Understanding Error Handling: Try, Catch, and Finally Blocks
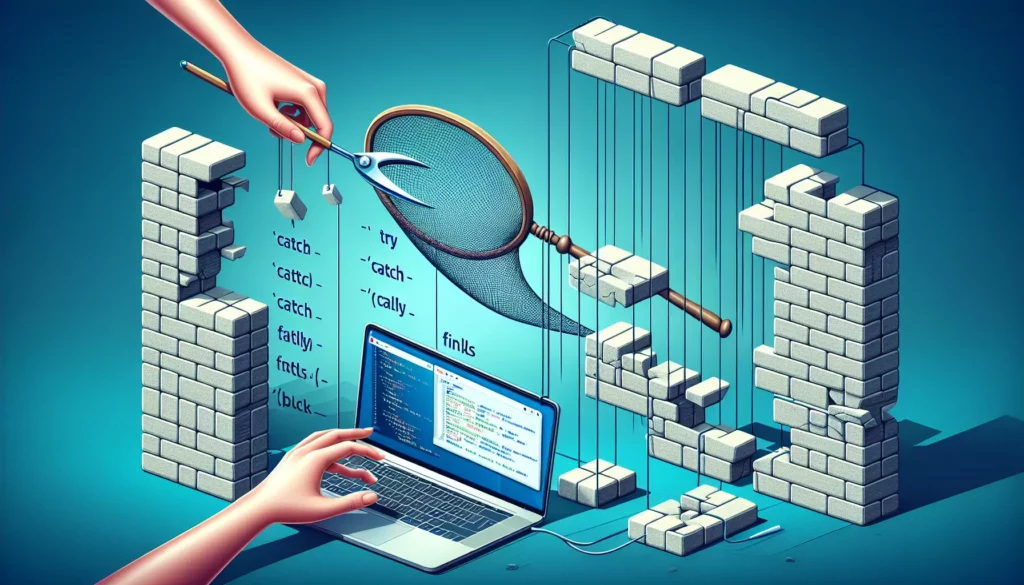
Error handling is a crucial aspect of writing robust and reliable code. As developers, we need to anticipate and gracefully handle potential errors that may occur during program execution. In this comprehensive guide, we’ll explore the concept of error handling, focusing on the try, catch, and finally blocks. We’ll dive deep into their usage, best practices, and how they can improve your code’s reliability and maintainability.
What is Error Handling?
Error handling is the process of anticipating, detecting, and resolving errors or exceptional conditions that may occur during program execution. It’s an essential practice in software development that helps prevent crashes, improves user experience, and makes debugging easier.
In many programming languages, including Java, C#, Python, and JavaScript, error handling is implemented using a mechanism called “exception handling.” This mechanism typically involves three key components: try, catch, and finally blocks.
The Try Block
The try block is used to enclose the code that might throw an exception. It’s the first step in implementing exception handling in your code. Here’s the basic syntax:
try {
// Code that might throw an exception
}
When you suspect that a particular piece of code might cause an error, you wrap it in a try block. This tells the program to monitor this code for potential exceptions.
Example of a Try Block
Let’s look at a simple example in Python where we’re trying to divide two numbers:
try:
numerator = 10
denominator = 0
result = numerator / denominator
print(result)
In this case, we’re attempting to divide by zero, which will raise a ZeroDivisionError in Python. Without proper error handling, this would cause our program to crash.
The Catch Block
The catch block is used to handle the exception that was thrown in the try block. It follows immediately after the try block and specifies the type of exception it can handle. Here’s the basic syntax:
try {
// Code that might throw an exception
} catch (ExceptionType e) {
// Code to handle the exception
}
When an exception occurs in the try block, the program flow immediately transfers to the appropriate catch block. This allows you to gracefully handle the error and prevent your program from crashing.
Example of a Catch Block
Continuing our Python example, let’s add a catch block to handle the ZeroDivisionError:
try:
numerator = 10
denominator = 0
result = numerator / denominator
print(result)
except ZeroDivisionError:
print("Error: Cannot divide by zero")
Now, instead of crashing, our program will print an error message when a division by zero is attempted.
The Finally Block
The finally block is an optional block that follows the try and catch blocks. It contains code that will be executed regardless of whether an exception was thrown or not. Here’s the basic syntax:
try {
// Code that might throw an exception
} catch (ExceptionType e) {
// Code to handle the exception
} finally {
// Code that will always be executed
}
The finally block is often used for cleanup operations, such as closing files or releasing resources, that should be performed whether or not an exception occurred.
Example of a Finally Block
Let’s extend our Python example to include a finally block:
try:
numerator = 10
denominator = 0
result = numerator / denominator
print(result)
except ZeroDivisionError:
print("Error: Cannot divide by zero")
finally:
print("This code will always be executed")
In this case, the message in the finally block will be printed regardless of whether the division was successful or not.
Multiple Catch Blocks
In many programming languages, you can have multiple catch blocks to handle different types of exceptions. This allows you to provide specific handling for different error scenarios. Here’s an example in Java:
try {
// Code that might throw multiple types of exceptions
} catch (ArithmeticException e) {
System.out.println("Arithmetic Exception occurred");
} catch (NullPointerException e) {
System.out.println("Null Pointer Exception occurred");
} catch (Exception e) {
System.out.println("Some other exception occurred");
}
In this example, we’re handling ArithmeticException and NullPointerException specifically, and then providing a catch-all for any other type of Exception.
Best Practices for Error Handling
Now that we understand the basics of try, catch, and finally blocks, let’s explore some best practices for effective error handling:
1. Be Specific with Exception Types
When possible, catch specific exception types rather than using a general Exception catch-all. This allows you to provide more targeted error handling and makes your code more maintainable.
2. Don’t Catch Exceptions You Can’t Handle
Only catch exceptions that you can meaningfully handle. If you can’t do anything useful with an exception, it’s often better to let it propagate up the call stack.
3. Log Exceptions
When catching exceptions, make sure to log them. This can be crucial for debugging and understanding what went wrong in production environments.
4. Use Finally Blocks for Cleanup
Use finally blocks to ensure that resources are properly released, regardless of whether an exception occurred or not. This is particularly important for operations like closing files or database connections.
5. Don’t Ignore Exceptions
Never catch an exception and do nothing. At the very least, log the exception. Ignoring exceptions can lead to silent failures that are difficult to debug.
6. Throw Exceptions at the Appropriate Level
When you catch an exception, consider whether you should handle it at the current level or throw a new, more appropriate exception to be handled higher up in the call stack.
Advanced Error Handling Techniques
As you become more proficient with error handling, you might encounter more advanced techniques and concepts. Let’s explore a few of these:
Custom Exceptions
Many programming languages allow you to create custom exception types. This can be useful when you want to represent application-specific error conditions. Here’s an example in Python:
class CustomError(Exception):
def __init__(self, message):
self.message = message
try:
raise CustomError("This is a custom error")
except CustomError as e:
print(f"Caught custom error: {e.message}")
Exception Chaining
Exception chaining involves catching an exception and then raising a new exception while preserving the original exception information. This can be useful for providing context about where and why an error occurred. Here’s an example in Python:
try:
# Some code that might raise an exception
raise ValueError("Original error")
except ValueError as e:
raise RuntimeError("A runtime error occurred") from e
Context Managers
Many modern programming languages provide context managers, which can be used to automatically handle resource management and error handling. In Python, this is implemented using the ‘with’ statement:
with open('file.txt', 'r') as file:
content = file.read()
# File is automatically closed after this block, even if an exception occurs
Error Handling in Different Programming Languages
While the concepts of try, catch, and finally are common across many programming languages, the exact syntax and capabilities can vary. Let’s look at how error handling is implemented in a few popular languages:
JavaScript
JavaScript uses try, catch, and finally blocks similar to many other languages:
try {
// Code that might throw an error
throw new Error("An error occurred");
} catch (error) {
console.error("Caught an error:", error.message);
} finally {
console.log("This will always execute");
}
Java
Java has a robust exception handling system, including checked and unchecked exceptions:
try {
// Code that might throw an exception
throw new Exception("An exception occurred");
} catch (Exception e) {
System.out.println("Caught exception: " + e.getMessage());
} finally {
System.out.println("This will always execute");
}
C#
C# exception handling is similar to Java:
try
{
// Code that might throw an exception
throw new Exception("An exception occurred");
}
catch (Exception e)
{
Console.WriteLine("Caught exception: " + e.Message);
}
finally
{
Console.WriteLine("This will always execute");
}
Error Handling in Asynchronous Code
Error handling in asynchronous code can be more challenging. Many modern languages provide special syntax for handling errors in asynchronous operations. For example, in JavaScript, you might use async/await with try/catch:
async function fetchData() {
try {
const response = await fetch('https://api.example.com/data');
const data = await response.json();
return data;
} catch (error) {
console.error('Error fetching data:', error);
}
}
Testing Error Handling
It’s important to test your error handling code to ensure it behaves as expected. This often involves deliberately triggering errors and verifying that they’re caught and handled correctly. Here’s an example of a unit test for error handling in Python using the unittest framework:
import unittest
def divide(a, b):
try:
return a / b
except ZeroDivisionError:
return "Cannot divide by zero"
class TestErrorHandling(unittest.TestCase):
def test_divide_by_zero(self):
self.assertEqual(divide(10, 0), "Cannot divide by zero")
def test_normal_division(self):
self.assertEqual(divide(10, 2), 5)
if __name__ == '__main__':
unittest.main()
Conclusion
Error handling is a critical skill for any programmer. By effectively using try, catch, and finally blocks, you can create more robust and reliable code that gracefully handles unexpected situations. Remember to be specific with your exception handling, always log errors, and use finally blocks for cleanup operations.
As you continue to develop your programming skills, pay close attention to error handling. It’s an area where experience and careful thought can significantly improve the quality of your code. Practice implementing error handling in your projects, and don’t be afraid to dive deeper into language-specific error handling features and best practices.
Error handling might seem like a chore at first, but as you become more proficient, you’ll find that it’s an essential tool for writing high-quality, production-ready code. Happy coding, and may your exceptions always be caught!