Understanding Design Patterns and When to Use Them
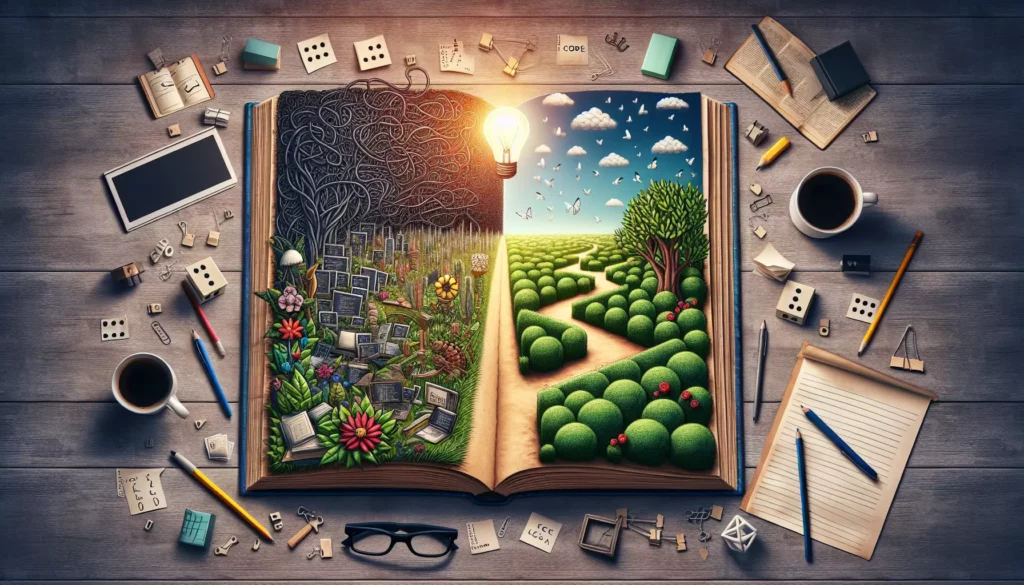
Design patterns are essential tools in a programmer’s toolkit, offering proven solutions to common software design problems. They provide a structured approach to solving recurring issues in software development, making code more maintainable, flexible, and easier to understand. In this comprehensive guide, we’ll explore various design patterns, their categories, and when to use them effectively in your projects.
What Are Design Patterns?
Design patterns are reusable solutions to common problems in software design. They are not specific code implementations but rather templates or blueprints that can be applied to various situations. Design patterns help developers create more efficient, scalable, and maintainable code by following established best practices.
The concept of design patterns was popularized by the “Gang of Four” (GoF) – Erich Gamma, Richard Helm, Ralph Johnson, and John Vlissides – in their seminal book “Design Patterns: Elements of Reusable Object-Oriented Software.” While the original patterns were focused on object-oriented programming, the concept has since expanded to cover various programming paradigms and architectural styles.
Categories of Design Patterns
Design patterns are typically categorized into three main groups:
1. Creational Patterns
Creational patterns deal with object creation mechanisms, trying to create objects in a manner suitable to the situation. These patterns abstract the instantiation process, making a system independent of how its objects are created, composed, and represented.
Examples of creational patterns include:
- Singleton
- Factory Method
- Abstract Factory
- Builder
- Prototype
2. Structural Patterns
Structural patterns focus on how classes and objects are composed to form larger structures. These patterns help ensure that when one part of a system changes, the entire structure doesn’t need to change.
Examples of structural patterns include:
- Adapter
- Bridge
- Composite
- Decorator
- Facade
- Flyweight
- Proxy
3. Behavioral Patterns
Behavioral patterns are concerned with algorithms and the assignment of responsibilities between objects. They describe not just patterns of objects or classes but also the patterns of communication between them.
Examples of behavioral patterns include:
- Observer
- Strategy
- Command
- State
- Chain of Responsibility
- Mediator
- Iterator
When to Use Design Patterns
While design patterns offer numerous benefits, it’s crucial to understand when and how to apply them effectively. Here are some guidelines for using design patterns:
1. When Solving Common Design Problems
Design patterns are most useful when you encounter problems that have been solved many times before. If you’re facing a common design challenge, chances are there’s a pattern that addresses it. For example:
- Use the Singleton pattern when you need to ensure that a class has only one instance and provide a global point of access to it.
- Apply the Observer pattern when you have a one-to-many dependency between objects, where multiple objects need to be notified when one object changes state.
- Implement the Strategy pattern when you want to define a family of algorithms, encapsulate each one, and make them interchangeable.
2. When Improving Code Flexibility and Maintainability
Design patterns can significantly enhance the flexibility and maintainability of your code. Use them when you want to:
- Decouple components of your system to reduce dependencies
- Make your code more modular and easier to extend
- Improve the readability and understandability of your codebase
3. When Communicating Design Ideas
Design patterns provide a common vocabulary for developers to discuss and document software designs. Use them when you want to:
- Communicate complex design concepts more easily with your team
- Document the architecture of your system in a standardized way
- Onboard new team members more quickly by referring to well-known patterns
4. When Refactoring Legacy Code
Design patterns can be particularly useful when refactoring legacy code. Use them when you want to:
- Improve the structure of existing code without changing its external behavior
- Introduce more flexibility into a rigid codebase
- Reduce code duplication and improve overall code quality
Examples of Design Patterns in Action
Let’s explore some common design patterns and see how they can be implemented in practice.
Singleton Pattern
The Singleton pattern ensures a class has only one instance and provides a global point of access to it. This is useful for managing shared resources or coordinating system-wide actions.
Here’s a simple implementation of the Singleton pattern in Python:
class Singleton:
_instance = None
def __new__(cls):
if cls._instance is None:
cls._instance = super().__new__(cls)
return cls._instance
def some_business_logic(self):
# ...
# Usage
s1 = Singleton()
s2 = Singleton()
print(s1 is s2) # Output: True
Factory Method Pattern
The Factory Method pattern provides an interface for creating objects in a superclass, but allows subclasses to alter the type of objects that will be created. This is useful when a class can’t anticipate the type of objects it needs to create.
Here’s an example of the Factory Method pattern in Java:
interface Product {
void operation();
}
class ConcreteProductA implements Product {
public void operation() {
System.out.println("ConcreteProductA operation");
}
}
class ConcreteProductB implements Product {
public void operation() {
System.out.println("ConcreteProductB operation");
}
}
abstract class Creator {
public abstract Product factoryMethod();
public void someOperation() {
Product product = factoryMethod();
product.operation();
}
}
class ConcreteCreatorA extends Creator {
public Product factoryMethod() {
return new ConcreteProductA();
}
}
class ConcreteCreatorB extends Creator {
public Product factoryMethod() {
return new ConcreteProductB();
}
}
// Usage
Creator creator = new ConcreteCreatorA();
creator.someOperation(); // Output: ConcreteProductA operation
creator = new ConcreteCreatorB();
creator.someOperation(); // Output: ConcreteProductB operation
Observer Pattern
The Observer pattern defines a one-to-many dependency between objects so that when one object changes state, all its dependents are notified and updated automatically. This is useful for implementing distributed event handling systems.
Here’s an example of the Observer pattern in JavaScript:
class Subject {
constructor() {
this.observers = [];
}
addObserver(observer) {
this.observers.push(observer);
}
removeObserver(observer) {
const index = this.observers.indexOf(observer);
if (index > -1) {
this.observers.splice(index, 1);
}
}
notifyObservers(data) {
for (let observer of this.observers) {
observer.update(data);
}
}
}
class Observer {
update(data) {
console.log(`Received update: ${data}`);
}
}
// Usage
const subject = new Subject();
const observer1 = new Observer();
const observer2 = new Observer();
subject.addObserver(observer1);
subject.addObserver(observer2);
subject.notifyObservers("Hello, observers!");
// Output:
// Received update: Hello, observers!
// Received update: Hello, observers!
Best Practices for Using Design Patterns
While design patterns are powerful tools, it’s important to use them judiciously. Here are some best practices to keep in mind:
1. Understand the Problem First
Before applying a design pattern, make sure you fully understand the problem you’re trying to solve. Don’t force a pattern onto a problem just because you’re familiar with it. The pattern should naturally fit the problem at hand.
2. Keep It Simple
Always strive for simplicity in your designs. If a simpler solution works just as well, prefer it over a more complex design pattern. Remember, the goal is to solve problems efficiently, not to showcase your knowledge of patterns.
3. Consider the Trade-offs
Every design pattern comes with its own set of trade-offs. For example, while the Singleton pattern provides a global point of access, it can make unit testing more difficult. Always weigh the benefits against the potential drawbacks before implementing a pattern.
4. Combine Patterns When Appropriate
Often, the best solutions come from combining multiple patterns. For example, you might use the Factory Method pattern to create objects, and then use the Observer pattern to notify other parts of your system when these objects change state.
5. Document Your Use of Patterns
When you implement a design pattern, make sure to document it clearly in your code comments or design documentation. This helps other developers (including your future self) understand the reasoning behind your design choices.
6. Stay Updated
Design patterns evolve over time, and new patterns emerge to address modern software development challenges. Stay updated with the latest trends and best practices in software design.
Common Pitfalls to Avoid
While design patterns are valuable tools, there are some common pitfalls to be aware of:
1. Overuse of Patterns
Don’t try to fit design patterns into every aspect of your code. Overusing patterns can lead to unnecessary complexity and reduced readability. Use patterns where they provide clear benefits.
2. Misapplying Patterns
Using the wrong pattern for a given problem can lead to more issues than it solves. Make sure you understand both the problem and the pattern thoroughly before implementation.
3. Ignoring the Context
Design patterns are not one-size-fits-all solutions. Always consider the specific context of your project, including performance requirements, scalability needs, and team expertise.
4. Premature Optimization
Don’t apply complex patterns in anticipation of future needs that may never materialize. Start with simple designs and refactor to patterns when the need becomes clear.
5. Neglecting SOLID Principles
Design patterns should be used in conjunction with, not instead of, fundamental design principles like SOLID (Single Responsibility, Open-Closed, Liskov Substitution, Interface Segregation, and Dependency Inversion).
Conclusion
Design patterns are powerful tools in a developer’s arsenal, offering tested solutions to common software design problems. By understanding various design patterns and when to apply them, you can create more robust, flexible, and maintainable software systems.
Remember that design patterns are guidelines, not strict rules. They should be adapted to fit your specific needs and used in conjunction with other software design principles and best practices. As you gain experience, you’ll develop a better intuition for when and how to apply these patterns effectively.
Continuous learning and practice are key to mastering design patterns. Experiment with different patterns in your projects, analyze their impact, and learn from both successes and failures. Over time, you’ll build a deep understanding of how to leverage design patterns to create elegant and efficient software solutions.
By incorporating design patterns into your development process, you’ll not only improve the quality of your code but also enhance your ability to communicate complex design ideas and solve challenging software engineering problems. As you progress in your coding journey, design patterns will become invaluable tools in your quest to become a more effective and proficient developer.