Understanding Databases: SQL vs. NoSQL – A Comprehensive Guide
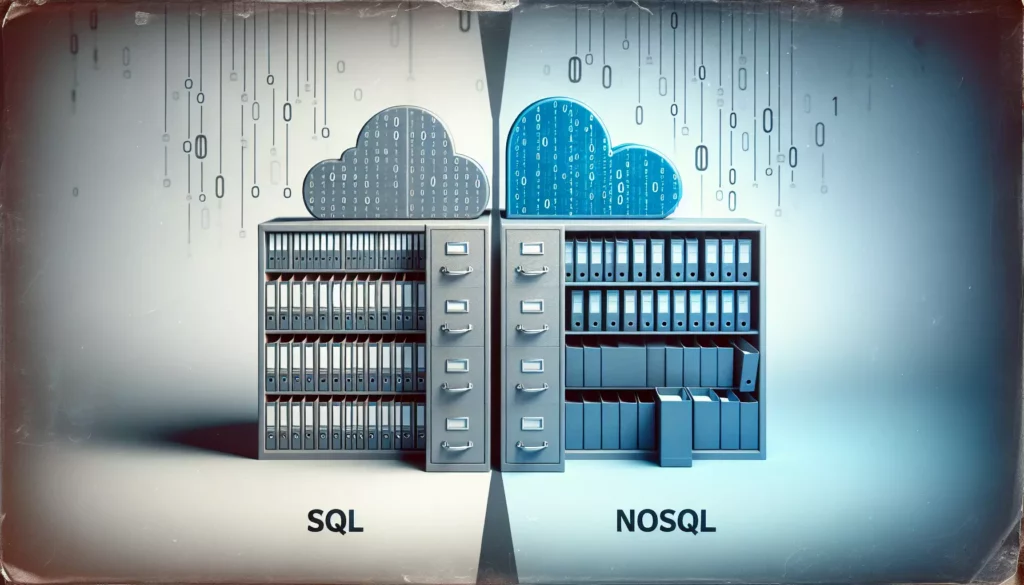
In the world of software development and data management, choosing the right database system is crucial for the success of your project. Two major categories of databases dominate the landscape: SQL (Structured Query Language) and NoSQL (Not Only SQL). Each has its strengths, weaknesses, and ideal use cases. In this comprehensive guide, we’ll dive deep into the world of SQL and NoSQL databases, exploring their differences, advantages, and when to use each type.
Table of Contents
- Introduction to Databases
- SQL Databases
- NoSQL Databases
- Key Differences Between SQL and NoSQL
- Use Cases for SQL and NoSQL Databases
- Performance Comparison
- Scalability Considerations
- Data Modeling in SQL and NoSQL
- Query Languages and Data Manipulation
- ACID Properties and Consistency Models
- Popular SQL and NoSQL Databases
- Choosing Between SQL and NoSQL
- Conclusion
1. Introduction to Databases
Before we dive into the specifics of SQL and NoSQL databases, let’s start with a brief introduction to databases in general. A database is an organized collection of data stored and accessed electronically. Databases are used to store, retrieve, and manage data efficiently, and they play a crucial role in modern software applications.
The two main categories of databases we’ll be discussing are:
- SQL Databases: Also known as relational databases, these use structured query language (SQL) for defining and manipulating data.
- NoSQL Databases: These are non-relational databases designed for distributed data stores with humongous data storage needs.
2. SQL Databases
SQL databases have been the go-to choice for data storage and management for decades. They are based on the relational model, which organizes data into tables with rows and columns. Each table represents an entity, and relationships between entities are established through foreign keys.
Key Features of SQL Databases:
- Structured Data: Data is organized into tables with predefined schemas.
- ACID Compliance: Ensures data validity and integrity through Atomicity, Consistency, Isolation, and Durability.
- Powerful Querying: SQL provides a standardized language for complex queries and data manipulation.
- Joins: Allows efficient combining of data from multiple tables.
- Normalization: Reduces data redundancy and improves data integrity.
Example of SQL Table Creation:
CREATE TABLE users (
id INT PRIMARY KEY,
username VARCHAR(50) NOT NULL,
email VARCHAR(100) UNIQUE,
created_at TIMESTAMP DEFAULT CURRENT_TIMESTAMP
);
This SQL statement creates a table named “users” with columns for id, username, email, and creation timestamp.
3. NoSQL Databases
NoSQL databases emerged as a response to the limitations of traditional SQL databases, especially when dealing with large-scale, distributed systems and unstructured data. They offer more flexibility in terms of data models and are often used in big data and real-time web applications.
Key Features of NoSQL Databases:
- Flexible Schema: Allows for storing unstructured and semi-structured data.
- Scalability: Designed for horizontal scaling across multiple servers.
- High Performance: Optimized for specific data models and access patterns.
- Eventual Consistency: Sacrifices strong consistency for better availability and partition tolerance.
- Variety of Data Models: Includes document, key-value, wide-column, and graph databases.
Example of NoSQL Document Creation (MongoDB):
db.users.insertOne({
username: "johndoe",
email: "john@example.com",
created_at: new Date(),
preferences: {
theme: "dark",
notifications: true
}
})
This MongoDB command inserts a new document into the “users” collection with nested data structures.
4. Key Differences Between SQL and NoSQL
Understanding the fundamental differences between SQL and NoSQL databases is crucial for making informed decisions about which type to use for your projects. Here are the main distinctions:
1. Data Model
- SQL: Rigid, predefined schema with tables and relationships.
- NoSQL: Flexible schema that can adapt to changing data requirements.
2. Scalability
- SQL: Vertically scalable (scaling up by adding more power to existing hardware).
- NoSQL: Horizontally scalable (scaling out by adding more servers to handle increased load).
3. ACID Compliance
- SQL: Strictly ACID compliant, ensuring data integrity and consistency.
- NoSQL: Some databases sacrifice ACID compliance for performance and scalability.
4. Query Language
- SQL: Uses standardized SQL for querying and manipulating data.
- NoSQL: Each database may have its own query language or API.
5. Join Operations
- SQL: Supports complex JOIN operations between tables.
- NoSQL: Typically doesn’t support JOINs; data is often denormalized.
5. Use Cases for SQL and NoSQL Databases
Choosing between SQL and NoSQL databases depends on your specific use case and requirements. Here are some common scenarios where each type excels:
SQL Database Use Cases:
- Financial systems and transactions
- E-commerce platforms with complex product relationships
- Content management systems with structured content
- Applications requiring complex queries and reporting
- Systems with strong consistency requirements
NoSQL Database Use Cases:
- Real-time big data applications
- Content delivery networks
- IoT and sensor data storage
- Social networks and user profiles
- Gaming applications with high write loads
6. Performance Comparison
Performance is a critical factor when choosing a database system. Both SQL and NoSQL databases can offer high performance, but their strengths lie in different areas:
SQL Database Performance:
- Excels at complex queries involving multiple tables
- Optimized for read-heavy workloads with proper indexing
- Maintains consistency even under high concurrency
- May struggle with extremely high write loads
NoSQL Database Performance:
- Designed for high-speed, simple read/write operations
- Excels at handling large volumes of unstructured data
- Can offer better write performance for certain use cases
- May sacrifice some read performance for scalability
It’s important to note that performance can vary greatly depending on the specific database implementation, hardware, and workload. Always benchmark your specific use case when making performance comparisons.
7. Scalability Considerations
Scalability is the ability of a database to handle growing amounts of data and user load. SQL and NoSQL databases approach scalability differently:
SQL Database Scalability:
- Vertical Scaling: Increase the power of a single server (CPU, RAM, SSD).
- Replication: Create read replicas to distribute read loads.
- Sharding: Partition data across multiple databases (complex to implement).
NoSQL Database Scalability:
- Horizontal Scaling: Add more servers to a cluster to distribute load.
- Auto-sharding: Automatically distribute data across nodes.
- Elastic Scalability: Easily add or remove nodes as needed.
NoSQL databases are often favored for applications that require handling large amounts of data or high write throughput, as they can scale out more easily than traditional SQL databases.
8. Data Modeling in SQL and NoSQL
Data modeling is the process of creating a conceptual representation of data structures and relationships. The approach to data modeling differs significantly between SQL and NoSQL databases:
SQL Data Modeling:
- Uses Entity-Relationship (ER) diagrams
- Focuses on normalization to reduce data redundancy
- Defines relationships between tables (one-to-one, one-to-many, many-to-many)
- Requires careful planning of table schemas
Example SQL Data Model:
CREATE TABLE customers (
id INT PRIMARY KEY,
name VARCHAR(100),
email VARCHAR(100)
);
CREATE TABLE orders (
id INT PRIMARY KEY,
customer_id INT,
order_date DATE,
FOREIGN KEY (customer_id) REFERENCES customers(id)
);
NoSQL Data Modeling:
- Focuses on query patterns and data access
- Often denormalizes data for performance
- Allows for nested structures within documents
- Adapts to changing data requirements more easily
Example NoSQL Data Model (MongoDB):
{
"_id": ObjectId("5f8a7b2b9d3e7a1c9c8b4567"),
"name": "John Doe",
"email": "john@example.com",
"orders": [
{
"id": "ORD001",
"date": ISODate("2023-04-15T10:30:00Z"),
"items": [
{ "product": "Widget A", "quantity": 2 },
{ "product": "Gadget B", "quantity": 1 }
]
}
]
}
9. Query Languages and Data Manipulation
The way you interact with and manipulate data in SQL and NoSQL databases can be quite different:
SQL Query Language:
- Uses standardized SQL for querying and data manipulation
- Supports complex JOIN operations and subqueries
- Provides powerful aggregation and grouping functions
- Allows for transaction management with BEGIN, COMMIT, and ROLLBACK
Example SQL Query:
SELECT c.name, COUNT(o.id) as order_count
FROM customers c
LEFT JOIN orders o ON c.id = o.customer_id
GROUP BY c.id
HAVING order_count > 5
ORDER BY order_count DESC;
NoSQL Query Languages:
- Vary depending on the database type (e.g., MongoDB query language, Cassandra CQL)
- Often use JSON-like syntax for querying document databases
- May provide specific APIs for different data models (e.g., graph traversal for graph databases)
- Some NoSQL databases support SQL-like query languages for familiarity
Example NoSQL Query (MongoDB):
db.customers.aggregate([
{ $lookup: {
from: "orders",
localField: "_id",
foreignField: "customer_id",
as: "orders"
}},
{ $project: {
name: 1,
order_count: { $size: "$orders" }
}},
{ $match: { order_count: { $gt: 5 } }},
{ $sort: { order_count: -1 }}
])
10. ACID Properties and Consistency Models
ACID properties (Atomicity, Consistency, Isolation, Durability) are a set of guarantees that ensure database transactions are processed reliably. SQL and NoSQL databases handle these properties differently:
SQL Databases and ACID:
- Strictly adhere to ACID properties
- Ensure strong consistency across all nodes
- Provide robust transaction support
- May sacrifice some performance for consistency
NoSQL Databases and CAP Theorem:
NoSQL databases often follow the CAP theorem, which states that a distributed system can only provide two out of three guarantees: Consistency, Availability, and Partition tolerance.
- Many NoSQL databases prioritize availability and partition tolerance over strict consistency
- Implement eventual consistency models
- Some NoSQL databases offer tunable consistency levels
- May provide ACID guarantees at a single-document level
11. Popular SQL and NoSQL Databases
Let’s look at some of the most popular database systems in both categories:
Popular SQL Databases:
- MySQL: Open-source, widely used for web applications
- PostgreSQL: Advanced open-source database with strong feature set
- Oracle: Enterprise-grade database with extensive features
- Microsoft SQL Server: Comprehensive database platform for Windows environments
- SQLite: Lightweight, serverless database engine
Popular NoSQL Databases:
- MongoDB: Document-oriented database with flexible schema
- Cassandra: Wide-column store designed for high scalability
- Redis: In-memory data structure store used as a database, cache, and message broker
- Couchbase: Distributed multi-model NoSQL document database
- Neo4j: Graph database optimized for highly connected data
12. Choosing Between SQL and NoSQL
Selecting the right database type for your project depends on various factors. Here are some guidelines to help you make an informed decision:
Choose SQL when:
- Your data has a clear, unchanging structure
- You need complex queries and transactions
- Data integrity is crucial (e.g., financial applications)
- You have relational data with many foreign key relationships
- You need strong consistency guarantees
Choose NoSQL when:
- You have large volumes of unstructured or semi-structured data
- You need high write throughput
- You require horizontal scalability for big data
- Your application needs real-time data with low latency
- You’re working with rapidly changing data models
Consider a Multi-Model Approach:
In some cases, using both SQL and NoSQL databases in a single application can provide the best of both worlds. This approach, known as polyglot persistence, allows you to leverage the strengths of each database type for different parts of your application.
13. Conclusion
Both SQL and NoSQL databases have their place in modern software development. SQL databases excel in handling structured data with complex relationships and providing strong consistency guarantees. They are ideal for applications that require complex queries and transactions, such as financial systems and traditional business applications.
On the other hand, NoSQL databases shine in scenarios involving large volumes of unstructured or semi-structured data, high write loads, and the need for horizontal scalability. They are well-suited for real-time big data applications, content delivery networks, and systems that require flexible data models.
As a developer or architect, it’s crucial to understand the strengths and weaknesses of both SQL and NoSQL databases. By carefully considering your project’s requirements, data model, scalability needs, and consistency requirements, you can make an informed decision that will set your application up for success.
Remember that the database landscape is continually evolving, with new features and hybrid solutions emerging. Stay informed about the latest developments in database technologies to ensure you’re always choosing the best solution for your specific use case.