Understanding Heap Data Structures for Coding Interviews: A Comprehensive Guide
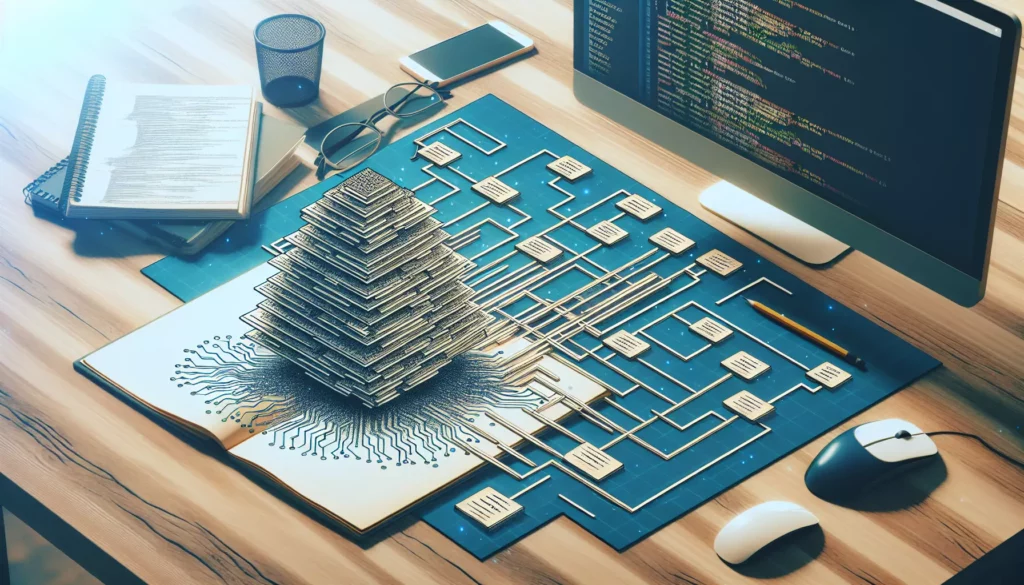
In the world of computer science and coding interviews, particularly those conducted by major tech companies like FAANG (Facebook, Amazon, Apple, Netflix, and Google), understanding data structures is crucial. Among these, the heap data structure stands out as a fundamental concept that often appears in technical interviews and real-world programming scenarios. This comprehensive guide will delve deep into heap data structures, their implementation, operations, and common interview questions, providing you with the knowledge and confidence to ace your next coding interview.
Table of Contents
- What is a Heap?
- Types of Heaps
- Heap Properties
- Heap Operations
- Implementing a Heap
- Applications of Heaps
- Common Heap Interview Questions
- Heaps vs. Other Data Structures
- Heap Optimization Techniques
- Conclusion
1. What is a Heap?
A heap is a specialized tree-based data structure that satisfies the heap property. It’s a complete binary tree where each node is either greater than or equal to (max-heap) or less than or equal to (min-heap) its child nodes. Heaps are commonly used to implement priority queues and are essential in various algorithms, such as Heap Sort and Dijkstra’s shortest path algorithm.
2. Types of Heaps
There are two main types of heaps:
2.1 Max Heap
In a max heap, the parent node is always greater than or equal to its children. The root node contains the maximum element in the heap.
2.2 Min Heap
In a min heap, the parent node is always less than or equal to its children. The root node contains the minimum element in the heap.
3. Heap Properties
Heaps have several important properties that distinguish them from other tree-based data structures:
- Shape Property: A heap is a complete binary tree, meaning all levels are fully filled except possibly the last level, which is filled from left to right.
- Heap Property: The heap property dictates the relationship between parent and child nodes (max-heap or min-heap).
- Efficient Access: The maximum (for max-heap) or minimum (for min-heap) element can be accessed in O(1) time.
- Efficient Insertion and Deletion: Both operations can be performed in O(log n) time.
4. Heap Operations
Understanding the basic operations of a heap is crucial for implementing and working with this data structure effectively. Let’s explore the key operations:
4.1 Insertion
To insert an element into a heap:
- Add the element to the next available position to maintain the complete binary tree property.
- Compare the inserted element with its parent and swap if necessary (heapify-up).
- Repeat step 2 until the heap property is satisfied.
4.2 Deletion (Extract Max/Min)
To remove the root element (maximum in max-heap or minimum in min-heap):
- Replace the root with the last element in the heap.
- Remove the last element.
- Compare the new root with its children and swap if necessary (heapify-down).
- Repeat step 3 until the heap property is satisfied.
4.3 Peek
Return the root element without removing it. This operation takes O(1) time.
4.4 Heapify
Convert an array into a heap. This can be done in O(n) time using the bottom-up approach.
5. Implementing a Heap
Let’s implement a basic max heap in Python to illustrate these concepts:
class MaxHeap:
def __init__(self):
self.heap = []
def parent(self, i):
return (i - 1) // 2
def left_child(self, i):
return 2 * i + 1
def right_child(self, i):
return 2 * i + 2
def swap(self, i, j):
self.heap[i], self.heap[j] = self.heap[j], self.heap[i]
def insert(self, key):
self.heap.append(key)
self._heapify_up(len(self.heap) - 1)
def _heapify_up(self, i):
parent = self.parent(i)
if i > 0 and self.heap[i] > self.heap[parent]:
self.swap(i, parent)
self._heapify_up(parent)
def extract_max(self):
if len(self.heap) == 0:
return None
if len(self.heap) == 1:
return self.heap.pop()
max_val = self.heap[0]
self.heap[0] = self.heap.pop()
self._heapify_down(0)
return max_val
def _heapify_down(self, i):
max_index = i
left = self.left_child(i)
right = self.right_child(i)
if left < len(self.heap) and self.heap[left] > self.heap[max_index]:
max_index = left
if right < len(self.heap) and self.heap[right] > self.heap[max_index]:
max_index = right
if i != max_index:
self.swap(i, max_index)
self._heapify_down(max_index)
def peek(self):
if len(self.heap) == 0:
return None
return self.heap[0]
This implementation provides the basic structure and operations of a max heap. You can use this as a starting point and modify it to create a min heap or add additional functionality as needed.
6. Applications of Heaps
Heaps are versatile data structures with numerous applications in computer science and software engineering. Some common use cases include:
- Priority Queues: Heaps are often used to implement priority queues, where elements with higher priority are served before elements with lower priority.
- Heap Sort: An efficient sorting algorithm with a time complexity of O(n log n).
- Graph Algorithms: Used in algorithms like Dijkstra’s shortest path and Prim’s minimum spanning tree.
- Memory Management: In some systems, heaps are used for memory allocation and garbage collection.
- Median Maintenance: Efficiently find the median of a stream of numbers.
- K-th Largest/Smallest Element: Quickly find the k-th largest or smallest element in an unsorted array.
7. Common Heap Interview Questions
To help you prepare for coding interviews, here are some common heap-related questions you might encounter:
7.1 Kth Largest Element in an Array
Problem: Find the k-th largest element in an unsorted array.
Solution approach: Use a min-heap of size k. Iterate through the array, adding elements to the heap. If the heap size exceeds k, remove the smallest element. The top of the heap will be the k-th largest element.
import heapq
def findKthLargest(nums, k):
heap = []
for num in nums:
heapq.heappush(heap, num)
if len(heap) > k:
heapq.heappop(heap)
return heap[0]
7.2 Merge K Sorted Lists
Problem: Merge k sorted linked lists into one sorted list.
Solution approach: Use a min-heap to keep track of the smallest element from each list. Continuously pop the smallest element and add it to the result list.
import heapq
class ListNode:
def __init__(self, val=0, next=None):
self.val = val
self.next = next
def mergeKLists(lists):
heap = []
dummy = ListNode(0)
current = dummy
# Add the first node of each list to the heap
for i, lst in enumerate(lists):
if lst:
heapq.heappush(heap, (lst.val, i, lst))
while heap:
val, i, node = heapq.heappop(heap)
current.next = ListNode(val)
current = current.next
if node.next:
heapq.heappush(heap, (node.next.val, i, node.next))
return dummy.next
7.3 Top K Frequent Elements
Problem: Given an array of integers, find the k most frequent elements.
Solution approach: Use a hash map to count frequencies, then use a heap to find the k elements with the highest frequencies.
from collections import Counter
import heapq
def topKFrequent(nums, k):
count = Counter(nums)
return heapq.nlargest(k, count.keys(), key=count.get)
8. Heaps vs. Other Data Structures
Understanding how heaps compare to other data structures can help you choose the right tool for specific problems. Here’s a comparison:
8.1 Heap vs. Binary Search Tree (BST)
- Structure: Heaps are complete binary trees, while BSTs can be unbalanced.
- Order: Heaps maintain a partial order, BSTs maintain a total order.
- Operations: Heaps excel at finding min/max, BSTs are better for searching specific elements.
- Time Complexity: Heap operations are generally O(log n), similar to balanced BSTs.
8.2 Heap vs. Array
- Access: Arrays allow random access, heaps do not.
- Insertion/Deletion: Heaps maintain order efficiently, arrays require shifting elements.
- Finding Min/Max: Heaps find min/max in O(1), arrays require O(n) search.
8.3 Heap vs. Linked List
- Memory: Heaps can be implemented using arrays, potentially more memory-efficient than linked lists.
- Operations: Heaps offer faster insertion and deletion of the min/max element.
- Traversal: Linked lists allow easier sequential access.
9. Heap Optimization Techniques
To improve the performance of heap operations in certain scenarios, consider these optimization techniques:
9.1 Decrease Key Operation
Implement a “decrease key” operation to efficiently update priorities in a min-heap. This is useful in algorithms like Dijkstra’s shortest path.
9.2 Fibonacci Heap
For advanced applications, consider using a Fibonacci heap, which offers amortized O(1) time for several operations, including decrease key.
9.3 Binary Heap Implementation
Use an array-based implementation of a binary heap for cache efficiency and to reduce memory overhead.
9.4 Lazy Deletion
In scenarios with frequent deletions, consider marking elements as deleted instead of removing them immediately, and periodically rebuilding the heap.
10. Conclusion
Mastering heap data structures is essential for excelling in coding interviews and solving complex algorithmic problems efficiently. By understanding the fundamental concepts, operations, and applications of heaps, you’ll be well-equipped to tackle a wide range of programming challenges.
Remember to practice implementing heaps from scratch and solving related problems to reinforce your understanding. As you continue your journey in coding education and skills development, heaps will prove to be a valuable tool in your algorithmic toolkit.
Keep exploring, practicing, and refining your skills with platforms like AlgoCademy, which offer interactive coding tutorials and AI-powered assistance to help you progress from beginner-level coding to mastering advanced concepts like heap data structures. With dedication and consistent practice, you’ll be well-prepared to tackle even the most challenging technical interviews at major tech companies.