Understanding Data Serialization and Deserialization: A Comprehensive Guide
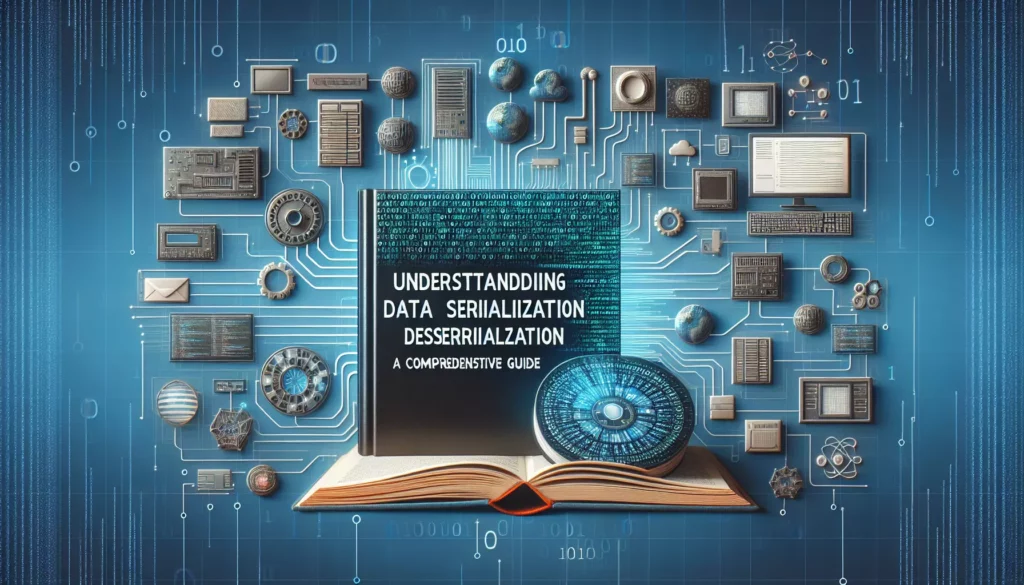
In the world of software development and data processing, the concepts of serialization and deserialization play a crucial role. These processes are fundamental to how data is stored, transmitted, and reconstructed in various applications. Whether you’re a beginner programmer or preparing for technical interviews at major tech companies, understanding these concepts is essential. In this comprehensive guide, we’ll dive deep into data serialization and deserialization, exploring their importance, methods, and practical applications.
What is Data Serialization?
Data serialization is the process of converting complex data structures or object states into a format that can be easily stored, transmitted, or reconstructed later. This format is typically a sequence of bytes or a more human-readable format like JSON or XML. Serialization is crucial when you need to:
- Save the state of an object to a file or database
- Send data over a network
- Store complex data structures in a format that can be easily retrieved
The primary goal of serialization is to preserve the object’s data in a way that allows it to be accurately recreated when needed.
What is Data Deserialization?
Deserialization is the reverse process of serialization. It involves taking serialized data (like a stream of bytes or a JSON string) and reconstructing it back into a complex data structure or object that can be used in your program. Deserialization is essential when you need to:
- Load saved object states from a file or database
- Receive and process data sent over a network
- Convert stored data back into usable program objects
The Importance of Serialization and Deserialization
Understanding and implementing serialization and deserialization is crucial for several reasons:
- Data Persistence: Serialization allows you to save the state of objects, making it possible to store data between program executions.
- Data Transfer: These processes enable the efficient transfer of complex data structures over networks or between different systems.
- Interoperability: Serialization can convert data into standard formats, allowing different systems or programming languages to share and understand the data.
- Caching: Serialized data can be used in caching mechanisms to improve application performance.
- Version Control: Proper serialization techniques can help manage different versions of data structures as your application evolves.
Common Serialization Formats
There are several popular formats used for data serialization. Each has its own advantages and use cases:
1. JSON (JavaScript Object Notation)
JSON is a lightweight, text-based, language-independent data interchange format. It’s widely used for transmitting data objects consisting of attribute–value pairs and array data types.
Example of JSON serialization:
{
"name": "John Doe",
"age": 30,
"city": "New York",
"skills": ["Python", "JavaScript", "SQL"]
}
2. XML (eXtensible Markup Language)
XML is a markup language that defines a set of rules for encoding documents in a format that is both human-readable and machine-readable.
Example of XML serialization:
<person>
<name>John Doe</name>
<age>30</age>
<city>New York</city>
<skills>
<skill>Python</skill>
<skill>JavaScript</skill>
<skill>SQL</skill>
</skills>
</person>
3. Protocol Buffers (protobuf)
Developed by Google, Protocol Buffers is a method of serializing structured data. It’s language-neutral, platform-neutral, and provides a compact binary format.
4. YAML (YAML Ain’t Markup Language)
YAML is a human-friendly data serialization standard that can be used for all programming languages. It’s often used for configuration files.
Example of YAML serialization:
name: John Doe
age: 30
city: New York
skills:
- Python
- JavaScript
- SQL
5. Binary Serialization
Binary serialization converts objects directly into a binary format. This method is typically faster and results in smaller file sizes, but the output is not human-readable.
Serialization in Different Programming Languages
Different programming languages have their own built-in methods and libraries for handling serialization and deserialization. Let’s look at how this is done in some popular languages:
Python Serialization
Python provides several modules for serialization:
- pickle: A Python-specific data serialization module.
- json: For JSON serialization and deserialization.
- marshal: A more low-level serialization module.
Example using pickle:
import pickle
# Serialization
data = {"name": "John", "age": 30, "city": "New York"}
serialized = pickle.dumps(data)
# Deserialization
deserialized = pickle.loads(serialized)
print(deserialized) # Output: {'name': 'John', 'age': 30, 'city': 'New York'}
Java Serialization
Java provides built-in serialization through the Serializable interface:
import java.io.*;
class Person implements Serializable {
private String name;
private int age;
// Constructor, getters, setters...
}
// Serialization
Person person = new Person("John", 30);
FileOutputStream fileOut = new FileOutputStream("person.ser");
ObjectOutputStream out = new ObjectOutputStream(fileOut);
out.writeObject(person);
out.close();
fileOut.close();
// Deserialization
FileInputStream fileIn = new FileInputStream("person.ser");
ObjectInputStream in = new ObjectInputStream(fileIn);
Person deserializedPerson = (Person) in.readObject();
in.close();
fileIn.close();
JavaScript Serialization
In JavaScript, JSON is commonly used for serialization:
// Serialization
const data = { name: "John", age: 30, city: "New York" };
const serialized = JSON.stringify(data);
// Deserialization
const deserialized = JSON.parse(serialized);
console.log(deserialized); // Output: { name: "John", age: 30, city: "New York" }
Best Practices for Serialization and Deserialization
When working with serialization and deserialization, keep these best practices in mind:
- Choose the Right Format: Select a serialization format that best fits your needs in terms of readability, size, and performance.
- Handle Versioning: Implement a versioning system for your serialized data to manage changes in your data structures over time.
- Secure Sensitive Data: Be cautious about serializing sensitive information. Consider encrypting sensitive data before serialization.
- Validate Input: Always validate and sanitize data during deserialization to prevent security vulnerabilities.
- Test Thoroughly: Ensure your serialization and deserialization processes work correctly across different scenarios and edge cases.
- Consider Performance: For large-scale applications, consider the performance implications of your chosen serialization method.
- Use Standard Libraries: Whenever possible, use well-established libraries for serialization to avoid common pitfalls and security issues.
Common Challenges in Serialization and Deserialization
While working with serialization and deserialization, you may encounter several challenges:
1. Circular References
Objects that reference each other in a circular manner can cause issues during serialization. Many serialization libraries provide mechanisms to handle this, such as reference tracking.
2. Large Data Sets
Serializing and deserializing large amounts of data can be time-consuming and memory-intensive. Consider using streaming serialization for large datasets.
3. Cross-Language Compatibility
When working across different programming languages, ensure that your serialization format is supported and interpreted correctly in all target languages.
4. Schema Evolution
As your data structures evolve, you need to ensure that older serialized data can still be deserialized correctly. This often requires careful versioning and backwards compatibility considerations.
5. Security Concerns
Deserialization of untrusted data can lead to security vulnerabilities. Always validate and sanitize data before deserialization.
Advanced Topics in Serialization
Custom Serialization
Sometimes, the default serialization behavior doesn’t meet specific needs. In such cases, you can implement custom serialization methods. For example, in Java:
import java.io.*;
public class CustomPerson implements Serializable {
private String name;
private transient int age; // transient fields are not serialized by default
private void writeObject(ObjectOutputStream out) throws IOException {
out.defaultWriteObject();
out.writeInt(age); // Custom writing of age
}
private void readObject(ObjectInputStream in) throws IOException, ClassNotFoundException {
in.defaultReadObject();
age = in.readInt(); // Custom reading of age
}
}
Serialization in Distributed Systems
In distributed systems, serialization plays a crucial role in data exchange between different components. Technologies like Apache Thrift or gRPC use efficient binary protocols for serialization in these scenarios.
Compression and Encryption
For large datasets or sensitive information, you might need to compress or encrypt your serialized data. This can be done either before serialization or as part of a custom serialization process.
Serialization in Database Operations
Serialization is not just about network communication or file storage. It’s also relevant in database operations, especially when working with object-relational mapping (ORM) tools. ORMs often use serialization techniques to convert complex objects into a format that can be stored in relational databases.
Example: Serialization with SQLAlchemy (Python)
from sqlalchemy import Column, Integer, String, PickleType
from sqlalchemy.ext.declarative import declarative_base
Base = declarative_base()
class User(Base):
__tablename__ = 'users'
id = Column(Integer, primary_key=True)
name = Column(String)
preferences = Column(PickleType) # This column will store serialized data
# Usage
user = User(name="Alice", preferences={"theme": "dark", "notifications": True})
session.add(user)
session.commit()
In this example, the ‘preferences’ column uses PickleType, which automatically serializes and deserializes Python objects.
Serialization in Caching
Caching systems often use serialization to store complex data structures. For instance, when using Redis as a cache:
import redis
import json
r = redis.Redis(host='localhost', port=6379, db=0)
# Serializing and storing data
user_data = {"id": 1, "name": "John", "email": "john@example.com"}
r.set('user:1', json.dumps(user_data))
# Retrieving and deserializing data
stored_user = json.loads(r.get('user:1'))
print(stored_user) # Output: {'id': 1, 'name': 'John', 'email': 'john@example.com'}
Serialization in API Design
When designing APIs, especially RESTful APIs, serialization is used to convert internal data representations into formats suitable for API responses. Libraries like Django Rest Framework in Python provide powerful serialization capabilities:
from rest_framework import serializers
class UserSerializer(serializers.Serializer):
id = serializers.IntegerField(read_only=True)
username = serializers.CharField(max_length=100)
email = serializers.EmailField()
# Usage in a view
user = User.objects.get(id=1)
serializer = UserSerializer(user)
return Response(serializer.data)
Performance Considerations
When working with serialization, especially in performance-critical applications, consider the following:
- Format Choice: Binary formats like Protocol Buffers are generally faster and more compact than text-based formats like JSON or XML.
- Partial Serialization: If you only need part of an object, consider serializing only the necessary fields.
- Caching: For frequently accessed data, consider caching the serialized form to avoid repeated serialization.
- Lazy Loading: For large objects, consider implementing lazy loading of certain fields during deserialization.
Security Implications
Serialization and deserialization can introduce security vulnerabilities if not handled carefully:
- Deserialization of Untrusted Data: This can lead to remote code execution in some cases. Always validate and sanitize data before deserialization.
- Information Disclosure: Be careful not to serialize sensitive information that shouldn’t be exposed.
- Denial of Service: Maliciously crafted serialized data might consume excessive resources during deserialization.
To mitigate these risks:
- Use safe, well-tested serialization libraries.
- Implement strict input validation.
- Consider using signed or encrypted serialized data when working with untrusted sources.
Conclusion
Data serialization and deserialization are fundamental concepts in computer science and software engineering. They play a crucial role in data storage, transmission, and processing across various domains of application development. Understanding these concepts thoroughly is essential for any programmer, especially those preparing for technical interviews at major tech companies.
As you continue to develop your programming skills, remember that effective use of serialization and deserialization can significantly impact the performance, security, and interoperability of your applications. Practice implementing these concepts in different scenarios, explore various serialization formats, and always keep security and performance considerations in mind.
Whether you’re building a simple application or designing complex distributed systems, the principles of serialization and deserialization will be invaluable tools in your programming toolkit. Keep exploring, practicing, and applying these concepts to become a more proficient and versatile developer.