Understanding Data Structures and Algorithms: A Complete Guide
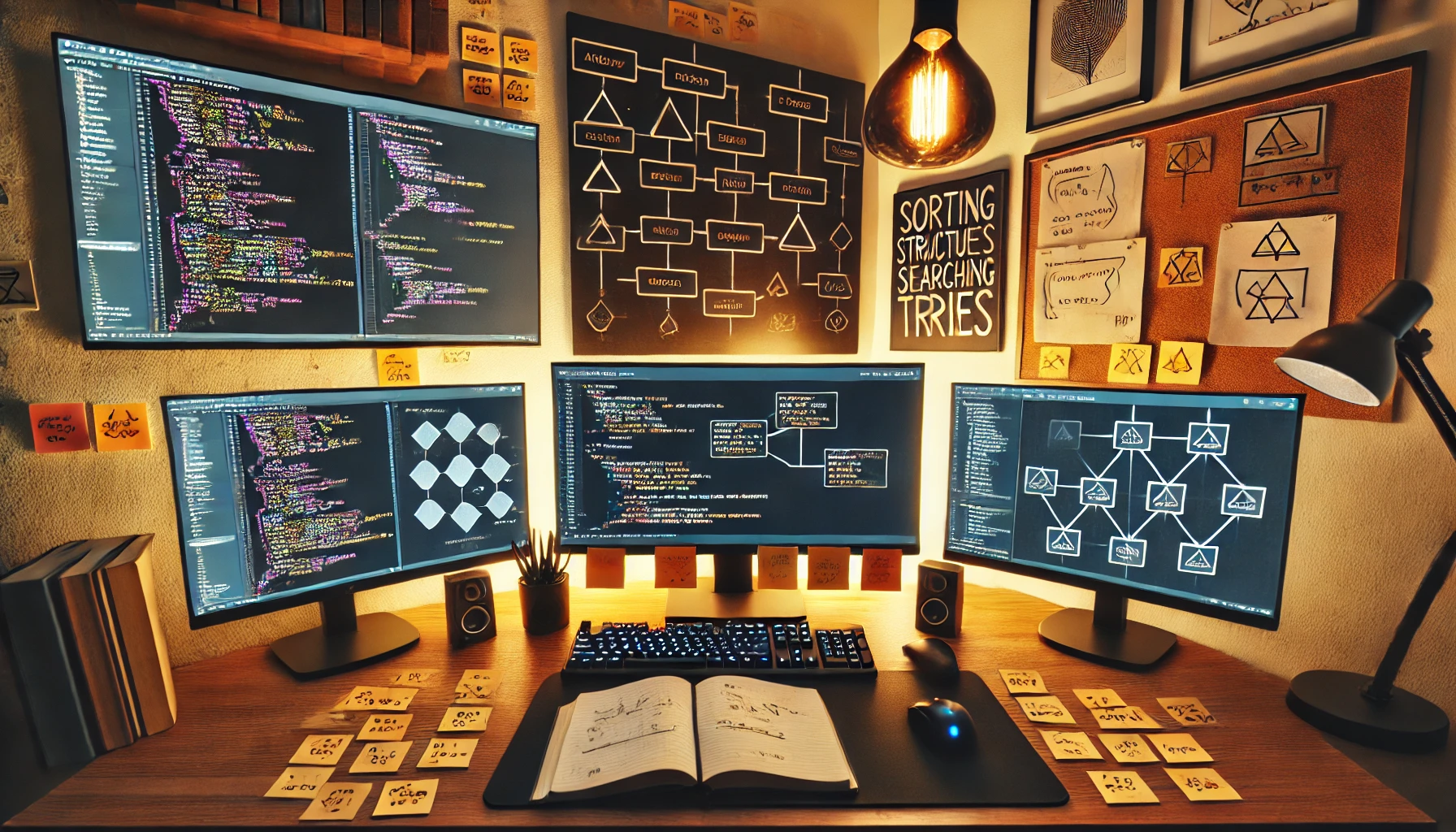
Data structures and algorithms are fundamental concepts in computer science. They help us organize and manipulate data efficiently, making our code faster and more effective. Understanding these concepts is crucial for anyone looking to improve their programming skills, prepare for technical interviews, or compete in coding challenges.
Key Takeaways
- Data structures organize data in a way that makes it easy to access and modify.
- Algorithms are step-by-step instructions for solving specific problems.
- Common data structures include arrays, linked lists, stacks, queues, trees, and graphs.
- Understanding time and space complexity helps in analyzing the efficiency of algorithms.
- Mastering data structures and algorithms is essential for technical interviews and competitive programming.
Fundamental Concepts of Data Structures and Algorithms
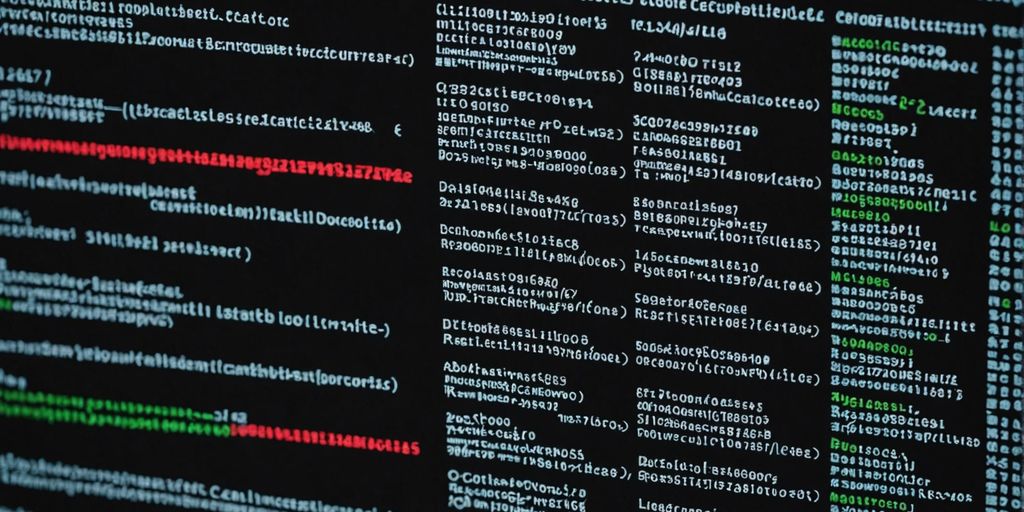
Definition and Importance
Data structures are the fundamental building blocks of computer programming. They define how data is organized, stored, and manipulated within a program. Algorithms, on the other hand, are sets of steps for solving specific problems. Together, they form the backbone of efficient coding and problem-solving.
Basic Terminology
Understanding the basic terminology is crucial:
- Data Structure: A way to organize and store data.
- Algorithm: A set of steps to solve a problem.
- Array: A collection of elements identified by index or key.
- Linked List: A sequence of elements, each pointing to the next.
- Stack: A collection of elements with Last In, First Out (LIFO) access.
- Queue: A collection of elements with First In, First Out (FIFO) access.
- Tree: A hierarchical structure with nodes.
- Graph: A set of nodes connected by edges.
Mathematical Foundations
Mathematics underpins many concepts in data structures and algorithms. Key areas include:
- Set Theory and Logic: Essential for understanding operations on data structures.
- Probability and Combinatorics: Useful for analyzing algorithm efficiency.
Mastering these fundamental concepts is essential for anyone looking to excel in computer science and software development.
Core Data Structures
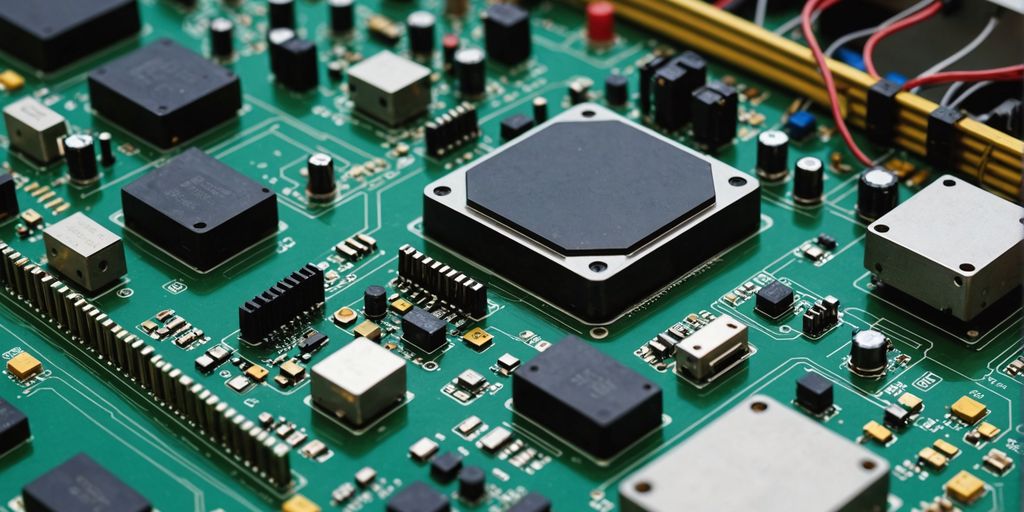
Arrays and Linked Lists
Arrays and linked lists are fundamental data structures used to store collections of items. An array is a linear data structure where elements are stored in contiguous memory locations, allowing for constant-time access. Each element in an array has a unique index number. On the other hand, a linked list stores elements in nodes that are linked using pointers, making it easier to insert and delete elements without reorganizing the entire structure.
Stacks and Queues
Stacks and queues are data structures that follow specific order principles. A stack operates on the Last In, First Out (LIFO) principle, where items are added and removed from the top. Conversely, a queue follows the First In, First Out (FIFO) principle, where items are added at the back and removed from the front. These structures are commonly used in algorithms for managing data.
Trees and Graphs
Trees and graphs are non-linear data structures used to represent hierarchical and network relationships. A tree consists of nodes with a root node and child nodes, forming a tree-like structure. Graphs, however, consist of vertices (or nodes) and edges that connect them, representing relationships between entities. These structures are essential for tasks like network analysis and route planning.
Hash Tables
A hash table is a data structure that allows for quick data storage and retrieval. It uses a hash function to map data to specific indices in an array. When data is inserted, the hash function calculates the index, and the data is placed at that index. To retrieve data, the same hash function is used to find the index, making hash tables very efficient for tasks like implementing sets and maps.
Essential Algorithms
Sorting Algorithms
Sorting algorithms are used to arrange data in a specific order, such as ascending or descending. Some common sorting algorithms include:
- Quick Sort: Efficient for large datasets, uses a divide-and-conquer approach.
- Merge Sort: Stable and works well with linked lists.
- Heap Sort: Utilizes a binary heap data structure.
- Insertion Sort: Simple but inefficient for large datasets.
Searching Algorithms
Searching algorithms help find specific data within a collection. Key examples are:
- Binary Search: Efficient for sorted arrays, works by repeatedly dividing the search interval in half.
- Linear Search: Simple but less efficient, checks each element one by one.
Graph Algorithms
Graph algorithms are essential for solving problems related to graphs. Important algorithms include:
- Breadth-First Search (BFS): Explores nodes level by level.
- Depth-First Search (DFS): Explores as far as possible along each branch before backtracking.
- Dijkstra’s Algorithm: Finds the shortest path between nodes in a graph.
Dynamic Programming
Dynamic programming is a technique for solving complex problems by breaking them down into simpler subproblems. Key concepts include:
- Knapsack Problem: Determines the most valuable combination of items that fit within a given weight limit.
- Fibonacci Sequence: Calculates the nth Fibonacci number efficiently.
Mastering these essential algorithms is crucial for any programmer. They form the backbone of efficient problem-solving and coding.
Analyzing Algorithm Efficiency
Time Complexity
Time complexity tells us how long an algorithm takes to run as the input size grows. We want algorithms to be as fast as possible. To measure this, we use Big O notation, which gives us an upper limit on the running time. For example, an algorithm with O(n) time complexity grows linearly with the input size.
Space Complexity
Space complexity measures how much memory an algorithm uses. Just like with time, we want to use as little memory as possible. Big O notation also helps us here. For instance, an algorithm with O(1) space complexity uses a constant amount of memory, no matter how big the input is.
Big O Notation
Big O notation is a way to describe the performance of an algorithm. It helps us understand the worst, average, and best case analysis of algorithms. Here are some common time complexities:
- O(1): Constant time
- O(log n): Logarithmic time
- O(n): Linear time
- O(n log n): Linearithmic time
- O(n^2): Quadratic time
Understanding these complexities helps us choose the best algorithm for the job. Always aim for the lowest time and space complexity to make your programs run faster and use less memory.
Advanced Topics in Data Structures and Algorithms
Advanced Data Structures
In this section, we explore complex structures that go beyond the basics. These include Tries, Segment Trees, Fenwick Trees, and Disjoint Set Union (DSU). Each of these structures is designed to handle specific problems more efficiently.
- Tries: Useful for tasks like autocomplete and spell checking.
- Segment Trees: Ideal for range query problems.
- Fenwick Trees: Also known as Binary Indexed Trees, used for frequency counting and cumulative frequency tables.
- Disjoint Set Union (DSU): Helps in managing and merging disjoint sets, often used in network connectivity problems.
Complex Algorithms
Here, we delve into algorithms that require a deeper understanding and are often used in specialized fields. These include:
- Graph Algorithms: Advanced algorithms like Dijkstra’s, Bellman-Ford, Floyd-Warshall, and A* for shortest path problems.
- String Algorithms: Pattern matching algorithms such as KMP, Rabin-Karp, and the Z-algorithm for string processing tasks.
- Dynamic Programming: Techniques to solve problems by breaking them down into simpler subproblems, like the Knapsack problem and the Fibonacci sequence.
Real-World Applications
Data structures and algorithms are not just theoretical concepts; they have practical applications in the real world. From search engines to social media platforms, these techniques are used to solve complex problems efficiently.
Understanding these advanced topics is crucial for anyone looking to excel in technical interviews or competitive programming. They are the building blocks for solving real-world coding challenges.
- Search Engines: Use algorithms to index and retrieve data quickly.
- Social Media: Employs data structures to manage and display user information.
- Network Routing: Utilizes graph algorithms to find the shortest path for data packets.
By mastering these advanced topics, you complete your journey in understanding data structures and algorithms (DSA).
Practical Applications and Problem-Solving
Technical Interviews
Technical interviews often focus on your understanding of data structures and algorithms. Mastering these concepts can significantly boost your chances of landing a job. Here are some tips to excel:
- Understand the Basics: Make sure you have a strong grasp of fundamental data structures and algorithms.
- Practice Coding: Regularly solve problems on platforms like LeetCode, HackerRank, and Codeforces.
- Mock Interviews: Participate in mock interviews to get a feel of the real scenario.
- Review and Analyze: After solving problems, review your solutions and understand the time and space complexities.
Competitive Programming
Competitive programming is a great way to improve your problem-solving skills. It involves solving complex problems within a time limit. Here are some steps to get started:
- Choose a Platform: Start with platforms like Codeforces, CodeChef, or AtCoder.
- Learn Algorithms: Focus on learning and implementing various algorithms and data structures.
- Participate in Contests: Regularly participate in online contests to test your skills.
- Analyze Solutions: After contests, review the solutions and learn from your mistakes.
Real-World Coding Challenges
Applying your knowledge to real-world problems can be very rewarding. Here are some ways to do that:
- Work on Projects: Develop projects that solve real-world problems or enhance your portfolio. Examples include recommendation systems, file systems, and personal finance trackers.
- Contribute to Open Source: Join open-source projects to apply your skills, collaborate with others, and contribute to real-world applications.
- Join Study Groups: Collaborate with peers to discuss problems and solutions.
Consistent practice and real-world application of data structures and algorithms can significantly enhance your problem-solving skills and prepare you for various challenges.
Conclusion
In wrapping up, understanding data structures and algorithms is key to becoming a skilled programmer. These concepts help you organize and process data efficiently, making your code faster and more reliable. Whether you’re preparing for a job interview, tackling a coding challenge, or just aiming to improve your skills, mastering data structures and algorithms is essential. Remember, the journey might be tough, but with consistent practice and the right resources, you’ll get there. Keep learning, keep coding, and you’ll see the benefits in no time.
Frequently Asked Questions
What are data structures and algorithms?
Data structures are ways to organize and store data so it can be used efficiently. Algorithms are sets of steps to solve specific problems. Both are key to computer science and help in writing efficient code.
Why are data structures and algorithms important?
They are crucial because they help in solving problems efficiently and effectively. Understanding them can improve coding skills, making it easier to tackle complex tasks and perform well in technical interviews.
What are some common data structures?
Some common data structures include arrays, linked lists, stacks, queues, trees, and graphs. Each has its own strengths and is used for different types of tasks.
What is Big O notation?
Big O notation is a way to describe the efficiency of an algorithm in terms of time and space. It helps in understanding how an algorithm’s performance changes as the input size grows.
How do I start learning data structures and algorithms?
Begin with the basics of programming, then move on to simple data structures like arrays and linked lists. Practice by solving problems and gradually tackle more complex structures and algorithms.
Where can I find resources to learn data structures and algorithms?
There are many resources available, including books like ‘Introduction to Algorithms,’ online courses on platforms like Coursera and Khan Academy, and tutorials on websites like GeeksforGeeks.