Understanding Database Indexes and Their Importance in Modern Software Development
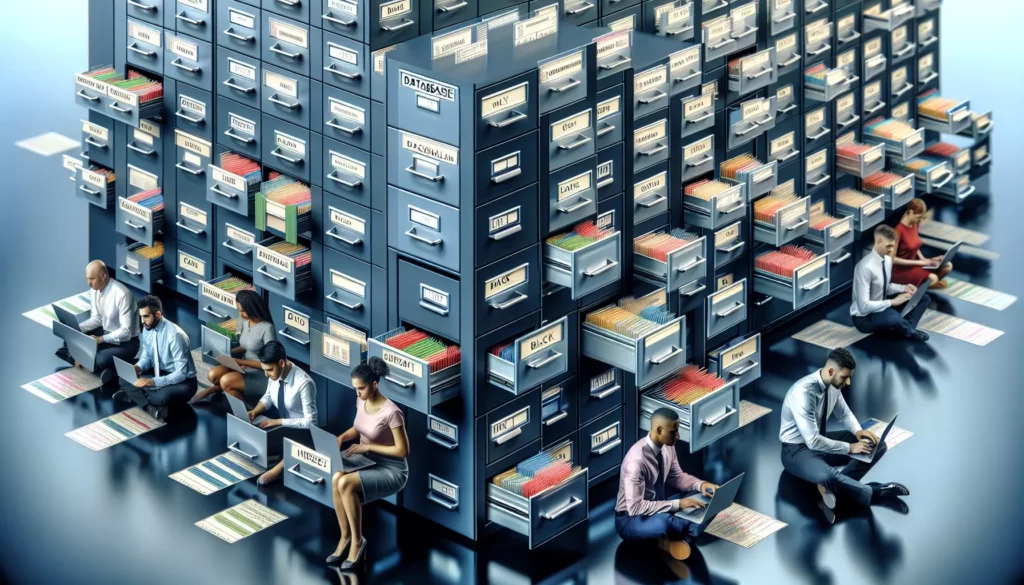
In the world of software development and database management, efficiency is key. As applications grow in complexity and data volumes increase exponentially, the need for optimized database performance becomes crucial. This is where database indexes come into play. In this comprehensive guide, we’ll explore what database indexes are, why they’re important, and how they can significantly improve the performance of your applications.
What Are Database Indexes?
Database indexes are data structures that improve the speed of data retrieval operations on a database table. They work similarly to the index at the back of a book, allowing the database engine to quickly locate and access the data without having to scan the entire table.
An index is essentially a copy of selected columns of data from a table, organized in a way that allows for rapid searching and sorting. When you create an index on a column (or set of columns), the database management system maintains a separate structure that contains the indexed column(s) along with a pointer to the corresponding row in the table.
Types of Database Indexes
There are several types of database indexes, each suited for different scenarios:
1. Single-Column Indexes
These are the simplest form of indexes, created on a single column of a table. They’re useful when you frequently search or sort by that specific column.
2. Composite Indexes
Also known as multi-column indexes, these are created on two or more columns of a table. They’re beneficial when you often query using multiple columns in combination.
3. Unique Indexes
These ensure that no two rows in a table have the same value in the indexed column(s). They’re often used to enforce data integrity and uniqueness constraints.
4. Clustered Indexes
A clustered index determines the physical order of data in a table. Each table can have only one clustered index. In SQL Server, the primary key of a table is automatically a clustered index unless specified otherwise.
5. Non-Clustered Indexes
These indexes maintain a separate structure from the data, containing the indexed columns and a pointer to the table rows. A table can have multiple non-clustered indexes.
The Importance of Database Indexes
Now that we understand what database indexes are, let’s explore why they’re so important in modern software development:
1. Improved Query Performance
The primary benefit of indexes is significantly faster data retrieval. Without indexes, the database engine would need to perform a full table scan for every query, which can be extremely slow for large tables. Indexes allow the engine to quickly locate the relevant rows, dramatically reducing query execution time.
2. Efficient Sorting
Indexes can also speed up sorting operations. When a query includes an ORDER BY clause on an indexed column, the database can use the index to retrieve the data in the desired order without performing an expensive sort operation.
3. Enforcing Constraints
Unique indexes help enforce data integrity by ensuring that no duplicate values are inserted into the indexed column(s). This is particularly useful for implementing primary keys and unique constraints.
4. Optimizing Join Operations
When tables are joined, having indexes on the join columns can significantly improve the performance of these operations, especially for large tables.
5. Supporting FOREIGN KEY Constraints
Indexes are automatically created on columns that are part of a FOREIGN KEY constraint, which helps maintain referential integrity and improves the performance of operations involving these constraints.
When to Use Database Indexes
While indexes offer numerous benefits, it’s important to use them judiciously. Here are some scenarios where creating an index is typically beneficial:
- Columns that are frequently used in WHERE clauses
- Columns used in JOIN operations
- Columns used in ORDER BY or GROUP BY clauses
- Columns with a high degree of uniqueness (many distinct values)
- Large tables where retrieving a small percentage of rows is common
When to Avoid Database Indexes
Despite their benefits, indexes aren’t always the best solution. Here are some situations where you might want to reconsider using an index:
- Small tables where a full table scan is fast enough
- Columns with low cardinality (few distinct values)
- Columns that are frequently updated, as maintaining the index can become costly
- Tables that are frequently subject to large batch update or insert operations
Implementing Database Indexes
The syntax for creating indexes varies slightly between different database management systems, but the general concept remains the same. Here are examples of how to create indexes in some popular databases:
SQL Server
CREATE INDEX idx_lastname
ON Employees (LastName);
MySQL
CREATE INDEX idx_lastname
ON Employees (LastName);
PostgreSQL
CREATE INDEX idx_lastname
ON Employees (LastName);
Oracle
CREATE INDEX idx_lastname
ON Employees (LastName);
To create a composite index:
CREATE INDEX idx_lastname_firstname
ON Employees (LastName, FirstName);
Best Practices for Using Database Indexes
To get the most out of database indexes, consider the following best practices:
1. Index Selectivity
Choose columns with high selectivity (many unique values) for indexing. Indexes on columns with low selectivity (few unique values) are less effective.
2. Avoid Over-Indexing
While indexes improve read performance, they can slow down write operations. Each index needs to be updated when the data changes, so having too many indexes can negatively impact insert, update, and delete operations.
3. Consider the Order of Columns in Composite Indexes
In a composite index, the order of columns matters. Place the most frequently used columns first, followed by less frequently used ones.
4. Monitor and Maintain Indexes
Regularly review the usage and performance of your indexes. Remove unused indexes and consider rebuilding or reorganizing fragmented indexes.
5. Use Covering Indexes
A covering index includes all the columns needed to execute a query. This can significantly improve performance by avoiding the need to access the table data.
6. Be Mindful of Index Size
Indexes consume storage space and memory. Be cautious when indexing large columns like TEXT or BLOB fields.
The Impact of Indexes on Database Performance
To illustrate the impact of indexes on database performance, let’s consider a simple example. Imagine we have a table called ‘Customers’ with millions of records:
CREATE TABLE Customers (
CustomerID INT PRIMARY KEY,
FirstName VARCHAR(50),
LastName VARCHAR(50),
Email VARCHAR(100),
City VARCHAR(50)
);
Now, let’s say we frequently run queries to find customers by their last name:
SELECT * FROM Customers WHERE LastName = 'Smith';
Without an index on the LastName column, the database would need to perform a full table scan, checking every single row to find matches. This could take several seconds or even minutes, depending on the table size.
If we create an index on the LastName column:
CREATE INDEX idx_lastname ON Customers (LastName);
The same query could now execute in milliseconds. The database can use the index to quickly locate the rows with the LastName ‘Smith’ without scanning the entire table.
Analyzing Index Performance
Most database management systems provide tools to analyze query performance and index usage. Here are some common techniques:
1. EXPLAIN Plans
The EXPLAIN command (or its equivalent) shows how the database plans to execute a query. It can reveal whether indexes are being used and how many rows the database expects to process.
2. Query Execution Time
Comparing the execution time of queries before and after adding an index can provide a clear picture of the performance improvement.
3. Index Usage Statistics
Many databases maintain statistics on how often each index is used. This information can help identify unused or rarely used indexes that might be candidates for removal.
Advanced Indexing Techniques
As you become more comfortable with basic indexing, you might want to explore some advanced techniques:
1. Filtered Indexes
In some databases, you can create an index on a subset of rows that match a specific condition. This can be useful when you frequently query for a particular subset of data.
2. Indexed Views
Some databases allow you to create indexes on views, which can dramatically improve the performance of complex queries involving joins or aggregations.
3. Full-Text Indexes
For efficient searching of text data, many databases offer specialized full-text indexes that support complex text-based queries.
4. Spatial Indexes
For geographic or geometric data, spatial indexes can significantly improve the performance of location-based queries.
The Role of Indexes in Modern Application Development
In today’s world of big data and high-performance applications, understanding and effectively using database indexes is crucial for developers. Whether you’re building a small web application or a large-scale enterprise system, proper indexing can be the difference between a sluggish, unresponsive application and one that delivers lightning-fast results.
As data volumes continue to grow, the importance of efficient data retrieval becomes even more critical. Cloud-based databases and distributed systems add another layer of complexity, making optimized indexing strategies essential for maintaining performance at scale.
Conclusion
Database indexes are powerful tools that can dramatically improve the performance of your applications. By allowing for faster data retrieval, efficient sorting, and improved join operations, indexes play a crucial role in modern software development.
However, it’s important to remember that indexes are not a one-size-fits-all solution. They require careful consideration and ongoing maintenance to ensure they’re providing the maximum benefit. By understanding the principles behind database indexes and following best practices, you can significantly enhance the performance and scalability of your database-driven applications.
As you continue your journey in software development, keep in mind that effective use of database indexes is a key skill that can set you apart in technical interviews and real-world projects. Whether you’re preparing for a job at a major tech company or building your own applications, mastering the art of database indexing will serve you well throughout your career.