Understanding Data Types: Primitives, Objects, and More
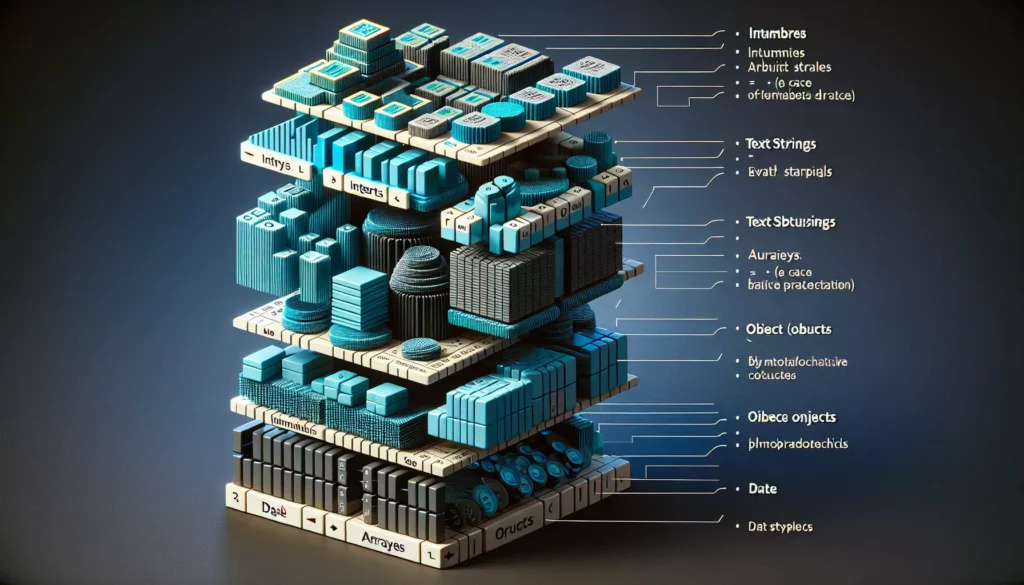
In the world of programming, data types are fundamental building blocks that determine how we store, manipulate, and process information. Whether you’re a beginner just starting your coding journey or an experienced developer preparing for technical interviews at top tech companies, a solid understanding of data types is crucial. In this comprehensive guide, we’ll dive deep into the realm of data types, exploring primitives, objects, and more, to help you build a strong foundation for your programming skills.
1. Introduction to Data Types
Before we delve into the specifics, let’s start with a basic definition: A data type is a classification that specifies which type of value a variable can hold. Data types are essential because they:
- Determine how much memory is allocated for a variable
- Define what operations can be performed on the data
- Influence how the data is stored and retrieved from memory
- Help prevent errors by ensuring that the right kind of data is used in the right context
In most programming languages, data types can be broadly categorized into two main groups: primitive data types and non-primitive (or reference) data types.
2. Primitive Data Types
Primitive data types are the most basic data types available in programming languages. They are typically built-in or predefined by the language and are used to store simple values of a particular type. Let’s explore some common primitive data types:
2.1 Integer (int)
Integers are whole numbers without any decimal points. They can be positive, negative, or zero. For example:
int age = 25;
int temperature = -10;
int count = 0;
The range of integers depends on the programming language and the number of bits used to store them. In many languages, integers are typically 32 or 64 bits long.
2.2 Floating-Point (float/double)
Floating-point numbers are used to represent real numbers with decimal points. There are typically two types:
- float: Single-precision floating-point (usually 32 bits)
- double: Double-precision floating-point (usually 64 bits)
Example:
float pi = 3.14159f;
double e = 2.71828;
Doubles are more precise than floats but require more memory.
2.3 Boolean (bool)
Boolean data type represents logical values and can only have two possible states: true or false. Booleans are often used in conditional statements and loops. For example:
bool isRaining = true;
bool hasPassedExam = false;
2.4 Character (char)
The character data type is used to store a single character, which can be a letter, digit, or special symbol. Characters are typically enclosed in single quotes. For instance:
char grade = 'A';
char symbol = '$';
2.5 Byte
A byte is a very small integer, typically 8 bits long. It can store values from -128 to 127 (or 0 to 255 for unsigned bytes). Bytes are often used when working with binary data or when memory conservation is crucial. Example:
byte smallNumber = 42;
3. Non-Primitive (Reference) Data Types
Non-primitive data types, also known as reference types, are more complex than primitive types. They are used to store collections of data or more complex structures. Let’s explore some common non-primitive data types:
3.1 String
Although strings are often treated as a primitive type in many high-level languages, they are actually objects composed of an array of characters. Strings are used to store and manipulate text. For example:
String greeting = "Hello, World!";
String name = "Alice";
Strings often come with built-in methods for manipulation, such as concatenation, substring extraction, and searching.
3.2 Array
An array is a collection of elements of the same data type, stored in contiguous memory locations. Arrays have a fixed size once they are created. Here’s an example of an integer array:
int[] numbers = {1, 2, 3, 4, 5};
String[] fruits = {"apple", "banana", "orange"};
Arrays are zero-indexed, meaning the first element is accessed with index 0.
3.3 List
Lists are similar to arrays but are typically dynamic in size, allowing for easy addition and removal of elements. In many languages, lists are implemented as classes or objects. For example, in Java:
import java.util.ArrayList;
ArrayList<String> todoList = new ArrayList<>();
todoList.add("Buy groceries");
todoList.add("Finish homework");
todoList.add("Call mom");
3.4 Set
A set is an unordered collection of unique elements. Sets are useful when you need to ensure that no duplicates exist in your data. Here’s an example in Python:
unique_numbers = {1, 2, 3, 4, 5}
unique_numbers.add(6) # Adds 6 to the set
unique_numbers.add(1) # Does nothing, as 1 is already in the set
3.5 Map (or Dictionary)
Maps, also known as dictionaries in some languages, store key-value pairs. They provide fast lookups and are excellent for representing relationships between data. Here’s an example in JavaScript:
let person = {
"name": "John Doe",
"age": 30,
"city": "New York"
};
console.log(person["name"]); // Outputs: John Doe
3.6 Object
Objects are instances of classes and can contain both data (in the form of properties) and code (in the form of methods). They are the foundation of object-oriented programming. Here’s a simple example in Java:
public class Car {
String brand;
String model;
int year;
public void startEngine() {
System.out.println("The " + brand + " " + model + " is starting.");
}
}
Car myCar = new Car();
myCar.brand = "Toyota";
myCar.model = "Corolla";
myCar.year = 2022;
myCar.startEngine(); // Outputs: The Toyota Corolla is starting.
4. Special Data Types
In addition to the primitive and non-primitive data types, there are some special data types worth mentioning:
4.1 Null
Null represents the intentional absence of any object value. It’s often used to indicate that a variable has no value or that an object reference doesn’t point to any object. For example, in Java:
String emptyString = null;
if (emptyString == null) {
System.out.println("The string is null");
}
4.2 Undefined
In some languages like JavaScript, undefined is a primitive value automatically assigned to variables that have just been declared or to formal arguments for which there are no actual arguments. For instance:
let x;
console.log(x); // Outputs: undefined
4.3 Pointer
Pointers are variables that store memory addresses. They’re commonly used in languages like C and C++ for direct memory manipulation. Here’s an example in C:
int number = 42;
int* ptr = &number; // ptr now holds the memory address of number
printf("%d", *ptr); // Outputs: 42
5. Type Systems and Type Checking
Understanding data types also involves grasping the concepts of type systems and type checking. These mechanisms help ensure that operations performed on data are valid and consistent.
5.1 Static vs. Dynamic Typing
Programming languages can be categorized based on when type checking occurs:
- Static Typing: Types are checked at compile-time. Languages like Java, C++, and Rust use static typing.
- Dynamic Typing: Types are checked at runtime. Languages like Python, JavaScript, and Ruby use dynamic typing.
Here’s an example to illustrate the difference:
// Java (Static Typing)
int number = 5;
number = "Hello"; // Compile-time error
# Python (Dynamic Typing)
number = 5
number = "Hello" # No error, perfectly valid
5.2 Strong vs. Weak Typing
This categorization refers to how strictly types are distinguished:
- Strong Typing: The language prevents “type coercion” or implicit type conversion. Python is an example of a strongly typed language.
- Weak Typing: The language may perform implicit type conversion. JavaScript is an example of a weakly typed language.
Example:
# Python (Strong Typing)
print("5" + 5) # Raises a TypeError
// JavaScript (Weak Typing)
console.log("5" + 5); // Outputs: "55" (string concatenation)
6. Type Conversion and Casting
Sometimes, you need to convert data from one type to another. This process is known as type conversion or casting.
6.1 Implicit Type Conversion (Coercion)
This occurs automatically when an operation involves different data types. For example, in JavaScript:
let result = 5 + "5";
console.log(result); // Outputs: "55" (number 5 is converted to a string)
6.2 Explicit Type Conversion (Casting)
This is when you manually convert a value from one type to another. Here’s an example in Java:
double pi = 3.14159;
int roundedPi = (int) pi; // Explicitly cast double to int
System.out.println(roundedPi); // Outputs: 3
7. Data Types in Different Programming Languages
While the concepts of data types are universal, their implementation can vary across programming languages. Let’s look at some examples:
7.1 Python
Python is dynamically typed and supports the following main data types:
- int, float, complex
- str
- list, tuple, range
- dict
- set, frozenset
- bool
- bytes, bytearray, memoryview
7.2 Java
Java is statically typed and has the following primitive data types:
- byte, short, int, long
- float, double
- boolean
- char
Java also has reference types like String, arrays, and user-defined classes.
7.3 JavaScript
JavaScript is dynamically typed and has the following data types:
- Number
- String
- Boolean
- Undefined
- Null
- Object (including arrays and functions)
- Symbol (added in ES6)
8. Advanced Topics in Data Types
8.1 Generics
Generics allow you to write code that can work with any data type while still providing compile-time type safety. They’re particularly useful in statically-typed languages. Here’s an example in Java:
public class Box<T> {
private T content;
public void set(T content) {
this.content = content;
}
public T get() {
return content;
}
}
Box<Integer> intBox = new Box<>();
intBox.set(10);
int value = intBox.get(); // No casting needed
8.2 Union Types
Union types allow a value to be one of several types. They’re common in TypeScript and some other modern languages. For example:
let result: number | string;
result = 42; // Valid
result = "hello"; // Also valid
result = true; // Error: Type 'boolean' is not assignable to type 'number | string'
8.3 Algebraic Data Types
Algebraic Data Types (ADTs) are composite types, often found in functional programming languages. They come in two main flavors:
- Sum Types (OR): A type that can be one of several things. Similar to union types.
- Product Types (AND): A type that contains multiple fields. Similar to structs or classes.
Here’s an example in Haskell:
data Shape = Circle Float | Rectangle Float Float
area :: Shape -> Float
area (Circle r) = pi * r * r
area (Rectangle w h) = w * h
9. Data Types and Memory Management
Understanding data types is crucial for efficient memory management and optimization. Different data types occupy different amounts of memory:
- A boolean typically uses 1 byte
- An integer often uses 4 bytes (32 bits) or 8 bytes (64 bits)
- A double usually uses 8 bytes
- The size of objects and arrays depends on their content and the specific implementation
When working with large datasets or memory-constrained environments, choosing appropriate data types can significantly impact performance and resource usage.
10. Conclusion
Data types are the foundation of programming, influencing how we structure and manipulate data in our code. From simple primitives to complex objects, understanding data types is crucial for writing efficient, bug-free code and excelling in technical interviews.
As you continue your journey in programming and prepare for interviews with top tech companies, remember that a solid grasp of data types will serve you well. Practice working with different data types, understand their nuances in various programming languages, and always consider the most appropriate data type for your specific use case.
By mastering data types, you’ll not only write better code but also gain a deeper understanding of how computers store and process information. This knowledge will be invaluable as you tackle complex algorithms, optimize performance, and build robust software systems.
Keep exploring, keep coding, and let your understanding of data types be the strong foundation upon which you build your programming expertise!