Understanding Heap and Priority Queue Implementations: A Comprehensive Guide
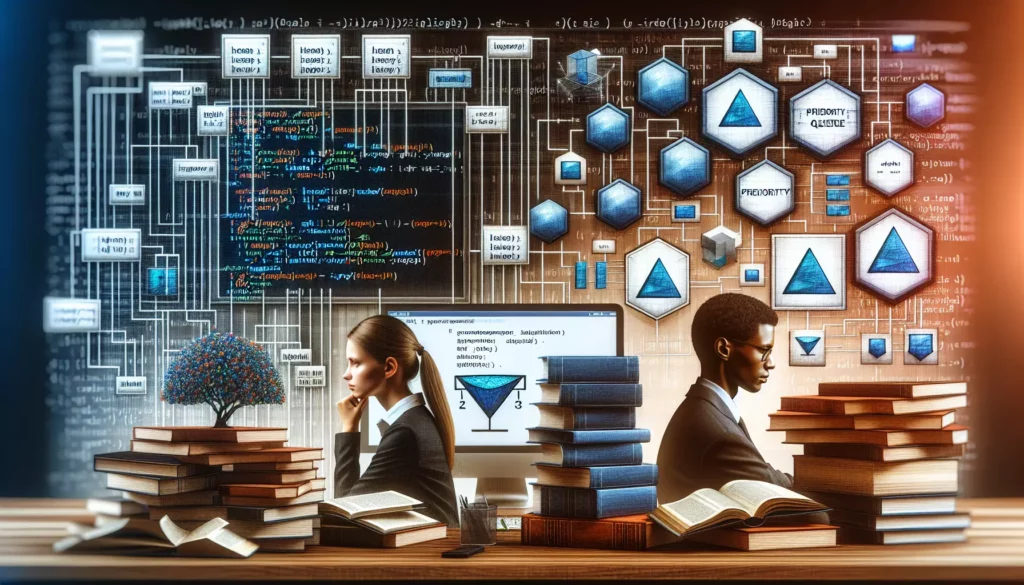
In the world of data structures and algorithms, heaps and priority queues play a crucial role in solving a wide range of problems efficiently. Whether you’re preparing for technical interviews at top tech companies or simply looking to enhance your coding skills, understanding these concepts is essential. In this comprehensive guide, we’ll dive deep into heap and priority queue implementations, exploring their characteristics, operations, and real-world applications.
What is a Heap?
A heap is a specialized tree-based data structure that satisfies the heap property. There are two types of heaps:
- Max Heap: The parent node is always greater than or equal to its children.
- Min Heap: The parent node is always smaller than or equal to its children.
Heaps are commonly implemented as binary trees, where each node has at most two children. The beauty of a heap lies in its ability to maintain a partially ordered structure, which allows for efficient insertion and removal of elements.
Key Properties of a Heap
- Shape Property: A heap is a complete binary tree, meaning all levels are fully filled except possibly the last level, which is filled from left to right.
- Heap Property: The key stored in each node is either greater than or equal to (max heap) or less than or equal to (min heap) the keys in the node’s children.
Implementing a Heap
While heaps can be implemented using node-based structures, they are most commonly implemented using arrays. This approach offers several advantages, including simplicity and memory efficiency. Let’s explore how to implement a max heap using an array in Python:
class MaxHeap:
def __init__(self):
self.heap = []
def parent(self, i):
return (i - 1) // 2
def left_child(self, i):
return 2 * i + 1
def right_child(self, i):
return 2 * i + 2
def swap(self, i, j):
self.heap[i], self.heap[j] = self.heap[j], self.heap[i]
def insert(self, key):
self.heap.append(key)
self._heapify_up(len(self.heap) - 1)
def _heapify_up(self, i):
parent = self.parent(i)
if i > 0 and self.heap[i] > self.heap[parent]:
self.swap(i, parent)
self._heapify_up(parent)
def extract_max(self):
if len(self.heap) == 0:
return None
if len(self.heap) == 1:
return self.heap.pop()
max_val = self.heap[0]
self.heap[0] = self.heap.pop()
self._heapify_down(0)
return max_val
def _heapify_down(self, i):
max_index = i
left = self.left_child(i)
right = self.right_child(i)
if left < len(self.heap) and self.heap[left] > self.heap[max_index]:
max_index = left
if right < len(self.heap) and self.heap[right] > self.heap[max_index]:
max_index = right
if max_index != i:
self.swap(i, max_index)
self._heapify_down(max_index)
This implementation provides the basic structure and operations of a max heap. Let’s break down the key methods:
insert(key)
: Adds a new element to the heap and maintains the heap property by calling_heapify_up
.extract_max()
: Removes and returns the maximum element (root) from the heap, then calls_heapify_down
to restore the heap property._heapify_up(i)
: Moves an element up the heap to its correct position after insertion._heapify_down(i)
: Moves an element down the heap to its correct position after extraction.
Time Complexity of Heap Operations
Understanding the time complexity of heap operations is crucial for assessing their efficiency in different scenarios:
- Insertion: O(log n)
- Extraction (of max/min element): O(log n)
- Peek (get max/min without removal): O(1)
- Heapify (building a heap from an array): O(n)
These efficient time complexities make heaps an excellent choice for many applications, especially when frequent insertions and extractions of the maximum (or minimum) element are required.
What is a Priority Queue?
A priority queue is an abstract data type that operates similarly to a regular queue, with one key difference: each element in a priority queue has an associated priority. Elements with higher priority are dequeued before elements with lower priority. In cases where elements have the same priority, they are typically dequeued based on their order in the queue.
Implementing a Priority Queue using a Heap
While priority queues can be implemented using various data structures, heaps are an excellent choice due to their efficiency. Let’s implement a priority queue using our previously defined max heap:
class PriorityQueue:
def __init__(self):
self.heap = MaxHeap()
def enqueue(self, item, priority):
self.heap.insert((priority, item))
def dequeue(self):
if self.is_empty():
return None
priority, item = self.heap.extract_max()
return item
def peek(self):
if self.is_empty():
return None
return self.heap.heap[0][1]
def is_empty(self):
return len(self.heap.heap) == 0
def size(self):
return len(self.heap.heap)
In this implementation, we use tuples to store both the priority and the item in the heap. The priority is used as the key for comparison in the heap, ensuring that items with higher priority are dequeued first.
Applications of Heaps and Priority Queues
Heaps and priority queues find applications in various algorithms and real-world scenarios. Let’s explore some common use cases:
1. Dijkstra’s Shortest Path Algorithm
Dijkstra’s algorithm uses a priority queue to efficiently find the shortest path in a weighted graph. The priority queue helps in selecting the node with the smallest distance at each step.
2. Huffman Coding
Huffman coding, used in data compression, utilizes a priority queue to build an optimal prefix tree based on character frequencies.
3. Heap Sort
Heap sort is a comparison-based sorting algorithm that uses a binary heap data structure. It has a time complexity of O(n log n) and is in-place, making it efficient for large datasets.
4. Event-driven Simulation
In event-driven simulations, a priority queue can manage events based on their scheduled time, ensuring they are processed in the correct order.
5. Task Scheduling
Operating systems often use priority queues to manage task scheduling, ensuring that high-priority tasks are executed before lower-priority ones.
Advanced Heap Concepts
As you delve deeper into heap implementations, you’ll encounter more advanced concepts and variations. Let’s explore a few of these:
1. Binomial Heaps
Binomial heaps are a more complex heap structure that consists of a collection of binomial trees. They offer efficient merging operations, making them useful in scenarios where combining heaps is frequent.
2. Fibonacci Heaps
Fibonacci heaps provide amortized time complexity improvements over binary heaps for certain operations. They’re particularly efficient for algorithms that involve frequent decrease-key operations, such as Dijkstra’s algorithm with decrease-key optimizations.
3. d-ary Heaps
d-ary heaps generalize binary heaps by allowing each node to have d children instead of just two. This can lead to improved performance in certain scenarios, especially when dealing with external memory.
Implementing a Min Heap
While we’ve focused on max heaps so far, min heaps are equally important and follow similar principles. Here’s a basic implementation of a min heap in Python:
class MinHeap:
def __init__(self):
self.heap = []
def parent(self, i):
return (i - 1) // 2
def left_child(self, i):
return 2 * i + 1
def right_child(self, i):
return 2 * i + 2
def swap(self, i, j):
self.heap[i], self.heap[j] = self.heap[j], self.heap[i]
def insert(self, key):
self.heap.append(key)
self._heapify_up(len(self.heap) - 1)
def _heapify_up(self, i):
parent = self.parent(i)
if i > 0 and self.heap[i] < self.heap[parent]:
self.swap(i, parent)
self._heapify_up(parent)
def extract_min(self):
if len(self.heap) == 0:
return None
if len(self.heap) == 1:
return self.heap.pop()
min_val = self.heap[0]
self.heap[0] = self.heap.pop()
self._heapify_down(0)
return min_val
def _heapify_down(self, i):
min_index = i
left = self.left_child(i)
right = self.right_child(i)
if left < len(self.heap) and self.heap[left] < self.heap[min_index]:
min_index = left
if right < len(self.heap) and self.heap[right] < self.heap[min_index]:
min_index = right
if min_index != i:
self.swap(i, min_index)
self._heapify_down(min_index)
The structure and methods are similar to the max heap, with the key difference being the comparison operators in _heapify_up
and _heapify_down
.
Heap Operations in Practice
To better understand how heaps work in practice, let’s walk through a series of operations on a max heap:
max_heap = MaxHeap()
# Insert elements
max_heap.insert(10)
max_heap.insert(5)
max_heap.insert(15)
max_heap.insert(7)
max_heap.insert(20)
print(max_heap.heap) # Output: [20, 15, 10, 5, 7]
# Extract maximum element
max_val = max_heap.extract_max()
print(f"Extracted max: {max_val}") # Output: Extracted max: 20
print(max_heap.heap) # Output: [15, 7, 10, 5]
# Insert more elements
max_heap.insert(30)
max_heap.insert(12)
print(max_heap.heap) # Output: [30, 15, 12, 5, 7, 10]
This example demonstrates how the heap maintains its property through insertions and extractions, always keeping the maximum element at the root.
Common Interview Questions Related to Heaps and Priority Queues
When preparing for technical interviews, especially for top tech companies, it’s crucial to be familiar with heap and priority queue-related problems. Here are some common questions you might encounter:
- Merge K Sorted Lists: Given K sorted linked lists, merge them into a single sorted list using a min heap.
- Find Kth Largest Element: Find the kth largest element in an unsorted array using a min heap.
- Median of Data Stream: Design a data structure that can efficiently insert numbers and find the median of the data set.
- Top K Frequent Elements: Given an array of integers, find the k most frequent elements using a heap.
- Sliding Window Maximum: Given an array and a sliding window size, find the maximum for each window as it slides through the array.
These problems often require a combination of heap operations and other data structures or algorithms. Practice implementing solutions to these problems to solidify your understanding of heaps and priority queues.
Best Practices and Optimization Tips
As you work with heaps and priority queues, keep these best practices and optimization tips in mind:
- Choose the Right Heap Type: Decide between min heap and max heap based on your specific requirements.
- Consider Space Complexity: While heaps are generally space-efficient, be mindful of memory usage in large-scale applications.
- Optimize for Specific Use Cases: For example, if you frequently need to update priorities, consider using a Fibonacci heap.
- Use Built-in Libraries: Many programming languages offer built-in heap implementations. For example, Python’s
heapq
module provides efficient heap operations. - Balance Between Custom Implementation and Built-in Solutions: While implementing your own heap is great for learning, using built-in solutions can be more efficient in production code.
Conclusion
Heaps and priority queues are fundamental data structures that play a crucial role in many algorithms and real-world applications. By understanding their implementation, operations, and use cases, you’ll be better equipped to tackle complex programming challenges and optimize your code for efficiency.
As you continue your journey in coding education and skill development, remember that mastering these concepts is just one piece of the puzzle. Keep practicing, exploring different problem-solving approaches, and applying your knowledge to real-world scenarios. Whether you’re preparing for technical interviews at top tech companies or simply enhancing your algorithmic thinking, a solid grasp of heaps and priority queues will serve you well in your programming career.
Happy coding, and may your algorithms always be efficient and your data structures well-balanced!