Understanding Convex Hull Algorithms: A Comprehensive Guide
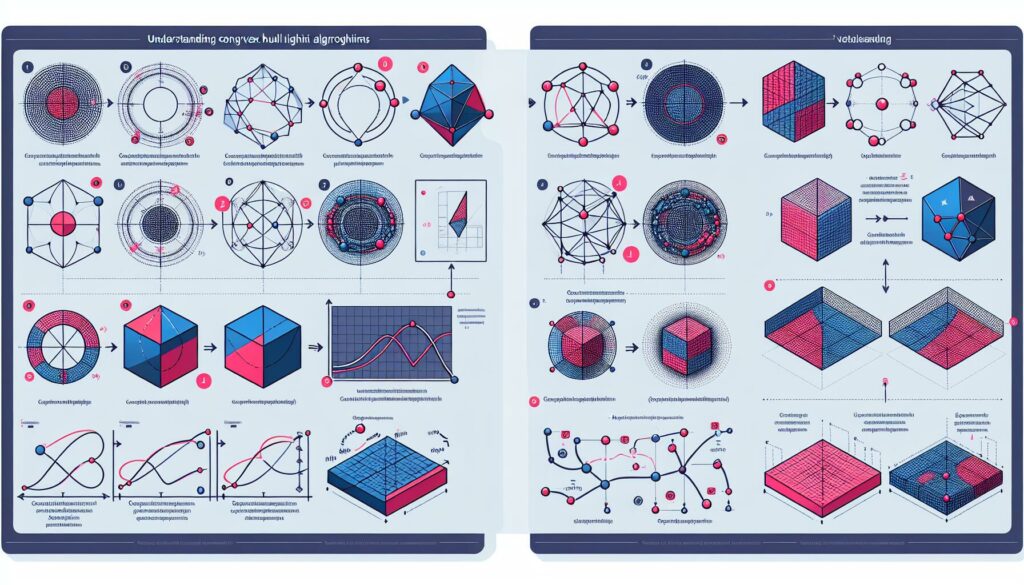
In the world of computational geometry and computer science, the concept of a convex hull is fundamental. It’s a problem that not only has practical applications in various fields but also serves as an excellent example for understanding algorithmic efficiency and geometric problem-solving. In this comprehensive guide, we’ll dive deep into convex hull algorithms, exploring what they are, why they’re important, and how to implement them.
What is a Convex Hull?
Before we delve into the algorithms, let’s first understand what a convex hull is. Imagine a set of points scattered on a plane. The convex hull of this set is the smallest convex polygon that encloses all the points. In simpler terms, if you were to wrap a rubber band around these points, the shape it forms would be the convex hull.
Mathematically, a convex hull can be defined as the intersection of all convex sets containing a given subset of a Euclidean space. For our purposes, we’ll focus on the two-dimensional case, although convex hulls can be computed in higher dimensions as well.
Why are Convex Hulls Important?
Convex hulls have numerous applications across various fields:
- Computer Graphics: Used in shape analysis and collision detection.
- Pattern Recognition: Helps in identifying shapes and clusters in data.
- Geographic Information Systems (GIS): Useful in analyzing geographical data and creating map overlays.
- Robotics: Assists in path planning and obstacle avoidance.
- Data Visualization: Helps in creating bounding boxes and identifying outliers.
Moreover, understanding convex hull algorithms is crucial for anyone preparing for technical interviews, especially at major tech companies. These algorithms demonstrate important concepts in computational geometry and showcase various algorithmic paradigms.
Common Convex Hull Algorithms
There are several algorithms for computing the convex hull of a set of points. We’ll discuss three of the most popular ones: Graham’s Scan, Jarvis March (Gift Wrapping), and QuickHull.
1. Graham’s Scan Algorithm
Graham’s Scan is one of the most efficient algorithms for computing the convex hull, with a time complexity of O(n log n), where n is the number of points.
How it works:
- Find the point with the lowest y-coordinate (and leftmost if there’s a tie).
- Sort the remaining points by polar angle in counterclockwise order around this point.
- Iterate through the sorted points, maintaining a stack of candidate points for the convex hull.
- For each point, while the last three points in the stack make a non-left turn, pop the middle of these three points.
- Push the current point onto the stack.
Here’s a Python implementation of Graham’s Scan:
from math import atan2
def orientation(p, q, r):
return (q[1] - p[1]) * (r[0] - q[0]) - (q[0] - p[0]) * (r[1] - q[1])
def graham_scan(points):
# Find the bottommost point (and leftmost if there are multiple)
bottom_point = min(points, key=lambda p: (p[1], p[0]))
# Sort points based on polar angle with respect to bottom_point
sorted_points = sorted(points, key=lambda p: (atan2(p[1] - bottom_point[1], p[0] - bottom_point[0]), p))
stack = [bottom_point, sorted_points[0]]
for point in sorted_points[1:]:
while len(stack) > 1 and orientation(stack[-2], stack[-1], point) <= 0:
stack.pop()
stack.append(point)
return stack
# Example usage
points = [(0, 3), (2, 2), (1, 1), (2, 1), (3, 0), (0, 0), (3, 3)]
convex_hull = graham_scan(points)
print("Convex Hull:", convex_hull)
2. Jarvis March (Gift Wrapping) Algorithm
The Jarvis March algorithm, also known as the Gift Wrapping algorithm, is conceptually simpler than Graham’s Scan but has a worse time complexity of O(nh), where h is the number of points on the hull.
How it works:
- Start with the leftmost point (point with minimum x-coordinate).
- Do the following until we reach the start point again:
- The current point is point ‘p’
- Find the point ‘q’ such that for any other point ‘r’, ‘q’ is counterclockwise to ‘pr’
- Add ‘q’ to the result as a point on the hull
- Set ‘p’ as ‘q’ for the next iteration
Here’s a Python implementation of the Jarvis March algorithm:
def orientation(p, q, r):
return (q[1] - p[1]) * (r[0] - q[0]) - (q[0] - p[0]) * (r[1] - q[1])
def jarvis_march(points):
n = len(points)
if n < 3:
return points
# Find the leftmost point
l = min(range(n), key=lambda i: points[i][0])
hull = []
p = l
while True:
hull.append(points[p])
q = (p + 1) % n
for i in range(n):
if orientation(points[p], points[i], points[q]) < 0:
q = i
p = q
if p == l:
break
return hull
# Example usage
points = [(0, 3), (2, 2), (1, 1), (2, 1), (3, 0), (0, 0), (3, 3)]
convex_hull = jarvis_march(points)
print("Convex Hull:", convex_hull)
3. QuickHull Algorithm
QuickHull is a divide-and-conquer algorithm that is particularly efficient for datasets with a large number of points inside the hull. Its average-case time complexity is O(n log n), but it can degrade to O(n^2) in the worst case.
How it works:
- Find the points with minimum and maximum x-coordinates, which are always part of the convex hull.
- Use the line formed by these two points to divide the set into two subsets of points on each side of the line.
- Find the point in each subset that is furthest from the line. These points form a triangle with the two points from step 1.
- The points inside this triangle cannot be part of the convex hull and can be ignored in subsequent steps.
- Repeat the process recursively for the two lines formed by the triangle, on the outside of the triangle.
Here’s a Python implementation of the QuickHull algorithm:
def find_side(p1, p2, p):
return (p[1] - p1[1]) * (p2[0] - p1[0]) - (p2[1] - p1[1]) * (p[0] - p1[0])
def line_dist(p1, p2, p):
return abs((p[1] - p1[1]) * (p2[0] - p1[0]) - (p2[1] - p1[1]) * (p[0] - p1[0]))
def quickhull(points):
if len(points) <= 3:
return points
def quick_hull_rec(p1, p2, points, side):
hull = []
max_dist = 0
farthest_point = None
for p in points:
dist = line_dist(p1, p2, p)
if find_side(p1, p2, p) == side and dist > max_dist:
max_dist = dist
farthest_point = p
if farthest_point is None:
return [p1, p2]
hull.extend(quick_hull_rec(p1, farthest_point, points, -find_side(p1, farthest_point, p2)))
hull.extend(quick_hull_rec(farthest_point, p2, points, -find_side(farthest_point, p2, p1)))
return hull
min_x = min(points, key=lambda p: p[0])
max_x = max(points, key=lambda p: p[0])
hull = quick_hull_rec(min_x, max_x, points, 1)
hull.extend(quick_hull_rec(min_x, max_x, points, -1))
return list(set(hull))
# Example usage
points = [(0, 3), (2, 2), (1, 1), (2, 1), (3, 0), (0, 0), (3, 3)]
convex_hull = quickhull(points)
print("Convex Hull:", convex_hull)
Comparing the Algorithms
Each of these algorithms has its strengths and weaknesses:
- Graham’s Scan: Efficient for most cases with O(n log n) time complexity. It’s a good all-around choice.
- Jarvis March: Simple to implement but can be slow for large hulls. It’s efficient when the number of points on the hull is small compared to the total number of points.
- QuickHull: Performs well on average, especially when many points are inside the hull. However, it can degrade to O(n^2) in the worst case.
Practical Considerations
When implementing convex hull algorithms, there are several practical considerations to keep in mind:
1. Numerical Precision
Floating-point arithmetic can lead to precision errors, especially when determining if three points are collinear. To mitigate this, you can use integer coordinates when possible or implement a tolerance threshold for floating-point comparisons.
2. Handling Collinear Points
Decide how to handle collinear points on the hull. Some implementations include all collinear points, while others only include the endpoints.
3. Input Validation
Ensure your implementation can handle edge cases such as all points being collinear or having fewer than three points in the input set.
4. Memory Efficiency
For large datasets, consider implementing the algorithm to work in-place or with minimal additional memory usage.
Advanced Topics
1. Dynamic Convex Hull
In some applications, points may be added or removed dynamically. Algorithms like Chan’s algorithm can maintain a convex hull efficiently under these conditions.
2. 3D Convex Hulls
Extending convex hull algorithms to three dimensions introduces new challenges and algorithms, such as the Gift Wrapping algorithm in 3D or the Quickhull algorithm adapted for 3D space.
3. Approximation Algorithms
For very large datasets or in real-time applications, approximation algorithms that compute a close approximation of the convex hull can be useful.
Conclusion
Understanding convex hull algorithms is crucial for anyone serious about computational geometry and advanced algorithm design. These algorithms showcase important concepts like divide-and-conquer, incremental construction, and geometric primitives.
For those preparing for technical interviews, especially at major tech companies, being able to implement and discuss these algorithms can demonstrate a strong grasp of algorithmic thinking and problem-solving skills. Moreover, the concepts underlying these algorithms often appear in various other computational geometry problems.
As you continue your journey in algorithm design and implementation, remember that convex hull algorithms are just the beginning. They open the door to a fascinating world of computational geometry, with applications ranging from computer graphics to machine learning and beyond.
Practice implementing these algorithms, analyze their performance on different datasets, and explore how they can be applied to real-world problems. This hands-on experience will not only deepen your understanding but also prepare you for the challenges you might face in technical interviews and real-world software development.
Further Reading and Resources
- “Computational Geometry: Algorithms and Applications” by Mark de Berg, Otfried Cheong, Marc van Kreveld, and Mark Overmars
- “Introduction to Algorithms” by Thomas H. Cormen, Charles E. Leiserson, Ronald L. Rivest, and Clifford Stein
- Online platforms like LeetCode and HackerRank for practicing implementation and solving related problems
- Research papers on advanced convex hull algorithms and their applications in various fields
By mastering convex hull algorithms and understanding their underlying principles, you’ll be well-equipped to tackle a wide range of geometric problems and advance your skills in algorithm design and implementation. Happy coding!