Understanding Data Structures: The Backbone of Efficient Code
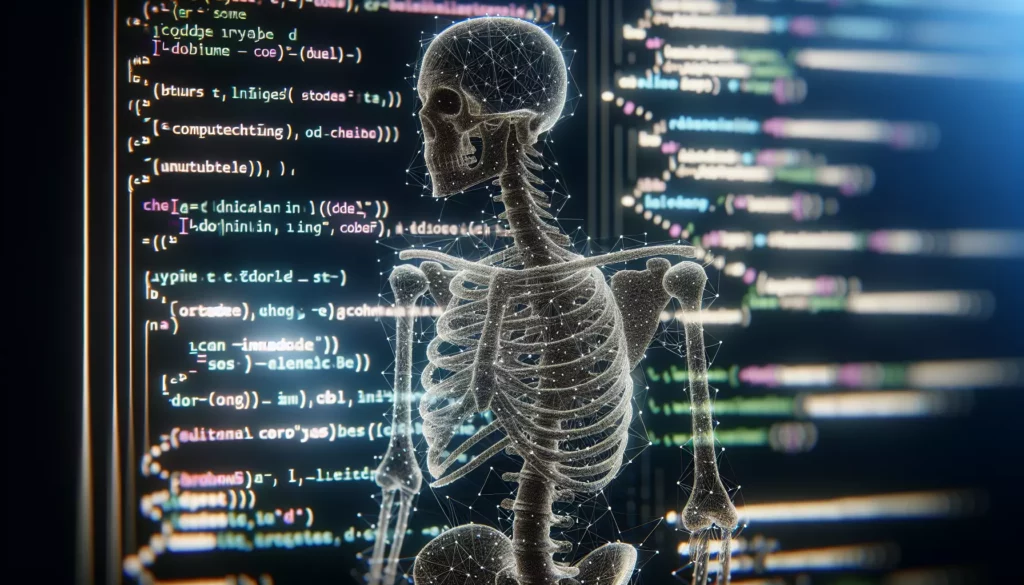
In the world of programming, data structures serve as the fundamental building blocks that enable efficient organization, storage, and manipulation of data. Whether you’re a beginner coder or preparing for technical interviews at major tech companies, a solid understanding of data structures is crucial for writing optimized and scalable code. In this comprehensive guide, we’ll dive deep into the world of data structures, exploring their importance, types, and practical applications in solving complex programming problems.
Why Data Structures Matter
Before we delve into specific data structures, it’s essential to understand why they are so important in the realm of computer science and software development:
- Efficiency: Proper use of data structures can significantly improve the time and space complexity of algorithms, leading to faster and more resource-efficient programs.
- Organization: Data structures provide a systematic way to organize and store data, making it easier to access, modify, and analyze information.
- Abstraction: They offer a level of abstraction that allows developers to focus on solving problems rather than worrying about low-level implementation details.
- Reusability: Many data structures are implemented as reusable components, saving time and effort in software development.
- Problem-solving: Different data structures are suited for different types of problems, and knowing when to use each one is key to effective problem-solving in programming.
Common Types of Data Structures
Let’s explore some of the most commonly used data structures in programming:
1. Arrays
Arrays are one of the simplest and most fundamental data structures. They store elements of the same data type in contiguous memory locations, allowing for constant-time access to individual elements using an index.
Key characteristics:
- Fixed size (in most programming languages)
- Constant-time access to elements (O(1))
- Efficient for random access
- Poor for insertion and deletion in the middle of the array
Example (Python):
numbers = [1, 2, 3, 4, 5]
print(numbers[2]) # Output: 3
2. Linked Lists
Linked lists consist of nodes, where each node contains a data element and a reference (or link) to the next node in the sequence. Unlike arrays, linked lists do not require contiguous memory allocation.
Key characteristics:
- Dynamic size
- Efficient insertion and deletion at the beginning (O(1))
- Linear-time access to elements (O(n))
- More memory overhead due to storage of references
Example (Python):
class Node:
def __init__(self, data):
self.data = data
self.next = None
class LinkedList:
def __init__(self):
self.head = None
def append(self, data):
new_node = Node(data)
if not self.head:
self.head = new_node
return
current = self.head
while current.next:
current = current.next
current.next = new_node
# Usage
linked_list = LinkedList()
linked_list.append(1)
linked_list.append(2)
linked_list.append(3)
3. Stacks
Stacks follow the Last-In-First-Out (LIFO) principle, where the last element added is the first one to be removed. They are often used in scenarios involving function calls, expression evaluation, and backtracking algorithms.
Key characteristics:
- Push and pop operations in constant time (O(1))
- Only the top element is accessible
- Useful for implementing undo functionality and parsing expressions
Example (Python):
class Stack:
def __init__(self):
self.items = []
def push(self, item):
self.items.append(item)
def pop(self):
if not self.is_empty():
return self.items.pop()
def is_empty(self):
return len(self.items) == 0
# Usage
stack = Stack()
stack.push(1)
stack.push(2)
stack.push(3)
print(stack.pop()) # Output: 3
4. Queues
Queues operate on the First-In-First-Out (FIFO) principle, where the first element added is the first one to be removed. They are commonly used in scenarios involving task scheduling, breadth-first search algorithms, and managing resources in a system.
Key characteristics:
- Enqueue and dequeue operations in constant time (O(1))
- Elements are added at the rear and removed from the front
- Useful for managing tasks in a specific order
Example (Python):
from collections import deque
class Queue:
def __init__(self):
self.items = deque()
def enqueue(self, item):
self.items.append(item)
def dequeue(self):
if not self.is_empty():
return self.items.popleft()
def is_empty(self):
return len(self.items) == 0
# Usage
queue = Queue()
queue.enqueue(1)
queue.enqueue(2)
queue.enqueue(3)
print(queue.dequeue()) # Output: 1
5. Trees
Trees are hierarchical data structures consisting of nodes connected by edges. They are widely used in various applications, including file systems, database indexing, and expression parsing.
Key characteristics:
- Hierarchical structure with a root node and child nodes
- Efficient for searching, inserting, and deleting elements
- Various types include binary trees, binary search trees, and balanced trees
Example (Python – Binary Search Tree):
class TreeNode:
def __init__(self, value):
self.value = value
self.left = None
self.right = None
class BinarySearchTree:
def __init__(self):
self.root = None
def insert(self, value):
if not self.root:
self.root = TreeNode(value)
else:
self._insert_recursive(self.root, value)
def _insert_recursive(self, node, value):
if value < node.value:
if node.left is None:
node.left = TreeNode(value)
else:
self._insert_recursive(node.left, value)
else:
if node.right is None:
node.right = TreeNode(value)
else:
self._insert_recursive(node.right, value)
# Usage
bst = BinarySearchTree()
bst.insert(5)
bst.insert(3)
bst.insert(7)
bst.insert(1)
bst.insert(9)
6. Graphs
Graphs are versatile data structures that consist of vertices (nodes) connected by edges. They are used to represent complex relationships and networks, such as social networks, computer networks, and transportation systems.
Key characteristics:
- Flexible structure for representing relationships between entities
- Can be directed (edges have a direction) or undirected
- Useful for solving problems like shortest path, connectivity, and network flow
Example (Python – Adjacency List):
class Graph:
def __init__(self):
self.graph = {}
def add_edge(self, u, v):
if u not in self.graph:
self.graph[u] = []
if v not in self.graph:
self.graph[v] = []
self.graph[u].append(v)
self.graph[v].append(u)
def print_graph(self):
for vertex in self.graph:
print(f"{vertex}: {' '.join(map(str, self.graph[vertex]))}")
# Usage
graph = Graph()
graph.add_edge(0, 1)
graph.add_edge(0, 2)
graph.add_edge(1, 2)
graph.add_edge(2, 3)
graph.print_graph()
# Output:
# 0: 1 2
# 1: 0 2
# 2: 0 1 3
# 3: 2
7. Hash Tables
Hash tables, also known as hash maps or dictionaries, provide efficient key-value pair storage and retrieval. They use a hash function to compute an index where a value is stored or retrieved.
Key characteristics:
- Constant-time average case for insertion, deletion, and lookup (O(1))
- Efficient for implementing associative arrays and caches
- May require handling of collisions (when two keys hash to the same index)
Example (Python – Using built-in dict):
hash_table = {}
hash_table['key1'] = 'value1'
hash_table['key2'] = 'value2'
hash_table['key3'] = 'value3'
print(hash_table['key2']) # Output: value2
print('key4' in hash_table) # Output: False
Choosing the Right Data Structure
Selecting the appropriate data structure for a given problem is crucial for writing efficient and maintainable code. Here are some factors to consider when choosing a data structure:
- Operations required: Consider the primary operations you’ll be performing on the data (e.g., insertion, deletion, search, traversal).
- Time complexity: Evaluate the time complexity of different operations for each data structure and choose the one that best fits your performance requirements.
- Space complexity: Consider the memory usage of the data structure, especially for large datasets or memory-constrained environments.
- Data organization: Think about how the data needs to be organized and accessed (e.g., sequential, hierarchical, key-value pairs).
- Flexibility: Consider whether you need a fixed-size structure or one that can dynamically grow and shrink.
- Implementation complexity: Weigh the trade-offs between using a simpler data structure that may be easier to implement versus a more complex one that offers better performance.
Data Structures in Technical Interviews
Understanding data structures is crucial for succeeding in technical interviews, especially at major tech companies. Here are some tips for leveraging your knowledge of data structures during interviews:
- Practice implementation: Be prepared to implement basic data structures from scratch, as this demonstrates a deep understanding of their inner workings.
- Analyze trade-offs: When presented with a problem, discuss the pros and cons of using different data structures to solve it.
- Optimize solutions: Use your knowledge of data structures to optimize the time and space complexity of your solutions.
- Explain your reasoning: Clearly articulate why you chose a particular data structure for a given problem.
- Be familiar with library implementations: Know how to use built-in data structures in your preferred programming language efficiently.
- Solve classic problems: Practice solving common algorithmic problems that heavily rely on data structures, such as graph traversals, tree balancing, and hash table collision resolution.
Advanced Data Structures
As you progress in your coding journey, you may encounter more advanced data structures that are optimized for specific use cases. Some examples include:
- Trie: An efficient tree-like structure for storing and searching strings, often used in autocomplete and spell-checking applications.
- Heap: A specialized tree-based structure that satisfies the heap property, useful for implementing priority queues and sorting algorithms.
- Segment Tree: A tree data structure used for storing information about intervals or segments, allowing for efficient range queries and updates.
- Disjoint Set (Union-Find): A data structure that keeps track of a set of elements partitioned into disjoint subsets, useful for solving connectivity problems in graphs.
- Bloom Filter: A space-efficient probabilistic data structure used to test whether an element is a member of a set, with a small probability of false positives.
Understanding these advanced data structures can give you an edge in solving complex problems and optimizing performance in specific scenarios.
Conclusion
Data structures are the backbone of efficient code and form an essential part of every programmer’s toolkit. By mastering various data structures and understanding their strengths and weaknesses, you’ll be better equipped to tackle a wide range of programming challenges, from everyday coding tasks to complex algorithm design.
As you continue your journey in coding education and skill development, remember that proficiency in data structures is not just about memorizing implementations. It’s about developing the intuition to recognize which structure is best suited for a given problem and understanding how to leverage their properties to create elegant and efficient solutions.
Whether you’re preparing for technical interviews at major tech companies or simply aiming to become a more proficient programmer, investing time in understanding and practicing data structures will undoubtedly pay off in the long run. Keep coding, keep learning, and don’t hesitate to explore the fascinating world of data structures beyond what we’ve covered in this guide!