Understanding Data Compression Algorithms: A Comprehensive Guide
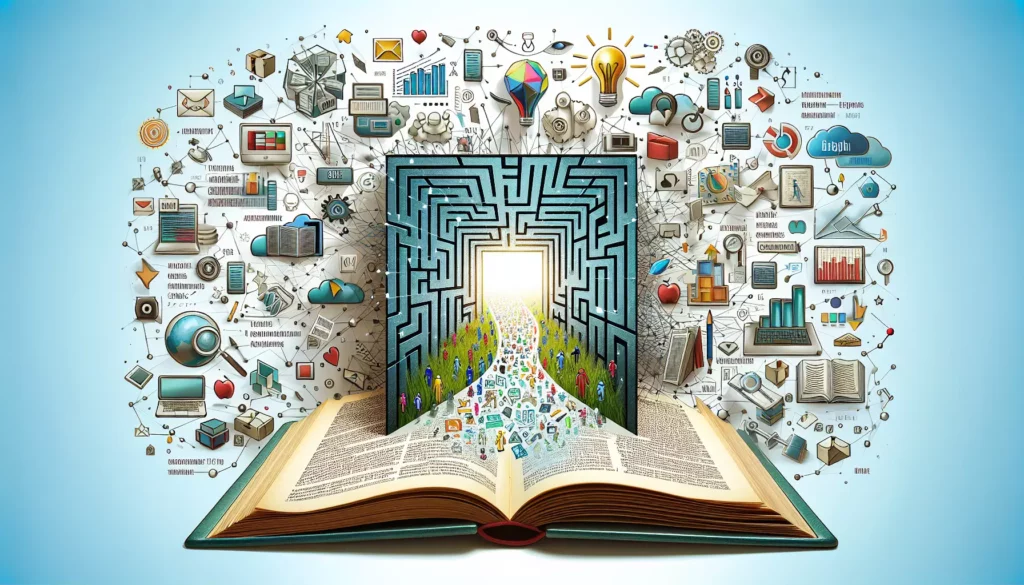
In the vast landscape of computer science and programming, data compression algorithms play a crucial role in optimizing storage and transmission of information. As aspiring developers and coding enthusiasts, understanding these algorithms is not just beneficial—it’s essential. This comprehensive guide will delve into the world of data compression, exploring various techniques, their applications, and how they contribute to efficient data management.
What is Data Compression?
Data compression is the process of encoding information using fewer bits than the original representation. It’s a technique used to reduce the size of data, making it easier to store and transmit. The primary goal of data compression is to minimize redundancy in data, thereby reducing its overall size without losing critical information.
There are two main types of data compression:
- Lossless compression: This method allows the original data to be perfectly reconstructed from the compressed data.
- Lossy compression: This technique reduces file size by permanently eliminating certain information, especially redundant information.
Why is Data Compression Important?
In today’s digital age, where data is generated at an unprecedented rate, compression algorithms are more crucial than ever. Here’s why:
- Reduced storage requirements
- Faster data transmission
- Efficient use of bandwidth
- Lower energy consumption in data centers
- Improved system performance
For developers, understanding these algorithms can lead to more efficient code, better resource management, and improved application performance.
Common Data Compression Algorithms
Let’s explore some of the most widely used data compression algorithms:
1. Run-Length Encoding (RLE)
RLE is one of the simplest forms of data compression. It works by replacing consecutive data elements (runs) with a single data value and count.
Example:
Original: WWWWWWWWWWWWBWWWWWWWWWWWWBBBWWWWWWWWWWWWWWWWWWB
Compressed: 12W1B12W3B24W1B
Python implementation:
def run_length_encode(data):
encoded = []
count = 1
for i in range(1, len(data)):
if data[i] == data[i-1]:
count += 1
else:
encoded.append((count, data[i-1]))
count = 1
encoded.append((count, data[-1]))
return encoded
# Example usage
data = "WWWWWWWWWWWWBWWWWWWWWWWWWBBBWWWWWWWWWWWWWWWWWWB"
print(run_length_encode(data))
# Output: [(12, 'W'), (1, 'B'), (12, 'W'), (3, 'B'), (24, 'W'), (1, 'B')]
2. Huffman Coding
Huffman coding is a popular technique for lossless data compression. It assigns variable-length codes to characters based on their frequencies, with more frequent characters getting shorter codes.
The algorithm works as follows:
- Calculate the frequency of each character in the input.
- Build a binary tree where each leaf represents a character and its weight is the character’s frequency.
- Traverse the tree to assign codes to characters (left child: 0, right child: 1).
Here’s a simple implementation in Python:
import heapq
from collections import defaultdict
class Node:
def __init__(self, char, freq):
self.char = char
self.freq = freq
self.left = None
self.right = None
def __lt__(self, other):
return self.freq < other.freq
def build_huffman_tree(text):
frequency = defaultdict(int)
for char in text:
frequency[char] += 1
heap = [Node(char, freq) for char, freq in frequency.items()]
heapq.heapify(heap)
while len(heap) > 1:
left = heapq.heappop(heap)
right = heapq.heappop(heap)
merged = Node(None, left.freq + right.freq)
merged.left = left
merged.right = right
heapq.heappush(heap, merged)
return heap[0]
def generate_codes(root, current_code, codes):
if root is None:
return
if root.char is not None:
codes[root.char] = current_code
return
generate_codes(root.left, current_code + "0", codes)
generate_codes(root.right, current_code + "1", codes)
def huffman_encoding(text):
root = build_huffman_tree(text)
codes = {}
generate_codes(root, "", codes)
encoded_text = ""
for char in text:
encoded_text += codes[char]
return encoded_text, codes
# Example usage
text = "this is an example for huffman encoding"
encoded_text, codes = huffman_encoding(text)
print("Encoded text:", encoded_text)
print("Huffman codes:", codes)
3. LZW (Lempel-Ziv-Welch) Compression
LZW is a dictionary-based algorithm that builds a dictionary of sequences it has seen before. It’s widely used in formats like GIF and TIFF.
The algorithm works as follows:
- Initialize the dictionary with all possible single-character strings.
- Find the longest string W in the dictionary that matches the current input.
- Output the dictionary index for W and remove W from the input.
- Add W + next character to the dictionary.
- Repeat steps 2-4 until the input is exhausted.
Here’s a Python implementation of LZW compression:
def lzw_compress(data):
dictionary = {chr(i): i for i in range(256)}
result = []
w = ""
code = 256
for c in data:
wc = w + c
if wc in dictionary:
w = wc
else:
result.append(dictionary[w])
dictionary[wc] = code
code += 1
w = c
if w:
result.append(dictionary[w])
return result
# Example usage
data = "TOBEORNOTTOBEORTOBEORNOT"
compressed = lzw_compress(data)
print("Compressed data:", compressed)
4. DEFLATE Algorithm
DEFLATE is a lossless data compression algorithm that combines LZ77 (a sliding window compression algorithm) and Huffman coding. It’s widely used in formats like ZIP and PNG.
The algorithm works in two steps:
- The input data is compressed using LZ77, which replaces repeated occurrences of data with references to a single copy of that data existing earlier in the input data stream.
- The result is then compressed further using Huffman coding.
Implementing DEFLATE from scratch is complex, but here’s a high-level pseudocode:
function deflate(input_data):
# Step 1: LZ77 compression
lz77_compressed = lz77_compress(input_data)
# Step 2: Huffman coding
huffman_compressed = huffman_encode(lz77_compressed)
return huffman_compressed
function lz77_compress(data):
# Implement sliding window and look-ahead buffer
# Replace repeated sequences with (distance, length) pairs
# ...
function huffman_encode(data):
# Build Huffman tree
# Encode data using Huffman codes
# ...
# Usage
compressed_data = deflate(input_data)
Choosing the Right Compression Algorithm
Selecting the appropriate compression algorithm depends on various factors:
- Type of data: Text, images, audio, or video
- Compression ratio required: How much size reduction is needed
- Processing speed: How fast the compression/decompression should be
- Lossless vs. Lossy: Whether data integrity is crucial or some loss is acceptable
For example:
- For text data where every character matters, lossless algorithms like Huffman or LZW are preferred.
- For images where some quality loss is acceptable, lossy algorithms like JPEG can achieve higher compression ratios.
- For real-time applications, faster algorithms like RLE might be more suitable despite potentially lower compression ratios.
Implementing Compression in Your Projects
As a developer, you can implement data compression in your projects in several ways:
1. Using Built-in Libraries
Many programming languages offer built-in compression libraries. For example, in Python:
import zlib
# Compression
data = b"Hello, world!"
compressed = zlib.compress(data)
# Decompression
decompressed = zlib.decompress(compressed)
print(f"Original size: {len(data)}")
print(f"Compressed size: {len(compressed)}")
print(f"Decompressed: {decompressed.decode()}")
2. Implementing Custom Algorithms
For learning purposes or specific requirements, you might implement compression algorithms from scratch. This can be an excellent way to understand the underlying concepts deeply.
3. Using Third-party Libraries
There are numerous third-party libraries available for various compression needs. For instance, in Python, you might use:
bz2
for bzip2 compressionlzma
for LZMA compressiongzip
for gzip compression
Real-world Applications of Data Compression
Data compression finds applications in numerous areas:
- File Compression: ZIP, RAR, 7z formats for reducing file sizes
- Image Compression: JPEG, PNG, WebP formats for efficient image storage and transmission
- Video Compression: MPEG, H.264, H.265 for streaming and storage of video content
- Audio Compression: MP3, AAC for music and podcast distribution
- Database Compression: For reducing storage requirements of large datasets
- Network Protocols: HTTP compression for faster web page loading
- Backup Systems: For efficient storage of backup data
Challenges in Data Compression
While data compression offers numerous benefits, it also comes with challenges:
- Compression-Decompression Time: Higher compression ratios often require more processing time
- Quality Loss in Lossy Compression: Finding the right balance between file size and quality
- Compatibility Issues: Ensuring compressed data can be decompressed on different systems
- Security Concerns: Compressed data can potentially be more vulnerable to certain types of attacks
- Scalability: Handling compression for extremely large datasets or real-time streaming data
Future of Data Compression
The field of data compression continues to evolve. Some exciting areas of research and development include:
- Machine Learning-based Compression: Using AI to develop more efficient compression algorithms
- Quantum Data Compression: Leveraging quantum computing principles for data compression
- Context-aware Compression: Algorithms that adapt based on the specific context or content being compressed
- DNA Data Storage and Compression: Exploring biological systems for data storage and compression
Conclusion
Data compression algorithms are a fundamental aspect of computer science and play a crucial role in our digital world. As a developer, understanding these algorithms can significantly enhance your ability to handle data efficiently, optimize storage, and improve application performance.
Whether you’re working on web applications, mobile apps, data analysis, or any other field of software development, the knowledge of data compression will prove invaluable. It’s not just about saving space—it’s about making your applications faster, more efficient, and capable of handling larger amounts of data.
As you continue your journey in coding and software development, make sure to explore these algorithms in depth. Implement them in your projects, experiment with different techniques, and stay updated with the latest advancements in this field. Remember, in the world of big data and high-speed communications, efficient data compression can be the key to unlocking new possibilities and solving complex challenges.
Happy coding, and may your data always be efficiently compressed!