Understanding Event-Driven Architecture: Building Reactive Systems for Modern Applications
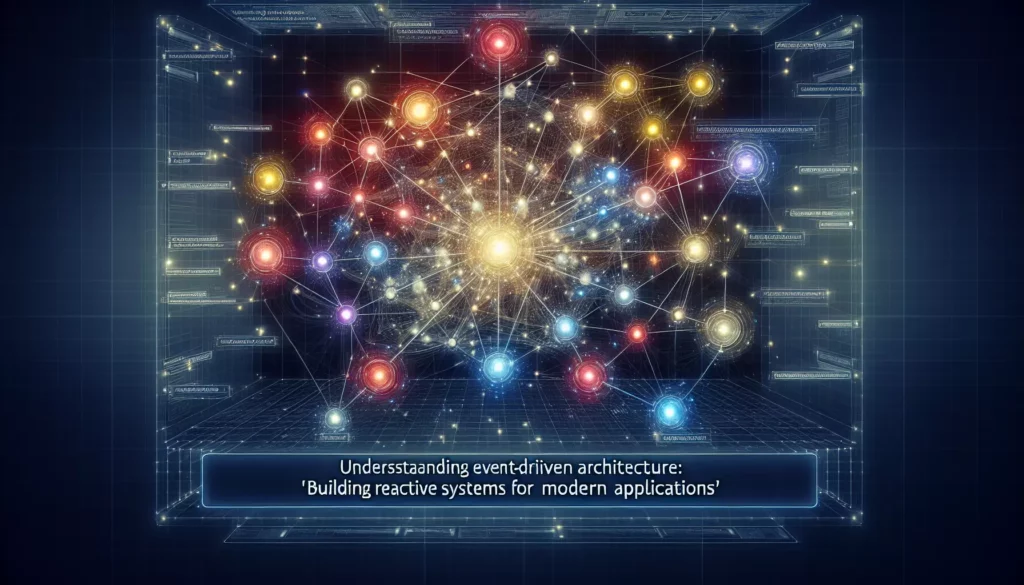
In the ever-evolving landscape of software development, Event-Driven Architecture (EDA) has emerged as a powerful paradigm for building scalable, flexible, and responsive applications. As we delve into the world of coding education and programming skills development, understanding EDA becomes crucial for aspiring developers aiming to create modern, reactive systems. In this comprehensive guide, we’ll explore the fundamentals of Event-Driven Architecture, its benefits, and how it can be applied to build robust applications that can handle the demands of today’s digital ecosystem.
What is Event-Driven Architecture?
Event-Driven Architecture is a software design pattern in which the flow of the program is determined by events such as user actions, sensor outputs, or messages from other programs or threads. In an event-driven system, there are typically three key components:
- Event producers: Components that generate events
- Event channels: Mechanisms for transmitting events
- Event consumers: Components that receive and process events
The core idea behind EDA is that when an event occurs, it triggers a reaction in one or more event consumers. This decoupling of event producers and consumers allows for greater flexibility and scalability in system design.
Key Concepts in Event-Driven Architecture
1. Events
An event is a significant change in state or an important occurrence within a system. Events can be anything from a user clicking a button to a sensor detecting a temperature change. In programming terms, an event is typically represented as a data structure containing information about what happened.
2. Event Producers
Event producers are components or services that generate events. They are responsible for detecting when an event occurs and publishing it to the event channel. Producers are decoupled from consumers, meaning they don’t need to know which components will process the events they generate.
3. Event Channels
Event channels, also known as event buses or message brokers, are the communication mechanisms that transport events from producers to consumers. Popular examples include Apache Kafka, RabbitMQ, and Amazon Simple Queue Service (SQS).
4. Event Consumers
Event consumers are components that subscribe to specific types of events and react when they receive them. Consumers can perform various actions in response to events, such as updating a database, sending notifications, or triggering other processes.
5. Event-Driven Processing
Event-driven processing refers to the way systems handle events asynchronously. Instead of waiting for a response after each action, event-driven systems can continue processing other tasks while waiting for events to occur.
Benefits of Event-Driven Architecture
Adopting an Event-Driven Architecture offers several advantages for modern application development:
1. Scalability
EDA allows systems to scale more easily by decoupling components. Event producers and consumers can be scaled independently based on their specific load requirements.
2. Flexibility
Adding new features or modifying existing ones becomes simpler in an event-driven system. New consumers can be added to react to existing events without affecting other parts of the system.
3. Responsiveness
Event-driven systems can respond quickly to changes and user actions, improving the overall user experience.
4. Resilience
By decoupling components, EDA can improve system resilience. If one component fails, it doesn’t necessarily affect the entire system.
5. Real-time Processing
EDA is well-suited for applications that require real-time data processing and updates, such as financial trading systems or IoT applications.
Implementing Event-Driven Architecture
To implement Event-Driven Architecture in your applications, consider the following steps:
1. Identify Events
Start by identifying the key events in your system. These could be user actions, system state changes, or external triggers.
2. Design Event Schema
Create a standardized format for your events. This typically includes information such as event type, timestamp, and relevant data payload.
3. Choose an Event Channel
Select an appropriate event channel or message broker based on your system requirements. Factors to consider include scalability, reliability, and message ordering guarantees.
4. Implement Event Producers
Develop components that can detect and publish events to the chosen event channel. Here’s a simple example in Python using the pika
library to publish events to RabbitMQ:
import pika
import json
connection = pika.BlockingConnection(pika.ConnectionParameters('localhost'))
channel = connection.channel()
channel.queue_declare(queue='event_queue')
event = {
'type': 'user_registered',
'data': {
'user_id': 12345,
'username': 'john_doe',
'email': 'john@example.com'
}
}
channel.basic_publish(exchange='',
routing_key='event_queue',
body=json.dumps(event))
print("Event published")
connection.close()
5. Implement Event Consumers
Create components that can subscribe to and process events from the event channel. Here’s an example of a simple event consumer in Python:
import pika
import json
def callback(ch, method, properties, body):
event = json.loads(body)
print(f"Received event: {event['type']}")
# Process the event here
connection = pika.BlockingConnection(pika.ConnectionParameters('localhost'))
channel = connection.channel()
channel.queue_declare(queue='event_queue')
channel.basic_consume(queue='event_queue',
auto_ack=True,
on_message_callback=callback)
print('Waiting for events. To exit press CTRL+C')
channel.start_consuming()
6. Handle Error Scenarios
Implement error handling and retry mechanisms to ensure reliability in your event-driven system. This might include dead-letter queues for failed events and circuit breakers to handle service failures.
7. Monitor and Scale
Set up monitoring for your event-driven components and be prepared to scale producers, consumers, or event channels as needed to handle increased load.
Event-Driven Architecture Patterns
Several patterns have emerged to address common challenges in event-driven systems:
1. Event Sourcing
Event Sourcing is a pattern where the state of an application is determined by a sequence of events. Instead of storing the current state, the system stores all events that led to that state. This provides a complete audit trail and allows for easy reconstruction of past states.
2. Command Query Responsibility Segregation (CQRS)
CQRS separates the read and write operations of a system. It uses different models for updating and reading information, which can be beneficial for complex domains and high-performance applications.
3. Saga Pattern
The Saga pattern is used to manage transactions and data consistency in distributed systems. It breaks down long-lived transactions into a sequence of smaller, local transactions coordinated through asynchronous messaging.
4. Event Stream Processing
This pattern involves processing a continuous stream of events in real-time. It’s commonly used in scenarios requiring immediate insights or actions based on incoming data, such as fraud detection or real-time analytics.
Challenges in Event-Driven Architecture
While Event-Driven Architecture offers many benefits, it also comes with its own set of challenges:
1. Eventual Consistency
In distributed event-driven systems, achieving immediate consistency across all components can be challenging. Developers need to design their systems to handle eventual consistency and potential data conflicts.
2. Event Versioning
As systems evolve, event schemas may change. Managing different versions of events and ensuring backward compatibility can become complex.
3. Debugging and Testing
Debugging asynchronous, event-driven systems can be more challenging than traditional synchronous applications. Proper logging and monitoring become crucial.
4. Ordering and Idempotency
Ensuring events are processed in the correct order and handling duplicate events (idempotency) are important considerations in event-driven systems.
Tools and Frameworks for Event-Driven Architecture
Several tools and frameworks can help in implementing Event-Driven Architecture:
1. Apache Kafka
A distributed streaming platform that can handle high-throughput, fault-tolerant real-time data feeds.
2. RabbitMQ
A message broker that supports multiple messaging protocols and can be used to implement various messaging patterns.
3. Apache Flink
A stream processing framework for distributed, high-performing, always-available, and accurate data streaming applications.
4. Akka
A toolkit for building highly concurrent, distributed, and resilient message-driven applications for Java and Scala.
5. Spring Cloud Stream
A framework for building highly scalable event-driven microservices connected with shared messaging systems.
Event-Driven Architecture in Practice: A Simple Example
Let’s consider a simple e-commerce scenario to illustrate how Event-Driven Architecture might be applied in practice. We’ll implement a basic order processing system using Python and RabbitMQ.
Setting Up RabbitMQ
First, ensure you have RabbitMQ installed and running. You can install the pika
library to interact with RabbitMQ:
pip install pika
Order Service (Event Producer)
This service will create new orders and publish “OrderCreated” events:
import pika
import json
import uuid
class OrderService:
def __init__(self):
self.connection = pika.BlockingConnection(pika.ConnectionParameters('localhost'))
self.channel = self.connection.channel()
self.channel.queue_declare(queue='order_events')
def create_order(self, user_id, product_id, quantity):
order_id = str(uuid.uuid4())
order = {
'order_id': order_id,
'user_id': user_id,
'product_id': product_id,
'quantity': quantity,
'status': 'created'
}
event = {
'type': 'OrderCreated',
'data': order
}
self.channel.basic_publish(
exchange='',
routing_key='order_events',
body=json.dumps(event)
)
print(f"Order created and event published: {order_id}")
return order_id
def close(self):
self.connection.close()
# Usage
order_service = OrderService()
order_service.create_order('user123', 'product456', 2)
order_service.close()
Inventory Service (Event Consumer)
This service will listen for “OrderCreated” events and update the inventory accordingly:
import pika
import json
class InventoryService:
def __init__(self):
self.connection = pika.BlockingConnection(pika.ConnectionParameters('localhost'))
self.channel = self.connection.channel()
self.channel.queue_declare(queue='order_events')
self.inventory = {} # Simplified inventory storage
def update_inventory(self, product_id, quantity):
if product_id not in self.inventory:
self.inventory[product_id] = 100 # Initial stock
self.inventory[product_id] -= quantity
print(f"Inventory updated for product {product_id}. New stock: {self.inventory[product_id]}")
def process_order_event(self, ch, method, properties, body):
event = json.loads(body)
if event['type'] == 'OrderCreated':
order_data = event['data']
self.update_inventory(order_data['product_id'], order_data['quantity'])
def start_consuming(self):
self.channel.basic_consume(
queue='order_events',
on_message_callback=self.process_order_event,
auto_ack=True
)
print('Inventory Service is waiting for order events. To exit press CTRL+C')
self.channel.start_consuming()
# Usage
inventory_service = InventoryService()
inventory_service.start_consuming()
Running the Example
To run this example, you would start the Inventory Service in one terminal:
python inventory_service.py
And then run the Order Service in another terminal to create orders:
python order_service.py
This simple example demonstrates the core concepts of Event-Driven Architecture:
- The Order Service acts as an event producer, creating orders and publishing events.
- RabbitMQ serves as the event channel, facilitating communication between services.
- The Inventory Service is an event consumer, reacting to order events and updating the inventory.
In a real-world scenario, you might have additional services like a Shipping Service or a Notification Service, all consuming the same events and reacting accordingly. This decoupled architecture allows each service to evolve independently and makes it easy to add new functionality to the system.
Conclusion
Event-Driven Architecture is a powerful approach for building scalable, flexible, and responsive applications. By decoupling components and focusing on the flow of events, developers can create systems that are better equipped to handle the complexities of modern software requirements.
As you continue your journey in coding education and skills development, understanding EDA will be invaluable. It’s a crucial concept for building reactive systems and is widely used in many real-world applications, from e-commerce platforms to IoT systems and beyond.
Remember that while EDA offers many benefits, it also comes with its own set of challenges. Proper design, careful consideration of event schemas, and robust error handling are essential for success. As you practice and gain experience, you’ll develop the skills to leverage EDA effectively in your projects.
Keep exploring, experimenting, and building with event-driven principles, and you’ll be well-prepared to tackle the complex, distributed systems that define modern software architecture. Happy coding!