Understanding Hash Tables and Their Interview Applications
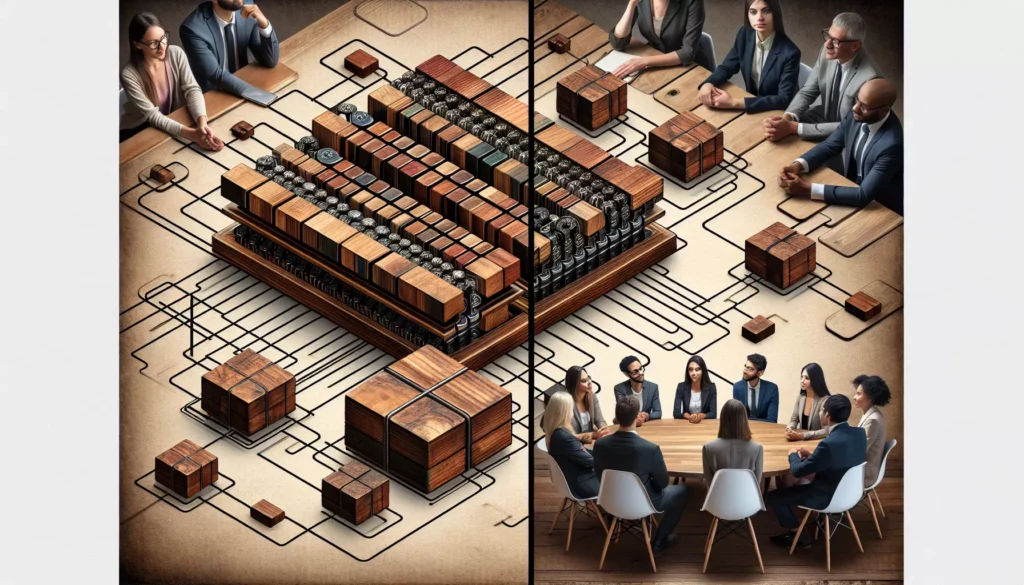
In the world of computer science and programming, hash tables stand out as a fundamental data structure that plays a crucial role in solving various algorithmic problems efficiently. As you progress from beginner-level coding to preparing for technical interviews at major tech companies like FAANG (Facebook, Amazon, Apple, Netflix, Google), understanding hash tables becomes increasingly important. In this comprehensive guide, we’ll dive deep into the concept of hash tables, explore their inner workings, and discuss their applications in common interview questions.
What are Hash Tables?
Hash tables, also known as hash maps, are data structures that implement an associative array abstract data type. They are designed to store key-value pairs and provide fast access to values based on their corresponding keys. The primary advantage of hash tables is their ability to offer constant-time average-case complexity for basic operations like insertion, deletion, and lookup.
The core idea behind hash tables is the use of a hash function to map keys to indices in an array. This mapping allows for quick retrieval of values without the need to search through the entire data structure.
Key Components of a Hash Table
- Array: The underlying storage mechanism for the key-value pairs.
- Hash Function: A function that converts keys into array indices.
- Collision Resolution Mechanism: A method to handle situations where multiple keys map to the same array index.
How Hash Tables Work
Let’s break down the process of how hash tables operate:
1. Hashing
The hash function takes a key as input and produces an integer output, which is used as an index in the array. A good hash function should:
- Be deterministic (same input always produces the same output)
- Distribute keys uniformly across the array
- Be fast to compute
Here’s a simple example of a hash function for string keys:
def hash_function(key, array_size):
hash_value = 0
for char in key:
hash_value += ord(char)
return hash_value % array_size
2. Insertion
To insert a key-value pair:
- Compute the hash value of the key
- Use the hash value as an index in the array
- Store the key-value pair at that index
3. Retrieval
To retrieve a value for a given key:
- Compute the hash value of the key
- Use the hash value as an index in the array
- Return the value stored at that index
4. Collision Handling
Collisions occur when two different keys hash to the same index. There are two main approaches to handle collisions:
a. Chaining
In this method, each array element is a linked list or another data structure. When a collision occurs, the new key-value pair is added to the existing list at that index.
b. Open Addressing
This method involves finding the next available slot in the array when a collision occurs. Common techniques include:
- Linear Probing: Check the next consecutive slot
- Quadratic Probing: Check slots at quadratic intervals
- Double Hashing: Use a second hash function to determine the interval
Implementing a Hash Table in Python
Let’s implement a basic hash table using chaining for collision resolution:
class HashTable:
def __init__(self, size=10):
self.size = size
self.table = [[] for _ in range(self.size)]
def _hash(self, key):
return hash(key) % self.size
def insert(self, key, value):
index = self._hash(key)
for item in self.table[index]:
if item[0] == key:
item[1] = value
return
self.table[index].append([key, value])
def get(self, key):
index = self._hash(key)
for item in self.table[index]:
if item[0] == key:
return item[1]
raise KeyError(key)
def remove(self, key):
index = self._hash(key)
for i, item in enumerate(self.table[index]):
if item[0] == key:
del self.table[index][i]
return
raise KeyError(key)
# Usage example
ht = HashTable()
ht.insert("name", "John")
ht.insert("age", 30)
print(ht.get("name")) # Output: John
ht.remove("name")
try:
ht.get("name")
except KeyError:
print("Key not found") # Output: Key not found
Time Complexity Analysis
The time complexity of hash table operations depends on the quality of the hash function and the load factor (ratio of occupied slots to total slots). Under ideal conditions:
- Insertion: O(1) average case, O(n) worst case
- Deletion: O(1) average case, O(n) worst case
- Lookup: O(1) average case, O(n) worst case
The worst-case scenario occurs when all keys hash to the same index, essentially turning the hash table into a linked list.
Hash Tables in Programming Languages
Many programming languages provide built-in implementations of hash tables:
- Python: dict
- Java: HashMap
- JavaScript: Object and Map
- C++: unordered_map
- Ruby: Hash
These implementations often include optimizations and advanced features beyond basic hash table functionality.
Common Interview Questions Involving Hash Tables
Hash tables are frequently used in coding interviews due to their efficiency in solving various problems. Here are some common types of questions and how hash tables can be applied:
1. Two Sum
Problem: Given an array of integers and a target sum, find two numbers in the array that add up to the target.
Solution using Hash Table:
def two_sum(nums, target):
num_dict = {}
for i, num in enumerate(nums):
complement = target - num
if complement in num_dict:
return [num_dict[complement], i]
num_dict[num] = i
return []
# Example usage
print(two_sum([2, 7, 11, 15], 9)) # Output: [0, 1]
2. First Non-Repeating Character
Problem: Find the first non-repeating character in a string.
Solution using Hash Table:
from collections import Counter
def first_non_repeating_char(s):
char_count = Counter(s)
for char in s:
if char_count[char] == 1:
return char
return None
# Example usage
print(first_non_repeating_char("leetcode")) # Output: l
3. Group Anagrams
Problem: Given an array of strings, group anagrams together.
Solution using Hash Table:
from collections import defaultdict
def group_anagrams(strs):
anagram_groups = defaultdict(list)
for s in strs:
sorted_s = ''.join(sorted(s))
anagram_groups[sorted_s].append(s)
return list(anagram_groups.values())
# Example usage
print(group_anagrams(["eat", "tea", "tan", "ate", "nat", "bat"]))
# Output: [["eat","tea","ate"], ["tan","nat"], ["bat"]]
4. LRU Cache
Problem: Implement a Least Recently Used (LRU) cache with O(1) time complexity for both get and put operations.
Solution using Hash Table and Doubly Linked List:
class Node:
def __init__(self, key=0, value=0):
self.key = key
self.value = value
self.prev = None
self.next = None
class LRUCache:
def __init__(self, capacity: int):
self.capacity = capacity
self.cache = {}
self.head = Node()
self.tail = Node()
self.head.next = self.tail
self.tail.prev = self.head
def get(self, key: int) -> int:
if key in self.cache:
node = self.cache[key]
self._remove(node)
self._add(node)
return node.value
return -1
def put(self, key: int, value: int) -> None:
if key in self.cache:
self._remove(self.cache[key])
node = Node(key, value)
self._add(node)
self.cache[key] = node
if len(self.cache) > self.capacity:
lru = self.head.next
self._remove(lru)
del self.cache[lru.key]
def _remove(self, node):
node.prev.next = node.next
node.next.prev = node.prev
def _add(self, node):
node.prev = self.tail.prev
node.next = self.tail
self.tail.prev.next = node
self.tail.prev = node
# Example usage
cache = LRUCache(2)
cache.put(1, 1)
cache.put(2, 2)
print(cache.get(1)) # Output: 1
cache.put(3, 3)
print(cache.get(2)) # Output: -1 (not found)
cache.put(4, 4)
print(cache.get(1)) # Output: -1 (not found)
print(cache.get(3)) # Output: 3
print(cache.get(4)) # Output: 4
Advanced Topics Related to Hash Tables
As you progress in your understanding of hash tables, consider exploring these advanced topics:
1. Perfect Hashing
Perfect hashing is a technique used when the set of keys is known in advance. It guarantees O(1) worst-case lookup time by creating a collision-free hash table.
2. Cuckoo Hashing
Cuckoo hashing is a scheme for resolving collisions in hash tables that achieves constant-time worst-case complexity for lookups, at the cost of a slightly more complex insertion procedure.
3. Consistent Hashing
Consistent hashing is a distributed hashing scheme that allows hash tables to scale without requiring all keys to be remapped when the number of slots changes.
4. Bloom Filters
Bloom filters are space-efficient probabilistic data structures used to test whether an element is a member of a set. They are based on the principles of hashing and can be used to reduce the number of expensive disk or network lookups.
Conclusion
Hash tables are powerful data structures that offer efficient solutions to many programming problems. Their ability to provide constant-time average-case complexity for basic operations makes them invaluable in various applications, from caching systems to database indexing.
As you prepare for technical interviews, especially for positions at major tech companies, mastering hash tables is crucial. They not only help you solve a wide range of algorithmic problems efficiently but also demonstrate your understanding of fundamental computer science concepts.
Remember to practice implementing hash tables from scratch, analyze their time and space complexities, and solve diverse problems using hash table-based approaches. This comprehensive understanding will significantly boost your problem-solving skills and prepare you for success in technical interviews and real-world programming challenges.
Keep coding, keep learning, and don’t hesitate to dive deeper into the fascinating world of hash tables and other data structures!