Understanding Fuzzy Logic and Its Applications in Modern Technology
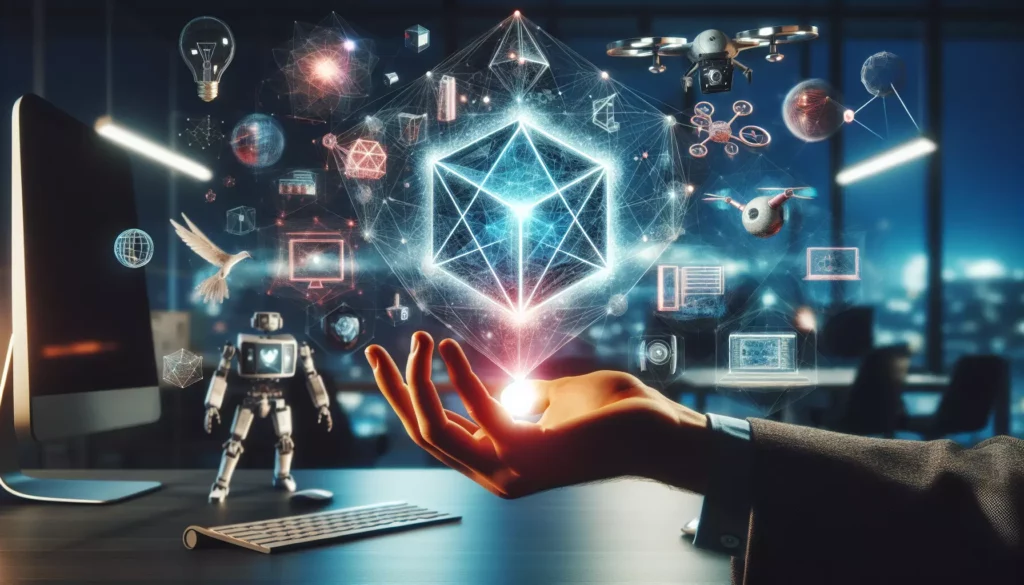
In the realm of computer science and artificial intelligence, fuzzy logic stands out as a powerful tool for handling uncertainty and imprecision. Unlike traditional binary logic, which deals with absolute truths and falsehoods, fuzzy logic embraces the shades of gray that exist in real-world scenarios. This approach to reasoning has found numerous applications across various industries, from consumer electronics to industrial control systems. In this comprehensive guide, we’ll delve into the intricacies of fuzzy logic, explore its fundamental concepts, and examine its wide-ranging applications in modern technology.
What is Fuzzy Logic?
Fuzzy logic is a mathematical approach to computing based on “degrees of truth” rather than the usual “true or false” (1 or 0) Boolean logic on which modern computers are based. It was introduced by Dr. Lotfi Zadeh of the University of California at Berkeley in the 1960s as a means to model the uncertainty of natural language.
At its core, fuzzy logic allows for partial set membership rather than crisp set membership or non-membership. This means that an element can belong to a set to a certain degree, rather than either belonging or not belonging. This concept is particularly useful when dealing with real-world problems where the boundaries between categories are often blurry.
Key Concepts in Fuzzy Logic
- Fuzzy Sets: Unlike classical set theory where an element either belongs to a set or doesn’t, fuzzy sets allow partial membership. An element can belong to a set with a degree of membership ranging from 0 to 1.
- Membership Functions: These are curves that define how each point in the input space is mapped to a membership value between 0 and 1.
- Linguistic Variables: Variables whose values are words or sentences in natural language, rather than numbers.
- Fuzzy Rules: IF-THEN rules that use linguistic variables to describe the relationship between inputs and outputs.
- Defuzzification: The process of converting fuzzy output to a crisp numerical value.
The Mechanics of Fuzzy Logic
To understand how fuzzy logic works, let’s break down its process into steps:
1. Fuzzification
The first step in fuzzy logic is to convert crisp input values into fuzzy values. This is done using membership functions. For example, if we’re dealing with temperature, we might have fuzzy sets for “cold,” “warm,” and “hot.” A temperature of 20°C might have a membership of 0.7 in the “warm” set and 0.3 in the “cold” set.
2. Rule Evaluation
Once the inputs are fuzzified, we apply fuzzy rules. These are typically in the form of IF-THEN statements. For instance:
IF temperature IS warm AND humidity IS high THEN air_conditioning IS medium
The antecedents (IF part) are evaluated using fuzzy logical operations, and the consequent (THEN part) is inferred.
3. Aggregation of Rule Outputs
If multiple rules are triggered, their outputs are combined into a single fuzzy set. This is typically done using a maximum operation or a summation method.
4. Defuzzification
Finally, we need to convert the fuzzy output back into a crisp value. There are several methods for this, including:
- Center of Gravity (COG)
- Mean of Maximum (MOM)
- First of Maximum (FOM)
The choice of method depends on the specific application and desired behavior of the system.
Implementing Fuzzy Logic in Code
To give you a practical understanding of fuzzy logic, let’s implement a simple fuzzy logic system in Python. We’ll use the scikit-fuzzy
library, which provides tools for fuzzy logic operations.
First, you’ll need to install the library:
pip install scikit-fuzzy
Now, let’s create a simple fuzzy logic system that determines the tip amount based on food quality and service quality:
import numpy as np
import skfuzzy as fuzz
from skfuzzy import control as ctrl
# Input variables
food_quality = ctrl.Antecedent(np.arange(0, 11, 1), 'food_quality')
service_quality = ctrl.Antecedent(np.arange(0, 11, 1), 'service_quality')
# Output variable
tip = ctrl.Consequent(np.arange(0, 26, 1), 'tip')
# Membership functions for inputs
food_quality['poor'] = fuzz.trimf(food_quality.universe, [0, 0, 5])
food_quality['average'] = fuzz.trimf(food_quality.universe, [0, 5, 10])
food_quality['excellent'] = fuzz.trimf(food_quality.universe, [5, 10, 10])
service_quality['poor'] = fuzz.trimf(service_quality.universe, [0, 0, 5])
service_quality['average'] = fuzz.trimf(service_quality.universe, [0, 5, 10])
service_quality['excellent'] = fuzz.trimf(service_quality.universe, [5, 10, 10])
# Membership functions for output
tip['low'] = fuzz.trimf(tip.universe, [0, 0, 13])
tip['medium'] = fuzz.trimf(tip.universe, [0, 13, 25])
tip['high'] = fuzz.trimf(tip.universe, [13, 25, 25])
# Fuzzy rules
rule1 = ctrl.Rule(food_quality['poor'] | service_quality['poor'], tip['low'])
rule2 = ctrl.Rule(service_quality['average'], tip['medium'])
rule3 = ctrl.Rule(food_quality['excellent'] | service_quality['excellent'], tip['high'])
# Control system
tipping_ctrl = ctrl.ControlSystem([rule1, rule2, rule3])
tipping = ctrl.ControlSystemSimulation(tipping_ctrl)
# Set input values
tipping.input['food_quality'] = 6.5
tipping.input['service_quality'] = 9.8
# Compute the result
tipping.compute()
print(f"Recommended tip: {tipping.output['tip']:.2f}%")
This code creates a fuzzy logic system that takes food quality and service quality as inputs and produces a recommended tip percentage as output. The system uses triangular membership functions and three simple rules to make its decision.
Applications of Fuzzy Logic
Fuzzy logic has found its way into numerous applications across various industries. Let’s explore some of the most prominent ones:
1. Consumer Electronics
One of the earliest and most successful applications of fuzzy logic was in consumer electronics, particularly in Japan. Companies like Hitachi, Panasonic, and Sharp incorporated fuzzy logic into their products to improve performance and user experience.
- Washing Machines: Fuzzy logic controllers adjust washing time, water level, and detergent amount based on load size, fabric type, and dirt level.
- Air Conditioners: Fuzzy systems control temperature and humidity more efficiently by considering multiple factors like room temperature, outside temperature, and user preferences.
- Cameras: Autofocus systems in cameras use fuzzy logic to determine the best focus point, considering factors like subject movement and lighting conditions.
2. Automotive Industry
Fuzzy logic has made significant contributions to the automotive industry, enhancing both safety and comfort features:
- Anti-lock Braking Systems (ABS): Fuzzy controllers improve braking performance by considering factors like wheel speed, road conditions, and brake pressure.
- Transmission Control: Fuzzy logic helps in determining optimal gear shifting points based on driving conditions and driver behavior.
- Climate Control: Similar to home air conditioners, car climate control systems use fuzzy logic to maintain comfortable cabin temperatures.
3. Industrial Control Systems
Fuzzy logic has proven particularly useful in industrial control applications, where it can handle complex, non-linear processes:
- Chemical Process Control: Fuzzy controllers manage variables like temperature, pressure, and flow rates in chemical reactors.
- Water Treatment Plants: Fuzzy systems optimize the dosing of chemicals and control filtration processes.
- Power Plants: Fuzzy logic helps in load forecasting and maintaining power quality in electrical grids.
4. Financial Systems
The ability of fuzzy logic to handle uncertainty makes it valuable in financial applications:
- Stock Market Prediction: Fuzzy systems can analyze multiple factors to predict market trends.
- Credit Score Assessment: Fuzzy logic can provide more nuanced credit scoring by considering various factors beyond just numerical data.
- Risk Assessment: In insurance and banking, fuzzy logic helps in evaluating risks associated with loans or insurance policies.
5. Medical Diagnosis
Fuzzy logic’s ability to handle imprecise information makes it useful in medical applications:
- Diagnostic Systems: Fuzzy expert systems can assist doctors in diagnosing diseases based on symptoms and test results.
- Medical Imaging: Fuzzy logic enhances image processing techniques used in MRI and CT scans.
- Drug Dosage Control: Fuzzy controllers can help determine optimal drug dosages based on patient characteristics and response.
Advantages and Limitations of Fuzzy Logic
Like any technology, fuzzy logic has its strengths and weaknesses. Understanding these can help in determining when and where to apply fuzzy logic effectively.
Advantages:
- Handling Uncertainty: Fuzzy logic is excellent at dealing with imprecise or uncertain information, making it suitable for real-world problems.
- Intuitive Modeling: Fuzzy rules can be expressed in natural language, making it easier for domain experts to contribute their knowledge.
- Flexibility: Fuzzy systems can be easily modified by adjusting rules or membership functions without rewriting the entire system.
- Non-linear Mapping: Fuzzy logic can model complex, non-linear relationships between inputs and outputs.
- Robustness: Fuzzy systems can often continue to function reasonably well even with noisy or incomplete input data.
Limitations:
- Lack of Precision: While great for approximation, fuzzy logic may not be suitable for applications requiring high precision.
- Complexity: As the number of inputs increases, the number of rules can grow exponentially, making the system difficult to manage.
- No Learning Capability: Unlike neural networks, traditional fuzzy systems don’t have built-in learning mechanisms. They rely on expert knowledge for rule creation.
- Validation and Testing: It can be challenging to rigorously test and validate fuzzy systems, especially for safety-critical applications.
- Computational Overhead: Fuzzy systems may require more computational resources compared to traditional crisp logic systems.
Future Directions and Emerging Trends
As technology continues to evolve, fuzzy logic is finding new applications and being combined with other AI techniques to create more powerful and flexible systems. Some emerging trends include:
1. Neuro-Fuzzy Systems
These hybrid systems combine the learning capabilities of neural networks with the interpretability of fuzzy logic. This allows for systems that can learn from data while still maintaining the transparency of fuzzy rules.
2. Type-2 Fuzzy Logic
Type-2 fuzzy sets introduce an additional degree of uncertainty, allowing for even better modeling of real-world imprecision. This is particularly useful in applications with high levels of uncertainty or where expert opinions differ.
3. Fuzzy Logic in Big Data and IoT
As the Internet of Things (IoT) generates vast amounts of data, fuzzy logic can play a crucial role in making sense of this data and enabling smart decision-making in complex environments.
4. Explainable AI
With growing concerns about the “black box” nature of some AI systems, fuzzy logic’s interpretability makes it an attractive option for creating more transparent and explainable AI models.
5. Quantum Fuzzy Logic
Researchers are exploring the integration of fuzzy logic with quantum computing, potentially leading to more powerful and efficient fuzzy systems.
Conclusion
Fuzzy logic represents a paradigm shift in how we approach problem-solving and decision-making in computing. By embracing the inherent uncertainty and imprecision of the real world, fuzzy logic provides a powerful tool for creating more intelligent and adaptive systems.
From washing machines to financial prediction models, fuzzy logic has demonstrated its versatility and effectiveness across a wide range of applications. As we continue to push the boundaries of artificial intelligence and tackle increasingly complex problems, the principles of fuzzy logic will undoubtedly play a crucial role in shaping the future of technology.
For aspiring programmers and AI enthusiasts, understanding fuzzy logic opens up new avenues for creating more nuanced and human-like reasoning in software systems. Whether you’re developing control systems, working on data analysis, or exploring the frontiers of AI, fuzzy logic offers valuable tools and perspectives that can enhance your problem-solving toolkit.
As we’ve seen through the examples and applications discussed, fuzzy logic is not just a theoretical concept but a practical approach with real-world impact. By mastering fuzzy logic, you’ll be better equipped to handle the complexities and uncertainties inherent in many technological challenges, positioning yourself at the forefront of innovation in computer science and artificial intelligence.