Understanding For Loops: From While to For
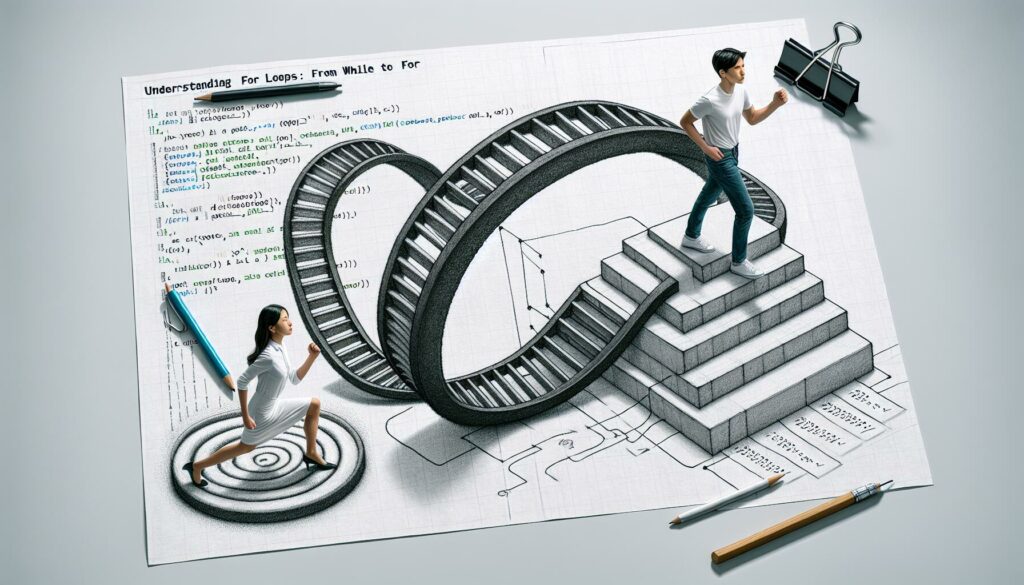
For loops are a fundamental construct in many programming languages, including JavaScript and C-style languages. They provide a concise way to iterate over a sequence of values or perform a set of instructions repeatedly. In this post, we’ll break down how a for loop relates to a while loop, helping you understand its structure and functionality.
The Anatomy of a While Loop
Let’s start with a basic while loop structure:
initialization;
while (condition) {
// do stuff
// go to next step
}
This loop consists of three main parts:
- Initialization: Sets up the initial state before the loop begins.
- Condition: Checked before each iteration to determine if the loop should continue.
- Next step: Updates the state at the end of each iteration.
Transforming While to For
A for loop encapsulates these same three components in a more compact syntax:
for (initialization; condition; go to next step) {
// do stuff
}
Let’s break down how this transformation works:
- The initialization is moved inside the parentheses at the beginning.
- The condition remains in the middle, just like in the while loop.
- The “go to next step” part is placed at the end of the parentheses.
This structure allows us to see all the loop control elements in one line, making the code more readable and compact.
Example: Counting from 1 to 5
To illustrate this transformation, let’s look at a simple example of counting from 1 to 5:
While loop version:
let i = 1;
while (i <= 5) {
console.log(i);
i++;
}
For loop version:
for (let i = 1; i <= 5; i++) {
console.log(i);
}
As you can see, the for loop version is more concise and groups the loop control elements together.
Conclusion
Understanding the relationship between while loops and for loops can help you write more efficient and readable code. The for loop syntax provides a streamlined way to express iterative processes, especially when you know the number of iterations in advance or when working with arrays and other sequences.
Remember, while both loops can often be used interchangeably, for loops are typically preferred when the number of iterations is known beforehand, while while loops are more suitable for situations where the termination condition is less predictable.
Practice transforming while loops into for loops to become more comfortable with this powerful programming construct!
Advanced Uses of For Loops
While many beginners primarily use for loops to iterate through arrays, their versatility extends far beyond this basic application. Let’s explore some advanced uses:
1. Nested Loops
Nested loops are crucial for working with multi-dimensional data or solving complex problems. Here’s an example that prints a simple multiplication table:
javascriptCopyfor (let i = 1; i <= 5; i++) {
for (let j = 1; j <= 5; j++) {
console.log(`${i} x ${j} = ${i * j}`);
}
console.log('---');
}
2. Iterating Over Object Properties
You can use a for…in loop to iterate over object properties:
javascriptCopyconst person = { name: 'Alice', age: 30, city: 'New York' };
for (let key in person) {
console.log(`${key}: ${person[key]}`);
}
3. Iterating With a Step
You can modify the step size in a for loop:
javascriptCopy// Count by 2s
for (let i = 0; i <= 10; i += 2) {
console.log(i);
}
4. Reverse Iteration
For loops can easily count backwards:
javascriptCopyfor (let i = 10; i > 0; i--) {
console.log(i);
}
5. Multiple Counters
You can use multiple counters in a single for loop:
javascriptCopyfor (let i = 0, j = 10; i < 10; i++, j--) {
console.log(i, j);
}
Common Issues Beginners Face
Many bootcamp graduates and beginners struggle with for loops beyond simple array iteration. Here are some common issues and how to address them:
- Understanding the Loop Components: Beginners often struggle to grasp how the initialization, condition, and increment parts of a for loop work together. Practice breaking down each component and tracing the loop’s execution can help.
- Infinite Loops: Forgetting to increment the loop variable or setting an incorrect condition can lead to infinite loops. Always double-check these elements.
- Off-by-One Errors: Mistakes in loop boundaries (starting at 0 vs 1, using < vs <=) are common. Understanding array indexing and being careful with loop conditions can prevent these errors.
- Nested Loop Complexity: Many beginners find nested loops confusing. Start with simple examples and gradually increase complexity. Visualizing the iterations can be helpful.
- Modifying Loop Variables: Changing the loop variable within the loop body can lead to unexpected behavior. Generally, it’s best to avoid this unless you have a specific reason.
- Performance Considerations: Beginners might not consider the performance impact of nested loops or unnecessary iterations. Learning to optimize loops is an important skill.
- Loop Scope Issues: Understanding variable scope within loops, especially with
var
vslet
, can be challenging. Usinglet
in for loops helps avoid common pitfalls.
Exercises to Improve For Loop Skills
To become proficient with for loops, practice these exercises:
- Create a loop that generates the Fibonacci sequence up to a given number.
- Use nested loops to create a pattern of asterisks forming a triangle.
- Implement a simple bubble sort algorithm using for loops.
- Create a loop that finds all prime numbers up to a given limit.
- Use a for loop to flatten a nested array structure.
By mastering these advanced uses and understanding common pitfalls, developers can significantly enhance their ability to solve complex problems efficiently.
Remember, the key to mastering for loops is practice and gradually tackling more complex problems. Don’t be afraid to experiment with different loop structures and challenge yourself beyond simple array iterations.