Understanding Graph Connectivity and Components
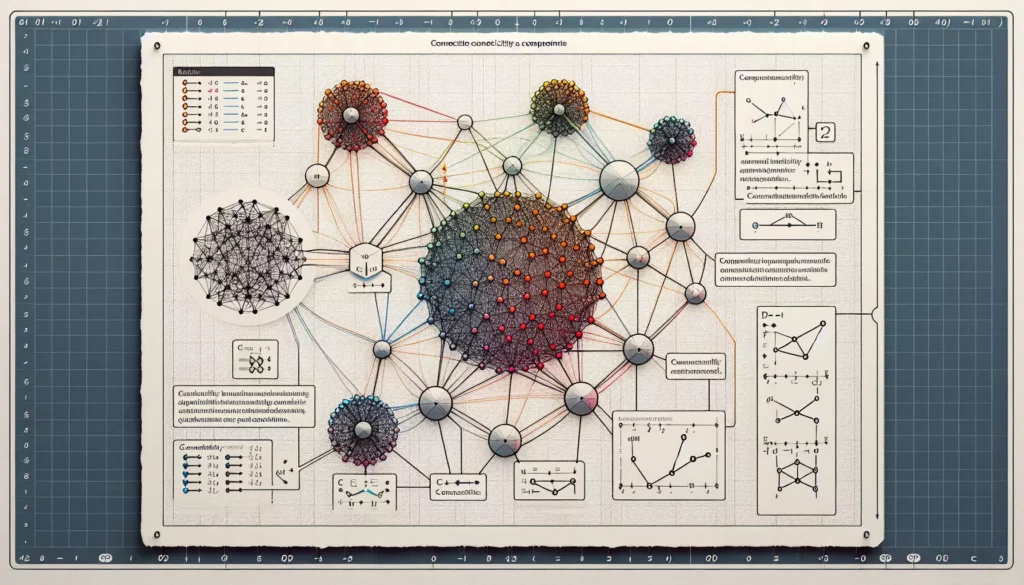
In the world of computer science and algorithms, graphs play a crucial role in representing complex relationships and solving various problems. One of the fundamental aspects of graph theory is understanding connectivity and components. This knowledge is essential for anyone looking to excel in coding interviews, especially for top tech companies like FAANG (Facebook, Amazon, Apple, Netflix, and Google). In this comprehensive guide, we’ll dive deep into graph connectivity and components, exploring their significance, types, and practical applications.
What is Graph Connectivity?
Graph connectivity refers to the property of a graph that determines how well-connected its vertices are. In simple terms, it measures the minimum number of elements (vertices or edges) that need to be removed to disconnect the remaining vertices from each other. Understanding graph connectivity is crucial for analyzing the robustness and reliability of networks, as well as solving various algorithmic problems.
Types of Connectivity
There are two main types of connectivity in graphs:
- Vertex Connectivity: The minimum number of vertices that need to be removed to disconnect the graph.
- Edge Connectivity: The minimum number of edges that need to be removed to disconnect the graph.
It’s important to note that for any graph, the edge connectivity is always less than or equal to the vertex connectivity.
Graph Components
Graph components are subgraphs of a larger graph that are connected within themselves but disconnected from other parts of the graph. Understanding components is crucial for analyzing the structure of complex networks and developing efficient algorithms.
Types of Components
There are several types of components in graph theory:
- Connected Components: Maximal connected subgraphs in an undirected graph.
- Strongly Connected Components (SCCs): Maximal strongly connected subgraphs in a directed graph.
- Weakly Connected Components: Connected components in the underlying undirected graph of a directed graph.
- Biconnected Components: Maximal biconnected subgraphs in an undirected graph.
Algorithms for Finding Components
Several algorithms can be used to find different types of components in graphs. Let’s explore some of the most common ones:
1. Depth-First Search (DFS) for Connected Components
Depth-First Search is a fundamental graph traversal algorithm that can be used to find connected components in an undirected graph. Here’s a simple implementation in Python:
def dfs(graph, vertex, visited):
visited[vertex] = True
for neighbor in graph[vertex]:
if not visited[neighbor]:
dfs(graph, neighbor, visited)
def find_connected_components(graph):
visited = {v: False for v in graph}
components = []
for vertex in graph:
if not visited[vertex]:
component = []
dfs(graph, vertex, visited)
for v in visited:
if visited[v]:
component.append(v)
visited[v] = False
components.append(component)
return components
# Example usage
graph = {
0: [1, 2],
1: [0, 2],
2: [0, 1],
3: [4],
4: [3],
5: []
}
print(find_connected_components(graph))
# Output: [[0, 1, 2], [3, 4], [5]]
2. Kosaraju’s Algorithm for Strongly Connected Components
Kosaraju’s algorithm is an efficient method for finding strongly connected components in a directed graph. It uses two passes of DFS to identify the SCCs. Here’s a Python implementation:
from collections import defaultdict
def dfs_first_pass(graph, vertex, visited, stack):
visited[vertex] = True
for neighbor in graph[vertex]:
if not visited[neighbor]:
dfs_first_pass(graph, neighbor, visited, stack)
stack.append(vertex)
def dfs_second_pass(graph, vertex, visited, component):
visited[vertex] = True
component.append(vertex)
for neighbor in graph[vertex]:
if not visited[neighbor]:
dfs_second_pass(graph, neighbor, visited, component)
def kosaraju(graph):
stack = []
visited = {v: False for v in graph}
# First pass: fill the stack
for vertex in graph:
if not visited[vertex]:
dfs_first_pass(graph, vertex, visited, stack)
# Create reversed graph
reversed_graph = defaultdict(list)
for vertex in graph:
for neighbor in graph[vertex]:
reversed_graph[neighbor].append(vertex)
# Second pass: find SCCs
visited = {v: False for v in graph}
sccs = []
while stack:
vertex = stack.pop()
if not visited[vertex]:
component = []
dfs_second_pass(reversed_graph, vertex, visited, component)
sccs.append(component)
return sccs
# Example usage
graph = {
0: [1, 3],
1: [2],
2: [0],
3: [4],
4: [5],
5: [3]
}
print(kosaraju(graph))
# Output: [[0, 2, 1], [3, 5, 4]]
3. Tarjan’s Algorithm for Strongly Connected Components
Tarjan’s algorithm is another efficient method for finding SCCs in a directed graph. It uses a single pass of DFS and is often considered more efficient than Kosaraju’s algorithm. Here’s a Python implementation:
def tarjan(graph):
index = 0
stack = []
on_stack = set()
indexes = {}
lowlinks = {}
sccs = []
def strongconnect(v):
nonlocal index
indexes[v] = index
lowlinks[v] = index
index += 1
stack.append(v)
on_stack.add(v)
for w in graph[v]:
if w not in indexes:
strongconnect(w)
lowlinks[v] = min(lowlinks[v], lowlinks[w])
elif w in on_stack:
lowlinks[v] = min(lowlinks[v], indexes[w])
if lowlinks[v] == indexes[v]:
scc = []
while True:
w = stack.pop()
on_stack.remove(w)
scc.append(w)
if w == v:
break
sccs.append(scc)
for v in graph:
if v not in indexes:
strongconnect(v)
return sccs
# Example usage
graph = {
0: [1, 3],
1: [2],
2: [0],
3: [4],
4: [5],
5: [3]
}
print(tarjan(graph))
# Output: [[5, 4, 3], [2, 1, 0]]
Applications of Graph Connectivity and Components
Understanding graph connectivity and components has numerous practical applications in computer science and real-world problem-solving. Here are some key areas where this knowledge is particularly useful:
1. Network Analysis
In network analysis, connectivity and components play a crucial role in understanding the structure and resilience of various types of networks, such as:
- Social Networks: Identifying communities and influential individuals.
- Computer Networks: Analyzing the robustness and fault-tolerance of network architectures.
- Transportation Networks: Optimizing routes and identifying critical connections.
2. Web Crawling and Search Engines
Search engines use graph connectivity concepts to:
- Discover and index web pages efficiently.
- Identify groups of related pages or websites.
- Implement link analysis algorithms like PageRank.
3. Compiler Design
In compiler optimization, graph connectivity is used for:
- Control flow analysis
- Dead code elimination
- Register allocation
4. Image Processing
Connected component analysis is widely used in image processing for tasks such as:
- Object recognition and counting
- Image segmentation
- Noise reduction
5. Bioinformatics
Graph connectivity concepts are applied in bioinformatics for:
- Protein-protein interaction network analysis
- Gene regulatory network modeling
- Metabolic pathway analysis
Advanced Topics in Graph Connectivity
As you delve deeper into graph theory and algorithms, you’ll encounter more advanced topics related to connectivity and components. Some of these include:
1. Articulation Points and Bridges
Articulation points (or cut vertices) are vertices in a graph whose removal increases the number of connected components. Bridges are edges whose removal increases the number of connected components. Identifying these critical elements is crucial for analyzing the vulnerability of networks.
2. k-Connectivity
A graph is said to be k-connected if it remains connected after removing any k-1 vertices. This concept is important for designing robust networks and analyzing fault tolerance.
3. Graph Expansion
Graph expansion is a measure of how well-connected a graph is, taking into account both the number of edges and the distribution of these edges. Expander graphs have applications in designing efficient communication networks and constructing error-correcting codes.
4. Spectral Graph Theory
Spectral graph theory studies the properties of graphs using the eigenvalues and eigenvectors of matrices associated with the graph, such as the adjacency matrix or the Laplacian matrix. This approach provides powerful tools for analyzing graph connectivity and structure.
Preparing for Coding Interviews
When preparing for coding interviews, especially for top tech companies, it’s essential to have a solid understanding of graph connectivity and components. Here are some tips to help you excel in this area:
- Master the Basics: Ensure you have a strong grasp of fundamental graph concepts, including representation (adjacency list vs. adjacency matrix), traversal algorithms (DFS and BFS), and basic properties.
- Practice Implementation: Implement the algorithms discussed in this article (DFS for connected components, Kosaraju’s algorithm, Tarjan’s algorithm) from scratch. This will help you understand the intricacies of each algorithm and improve your coding skills.
- Solve Related Problems: Work on problems that involve graph connectivity and components on platforms like LeetCode, HackerRank, or CodeForces. Some common problem types include:
- Finding the number of islands in a 2D grid
- Detecting cycles in a graph
- Topological sorting of a directed acyclic graph (DAG)
- Finding the shortest path between two nodes
- Analyze Time and Space Complexity: For each algorithm you implement or problem you solve, make sure you understand the time and space complexity. Be prepared to discuss trade-offs between different approaches.
- Explore Real-world Applications: Familiarize yourself with practical applications of graph connectivity and components in various domains. This will help you demonstrate the relevance of your knowledge during interviews.
- Study Advanced Topics: While not always necessary for interviews, having knowledge of advanced topics like articulation points, k-connectivity, or spectral graph theory can set you apart from other candidates and demonstrate your depth of understanding.
Conclusion
Graph connectivity and components are fundamental concepts in computer science and algorithm design. They provide powerful tools for analyzing and solving complex problems across various domains. By mastering these concepts and related algorithms, you’ll be well-equipped to tackle challenging coding interview questions and contribute to real-world applications in software development.
Remember that understanding the theory is just the first step. To truly excel in coding interviews and your career as a software developer, you need to practice implementing these concepts, solve diverse problems, and stay curious about their applications in different fields. Keep exploring, coding, and pushing your boundaries to become a proficient graph algorithm expert!