Understanding End-to-End Application Design: A Comprehensive Guide
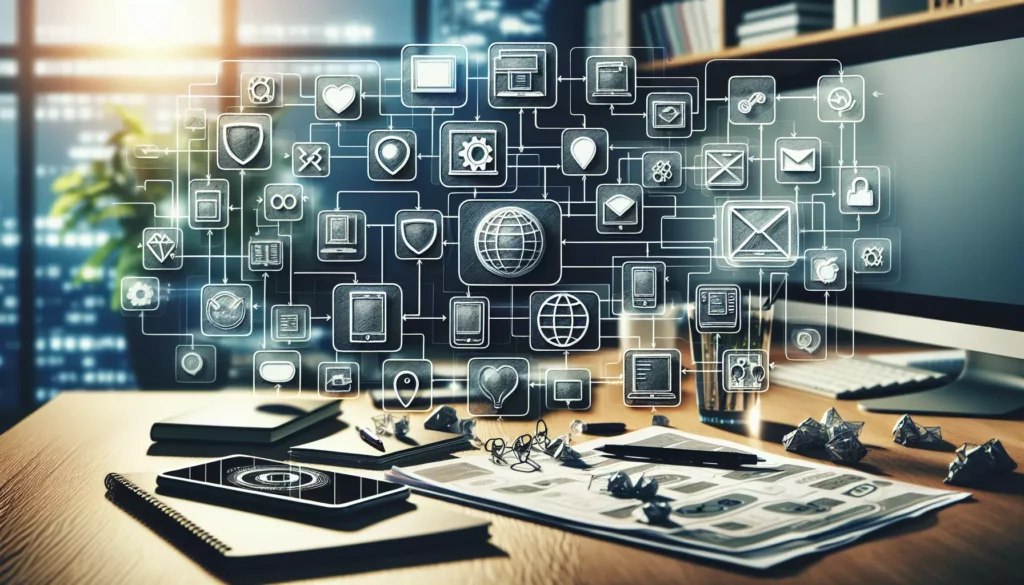
In today’s fast-paced digital world, the ability to design and develop robust, scalable applications is a crucial skill for any software engineer or aspiring programmer. End-to-end application design encompasses the entire process of conceptualizing, planning, implementing, and maintaining a software application from start to finish. This comprehensive guide will delve into the intricacies of end-to-end application design, providing you with the knowledge and insights needed to create successful applications that meet user needs and business requirements.
Table of Contents
- Introduction to End-to-End Application Design
- Gathering and Analyzing Requirements
- Designing the Application Architecture
- Frontend Development
- Backend Development
- Database Design and Management
- API Design and Integration
- Security Considerations
- Testing and Quality Assurance
- Deployment and DevOps
- Maintenance and Scalability
- Conclusion
1. Introduction to End-to-End Application Design
End-to-end application design is a holistic approach to creating software applications that considers every aspect of the development process, from initial concept to final deployment and beyond. This approach ensures that all components of an application work seamlessly together to deliver a cohesive user experience and meet business objectives.
Key aspects of end-to-end application design include:
- Understanding user needs and business requirements
- Designing a robust and scalable architecture
- Developing both frontend and backend components
- Implementing efficient data storage and retrieval mechanisms
- Ensuring security and performance throughout the application
- Testing and quality assurance
- Deployment and ongoing maintenance
By adopting an end-to-end approach, developers can create applications that are not only functional but also maintainable, scalable, and adaptable to changing requirements over time.
2. Gathering and Analyzing Requirements
The first step in end-to-end application design is to gather and analyze requirements. This crucial phase sets the foundation for the entire project and helps ensure that the final product meets the needs of both users and stakeholders.
2.1 User Research
Conduct thorough user research to understand the target audience, their pain points, and their expectations from the application. This can involve:
- Surveys and questionnaires
- User interviews
- Observational studies
- Analyzing existing user data and feedback
2.2 Stakeholder Analysis
Identify and engage with key stakeholders to understand business objectives, constraints, and success criteria. This may include:
- Project sponsors
- Department heads
- Marketing and sales teams
- Legal and compliance officers
2.3 Functional and Non-Functional Requirements
Based on user research and stakeholder input, define both functional and non-functional requirements:
- Functional requirements: Specific features and capabilities the application must have
- Non-functional requirements: Performance, security, scalability, and usability criteria
2.4 Creating User Stories and Use Cases
Translate requirements into user stories and use cases to provide a clear, user-centric view of the application’s functionality. For example:
As a [user type], I want to [action] so that [benefit].
Example: As a registered user, I want to reset my password so that I can regain access to my account if I forget my credentials.
3. Designing the Application Architecture
With requirements in hand, the next step is to design the overall architecture of the application. This involves making high-level decisions about the structure and organization of the system.
3.1 Choosing an Architectural Pattern
Select an appropriate architectural pattern based on the application’s requirements and constraints. Common patterns include:
- Monolithic architecture
- Microservices architecture
- Serverless architecture
- Event-driven architecture
3.2 Defining System Components
Break down the application into logical components or modules, each responsible for specific functionality. This might include:
- User authentication and authorization
- Data processing and storage
- External service integrations
- Reporting and analytics
3.3 Designing Data Flow
Map out how data will flow through the system, from user input to storage and retrieval. Consider:
- Input validation and sanitization
- Data transformation and processing
- Caching strategies
- Consistency and integrity measures
3.4 Technology Stack Selection
Choose appropriate technologies for each component of the application, considering factors such as:
- Team expertise
- Scalability requirements
- Performance needs
- Integration with existing systems
- Long-term maintainability
4. Frontend Development
Frontend development focuses on creating the user interface and experience of the application. This is what users directly interact with and often forms their first impression of the application.
4.1 UI/UX Design
Create wireframes, mockups, and prototypes to visualize the user interface. Consider:
- User flow and navigation
- Responsive design for various devices
- Accessibility standards
- Consistency with brand guidelines
4.2 Choosing a Frontend Framework
Select an appropriate frontend framework or library based on project requirements. Popular options include:
- React
- Vue.js
- Angular
- Svelte
4.3 State Management
Implement a state management solution to handle complex application states. Options include:
- Redux
- MobX
- Vuex (for Vue.js)
- Context API (for React)
4.4 Performance Optimization
Optimize frontend performance to ensure a smooth user experience:
- Implement lazy loading for images and components
- Minimize and bundle assets
- Use caching strategies
- Optimize rendering performance
5. Backend Development
Backend development involves creating the server-side logic, APIs, and data management systems that power the application.
5.1 Choosing a Backend Language and Framework
Select an appropriate backend language and framework based on project requirements and team expertise. Popular choices include:
- Node.js with Express
- Python with Django or Flask
- Ruby on Rails
- Java with Spring Boot
5.2 Implementing Business Logic
Develop the core functionality of the application, including:
- User authentication and authorization
- Data processing and validation
- Integration with external services
- Error handling and logging
5.3 Optimizing Performance
Implement performance optimizations to ensure the backend can handle high loads:
- Implement caching mechanisms
- Optimize database queries
- Use asynchronous processing where appropriate
- Implement rate limiting and request throttling
5.4 Implementing Scalability Measures
Design the backend to be scalable from the start:
- Use horizontal scaling with load balancers
- Implement stateless services
- Use message queues for asynchronous processing
- Consider containerization and orchestration (e.g., Docker and Kubernetes)
6. Database Design and Management
Effective data storage and retrieval are crucial for most applications. Proper database design ensures data integrity, performance, and scalability.
6.1 Choosing a Database System
Select an appropriate database system based on the application’s data requirements:
- Relational databases (e.g., PostgreSQL, MySQL)
- NoSQL databases (e.g., MongoDB, Cassandra)
- Time-series databases (e.g., InfluxDB)
- Graph databases (e.g., Neo4j)
6.2 Data Modeling
Design the data model to efficiently represent and store application data:
- Identify entities and their relationships
- Normalize or denormalize data based on access patterns
- Define indexes for frequently accessed data
- Plan for data growth and evolution
6.3 Query Optimization
Optimize database queries for performance:
- Use appropriate indexing strategies
- Write efficient queries
- Implement query caching
- Use database-specific optimization techniques
6.4 Data Migration and Versioning
Plan for data migrations and schema changes:
- Use database migration tools
- Implement versioning for database schemas
- Plan for backward compatibility
- Develop strategies for zero-downtime migrations
7. API Design and Integration
APIs (Application Programming Interfaces) are crucial for enabling communication between different components of the application and with external services.
7.1 Designing RESTful APIs
Follow REST (Representational State Transfer) principles when designing APIs:
- Use appropriate HTTP methods (GET, POST, PUT, DELETE)
- Design meaningful and consistent URL structures
- Implement proper status codes and error handling
- Version your APIs to manage changes over time
7.2 GraphQL APIs
Consider using GraphQL for more flexible and efficient data fetching:
- Design a schema that represents your data model
- Implement resolvers for each field
- Use query batching and caching to optimize performance
- Implement proper authentication and authorization
7.3 API Documentation
Provide comprehensive documentation for your APIs:
- Use tools like Swagger or OpenAPI for RESTful APIs
- Implement GraphQL introspection for self-documenting schemas
- Include example requests and responses
- Document authentication methods and error handling
7.4 Third-Party API Integration
When integrating with external APIs:
- Implement proper error handling and retries
- Use rate limiting to respect API quotas
- Cache responses when appropriate
- Implement fallback mechanisms for API outages
8. Security Considerations
Security is paramount in modern application design. Implementing robust security measures protects user data and maintains the integrity of the application.
8.1 Authentication and Authorization
Implement secure user authentication and authorization:
- Use secure password hashing algorithms (e.g., bcrypt)
- Implement multi-factor authentication
- Use JSON Web Tokens (JWT) or session-based authentication
- Implement role-based access control (RBAC)
8.2 Data Encryption
Protect sensitive data through encryption:
- Use HTTPS for all communications
- Encrypt sensitive data at rest
- Implement proper key management
- Use secure protocols for data transfer
8.3 Input Validation and Sanitization
Prevent common security vulnerabilities:
- Implement server-side input validation
- Sanitize user inputs to prevent XSS attacks
- Use parameterized queries to prevent SQL injection
- Implement proper CORS (Cross-Origin Resource Sharing) policies
8.4 Security Auditing and Monitoring
Continuously monitor and improve security:
- Implement logging for security-related events
- Regularly perform security audits
- Use automated vulnerability scanning tools
- Stay informed about security best practices and emerging threats
9. Testing and Quality Assurance
Comprehensive testing ensures the reliability, functionality, and performance of the application.
9.1 Unit Testing
Write and maintain unit tests for individual components:
// Example unit test in JavaScript using Jest
describe('User Authentication', () => {
test('should successfully authenticate valid user', async () => {
const user = { username: 'testuser', password: 'password123' };
const result = await authenticateUser(user);
expect(result.success).toBe(true);
expect(result.token).toBeDefined();
});
});
9.2 Integration Testing
Test the interaction between different components:
// Example integration test in Python using pytest
def test_user_registration_and_login():
# Register a new user
response = client.post('/api/register', json={
'username': 'newuser',
'email': 'newuser@example.com',
'password': 'securepassword'
})
assert response.status_code == 201
# Attempt to log in with the new user
response = client.post('/api/login', json={
'username': 'newuser',
'password': 'securepassword'
})
assert response.status_code == 200
assert 'token' in response.json()
9.3 End-to-End Testing
Automate testing of the entire application flow:
- Use tools like Selenium or Cypress for web applications
- Simulate user interactions and verify expected outcomes
- Test across different browsers and devices
9.4 Performance Testing
Ensure the application can handle expected loads:
- Conduct load testing to simulate multiple users
- Perform stress testing to identify breaking points
- Use tools like Apache JMeter or Gatling for performance testing
9.5 Security Testing
Identify and address security vulnerabilities:
- Conduct penetration testing
- Use automated security scanning tools
- Perform regular code reviews with a focus on security
10. Deployment and DevOps
Efficient deployment processes and DevOps practices ensure smooth releases and ongoing maintenance of the application.
10.1 Continuous Integration and Continuous Deployment (CI/CD)
Implement CI/CD pipelines for automated testing and deployment:
- Use tools like Jenkins, GitLab CI, or GitHub Actions
- Automate build, test, and deployment processes
- Implement feature flags for gradual rollouts
10.2 Containerization
Use containerization for consistent deployments:
- Create Docker containers for your application components
- Use Docker Compose for local development environments
- Implement Kubernetes for container orchestration in production
10.3 Infrastructure as Code
Manage infrastructure using code:
- Use tools like Terraform or AWS CloudFormation
- Version control your infrastructure configurations
- Implement immutable infrastructure patterns
10.4 Monitoring and Logging
Implement comprehensive monitoring and logging:
- Use tools like Prometheus and Grafana for metrics
- Implement centralized logging with ELK stack or similar solutions
- Set up alerts for critical issues
11. Maintenance and Scalability
Ongoing maintenance and scalability planning are crucial for the long-term success of the application.
11.1 Regular Updates and Patches
Keep the application up-to-date and secure:
- Regularly update dependencies
- Apply security patches promptly
- Refactor code to improve maintainability
11.2 Performance Optimization
Continuously optimize application performance:
- Profile and identify performance bottlenecks
- Optimize database queries and indexes
- Implement caching strategies at various levels
11.3 Scalability Planning
Plan for future growth and increased load:
- Design for horizontal scalability
- Implement database sharding strategies
- Use content delivery networks (CDNs) for static assets
- Consider serverless architectures for certain components
11.4 Technical Debt Management
Address technical debt regularly:
- Allocate time for refactoring in development cycles
- Maintain comprehensive documentation
- Conduct regular code reviews
- Implement automated code quality checks
12. Conclusion
End-to-end application design is a complex and multifaceted process that requires careful planning, execution, and ongoing maintenance. By following the principles and practices outlined in this guide, you can create robust, scalable, and maintainable applications that meet user needs and business objectives.
Remember that application design is an iterative process. As technology evolves and user needs change, be prepared to adapt and refine your approach. Continuous learning, experimentation, and improvement are key to success in the ever-changing landscape of software development.
Whether you’re building a small web application or a large-scale enterprise system, the principles of end-to-end application design will help you create high-quality software that stands the test of time. By considering all aspects of the application lifecycle, from initial concept to ongoing maintenance, you can deliver value to users and stakeholders while building a solid foundation for future growth and innovation.