure that the new implementation doesn’t violate the expected behavior of the base class method.
6. Use Abstract Classes Judiciously
Abstract classes are useful for defining a common interface with some default behavior, but overuse can lead to inflexibility.
Common Pitfalls and How to Avoid Them
Even experienced developers can fall into traps when working with inheritance and polymorphism. Here are some common pitfalls and how to avoid them:
1. The Diamond Problem
In multiple inheritance, when a class inherits from two classes that have a common ancestor, it can lead to ambiguity.
Solution: Use interfaces or avoid multiple inheritance altogether. In languages like C++ that support multiple inheritance, use virtual inheritance.
2. Overusing Inheritance
Creating deep inheritance hierarchies or using inheritance where composition would be more appropriate.
Solution: Always question whether inheritance is the best tool for the job. Consider composition or interfaces as alternatives.
3. Breaking Encapsulation
Subclasses having too much access to superclass internals, leading to tight coupling.
Solution: Use protected members judiciously and provide public methods for necessary interactions.
4. Ignoring the Liskov Substitution Principle
Creating subclasses that don’t fully substitute for their parent classes can lead to unexpected behavior.
Solution: Ensure that subclasses truly represent specializations of their parent classes and maintain the expected behavior.
5. Performance Overhead
Excessive use of virtual functions (in languages like C++) can lead to performance overhead due to dynamic dispatch.
Solution: Use virtual functions only when necessary. Consider alternatives like the Template Method pattern for some scenarios.
Advanced Concepts in Inheritance and Polymorphism
As you become more comfortable with the basics, it’s worth exploring some advanced concepts that can further enhance your use of inheritance and polymorphism:
1. Mixins and Traits
Mixins (in languages like Ruby) and traits (in languages like Scala or PHP) provide a way to share behavior among classes without using inheritance.
2. The Curiously Recurring Template Pattern (CRTP)
This C++ idiom allows a class to appear in its own base class’s template parameter, enabling static polymorphism.
3. Covariant Return Types
Some languages allow overridden methods to return a more specific type than the method in the superclass.
4. Virtual Inheritance
In C++, virtual inheritance is used to solve the diamond problem in multiple inheritance scenarios.
5. Aspect-Oriented Programming (AOP)
AOP complements OOP by allowing the modularization of cross-cutting concerns, often using inheritance-like mechanisms.
Conclusion
Inheritance and polymorphism are cornerstone concepts in object-oriented programming that enable developers to create flexible, reusable, and maintainable code. By understanding these concepts deeply and applying them judiciously, you can significantly improve your software design skills.
Remember that while these tools are powerful, they should be used thoughtfully. Always consider the specific needs of your project and the long-term maintainability of your code. As you gain experience, you’ll develop a keen sense of when and how to best apply inheritance and polymorphism in your software designs.
Continue to practice and explore these concepts in your projects. Experiment with different design patterns and architectural styles that leverage inheritance and polymorphism. As you do, you’ll not only become a more proficient programmer but also a more effective software architect, capable of designing robust and scalable systems.